package ui;
import java.awt.Color;
import java.awt.Container;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.util.ArrayList;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.Timer;
import data.ApplyNode;
import data.DataDefine;
import data.DataInfo;
import elevatorThread.Elevaltor;
public class MainFrame extends JFrame{
public Elevaltor[] elevaltors=new Elevaltor[4];
public JPanel[][] panels = new JPanel[DataDefine.totolFloors + 1][8];
public JLabel[] labels = new JLabel[DataDefine.totolFloors];
public JTextField[][] textFields = new JTextField[DataDefine.totolFloors][4];
public JButton[] ups = new JButton[DataDefine.totolFloors];
public JButton[] downs = new JButton[DataDefine.totolFloors];
public JButton[] flags = new JButton[DataDefine.totolFloors];
private JLabel[] titles = new JLabel[8];
public MainFrame(){
for(int i=0;i<4;i++){
elevaltors[i]=new Elevaltor(i);
}
DataInfo.directions[0] = -1;///////////////////////
DataInfo.directions[1] = 1;
DataInfo.directions[2] = 1;
DataInfo.directions[3] = 1;
//初始位置都置为最底层
DataInfo.positions[0][0] = 1;
DataInfo.positions[DataDefine.totolFloors-1][1] = 1;
DataInfo.positions[DataDefine.totolFloors-1][2] = 1;
DataInfo.positions[DataDefine.totolFloors-1][3] = 1;
// this.elevaltors[0].addADes(new ApplyNode(0, 0));
this.initTitles();
this.init();
Container contentPane = this.getContentPane();
contentPane.setLayout(new GridLayout(DataDefine.totolFloors + 1, 8));
for(int i = 0;i < DataDefine.totolFloors + 1;i++){
for(int j = 0;j < 8;j++){
contentPane.add(panels[i][j]);
}
}
this.pack();
this.setVisible(true);
this.setExtendedState(MAXIMIZED_BOTH);
//this.setExtendedState(MAXIMIZED_HORIZ);
this.setResizable(false);
for(int i = 0;i < DataDefine.totolFloors;i++){
ups[i].addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent arg0){
String str = ((JButton) arg0.getSource()).getName();
int floor = Integer.parseInt(str.substring(0, str.indexOf(":")));
int direction = str.substring(str.indexOf(":")+1).equals("up") ? 1 : -1;
buttonClick(floor, direction);
}
});
downs[i].addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent arg0){
String str = ((JButton) arg0.getSource()).getName();
int floor = Integer.parseInt(str.substring(0, str.indexOf(":")));
int direction = str.substring(str.indexOf(":")+1).equals("up") ? 1 : -1;
buttonClick(floor, direction);
}
});
}
new Timer(1000, taskPerformer).start();
}
private void addAExternApply(int f,int i){ // 有额外电梯申请应用
ApplyNode node=new ApplyNode(f,1);
this.elevaltors[i].addADes(node);
JOptionPane.showMessageDialog(this,"电梯 "+(i+1)+" 将为您服务");
DataInfo.stop=false;
}
private void buttonClick(int f,int direction){// f为外请求发生的层数,direction为向下或向上申请
//电梯选择算法
DataInfo.flags[f]=true;
boolean sameFlag=false;
DataInfo.stop=true;
int[] floors=new int[4];
int[] dirs=new int[4];
for(int i=0;i<4;i++){
floors[i]=DataInfo.getCurrentFloor(i);
dirs[i]=DataInfo.directions[i];
}
ArrayList<Integer> same=new ArrayList<Integer>(); //同方向链表
ArrayList<Integer> opp=new ArrayList<Integer>(); //反方向链表
ArrayList<Integer> stay=new ArrayList<Integer>();
for(int i=0;i<4;i++){
if(dirs[i]==0){
stay.add(i);
}else{
if(dirs[i]==direction){
same.add(i);
}else{
opp.add(i);
}
}
}
for(int i=0;i<4;i++){//如果当前层有电梯,则直接加入当前层电梯
if(floors[i]==f){
this.addAExternApply(f,i);
return;
}
}
if(same.size()!=0){//存在同向的电梯
int min=-1;
int index=-1;
int max=Integer.MAX_VALUE;
for(int i=0;i<same.size();i++){
switch(direction){
case 1://电梯在上行
if(floors[same.get(i)]>f&&floors[same.get(i)]>min){
min=floors[same.get(i)];
index=same.get(i);
}
continue;
case -1://电梯正在下行
if(floors[same.get(i)]<f&&floors[same.get(i)]<max){
max=floors[same.get(i)];
index=same.get(i);
}
continue;
}
}
if(index!=-1){
this.addAExternApply(f,index);
return;
}
sameFlag=true;
}
if(stay.size()!=0){
int min=Integer.MAX_VALUE;
int index=-1;
for(int i=0;i<stay.size();i++){
if(Math.abs(floors[stay.get(i)]-f)<min){
min=Math.abs(floors[stay.get(i)]-f);
index=stay.get(i);
}
}
if(index!=-1){
this.addAExternApply(f,index);
return;
}
}
if(sameFlag){
for(int i=0;i<same.size();i++){
opp.add(same.get(i));
}
int min=Integer.MAX_VALUE;
int index=-1;
for(int i=0;i<opp.size();i++){
if(Math.abs(floors[opp.get(i)]-f)<min){
min=Math.abs(floors[stay.get(i)]-f);
index=opp.get(i);
}
}
if(index!=-1){
this.addAExternApply(f,index);
return;
}
}
}
private ActionListener taskPerformer = new ActionListener(){
public void actionPerformed(ActionEvent evt){
if(DataInfo.stop != true){
for(int i=0;i<4;i++){
elevaltors[i].run();
}
for(int i = 0;i < DataDefine.totolFloors;i++){
if(DataInfo.flags[i]){
flags[i].setBackground(Color.RED);
}else{
flags[i].setBackground(Color.GRAY);
}
for(int j = 0;j < 4;j++){
if(DataInfo.positions[i][j] == 0){
textFields[i][j].setBackground(Color.BLACK);
}
if(DataInfo.positions[i][j] == 1){
textFields[i][j].setBackground(Color.GREEN);
textFields[i][j].setText(""
+ DataInfo.positions[i][j]);
}
//如果外部有人人要上电梯,电档显示黄色
if(DataInfo.positions[i][j]==2){
textFields[i][j].setBackground(Color.YELLOW);
DataInfo.positions[i][j]=1;
}
//如果内部有人到了目的地,电梯呈兰色
if(DataInfo.positions[i][j]==3){
textFields[i][j].setBackground(Color.BLUE);
DataInfo.positions[i][j]=1;
}
//如果电梯处于停止状态
if(DataInfo.positions[i][j]==4){
textFields[i][j].setBackground(Color.RED);
DataInfo.positions[i][j]=1;
}
}
}
DataInfo.update();
}
}
};
private void setJButtonName(){
for(int i = 0;i < DataDefine.totolFloors;i++){
ups[i].setName("" + i + ":up");
downs[i].setName("" + i + ":down");
}
}
private void initTitles(){
titles[0] = new JLabel("层数");
titles[1] = new JLabel("电梯1");
titles[2] = new JLabel("电梯2");
titles[3] = new JLabel("电梯3");
titles[4] = new JLabel("电梯4");
//titles[8]= new JLabel("电梯5");
titles[5] = new JLabel("向上请求");
titles[6] = new JLabel("向下请求");
titles[7] = new JLabel("指示灯");
titles[1].setName("0");
titles[1].setBackground(Color.MAGENTA);
titles[1].addMouseListener(new MouseListener(){
public void mouseClicked(MouseEvent arg0){
elevaltorSelect(0);
}
public void mousePressed(MouseEvent arg0){
}
public void mouseReleased(MouseEvent arg0){
}
public void m
dt.zip_java电梯_电梯
版权申诉
148 浏览量
2022-09-23
16:15:47
上传
评论
收藏 43KB ZIP 举报

林当时
- 粉丝: 100
- 资源: 1万+
最新资源
- com.aesq.zb_v1.0.35_danji100.com.apk
- 760996331259605建立门派1.360.apk
- 下面提供一些C语言的入门示例代码,并附有注释,以帮助理解每个部分的功能 1. Hello World程序 #include
- 下面提供一些C语言的入门示例代码,并附有注释,以帮助理解每个部分的功能 1. Hello World程序 #include
- 下面提供一些C语言的入门示例代码,并附有注释,以帮助理解每个部分的功能 1. Hello World程序 #include
- C语言是一种广泛使用的计算机编程语言,它是许多其他编程语言的基础 以下是一些C语言入门的例子和代码,适合初学者学习和实践
- C语言是一种广泛使用的计算机编程语言,它是许多其他编程语言的基础 以下是一些C语言入门的例子和代码,适合初学者学习和实践
- C语言是一种广泛使用的计算机编程语言,它是许多其他编程语言的基础 以下是一些C语言入门的例子和代码,适合初学者学习和实践
- C语言 入门例子和代码学习
- C语言 入门例子和代码学习
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


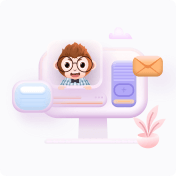