/
* Program Description: The program asks the user to choice from the menu an option
* A. Check to see if a number is prime.
* B. Count the number of vowels in a line.
* X. Exit the program.
*/
import java.io.*;
import java.util.*;
import java.lang.String;
public class Assignment_1
{
public static void main(String[] args)
throws IOException
{
Scanner selection = new Scanner(System.in);
BufferedReader stdin = new BufferedReader
(new InputStreamReader (System.in));
String menuSel, text;
for( ;; ){
// The menu section for the user to an option.
System.out.println("\n *******************Assignment 1 Menu*******************");
System.out.println("\t A. Check to see if a number is Prime");
System.out.println("\t B. Count the number of Vowels in a line.");
System.out.println("\t X. Exit the program.");
System.out.println("********************************************************\n");
System.out.print("Please select one of the above options (A, B or X): ");
menuSel = selection.next();
// calculates if the number you entered is a prime number
if (menuSel.equalsIgnoreCase("A"))
{
System.out.println("\n Please enter a Integar: ");
int num = Integer.parseInt(stdin.readLine());
if (num > 0 && (num % 2) != 0)
{
System.out.println("\n The number " +num+ " is prime.");
}
else if (num == 0)
{
System.out.println("\n Please enter a number larger than 0.");
}
else
System.out.println("\n The number " +num+ " is not prime");
}
// The section is to calculate the numbers of vowels in an inputed text.
else if (menuSel.equalsIgnoreCase("B"))
{
System.out.println("\n Please enter a line of text: ");
BufferedReader bf = new BufferedReader(new InputStreamReader(System.in));
text = bf.readLine();
int vowelCount = 0;
for (int i = 0; i < text.length(); i++)
{
char currentChar = text.charAt(i);
if (currentChar == 'A' || currentChar == 'a'
|| currentChar == 'E' || currentChar == 'e'
|| currentChar == 'I' || currentChar == 'i'
|| currentChar == 'O' || currentChar == 'o'
|| currentChar == 'U' || currentChar == 'u')
vowelCount++;
}
System.out.println("");
System.out.println("Number of vowels found in the line is " + vowelCount);
}
// The section terminates the program.
else if (menuSel.equalsIgnoreCase("X"))
{
System.out.println("Existing program goodbye...");
System.exit(0);
}
else if (menuSel != ("X"))
{
System.out.println("Error! invalid Selection");
}
} // End of the for loop
}
}
User_Menu_Choice.rar_The Count
版权申诉
90 浏览量
2022-09-23
08:07:52
上传
评论
收藏 1KB RAR 举报

alvarocfc
- 粉丝: 105
- 资源: 1万+
最新资源
- 王姿.html
- 51单片机学习(1)-软件keil下载
- 历届(第1-21届)希望杯数学竞赛初一试题及答案(最新整理).doc全国数学邀请赛(264页资料)
- 水滴.psd
- TokenPocket_V2.1.2_release.apk
- Apache-druid-kafka-rce.yaml
- 基于C#的ASP.NET数据库原理及应用技术课程指导平台的开发
- 基于ROS的智能车轨迹跟踪算法的仿真与设计源码运用PID跟踪算法.zip.zip
- Bug Bounty Tip - i春秋Self-XSS变废为宝的奇思妙想
- 1991-2015年全国初中化学竞赛复赛试题汇编(212页)(24年竞赛复赛真题).docx天原杯
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


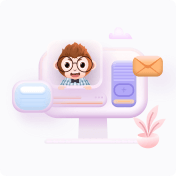