package cn.xt.util;
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.net.Socket;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
public class DbFH{
private static Log logger = LogFactory.getLog(DbFH.class);
private static Properties pros = getPprVue();
public static Map<String, String> backUpTableList = new ConcurrentHashMap<String, String>();
public static Map<String, String> recoverTableList = new ConcurrentHashMap<String, String>();
private static DbFH dbFH = new DbFH();
public static void main(String[] arg){
try {
String str = DbFH.getDbFH().backup("").toString();//璋冪敤鏁版嵁搴撳浠?
System.out.println(FileUtil.getFilesize(str));
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
public static DbFH getDbFH(){
return dbFH;
}
/**鎵ц鏁版嵁搴撳浠藉叆鍙?
* @param tableName 琛ㄥ悕
* @return
* @throws InterruptedException
* @throws ExecutionException
*/
public Object backup(String tableName) throws InterruptedException, ExecutionException {
if(null != backUpTableList.get(tableName)) return null;
backUpTableList.put(tableName, tableName); // 鏍囪宸茬粡鐢ㄤ簬澶囦唤(闃叉鍚屾椂閲嶅澶囦唤,姣斿澶囦唤涓?釜琛ㄧ殑绾跨▼姝e湪杩愯锛屽張鍙戞潵涓?釜澶囦唤姝よ〃鐨勫懡浠?
ExecutorService pool = Executors.newFixedThreadPool(2);
Callable<Object> fhc = new DbBackUpCallable(tableName); //鍒涘缓涓?釜鏈夎繑鍥炲?鐨勭嚎绋?
Future<Object> f1 = pool.submit(fhc); //鍚姩绾跨▼
String backstr = f1.get().toString(); //鑾峰彇绾跨▼鎵ц瀹屾瘯鐨勮繑鍥炲?
pool.shutdown(); //鍏抽棴绾跨▼
return backstr;
}
/**鎵ц鏁版嵁搴撹繕鍘熷叆鍙?
* @param tableName 琛ㄥ悕
* @param sqlFilePath 澶囦唤鏂囦欢瀛樻斁瀹屾暣璺緞
* @return
* @throws InterruptedException
* @throws ExecutionException
*/
public Object recover(String tableName,String sqlFilePath) throws InterruptedException, ExecutionException {
if(null != recoverTableList.get(tableName)) return null;
recoverTableList.put(tableName, tableName); // 鏍囪宸茬粡鐢ㄤ簬杩樺師(闃叉鍚屾椂閲嶅杩樺師,姣斿杩樺師涓?釜琛ㄧ殑绾跨▼姝e湪杩愯锛屽張鍙戞潵涓?釜杩樺師姝よ〃鐨勫懡浠?
ExecutorService pool = Executors.newFixedThreadPool(2);
Callable<Object> fhc = new DbRecoverCallable(tableName,sqlFilePath); //鍒涘缓涓?釜鏈夎繑鍥炲?鐨勭嚎绋?
Future<Object> f1 = pool.submit(fhc); //鍚姩绾跨▼
String backstr = f1.get().toString(); //鑾峰彇绾跨▼鎵ц瀹屾瘯鐨勮繑鍥炲?
pool.shutdown(); //鍏抽棴绾跨▼
return backstr;
}
/**鑾峰彇鏈暟鎹簱鐨勬墍鏈夎〃鍚?
* @return
* @throws SQLException
* @throws ClassNotFoundException
*/
public static Object[] getTables() throws ClassNotFoundException, SQLException{
String dbtype = pros.getProperty("dbtype"); //鏁版嵁搴撶被鍨?
String username = pros.getProperty("username"); //鐢ㄦ埛鍚?
String password = pros.getProperty("password"); //瀵嗙爜
String address = pros.getProperty("dbAddress"); //鏁版嵁搴撹繛鎺ュ湴鍧?
String dbport = pros.getProperty("dbport"); //绔彛
String databaseName = pros.getProperty("databaseName"); //鏁版嵁搴撳悕
Connection conn = DbFH.getCon(dbtype,username,password,address+":"+dbport,databaseName);
Object[] arrOb = {databaseName,DbFH.getTablesByCon(conn, "sqlserver".equals(dbtype)?null:databaseName),dbtype};
return arrOb;
}
/**
* @return 鑾峰彇conn瀵硅薄
* @throws ClassNotFoundException
* @throws SQLException
*/
public static Connection getFHCon() throws ClassNotFoundException, SQLException{
String dbtype = pros.getProperty("dbtype"); //鏁版嵁搴撶被鍨?
String username = pros.getProperty("username"); //鐢ㄦ埛鍚?
String password = pros.getProperty("password"); //瀵嗙爜
String address = pros.getProperty("dbAddress"); //鏁版嵁搴撹繛鎺ュ湴鍧?
String dbport = pros.getProperty("dbport"); //绔彛
String databaseName = pros.getProperty("databaseName"); //鏁版嵁搴撳悕
return DbFH.getCon(dbtype,username,password,address+":"+dbport,databaseName);
}
/**
* @param dbtype 鏁版嵁搴撶被鍨?
* @param username 鐢ㄦ埛鍚?
* @param password 瀵嗙爜
* @param dburl 鏁版嵁搴撹繛鎺ュ湴鍧?绔彛
* @param databaseName 鏁版嵁搴撳悕
* @return
* @throws SQLException
* @throws ClassNotFoundException
*/
public static Connection getCon(String dbtype,String username,String password,String dburl,String databaseName) throws SQLException, ClassNotFoundException{
if("mysql".equals(dbtype)){
Class.forName("com.mysql.jdbc.Driver");
return DriverManager.getConnection("jdbc:mysql://"+dburl+"/"+databaseName+"?user="+username+"&password="+password);
}else if("oracle".equals(dbtype)){
Class.forName("oracle.jdbc.driver.OracleDriver");
return DriverManager.getConnection("jdbc:oracle:thin:@"+dburl+":"+username, databaseName, password);
}else if("sqlserver".equals(dbtype)){
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
return DriverManager.getConnection("jdbc:sqlserver://"+dburl+"; DatabaseName="+databaseName, username, password);
}else{
return null;
}
}
/**鑾峰彇鏌愪釜conn涓嬬殑鎵?湁琛?
* @param conn 鏁版嵁搴撹繛鎺ュ璞?
* @param schema mysql:鏁版嵁搴撳悕; oracle:鐢ㄦ埛鍚?sqlserver:null
* @return
*/
public static List<String> getTablesByCon(Connection conn, String schema) {
try {
List<String> listTb = new ArrayList<String>();
DatabaseMetaData meta = conn.getMetaData();
ResultSet rs = meta.getTables(null, schema, null, new String[] { "TABLE" });
while (rs.next()) {
listTb.add(rs.getString(3));
}
return listTb;
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return null;
}
/**鐢ㄤ簬鎵ц鏌愯〃鐨勫浠?鍐呴儴绫?绾跨▼
* Callable 鏈夎繑鍥炲?鐨勭嚎绋嬫帴鍙?
*/
class DbBackUpCallable implements Callable<Object>{
String tableName = null;
public DbBackUpCallable(String tableName){
this.tableName = tableName;
}
public Object call() {
try {
String remoteDB = pros.getProperty("remoteDB"); //鏄惁杩滅▼澶囦唤鏁版嵁搴?yes or no
String DBSeverport = pros.getProperty("DBSeverport"); //杩滅▼鏈嶅姟鍣ㄥ浠界▼搴忕鍙?
String dbtype = pros.getProperty("dbtype"); //鏁版嵁搴撶被鍨?
String username = pros.getProperty("username"); //鐢ㄦ埛鍚?
String password = pros.getProperty("password"); //瀵嗙爜
String address = pros.getProperty("dbAddress"); //鏁版嵁搴撹繛鎺ュ湴鍧?
String databaseName = pros.getProperty("databaseName"); //鏁版嵁搴撳悕
String dbpath = pros.getProperty("dbpath"); //鏁版嵁搴撶殑瀹夎璺緞
String sqlpath = pros.getProperty("sqlFilePath"); //瀛樺偍璺緞
String ffilename = DateUtil.getSdfTimes();
String commandStr = "";
if(!"sqlserver".equals(dbtype))
没有合适的资源?快使用搜索试试~ 我知道了~
hr.zip_HR项目_core hr_hr_hr管理平台
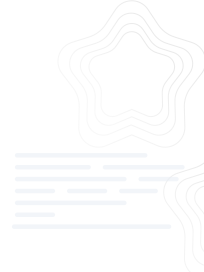
共1780个文件
svn-base:991个
gif:261个
html:98个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 64 浏览量
2022-09-21
20:46:09
上传
评论
收藏 5.06MB ZIP 举报
温馨提示
HR平台,HR管理软件,用于企业HR资源管理。 包含招聘、培训、薪酬等6大模块内容
资源详情
资源评论
资源推荐
收起资源包目录

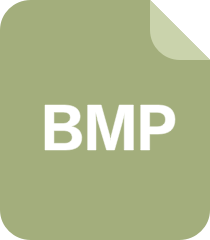
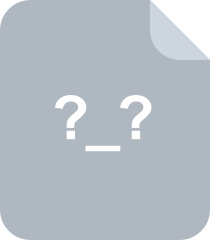
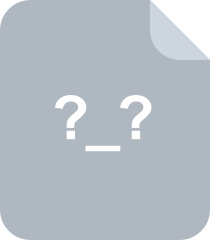
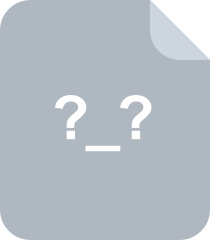
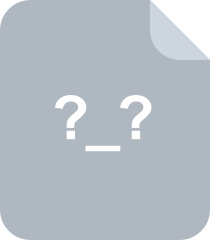
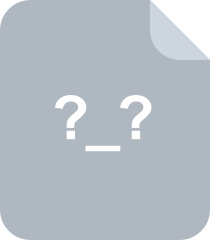
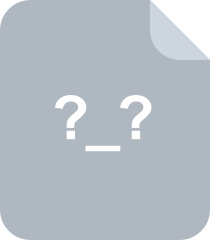
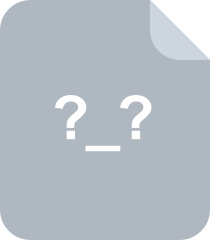
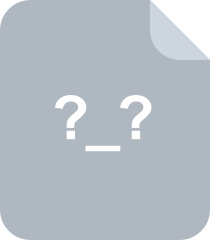
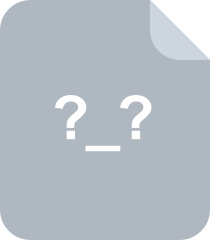
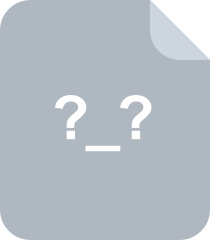
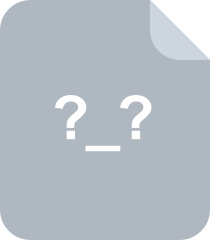
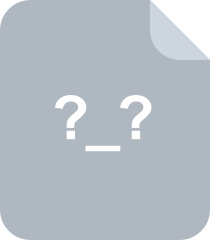
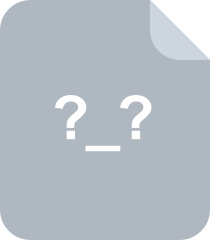
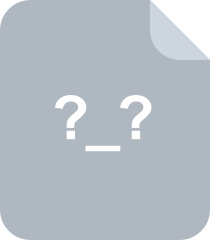
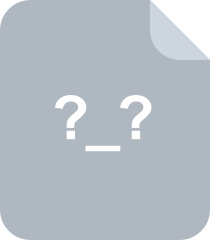
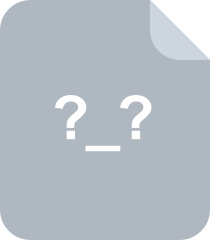
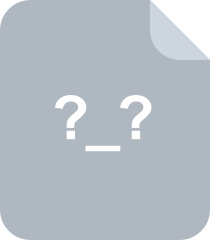
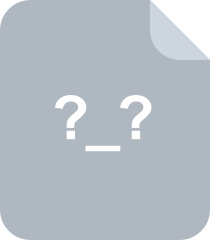
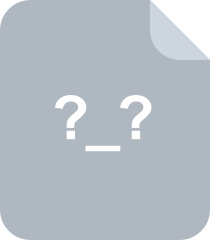
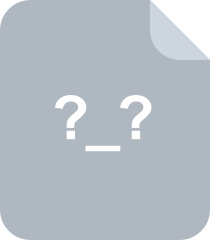
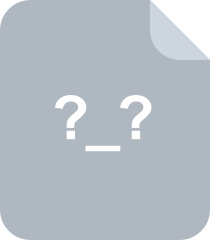
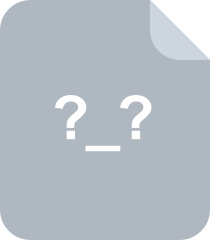
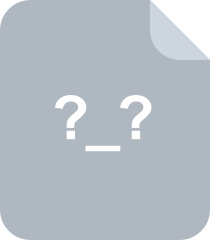
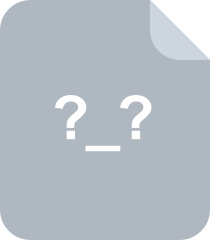
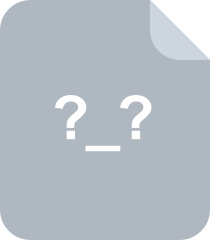
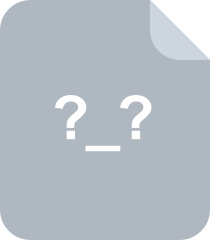
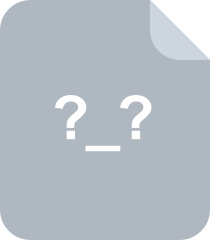
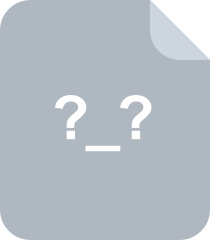
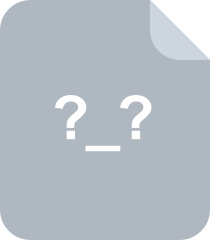
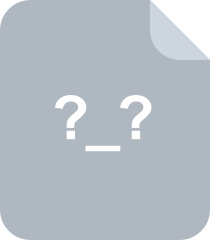
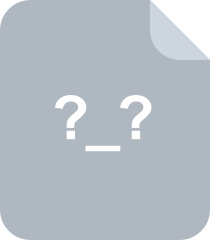
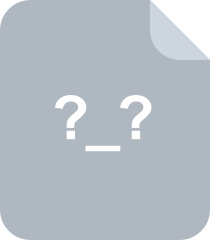
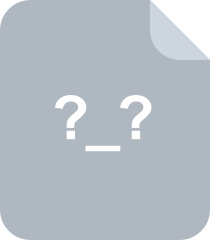
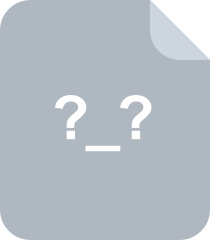
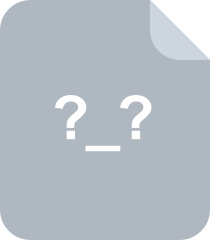
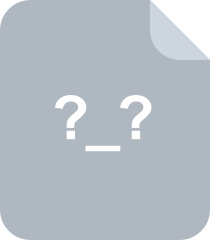
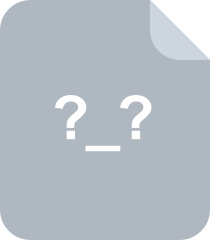
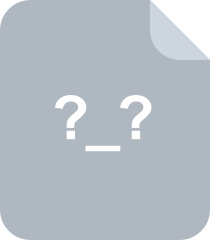
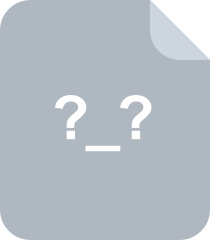
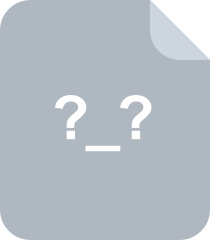
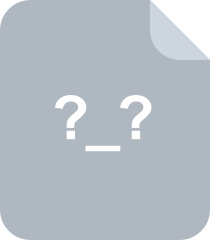
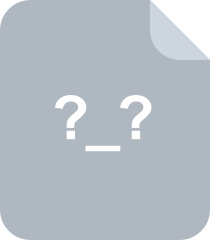
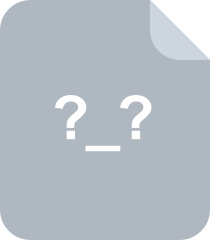
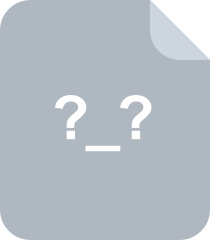
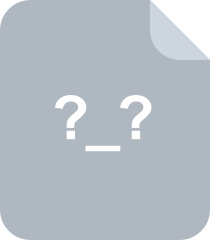
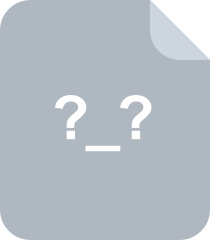
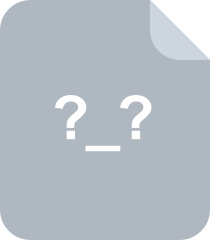
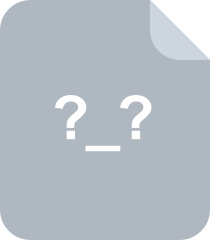
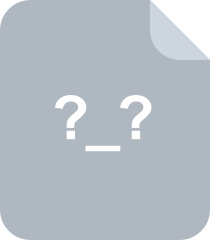
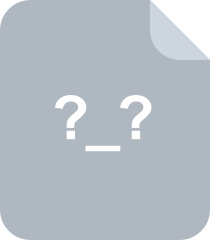
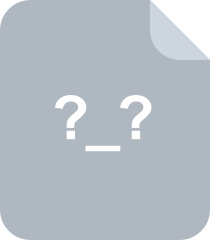
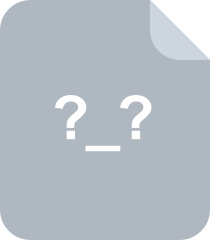
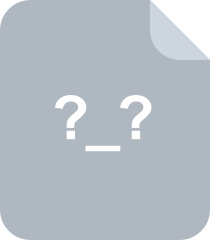
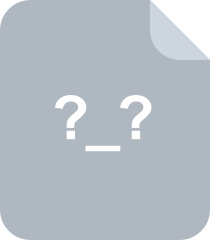
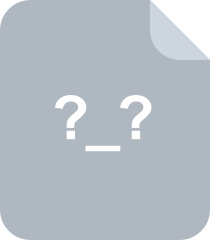
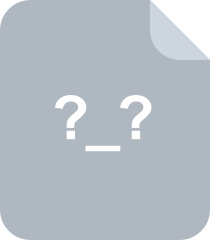
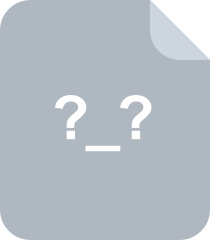
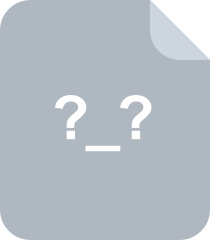
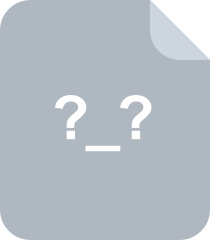
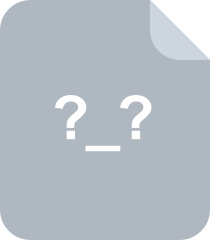
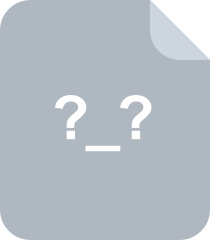
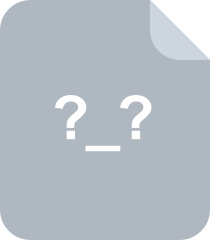
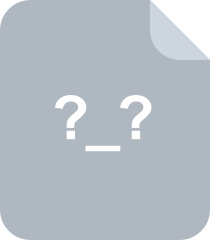
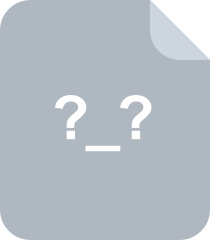
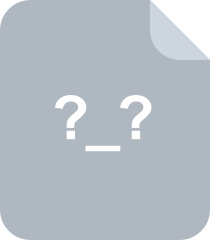
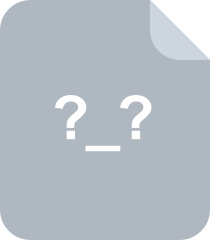
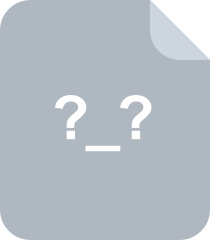
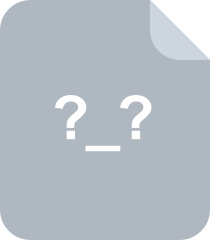
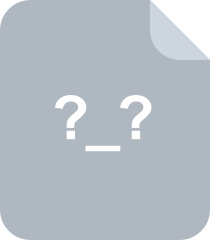
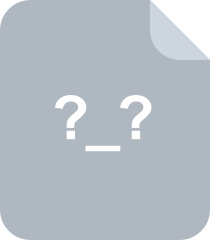
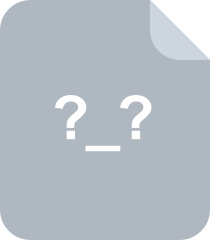
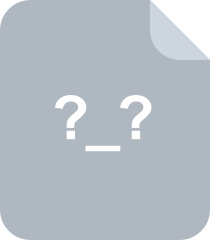
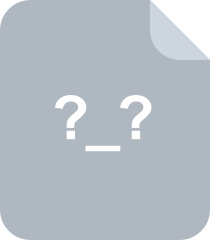
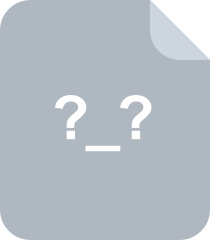
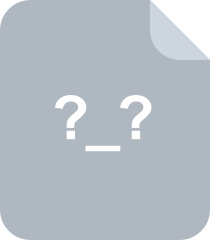
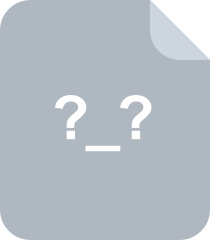
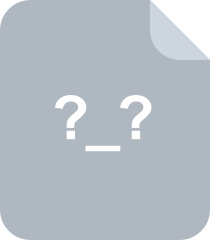
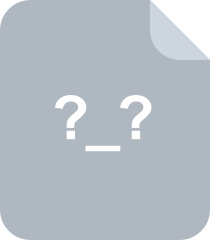
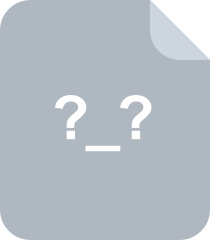
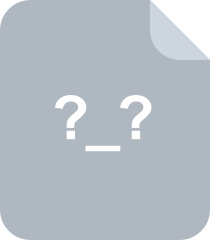
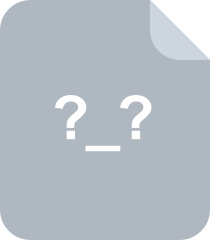
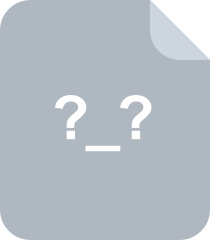
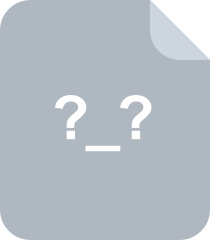
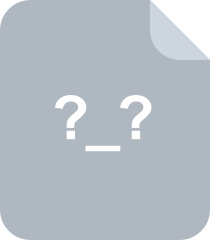
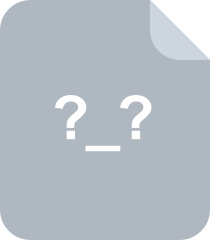
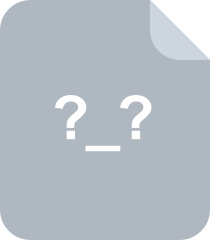
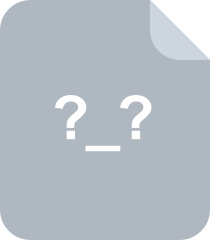
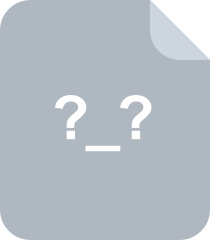
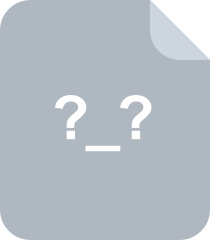
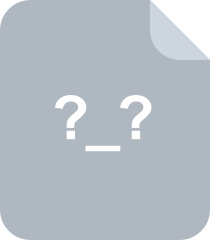
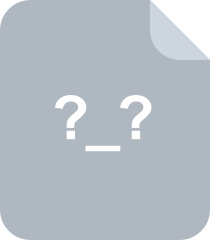
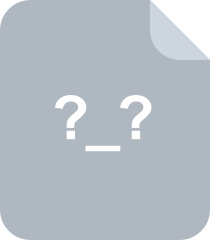
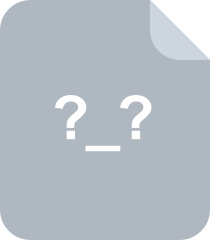
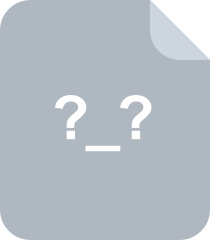
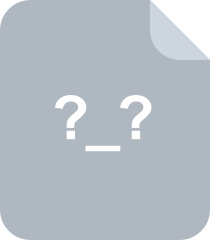
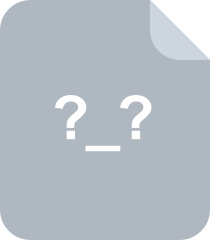
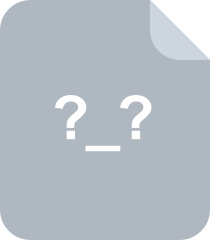
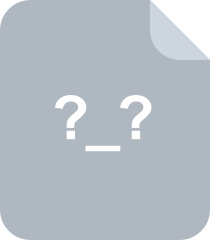
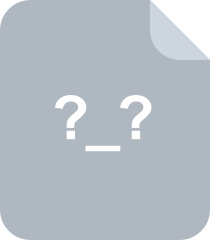
共 1780 条
- 1
- 2
- 3
- 4
- 5
- 6
- 18
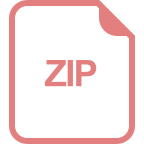
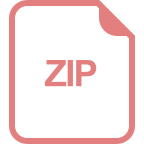
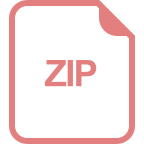
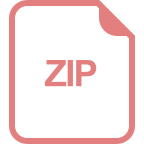
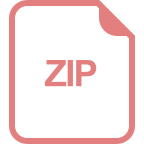
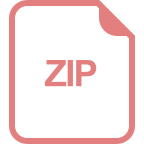
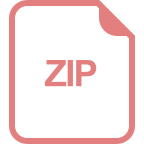
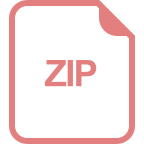
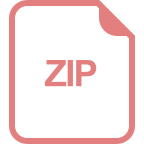
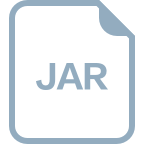
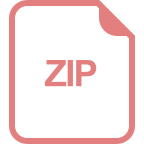
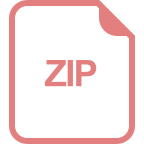
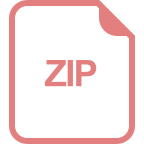
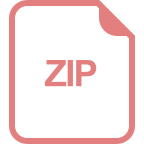
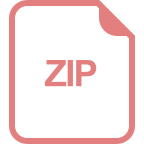
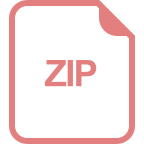
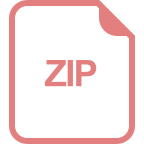
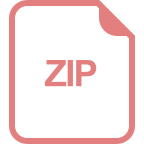

alvarocfc
- 粉丝: 105
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

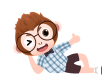
最新资源
- 同态加密python.zip
- 基于Python的PCA人脸识别算法的原理及实现代码详解+源码+详细代码解析+开发文档+数据(毕业设计&课程设计&项目开发)
- Decision tree20240105(1).ipynb
- zuoyezuoyezuoye
- zuoyezuoyezuoye
- 机械设计电机转子装配设备sw22非常好的设计图纸100%好用.zip
- 作业作业作业作业作业作业
- xdotool.c
- RLMD鲁棒性局部均值分解信号分量可视化(Matlab完整源码和数据)
- Screenshot_2024-04-26-17-17-26-36_9d26c6446fd7bb8e41d99b6262b17def.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


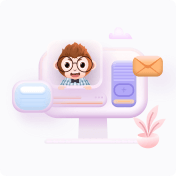
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0