// EDGE2WEB.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include "smtp.h"
#if !defined(OTL_ORA10G_R2)
#define OTL_ORA10G_R2 // Compile OTL 4.0/OCI10gR2
#endif
#define OTL_ORA_SUBSCRIBE // Enable the otl_subscriber interface
// The following two #define's are required for
// the otl_subscriber interface to function
#define OTL_ORA_OCI_ENV_CREATE
#define OTL_ORA_OCI_ENV_CREATE_MODE (OCI_OBJECT|OCI_EVENTS)
#include <otlv4.h> // include the OTL 4.0 header file
char _chhmmss[32];
char* currenttime()
{
// return current system time
time_t now;
struct tm *newtime;
time(&now);
newtime = localtime(&now);
strftime(_chhmmss, 32, "%m/%d/%Y %H:%M:%S ", newtime);
return _chhmmss;
}
SERVICE_STATUS g_ServiceStatus = {0};
SERVICE_STATUS_HANDLE g_StatusHandle = NULL;
HANDLE g_ServiceStopEvent = INVALID_HANDLE_VALUE;
VOID WINAPI ServiceMain (DWORD argc, LPTSTR *argv);
VOID WINAPI ServiceCtrlHandler (DWORD);
DWORD WINAPI ServiceWorkerThread (LPVOID lpParam);
// global variables for service maintenance
const int nBufferSize = 500;
char g_pServiceName[nBufferSize+1];
char g_pExeFile[nBufferSize+1];
// global variables for logging to file
std::streambuf* g_pOldBuf = NULL;
std::ofstream g_out;
char g_pLogFile[nBufferSize+1];
// global variable for configuration file
char g_pCfgFile[nBufferSize+1];
#define SERVICE_NAME "EDGE2PSEWebsiteUpdater"
//////////////////////////////////////////////////////////////////////
//
// Uninstall
//
VOID UnInstall(char* pName)
{
SC_HANDLE schSCManager = OpenSCManager( NULL, NULL, SC_MANAGER_ALL_ACCESS);
if (schSCManager==0)
{
long nError = GetLastError();
char pTemp[121];
sprintf(pTemp, "OpenSCManager failed, error code = %d", nError);
//WriteLog(pTemp);
}
else
{
SC_HANDLE schService = OpenService( schSCManager, pName, SERVICE_ALL_ACCESS);
if (schService==0)
{
long nError = GetLastError();
char pTemp[121];
sprintf(pTemp, "OpenService failed, error code = %d", nError);
//WriteLog(pTemp);
}
else
{
if(!DeleteService(schService))
{
char pTemp[121];
sprintf(pTemp, "Failed to delete service %s", pName);
//WriteLog(pTemp);
}
else
{
char pTemp[121];
sprintf(pTemp, "Service %s removed",pName);
//WriteLog(pTemp);
}
CloseServiceHandle(schService);
}
CloseServiceHandle(schSCManager);
}
}
//////////////////////////////////////////////////////////////////////
//
// Install
//
VOID Install(char* pPath, char* pName)
{
SC_HANDLE schSCManager = OpenSCManager( NULL, NULL, SC_MANAGER_CREATE_SERVICE);
if (schSCManager==0)
{
long nError = GetLastError();
char pTemp[121];
sprintf(pTemp, "OpenSCManager failed, error code = %d", nError);
//WriteLog(pTemp);
}
else
{
SC_HANDLE schService = CreateService
(
schSCManager, /* SCManager database */
pName, /* name of service */
pName, /* service name to display */
SERVICE_ALL_ACCESS, /* desired access */
SERVICE_WIN32_OWN_PROCESS|SERVICE_INTERACTIVE_PROCESS , /* service type */
SERVICE_AUTO_START, /* start type */
SERVICE_ERROR_NORMAL, /* error control type */
pPath, /* service's binary */
NULL, /* no load ordering group */
NULL, /* no tag identifier */
NULL, /* no dependencies */
NULL, /* LocalSystem account */
NULL
); /* no password */
if (schService==0)
{
long nError = GetLastError();
char pTemp[121];
sprintf(pTemp, "Failed to create service %s, error code = %d", pName, nError);
//WriteLog(pTemp);
}
else
{
char pTemp[121];
sprintf(pTemp, "Service %s installed", pName);
//WriteLog(pTemp);
CloseServiceHandle(schService);
}
CloseServiceHandle(schSCManager);
}
}
#ifdef _DEBUG
/* ControlHandler
Custom handler of Ctrl+C and Ctrl+Break, to ensure graceful system exit
*/
BOOL WINAPI ControlHandler(DWORD dwCtrlType)
{
switch( dwCtrlType ) {
case CTRL_BREAK_EVENT: // use Ctrl+C or Ctrl+Break to simulate
case CTRL_C_EVENT: // SERVICE_CONTROL_STOP in debug mode
case CTRL_CLOSE_EVENT:
case CTRL_LOGOFF_EVENT:
case CTRL_SHUTDOWN_EVENT:
::SetEvent(g_ServiceStopEvent);
return TRUE;
}
return FALSE;
}
#endif
int main(int argc, char* argv[])
{
// initialize MFC
if (!AfxWinInit(::GetModuleHandle(NULL), NULL, ::GetCommandLine(), 0))
{
// TODO: change error code to suit your needs
//_tprintf(_T("Fatal Error: MFC initialization failed\n"));
return 1;
}
// Initialize WinSock
WSADATA wsaData;
WORD wVer = MAKEWORD(2,2);
WSAStartup(wVer,&wsaData);
// initialize variables for .exe, .cfg, and .log file names
char pModuleFile[nBufferSize+1];
DWORD dwSize = GetModuleFileName(NULL,pModuleFile,nBufferSize);
pModuleFile[dwSize] = 0;
if(dwSize>4&&pModuleFile[dwSize-4]=='.')
{
sprintf(g_pExeFile,"%s",pModuleFile);
pModuleFile[dwSize-4] = 0;
sprintf(g_pCfgFile,"%s.cfg",pModuleFile);
// logfile is of the form exename_YYYYMM.LOG
// return current system time
time_t now;
char yyyymm[8];
struct tm *newtime;
time(&now);
newtime = localtime(&now);
strftime(yyyymm, 8, "%Y%m", newtime);
sprintf(g_pLogFile,"%s_%s.log",pModuleFile, yyyymm);
} else {
printf("Invalid module file name: %s\r\n", pModuleFile);
return 2;
}
// Read the service name from the configuration file
GetPrivateProfileString("Service Settings","ServiceName",SERVICE_NAME,
g_pServiceName,nBufferSize,g_pCfgFile);
#ifndef _DEBUG
// uninstall service if switch is "-u"
if(argc==2&&_stricmp("-u",argv[1])==0)
{
UnInstall(g_pServiceName);
return 0;
}
// install service if switch is "-i"
else if(argc==2&&_stricmp("-i",argv[1])==0)
{
Install(g_pExeFile, g_pServiceName);
return 0;
}
#endif
// Redirect cout as early as possible so we can use it for logging early errors
// redirect cout to file
g_pOldBuf = cout.rdbuf();
g_out.open(g_pLogFile, ios::out|ios::binary|ios::app);
std::cout.rdbuf(g_out.rdbuf()); // << this is the redirect command!!
cout << currenttime() << "========================" << endl << flush;
cout << currenttime() << "Service [" << g_pServiceName << "] has started." << endl << flush;
if (!AfxSocketInit()) {
cout << currenttime() << "AfxSocketInit failed. Email notification will not work." << endl;
}
#ifndef _DEBUG
SERVICE_TABLE_ENTRY ServiceTable[] =
{
{SERVICE_NAME, (LPSERVICE_MAIN_FUNCTION) ServiceMain},
{NULL, NULL}
};
if (StartServiceCtrlDispatcher (ServiceTable) == FALSE)
{
cout << currenttime() << "StartServiceCtrlDispatcher returned error." << endl << flush;
// restore cout
if(g_pOldBuf) {
cout.rdbuf(g_pOldBuf);
}
return GetLastError ();
}
// you don't get here unless the service is shutdown
cout << currenttime() << "Service [" << g_pServiceName << "] has been stopped." << endl << flush;
//////////////////////////////////////////////////////
// CLEAN-UP code goes here
Sleep(3000);
#else
g_ServiceStopEvent = CreateEvent(NULL, TRUE, FALSE, NULL);
BOOL bCtrlHandled = SetConsoleCtrlHandler(ControlHandler, TRUE);
HANDLE hThread = CreateThread (NULL, 0, ServiceWorkerThread, NULL, 0, NULL);
WaitForSingleObject(hThread, INFINITE);
if(bCtrlHandled) {
SetConsoleCtrlHandler(ControlHandler, FALSE);
}
cout << currenttime() << "ServiceWorkerThread stopped." << endl << flush;
CloseHandle(g_ServiceStopEvent);
#endif
// restore cout
if(g_pOldBuf) {
cout.rdbuf(g_pOldBuf);
}
return 0;
}
VOID WINAPI Servic

alvarocfc
- 粉丝: 134
- 资源: 1万+
最新资源
- springboot校车调度管理系统_r4le2--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- python基于机器学习心脏病预测源码+csv格式数据集(高分期末大作业)
- springboot宿舍管理系统_o4dvi--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 数据中台与业务中台设计方案.pptx
- springboot图书管理系统_g9e3a--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 元宇宙社区展厅规划设计.docx
- 城市照明管理数字化解决方案.docx
- 弱电智能化设计方案.docx
- springboot企业车辆管理系统设计与实现--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot汽车租赁管理系统_1ma2x--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot房屋交易系统_88j45-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot大学生班级管理系统_9809i--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot流浪猫狗救助救援网站_4a4i2--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于Java Web的智能二维码门禁管理系统本科毕业设计论文+源码(高分毕设)
- springboot基于协同过滤算法的私人诊所管理系统_6t4o8--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot健美操评分系统_o4o1y--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


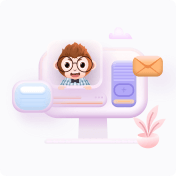