package com.fh.controller.weixin;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.net.ConnectException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import javax.annotation.Resource;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSocketFactory;
import javax.net.ssl.TrustManager;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import net.sf.json.JSONObject;
import org.marker.weixin.DefaultSession;
import org.marker.weixin.HandleMessageAdapter;
import org.marker.weixin.MySecurity;
import org.marker.weixin.msg.Data4Item;
import org.marker.weixin.msg.Msg4Event;
import org.marker.weixin.msg.Msg4Image;
import org.marker.weixin.msg.Msg4ImageText;
import org.marker.weixin.msg.Msg4Link;
import org.marker.weixin.msg.Msg4Location;
import org.marker.weixin.msg.Msg4Text;
import org.marker.weixin.msg.Msg4Video;
import org.marker.weixin.msg.Msg4Voice;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import com.fh.controller.base.BaseController;
import com.fh.service.weixin.command.CommandService;
import com.fh.service.weixin.imgmsg.ImgmsgService;
import com.fh.service.weixin.textmsg.TextmsgService;
import com.fh.util.Const;
import com.fh.util.PageData;
import com.fh.util.Tools;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import javax.net.ssl.X509TrustManager;
/**
*
* 类名称:WeixinController.java
* 类描述: 微信公共平台开发
* @author FH
* 作者单位:
* 联系方式:
* 创建时间:2014年7月10日
* @version 1.0
*/
@Controller
@RequestMapping(value="/weixin")
public class WeixinController extends BaseController{
@Resource(name="textmsgService")
private TextmsgService textmsgService;
@Resource(name="commandService")
private CommandService commandService;
@Resource(name="imgmsgService")
private ImgmsgService imgmsgService;
/**
* 接口验证,总入口
* @param out
* @param request
* @param response
* @throws Exception
*/
@RequestMapping(value="/index")
public void index(
PrintWriter out,
HttpServletRequest request,
HttpServletResponse response
) throws Exception{
logBefore(logger, "微信接口");
PageData pd = new PageData();
try{
pd = this.getPageData();
String signature = pd.getString("signature"); //微信加密签名
String timestamp = pd.getString("timestamp"); //时间戳
String nonce = pd.getString("nonce"); //随机数
String echostr = pd.getString("echostr"); //字符串
if(null != signature && null != timestamp && null != nonce && null != echostr){/* 接口验证 */
logBefore(logger, "进入身份验证");
List<String> list = new ArrayList<String>(3) {
private static final long serialVersionUID = 2621444383666420433L;
public String toString() { // 重写toString方法,得到三个参数的拼接字符串
return this.get(0) + this.get(1) + this.get(2);
}
};
list.add(Tools.readTxtFile(Const.WEIXIN)); //读取Token(令牌)
list.add(timestamp);
list.add(nonce);
Collections.sort(list); // 排序
String tmpStr = new MySecurity().encode(list.toString(),
MySecurity.SHA_1); // SHA-1加密
if (signature.equals(tmpStr)) {
out.write(echostr); // 请求验证成功,返回随机码
}else{
out.write("");
}
out.flush();
out.close();
}else{/* 消息处理 */
logBefore(logger, "进入消息处理");
response.reset();
sendMsg(request,response);
}
} catch(Exception e){
logger.error(e.toString(), e);
}
}
/**
* 处理微信服务器发过来的各种消息,包括:文本、图片、地理位置、音乐等等
* @param request
* @param response
* @throws Exception
*/
public void sendMsg(HttpServletRequest request, HttpServletResponse response) throws Exception{
InputStream is = request.getInputStream();
OutputStream os = response.getOutputStream();
final DefaultSession session = DefaultSession.newInstance();
session.addOnHandleMessageListener(new HandleMessageAdapter(){
/**
* 事件
*/
@Override
public void onEventMsg(Msg4Event msg) {
/** msg.getEvent()
* unsubscribe:取消关注 ; subscribe:关注
*/
if("subscribe".equals(msg.getEvent())){
returnMSg(msg,null,"关注");
}
}
/**
* 收到的文本消息
*/
@Override
public void onTextMsg(Msg4Text msg) {
returnMSg(null,msg,msg.getContent().trim());
}
@Override
public void onImageMsg(Msg4Image msg) {
// TODO Auto-generated method stub
super.onImageMsg(msg);
}
@Override
public void onLocationMsg(Msg4Location msg) {
// TODO Auto-generated method stub
super.onLocationMsg(msg);
}
@Override
public void onLinkMsg(Msg4Link msg) {
// TODO Auto-generated method stub
super.onLinkMsg(msg);
}
@Override
public void onVideoMsg(Msg4Video msg) {
// TODO Auto-generated method stub
super.onVideoMsg(msg);
}
@Override
public void onVoiceMsg(Msg4Voice msg) {
// TODO Auto-generated method stub
super.onVoiceMsg(msg);
}
@Override
public void onErrorMsg(int errorCode) {
// TODO Auto-generated method stub
super.onErrorMsg(errorCode);
}
/**
* 返回消息
* @param emsg
* @param tmsg
* @param getmsg
*/
public void returnMSg(Msg4Event emsg, Msg4Text tmsg, String getmsg){
PageData msgpd;
PageData pd = new PageData();
String toUserName,fromUserName,createTime;
if(null == emsg){
toUserName = tmsg.getToUserName();
fromUserName = tmsg.getFromUserName();
createTime = tmsg.getCreateTime();
}else{
toUserName = emsg.getToUserName();
fromUserName = emsg.getFromUserName();
createTime = emsg.getCreateTime();
}
pd.put("KEYWORD", getmsg);
try {
msgpd = textmsgService.findByKw(pd);
if(null != msgpd){
Msg4Text rmsg = new Msg4Text();
rmsg.setFromUserName(toUserName);
rmsg.setToUserName(fromUserName);
//rmsg.setFuncFlag("0");
rmsg.setContent(msgpd.getString("CONTENT")); //回复文字消息
session.callback(rmsg);
}else{
msgpd = imgmsgService.findByKw(pd);
if(null != msgpd){
Msg4ImageText mit = new Msg4ImageText();
mit.setFromUserName(toUserName);
mit.setToUserName(fromUserName);
mit.setCreateTime(cre

林当时
- 粉丝: 114
- 资源: 1万+
最新资源
- 基于Springboot+Vue的影院订票系统的设计与实现-毕业源码案例设计(源码+数据库).zip
- 基于Springboot+Vue的疫情管理系统-毕业源码案例设计(高分项目).zip
- 基于Springboot+Vue的影城管理电影购票系统毕业源码案例设计(95分以上).zip
- 贝加莱控制系统常见问题手册
- uDDS源程序subscriber
- 基于Springboot+Vue的游戏交易系统-毕业源码案例设计(源码+数据库).zip
- 基于Springboot+Vue的在线教育系统设计与实现毕业源码案例设计(源码+论文).zip
- 基于Springboot+Vue的在线拍卖系统毕业源码案例设计(高分毕业设计).zip
- PDF翻译器:各种语言的PDF互翻译,能完美保留公式、格式、图片,还能生成单独或者中英对照的PDF文件
- 基于Springboot+Vue的智能家居系统-毕业源码案例设计(源码+数据库).zip
- 基于Springboot+Vue的在线文档管理系统毕业源码案例设计(源码+项目说明+演示视频).zip
- 基于Springboot+Vue的智慧生活商城系统设计与实现-毕业源码案例设计(95分以上).zip
- 基于Springboot+Vue的装饰工程管理系统-毕业源码案例设计(源码+项目说明+演示视频).zip
- 基于Springboot+Vue的租房管理系统-毕业源码案例设计(高分毕业设计).zip
- 基于Springboot+Vue电影评论网站系统设计毕业源码案例设计(高分项目).zip
- 基于Springboot+Vue服装生产管理系统毕业源码案例设计(95分以上).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


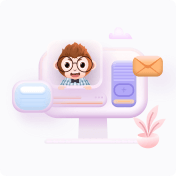