/**
* This demonstrates password-based encryption (PBE) using the
* DES algorithm. As mentioned before, DES is not very secure,
* but the SDK version used for this example does not support
* other (stronger) PBE algorithms
*
* The text file "clear.txt" will be read, encrypted and written back
* as "clear.txt.des".
*
* I've done all the "hardcoding" of the file names and password for
* simplicity of discussion.
*
*/
import java.io.*;
import java.security.*;
import javax.crypto.*;
import javax.crypto.spec.*;
import java.util.*;
public class FileEncryptor
{
private static String filename;
private static String password;
private static FileInputStream inFile;
private static FileOutputStream outFile;
/**
* Note: As with the pervious example, all kinds of exceptions
* can be thrown in main. See the API documentation for each
* method used.
*/
public static void main(String[] args) throws Exception
{
// File to encrypt. It does not have to be a text file!
filename = "out.jpg";
// Password must be at least 8 characters (bytes) long
String password = "abc123abc";
inFile = new FileInputStream(filename);
outFile = new FileOutputStream("result.dat");
// Use PBEKeySpec to create a key based on a password.
// The password is passed as a character array
PBEKeySpec keySpec = new PBEKeySpec(password.toCharArray());
SecretKeyFactory keyFactory =
SecretKeyFactory.getInstance("PBEWithMD5AndDES");
SecretKey passwordKey = keyFactory.generateSecret(keySpec);
// PBE = hashing + symmetric encryption. A 64 bit random
// number (the salt) is added to the password and hashed
// using a Message Digest Algorithm (MD5 in this example.).
// The number of times the password is hashed is determined
// by the interation count. Adding a random number and
// hashing multiple times enlarges the key space.
byte[] salt = new byte[8];
Random rnd = new Random();
rnd.nextBytes(salt);
int iterations = 100;
//Create the parameter spec for this salt and interation count
PBEParameterSpec parameterSpec = new PBEParameterSpec(salt, iterations);
// Create the cipher and initialize it for encryption.
Cipher cipher = Cipher.getInstance("PBEWithMD5AndDES");
cipher.init(Cipher.ENCRYPT_MODE, passwordKey, parameterSpec);
// Need to write the salt to the (encrypted) file. The
// salt is needed when reconstructing the key for decryption.
outFile.write(salt);
// Read the file and encrypt its bytes.
byte[] input = new byte[64];
int bytesRead;
while ((bytesRead = inFile.read(input)) != -1)
{
byte[] output = cipher.update(input, 0, bytesRead);
if (output != null) outFile.write(output);
}
byte[] output = cipher.doFinal();
if (output != null) outFile.write(output);
inFile.close();
outFile.flush();
outFile.close();
}
}

Kinonoyomeo
- 粉丝: 91
- 资源: 1万+
最新资源
- 6吨级轻型载货汽车驱动桥的设计及虚拟装配 (1)
- Springboot 学生管理系统更删改查
- 九缸星形发动机点火器3D
- 毕业设计-宿舍管理系统设计与实现
- 全面详解Ruby编程语言,从入门到高级实战
- CC2530无线zigbee裸机代码实现红外遥控器接收IRDecod、串口打印UART、12864液晶屏显示例程.zip
- 跨平台的云端爬虫系统项目全套技术资料.zip
- CC2530无线zigbee裸机代码实现红外遥控发送、串口打印、LCD12864液晶屏、按键程序.zip
- Delphi编程语言从基础知识到高级应用全面指南
- Scratch图形化编程语言入门与进阶指南
- 酒店后台管理系统项目全套技术资料.zip
- CC2530无线zigbee裸机代码实现RS485串口通讯.zip
- Day-03 Vue222222222222222222222
- Visual Basic语言入门与进阶教程
- 数据安全治理白皮书6.0-2024年最新版
- 基于pygame的圣诞小游戏
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


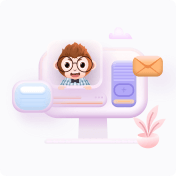