//###########################################################################
//
// FILE: DSP28_DefaultIsr.c
//
// TITLE: DSP28 Device Default Interrupt Service Routines.
//
//###########################################################################
//
// Ver | dd mmm yyyy | Who | Description of changes
// =====|=============|======|===============================================
// 0.55| 06 May 2002 | L.H. | EzDSP Alpha Release
// 0.56| 20 May 2002 | L.H. | No change
// 0.57| 27 May 2002 | L.H. | No change
//###########################################################################
#include "DSP28_Device.h"
//---------------------------------------------------------------------------
// INT13, INT14, NMI, XINT1, XINT2 Default ISRs:
//
interrupt void INT13_ISR(void) // INT13 or CPU-Timer1
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void INT14_ISR(void) // CPU-Timer2
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void NMI_ISR(void) // Non-maskable interrupt
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void XINT1_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void XINT2_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
//---------------------------------------------------------------------------
// DATALOG, RTOSINT, EMUINT, RTOS Default ISRs:
//
interrupt void DATALOG_ISR(void) // Datalogging interrupt
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void RTOSINT_ISR(void) // RTOS interrupt
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void EMUINT_ISR(void) // Emulation interrupt
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
//---------------------------------------------------------------------------
// ILLEGAL Instruction Trap ISR:
//
interrupt void ILLEGAL_ISR(void) // Illegal operation TRAP
{
// Insert ISR Code here
asm(" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
//---------------------------------------------------------------------------
// USER Traps Default ISRs:
//
interrupt void USER0_ISR(void) // User Defined trap 0
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER1_ISR(void) // User Defined trap 1
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER2_ISR(void) // User Defined trap 2
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER3_ISR(void) // User Defined trap 3
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER4_ISR(void) // User Defined trap 4
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER5_ISR(void) // User Defined trap 5
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER6_ISR(void) // User Defined trap 6
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER7_ISR(void) // User Defined trap 7
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER8_ISR(void) // User Defined trap 8
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER9_ISR(void) // User Defined trap 9
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER10_ISR(void) // User Defined trap 10
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
interrupt void USER11_ISR(void) // User Defined trap 11
{
// Insert ISR Code here
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
//---------------------------------------------------------------------------
// ADC Default ISR:
//
interrupt void ADCINT_ISR(void) // ADC
{
// Insert ISR Code here
// To recieve more interrupts from this PIE group, acknowledge this interrupt
// PieCtrl.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
//---------------------------------------------------------------------------
// CPU Timer 0 Default ISR:
//
interrupt void TINT0_ISR(void) // CPU-Timer 0
{
// Insert ISR Code here
// To recieve more interrupts from this PIE group, acknowledge this interrupt
// PieCtrl.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only - remove after inserting
// ISR Code
asm (" ESTOP0");
for(;;);
// Uncomment this line after adding ISR Code
// return;
}
//---------------------------------------------------------------------------
// Watchdog Default ISR:
//
interrupt void WAKEINT_ISR(void) // WD

alvarocfc
- 粉丝: 126
- 资源: 1万+
最新资源
- 基于 Vulkan、DirectX 12 和 Metal 的高性能图形抽象 API,具有简化的编程模型 .zip
- shia.common.1127
- 基于 Rust 的默认 Minecraft 渲染器的替代品.zip
- Python和PyCharm详细安装教程与配置方法
- 项目代码YOLOv8 车间工人、安全帽安全背心识别目标检测
- 毕业设计JavaScript开发的心理咨询预约系统小程序源码(包含前端+后端两部分).zip
- 基于 Node.JS 的易于使用的多人游戏服务器 适用于 HTML5 Websocket、Unity3D(Web,PC、Android、iOS 等)、Flash、C++,OpenGL,Dire.zip
- 海信HZ65A55E(1011)刷机程序(厂商刷机包)
- 基于 GameOverlay.NET 的覆盖库,依赖于 SharpDX (DirectX).zip
- 基于 Forge API 实现的图形技术,这是一个基于 Vulkan、DirectX、Metal 的跨平台渲染框架.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


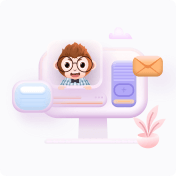