package cn.tuoyuan.user.order.service.impl;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.SortedMap;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import cn.tuoyuan.admin.common.exception.ServiceException;
import cn.tuoyuan.admin.order.entity.ConsigneeInfo;
import cn.tuoyuan.admin.order.entity.Order;
import cn.tuoyuan.admin.order.entity.OrderProduct;
import cn.tuoyuan.user.cart.service.CartServiceImmpl;
import cn.tuoyuan.user.order.dao.UserOrderDao;
import cn.tuoyuan.user.order.entity.GenerateOrderNumber;
import cn.tuoyuan.user.order.service.UserOrderService;
import cn.tuoyuan.user.weixinPay.WeiXinCommonUtil;
@Service
public class UserOrderServiceImpl implements UserOrderService{
@Autowired
private UserOrderDao userOrderDao;
/**
* 查找确认订单中商品信息和收货信息
* @param openId 用户唯一标识
* @param id 收货地址id
* @param sessionMap 购物车通过session传递的要购买的商品id和数量
*/
@Override
public Map<String, Object> findObjects(String openId,Integer id,Map<Integer,Integer>sessionMap) {
List<Map<String,Object>> list = new ArrayList<Map<String,Object>>();
Iterator<Entry<Integer, Integer>> it = sessionMap.entrySet().iterator();
while (it.hasNext()) {
Entry<Integer, Integer> entry = it.next();
Integer productId = entry.getKey();
Integer amount = entry.getValue();
Map<String,Object> map = userOrderDao.findProduct(productId);
map.put("amount", amount);
list.add(map);
}
//根据id判断,id==null表示没有选择更换地址,则显示默认地址,id!=null表示选择更换地址,显示该选中(id)地址
ConsigneeInfo consigneeInfo = new ConsigneeInfo();
if(id==null){
consigneeInfo = userOrderDao.findByState(openId);
}else{
consigneeInfo = userOrderDao.findById(id);
}
Map<String,Object> map = new HashMap<String,Object>();
map.put("consigneeInfo",consigneeInfo);
map.put("list", list);
return map;
}
/**
* 生成订单并微信支付
* @param session 获取要购买的商品id和数量
* @param extmId 收货地址id
* @param openId
*/
@Override
public SortedMap<String, Object> insertObjects(HttpSession session,Integer extmId,String openId) {
//生成订单号
String orderId = GenerateOrderNumber.getOrderNumber(userOrderDao,openId);
List<OrderProduct> orderProductList = new ArrayList<OrderProduct>();
//商品总数
int totalAmount = 0;
//总金额
BigDecimal totalMoney=new BigDecimal(0.00);
//要购买的各商品id及数量
@SuppressWarnings("unchecked")
Map<Integer, Integer> sessionMap = (Map<Integer, Integer>)session.getAttribute("order");
Iterator<Entry<Integer, Integer>> it = sessionMap.entrySet().iterator();
List<Integer> list = new ArrayList<Integer>();
while (it.hasNext()) {
Entry<Integer, Integer> entry = it.next();
Integer id = entry.getKey();
Integer amount = entry.getValue();
Map<String,Object> map = userOrderDao.findProduct(id);
BigDecimal price = (BigDecimal)map.get("productPrice");
//订单商品详情对象
OrderProduct op = new OrderProduct();
op.setOrderId(orderId);
op.setId(id);
op.setProductName((String)map.get("productName"));
op.setTypeName((String)map.get("typeName"));
op.setProductPrice(price);
op.setPictureName((String)map.get("pictureName"));
op.setProductAmount(amount);
op.setTotalPrice(price.multiply(new BigDecimal(amount)));
orderProductList.add(op);
totalAmount += amount;
totalMoney = totalMoney.add(price.multiply(new BigDecimal(amount)));
list.add(id);
}
//收货地址对象
ConsigneeInfo consigneeInfo = userOrderDao.findAddressById(extmId);
//订单对象
Order order = new Order();
order.setOrderId(orderId);
order.setOpenId(openId);
order.setName(consigneeInfo.getName());
order.setPhoneNumber(consigneeInfo.getPhoneNumber());
order.setAddress(consigneeInfo.getAddress());
order.setTotalAmount(totalAmount);
order.setTotalMoney(totalMoney);
//生成订单
int rows1=userOrderDao.insertOrder(order);
int rows2=0;
if(rows1!=0){
rows2 = userOrderDao.insertOrderProduct(orderProductList);
if(rows2==0)userOrderDao.deleteOrder(orderId);
}else if(rows1==0||rows2==0){
throw new ServiceException("插入订单失败");
}
//生成订单后删除购物车中已购商品
CartServiceImmpl c = new CartServiceImmpl();
for(int i=0;i<list.size();i++){
c.alter(list.get(i), null, session);
}
//请求微信支付
SortedMap<String, Object> map = null;
try {
//生成JSAPI页面调用的支付参数和签名
map = WeiXinCommonUtil.weixinPay(orderId,totalMoney.multiply(new BigDecimal(100)).intValue());
} catch (Exception e) {
e.printStackTrace();
}
return map;
}
/**
* 查找一个用户下的所有订单
*/
@Override
public Map<String, Object> findOrders(String openId) {
if(openId==null)
throw new ServiceException("openId不能为空");
List<Map<String,Object>> list = userOrderDao.findOrders(openId);
List<Order> orderList = userOrderDao.findOrdersByOpenId(openId);
Map<String,Object> map = new HashMap<String,Object>();
map.put("list", list);
map.put("orderList", orderList);
return map;
}
/**
* 修改订单状态
*/
@Override
public void updateOrderState(String orderId) {
if(orderId==null)
throw new ServiceException("被修改订单号不能为空");
//获得当前订单状态
Order order = userOrderDao.findOrder(orderId);
//修改
order.setOrderState(order.getOrderState()+1);
int rows = userOrderDao.updateOrderState(order);
if(rows==0)
throw new ServiceException("修改订单状态失败");
}
/**
* 删除订单
*/
@Override
public void deleteOrder(String orderId) {
if(orderId==null)
throw new ServiceException("被取消订单号不能为空");
//删除订单
int rows1 = userOrderDao.deleteOrder(orderId);
//删除订单详情
int rows2 = userOrderDao.deleteOrderProduct(orderId);
if(rows1==0||rows2==0)
throw new ServiceException("取消订单失败");
}
/**
* 查找一个用户下的所有收货信息
*/
@Override
public List<ConsigneeInfo> findAddress(String openId) {
if(openId==null)
throw new ServiceException("openId不能为空");
List<ConsigneeInfo> list = userOrderDao.findAddress(openId);
return list;
}
/**
* 删除收货信息
*/
@Override
public void deleteAddress(Integer id) {
if(id==null)
throw new ServiceException("收货地址id不能为空");
int rows = userOrderDao.deleteAddress(id);
if(rows==0)
throw new ServiceException("删除地址失败");
}
/**
* 添加收货信息
*/
@Override
public void insertAddress(String openId, ConsigneeInfo consigneeInfo) {
if(openId==null)
throw new ServiceException("openId不能为空");
if(consigneeInfo==null)
throw new ServiceException("保存的地址对象不能为空");
consigneeInfo.setOpenId(openId);
int rows = userOrderDao.insertAddress(consigneeInfo);
if(rows==0)
throw new ServiceException("保存地址失败");
}
/**
* 查找某条收货信息
*/
@Override
public ConsigneeInfo findAddressById(Integer id) {
if(id==null)throw new ServiceException("查询的地址id不能为空");
ConsigneeInfo consigneeInfo = userOrderDao.findAddressById(id);
if(consigneeInfo==null)throw new ServiceException("查询地址失败");
return consigneeInfo;
}
/**
* 修改收货信息
*/
@Override
public void updateAddress(ConsigneeInfo consigneeInfo) {
if(consigneeInfo==n
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
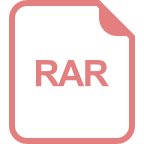
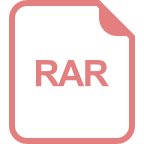
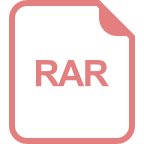
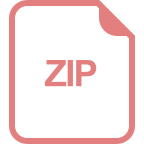
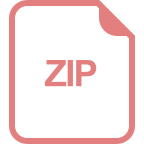
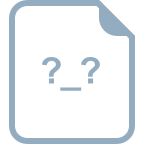
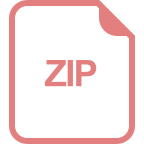
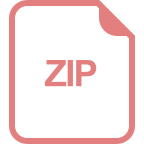
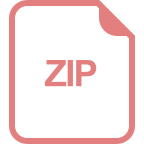
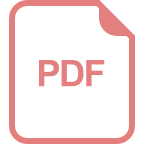
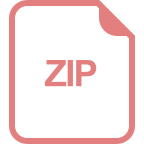
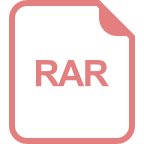
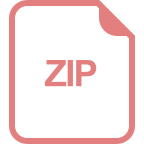
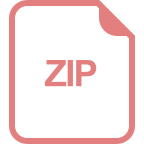
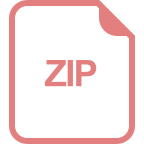
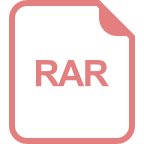
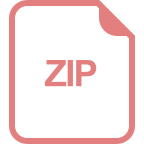
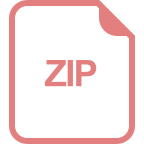
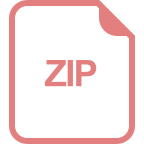
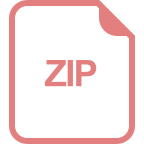
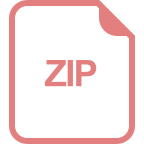
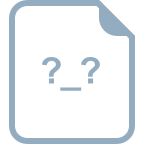
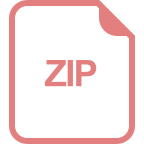
收起资源包目录

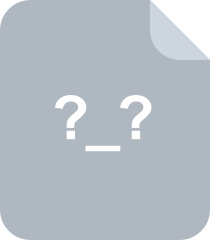
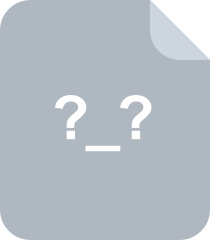
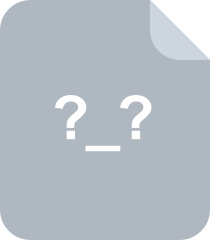
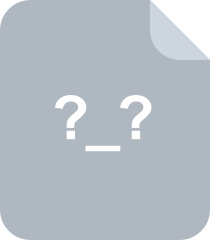
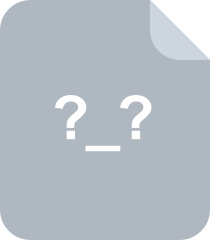
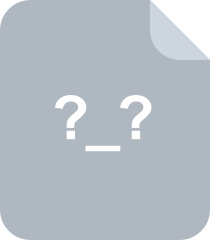
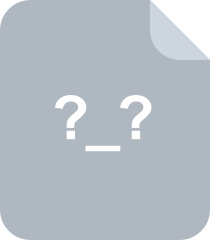
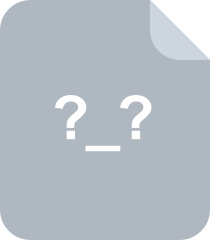
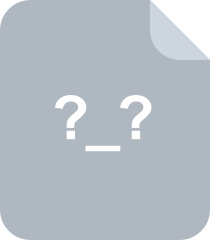
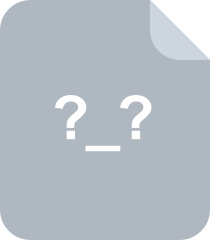
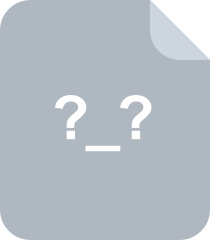
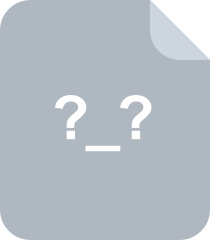
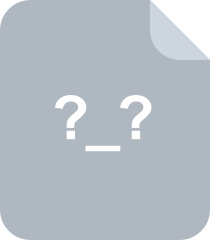
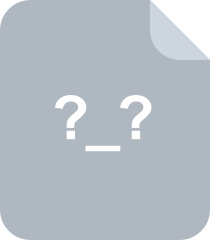
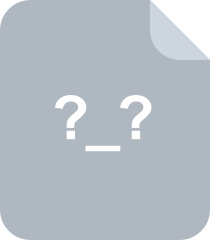
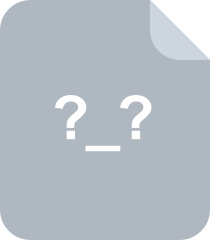
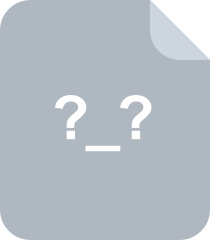
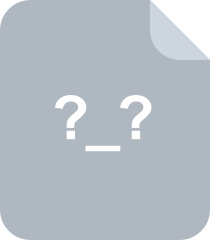
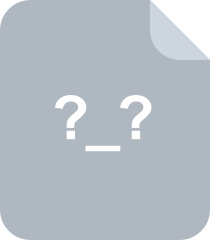
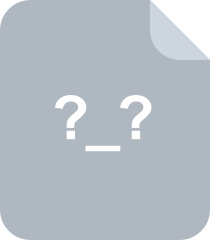
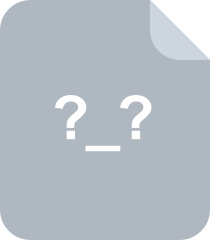
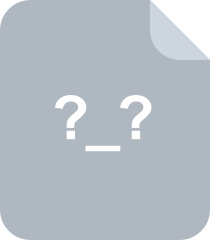
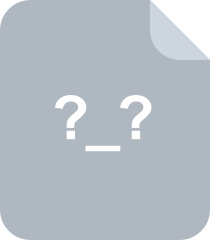
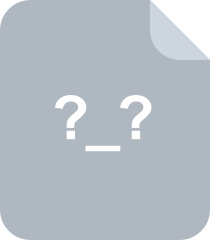
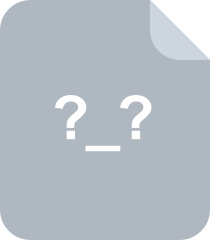
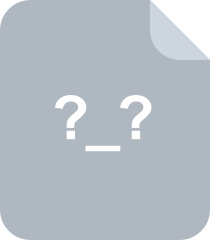
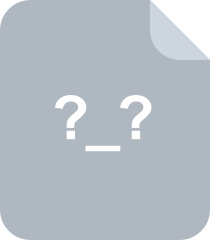
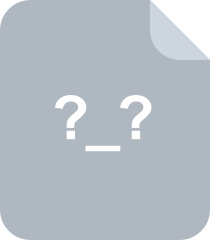
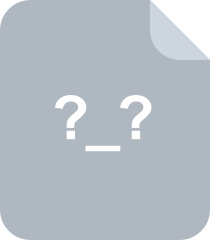
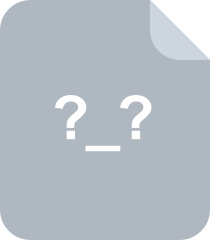
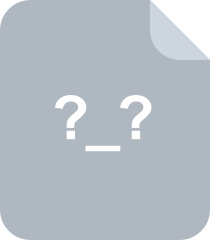
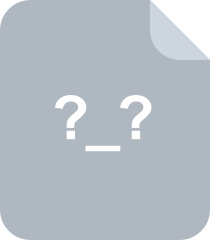
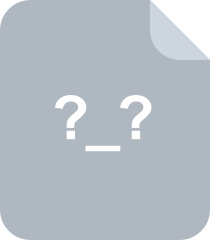
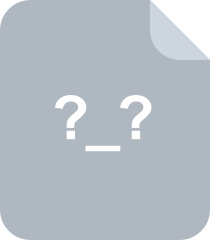
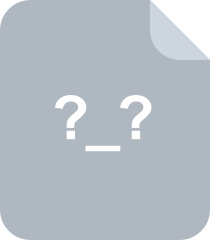
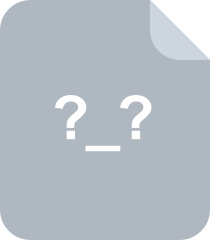
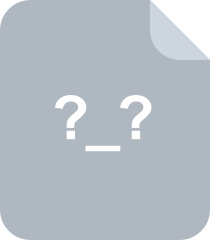
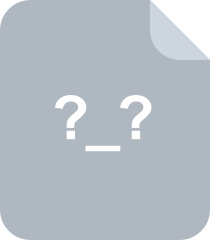
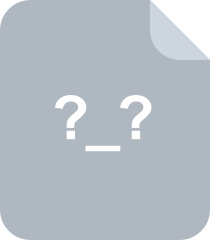
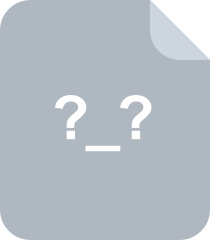
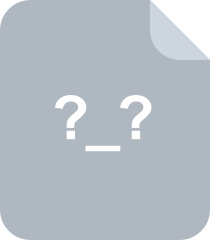
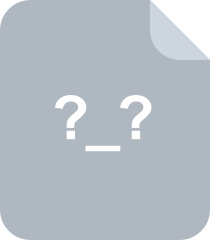
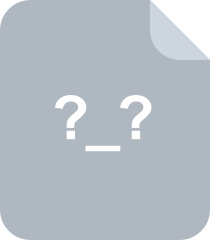
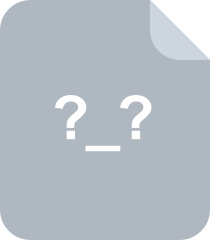
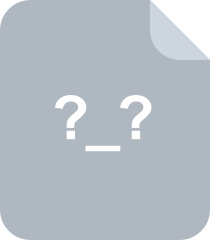
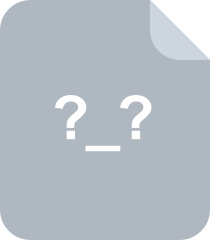
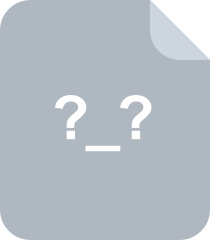
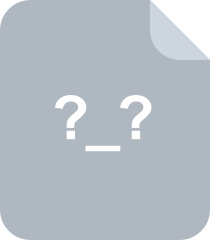
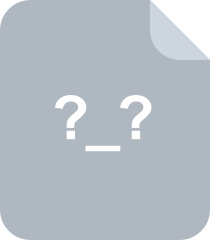
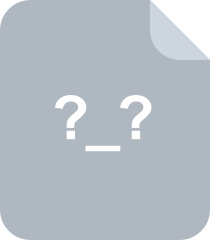
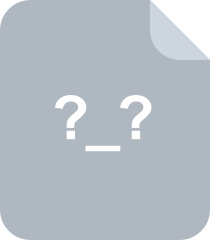
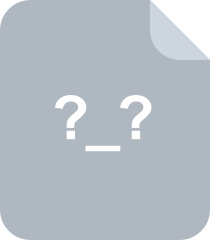
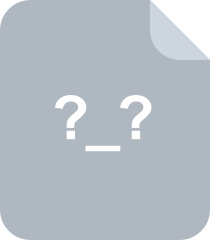
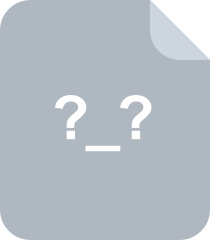
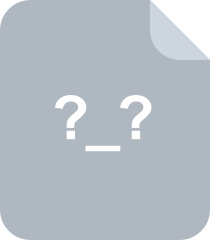
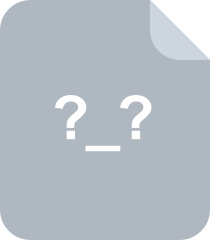
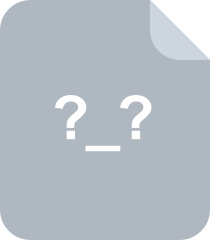
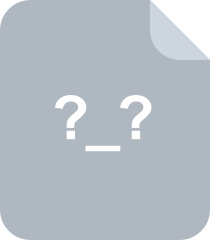
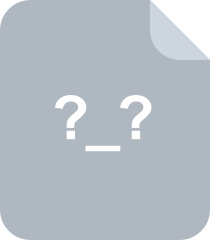
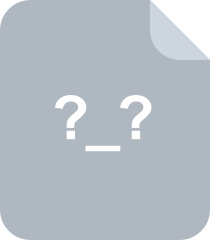
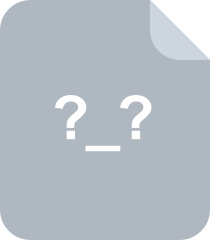
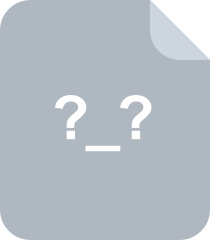
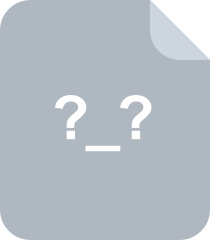
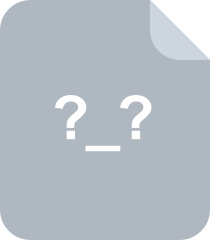
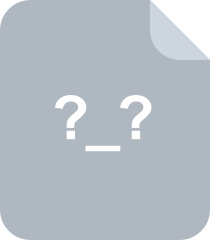
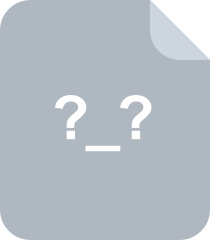
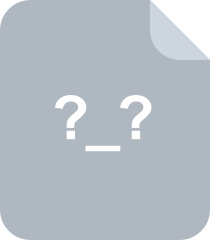
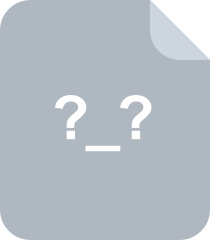
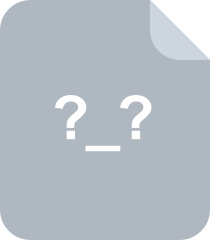
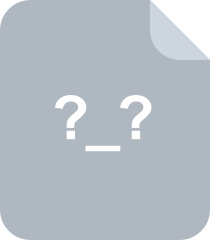
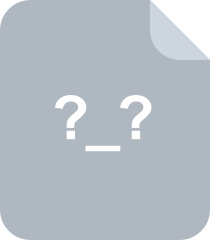
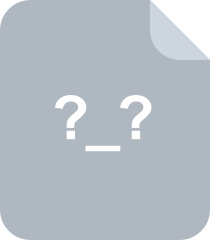
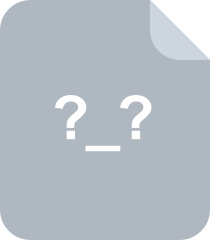
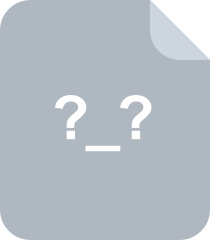
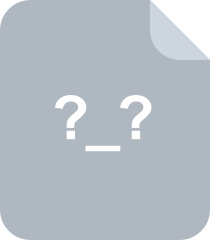
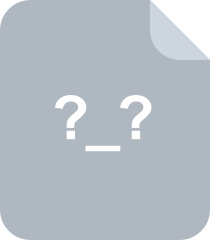
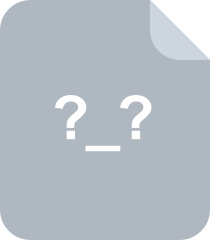
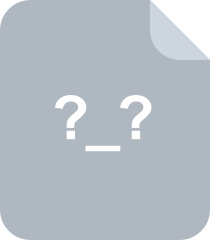
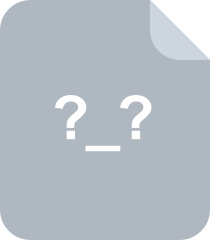
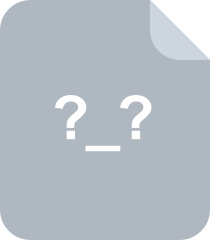
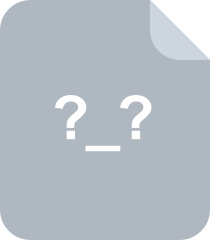
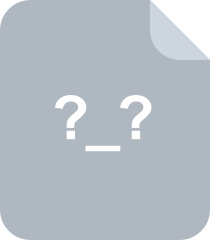
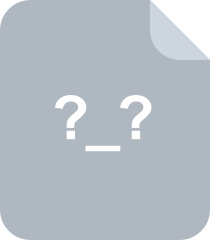
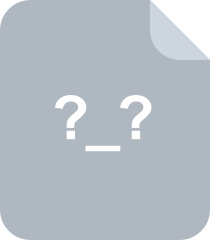
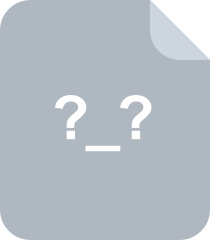
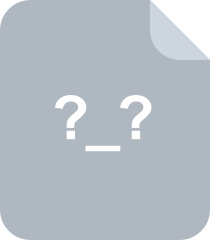
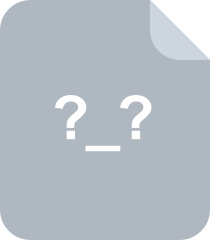
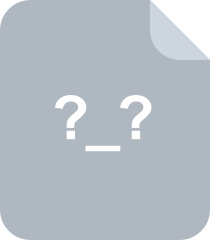
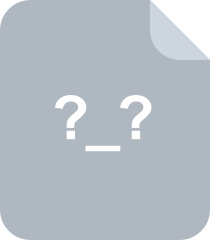
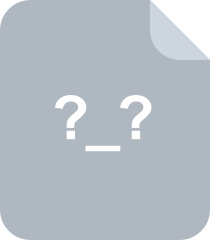
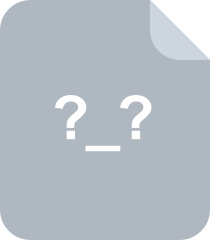
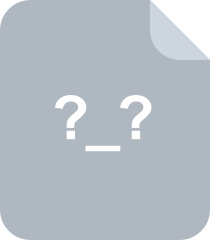
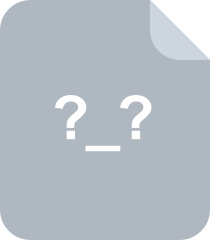
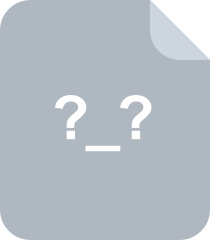
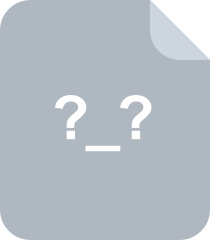
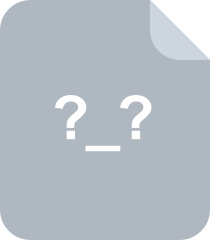
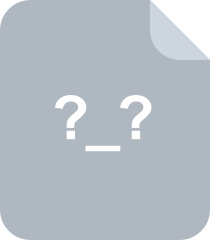
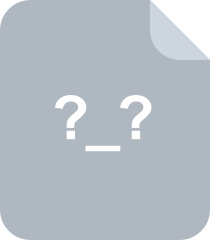
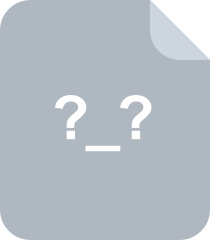
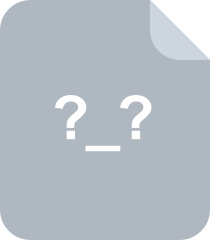
共 1375 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
资源评论
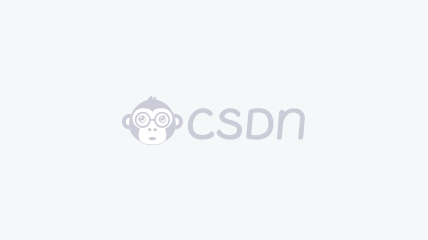

alvarocfc
- 粉丝: 131
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

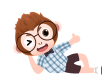
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


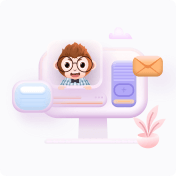
安全验证
文档复制为VIP权益,开通VIP直接复制
