xiaopingguo.rar_S3C2440 蜂鸣器
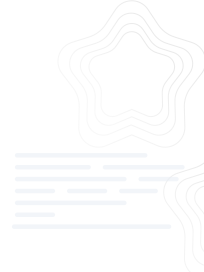

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
标题中的“xiaopingguo.rar_S3C2440 蜂鸣器”表明这是一个与S3C2440微处理器相关的项目,重点在于使用蜂鸣器播放电子音乐。S3C2440是一款由Samsung制造的ARM9处理器,常用于嵌入式系统开发,比如开发板。描述中提到“s3c2440利用蜂鸣器播放电子乐,可在开发板运行”,这意味着该项目旨在通过编程控制S3C2440处理器来驱动蜂鸣器,以播放各种音频信号,可能包括简单的旋律或节奏。 在嵌入式系统中,蜂鸣器是一种常见的硬件组件,它能够通过改变电流或电压产生不同频率的声音。在S3C2440的环境下,控制蜂鸣器通常涉及以下知识点: 1. **GPIO(General Purpose Input/Output)**:S3C2440的GPIO端口可以配置为输出模式,用以控制蜂鸣器的开和关。蜂鸣器通常连接到一个GPIO引脚,通过改变该引脚的电平状态(高电平或低电平)来控制蜂鸣器的振动频率,从而产生声音。 2. **定时器**:为了播放复杂的音乐,我们需要精确地控制蜂鸣器的开关间隔。S3C2440内置了多个定时器,可以设置为PWM(脉宽调制)模式,以生成不同频率的方波,进而控制蜂鸣器的音高。 3. **中断处理**:在连续播放音乐时,可能需要通过中断来处理其他任务,同时保持音乐播放的同步。S3C2440支持多种中断源,包括定时器中断,可以在中断服务程序中更新蜂鸣器的状态。 4. **驱动程序开发**:为了控制蜂鸣器,需要编写对应的驱动程序。这个驱动程序会与上层应用进行交互,处理音乐数据,并将其转换为对GPIO或定时器的控制命令。 5. **编译环境与工具链**:开发过程中,可能会使用交叉编译工具链,如GCC针对S3C2440的版本,以及构建系统如Makefile,确保代码能在目标平台正确运行。 6. **嵌入式操作系统**:若开发板上运行有嵌入式操作系统(如Linux、uCLinux或RTOS),则还需要理解操作系统的内核驱动模型,如设备树、驱动模型等,以及如何在用户空间通过系统调用来控制蜂鸣器。 7. **音乐编码**:如果要播放特定的音乐,可能需要将音乐文件(如MIDI或WAV)转换成适合蜂鸣器播放的简单格式,这可能涉及到音乐理论和数字音频处理的知识。 在压缩包中发现的“xiaopingguo.c”文件,很可能是实现这些功能的C语言源代码。通过分析这个源码,我们可以深入了解S3C2440如何具体控制蜂鸣器播放电子音乐,以及如何处理音乐数据和中断事件。源码的学习将有助于深入理解嵌入式系统中的硬件控制和软件编程技巧。
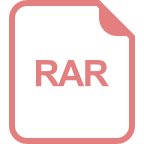
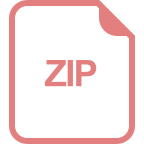
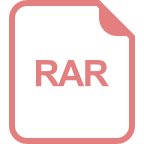
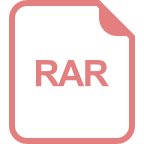
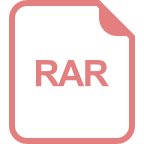
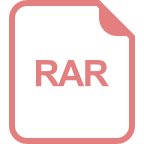
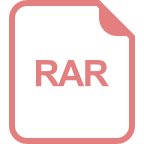
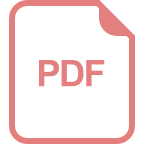
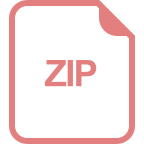
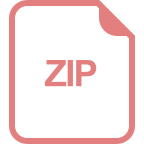
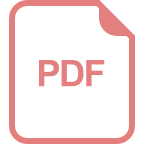
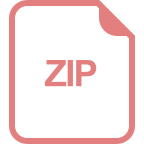

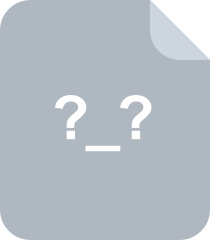
- 1
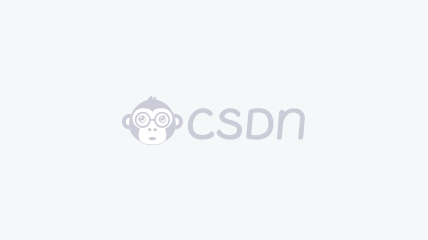

- 粉丝: 91
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

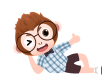
最新资源
- (源码)基于OVMS v3的无线控制台系统(WifiConsole).zip
- (源码)基于Arduino和ESP32的IoT计算机开关系统.zip
- (源码)基于Qt框架的PX4飞行控制器固件升级系统.zip
- (源码)基于Spring Boot和Vue的需求管理系统.zip
- 基于深度学习YOLOv5的车牌检测与识别项目源码
- (源码)基于Python的CSGO饰品价格分析与比较系统.zip
- ccs3.3安装补丁SR12-CCS-v3.3-SR-3.3.82.13 2
- (源码)基于Spring Boot框架的攀枝花物流系统.zip
- (源码)基于Spring Boot和Vue的权限管理系统.zip
- (源码)基于Python和HMM的酵母起始密码子预测系统.zip

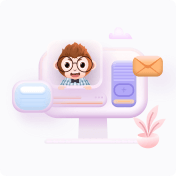
