/*
* mpkgTool program for EZX
* Copyright (C) 2007 Lasly <Flylasly@gmail.com>
*
*/
//#define QT_NO_TEXTCODEC
#define QT_NO_DRAGANDDROP
#define QT_NO_PROPERTIES
#include <E2_EZX_ZApplication.h>
#include <E2_EZX_ZKbMainWidget.h>
#include <E2_EZX_ZMessageDlg.h>
#include <E2_EZX_ZLabel.h>
#include <E2_EZX_ZScrollPanel.h>
#include <E2_EZX_ZSoftKey.h>
#include <E2_EZX_ZOptionsMenu.h>
#include <E2_EZX_ZComboBox.h>
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <qfile.h>
#include <qtextcodec.h>
#include <qlayout.h>
#include <qdir.h>
#include <pthread.h>
QTextCodec* gbk_codec = QTextCodec::codecForName("UTF-8");
QString ChineseAndEnglish(const char*ChineseString)
{
return gbk_codec->toUnicode(ChineseString);
}
typedef struct MpkgProgram
{
char name[64];
char showName[24];
char path[96];
char id[48];
int domain;
int isCard;
};
int initMpkgProgram(MpkgProgram** pplist )
{
QDir dir("/ezxlocal/.system/QTDownLoad");
int count = (int)dir.count();
dir.setPath("/mmc/mmca1/.system/QTDownLoad");
count += (int)dir.count();
*pplist = new MpkgProgram[count];
int index = 0;
if(*pplist != NULL )
{
QFile file("/mmc/mmca1/.system/java/CardRegistry");
int isMpkg = 0;
char tmp[256];
if( file.open(IO_ReadWrite) )
{
isMpkg = 0;
while( file.readLine( tmp, 256 ) != -1 && index < count)
{
if( tmp[0] == '[' )
{
if( isMpkg )
{
index++;
isMpkg = 0;
}
(*pplist)[index].isCard = 1;
strcpy( (*pplist)[index].id , tmp);
}
else if( strstr( tmp , "Directory" ) == tmp )
{
strcpy( (*pplist)[index].path , "/mmc/mmca1/.system/QTDownLoad/" );
strcat( (*pplist)[index].path , tmp + 12 );
(*pplist)[index].path[strlen((*pplist)[index].path) - 1 ] = 0;
}
else if( strstr( tmp , "Name" ) == tmp )
{
strcpy( (*pplist)[index].name , tmp + 7 );
(*pplist)[index].name[strlen((*pplist)[index].name) - 1 ] = 0;
if( strlen((*pplist)[index].name) > 20 )
{
memset((*pplist)[index].showName , 0 , 24 );
strncpy( (*pplist)[index].showName , (*pplist)[index].name , 16 );
strcat( (*pplist)[index].showName , "..." );
}
else
{
strcpy( (*pplist)[index].showName , (*pplist)[index].name );
}
}
else if( strstr( tmp , "GroupID" ) == tmp )
{
if( strstr( tmp , "ezx" ) != NULL )
{
(*pplist)[index].domain = 0;
}
else
{
(*pplist)[index].domain = 1;
}
}
else if( strstr( tmp , "MpkgFile" ) == tmp )
{
if( strchr( tmp , '/' ) != NULL )
{
isMpkg = 1;
}
}
}
file.close();
}
if( isMpkg )
{
index++;
}
isMpkg = 0;
// copy ^o^
QFile filephone("/ezx_user/download/appwrite/am/InstalledDB");
if( filephone.open(IO_ReadWrite) )
{
while( filephone.readLine( tmp, 256 ) != -1 && index < count )
{
if( tmp[0] == '[' )
{
if( isMpkg )
{
index++;
isMpkg = 0;
}
(*pplist)[index].isCard = 0;
strcpy( (*pplist)[index].id , tmp);
}
else if( strstr( tmp , "Directory" ) == tmp )
{
strcpy( (*pplist)[index].path , "/ezxlocal/.system/QTDownLoad/" );
strcat( (*pplist)[index].path , tmp + 12 );
(*pplist)[index].path[strlen((*pplist)[index].path) - 1 ] = 0;
}
else if( strstr( tmp , "Name" ) == tmp )
{
strcpy( (*pplist)[index].name , tmp + 7 );
(*pplist)[index].name[strlen((*pplist)[index].name) - 1 ] = 0;
if( strlen((*pplist)[index].name) > 20 )
{
memset((*pplist)[index].showName , 0 , 24 );
strncpy( (*pplist)[index].showName , (*pplist)[index].name , 16 );
strcat( (*pplist)[index].showName , "..." );
}
else
{
strcpy( (*pplist)[index].showName , (*pplist)[index].name );
}
}
else if( strstr( tmp , "GroupID" ) == tmp )
{
if( strstr( tmp , "ezx" ) != NULL )
{
(*pplist)[index].domain = 0;
}
else
{
(*pplist)[index].domain = 1;
}
}
else if( strstr( tmp , "MpkgFile" ) == tmp )
{
if( strchr( tmp , '/' ) != NULL )
{
isMpkg = 1;
}
}
}
filephone.close();
}
if( isMpkg )
{
index++;
}
}
if( index == 0 )
{
system("showQ \"\" 没有安装任何mpkg程序! 2");
exit(0);
}
return index;
}
void setDomain(char* id , int domain , int isCard)
{
char tmp[256];
if( isCard )
{
strcpy( tmp , "/mmc/mmca1/.system/java/CardRegistry");
}
else
{
strcpy( tmp , "/ezx_user/download/appwrite/am/InstalledDB");
}
QFile file(tmp);
if( file.open(IO_ReadWrite) )
{
printf(tmp);
int size = file.size();
char* buff = new char[size];
file.readBlock(buff , size);
file.close();
file.remove();
if( file.open(IO_ReadWrite) )
{
char* pre = strstr(buff , id);
char* pgroup = strstr( pre , "\nGroupID" );
file.writeBlock(buff , pgroup - buff + 11 );
switch( domain )
{
case 1:
file.writeBlock("root" , 4 );
break;
case 0:
default:
file.writeBlock("ezx" , 3 );
break;
}
pre = strchr( pgroup + 1, '\n' );
char* puserid = strstr( pre , "\nUserID" );
file.writeBlock(pre , puserid - pre + 10 );
switch( domain )
{
case 1:
file.writeBlock("root" , 4 );
break;
case 0:
default:
file.writeBlock("ezx" , 3 );
break;
}
pre = strchr( puserid + 1, '\n' );
file.writeBlock(pre , size - ( pre - buff ) );
}
file.close();
delete[] buff;
}
}
void* removeMpkg(void* p );
class MyDialog : public ZKbMainWidget
{
Q_OBJECT
public:
ZComboBox* nameBox;
ZComboBox* domainBox;
ZLabel* infoLabel;
MpkgProgram* pMpkgList;
MyDialog( ):ZKbMainWidget((ZHeader::HEADER_TYPE)3,NULL , "ZMainWidget" , 0)
{
setMainWidgetTitle(ChineseAndEnglish("mpkg管理工具"));
ZSoftKey* softKey = new ZSoftKey(NULL , this , this);
QRect rect;
ZOptionsMenu* menu = new ZOptionsMenu(rect, softKey, NULL , 0 ,(ZSkinService::WidgetClsID)2 );
menu->insertItem(ChineseAndEnglish("卸载") , NULL , NULL , true , 0 , 0 );
menu->insertItem(ChineseAndEnglish("退出") , NULL , NULL , true , 1 , 1 );
softKey->setOptMenu(ZSoftKey::LEFT, menu);
softKey->setText(ZSoftKey::LEFT, ChineseAndEnglish("选项"), (ZSoftKey::TEXT_PRIORITY)0);
softKey->setText(ZSoftKey::RIGHT, ChineseAndEnglish("关于"), (ZSoftKey::TEXT_PRIORITY)0);
softKey->setClickedSlot(ZSoftKey::RIGHT, this, SLOT(about()));
menu->connectItem(0, this, SLOT(remove()));
menu->connectItem(1, qApp, SLOT(quit()));
nameBox = new ZComboBox(this,"name",true);
domainBox = new ZComboBox(this,"domainBox",true);
infoLabel = new ZLabel(ChineseAndEnglish("") , this, "ZLabel", 0, (ZSkinService::WidgetClsID)4);
infoLabel->setPreferredWidth(240);
infoLabel->setAutoResize(true );
initNameBox();
domainBox->insertItem(ChineseAndEnglish("ezx"), 0 );
domainBox->insertItem(ChineseAndEnglish("root"), 1 );
QVBoxLayout* layout = getVBoxLayout();
ZScrollPanel *zsv = new ZScrollPanel(this , NULL , 0 , (ZSkinService::WidgetClsID)4);
int height = heightForWidth(240) + 10;
zsv->addChild(nameBox , 10 , height);
height += (nameBox->getContentRect()).height() + nameBox->getBtnHSpacing() * 4 ;
zsv->addChild(domainBox , 10 , height);
height += (domainBox->getContentRect()).height() + domainBox->getBtnHSpacing() * 4 ;
zsv->addChild(infoLabel , 10 , height);
layout->addWidget(zsv);
setSoftKey(softKey);
dlg = NULL;
startTimer(500);
currentIndex = -1;
}
~MyDialog( )
{
killTimers();
if( pMpkgList != NULL )
{
delete[] pMpkgList;
}
if( dlg != NULL )
{
delete dlg;
}
}
void initNameBox()
{
int number = initMpkgProgram( &pMpkgList );
nameBox->clear();
for( int i = 0 ; i < number ; i++ )
{
nameBox->insertItem(ChineseAndEnglish(pMpkgList[i].showName), i );
}
if( number > 0 )
{
nameBox->setCurrentItem( 0 );
}
else
{
delete[] pMpkgList;
pMpkgList = NULL;
QString a = ChineseAndEnglish("Error");
QString b = C
没有合适的资源?快使用搜索试试~ 我知道了~
e2-ezx-sdk_0.02_beta.tar.gz_beta
1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 182 浏览量
2022-09-19
16:54:55
上传
评论
收藏 10.54MB GZ 举报
温馨提示
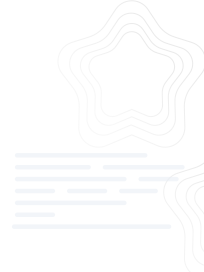
共541个文件
h:351个
so:139个
cpp~:12个

e2-ezx-sdk是用于开发摩托罗拉e2手机应用程序的开发工具,使用C++开发
资源推荐
资源详情
资源评论
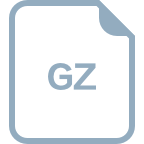
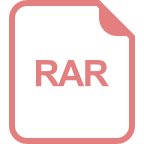
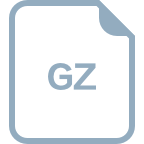
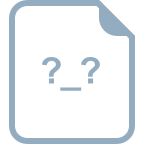
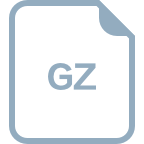
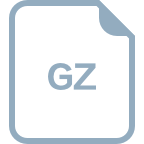
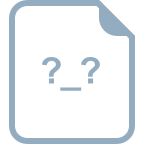
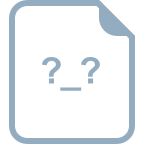
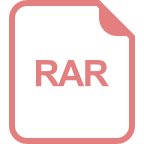
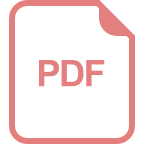
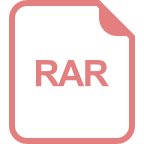
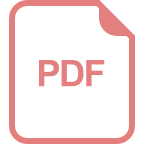
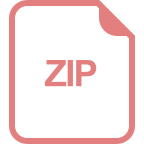
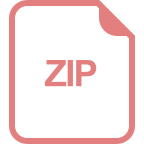
收起资源包目录

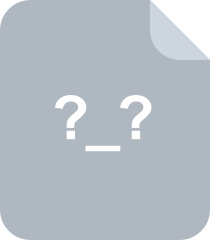
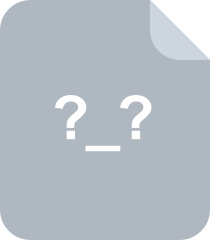
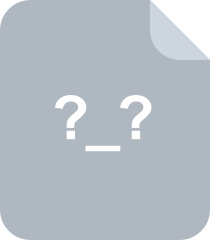
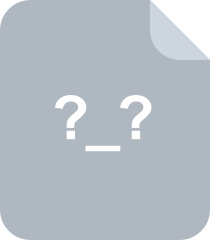
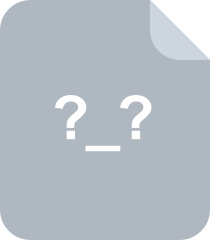
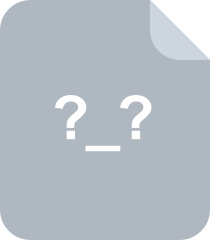
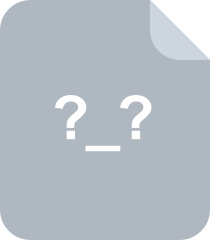
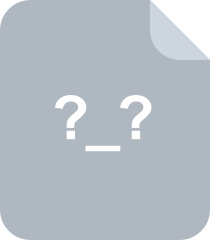
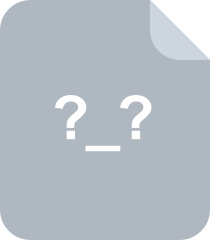
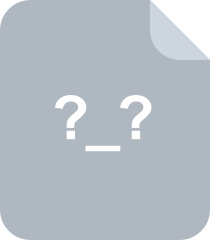
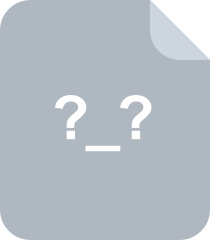
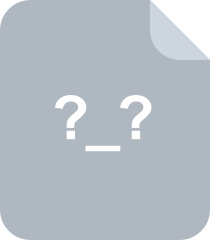
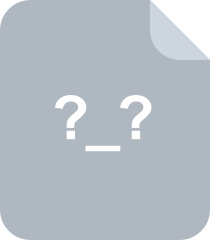
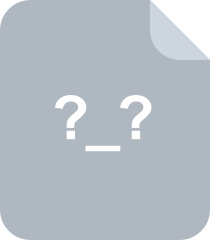
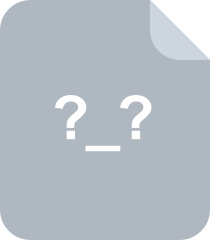
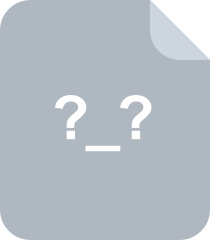
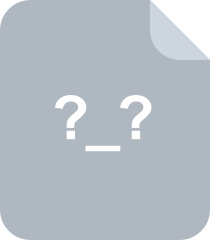
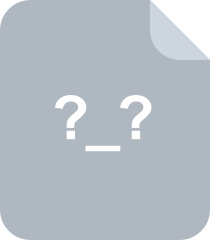
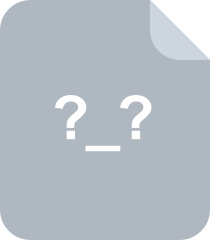
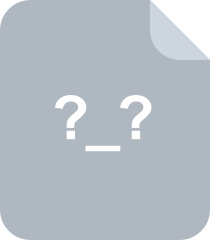
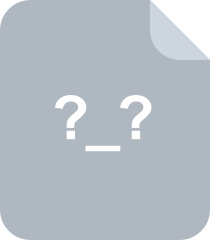
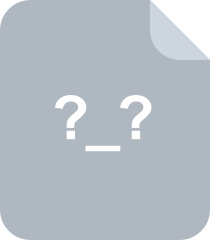
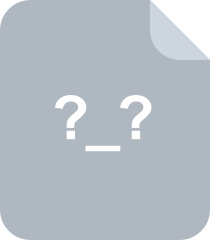
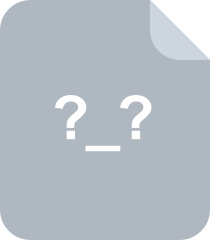
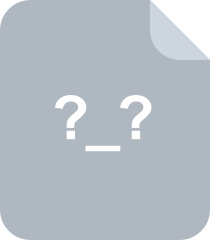
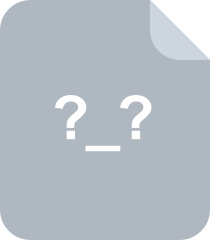
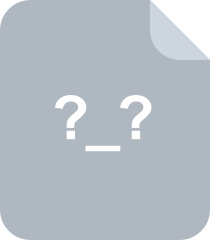
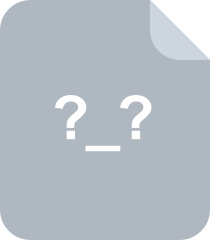
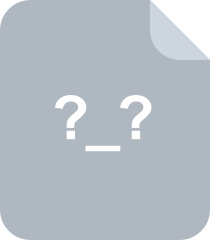
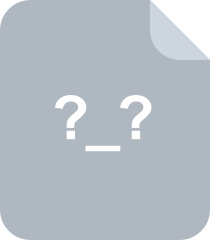
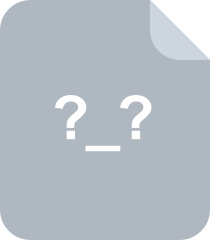
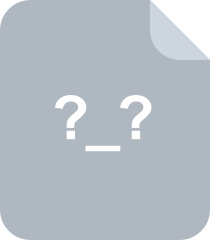
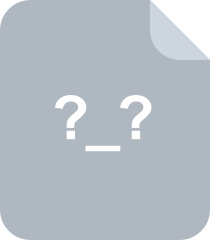
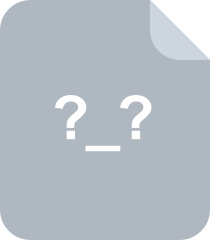
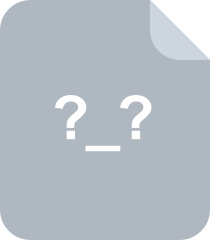
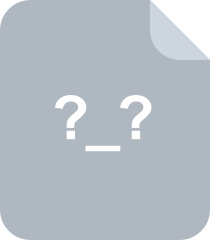
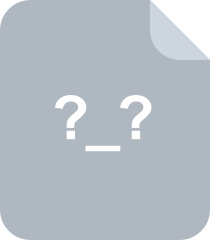
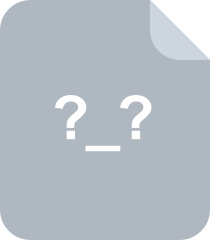
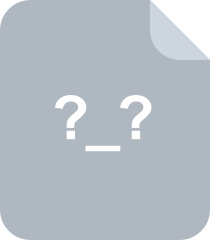
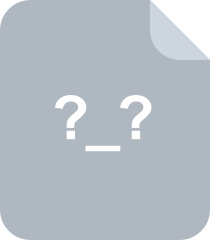
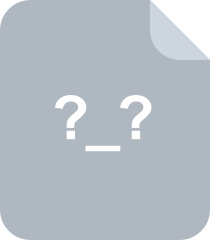
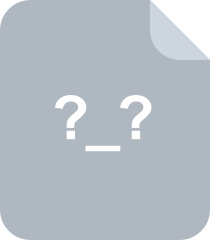
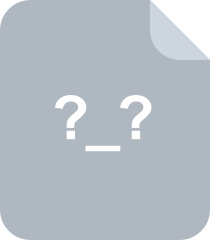
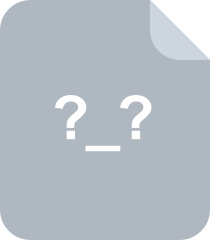
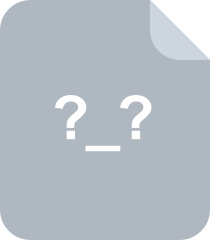
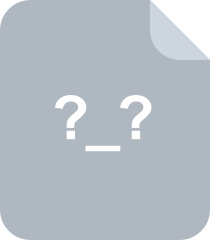
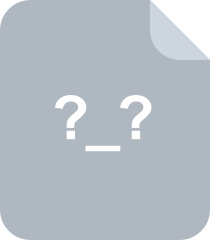
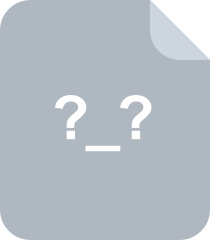
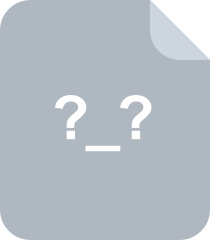
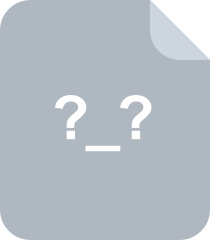
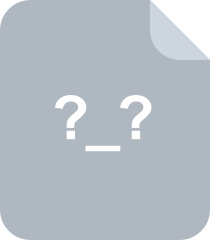
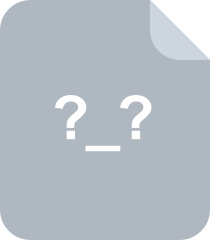
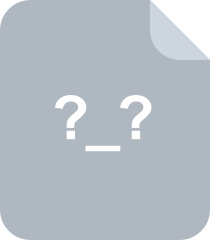
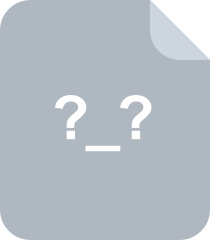
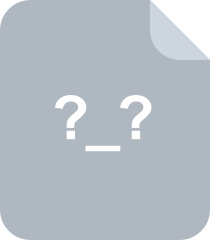
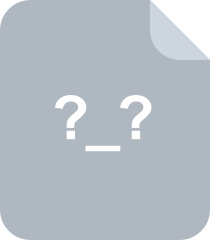
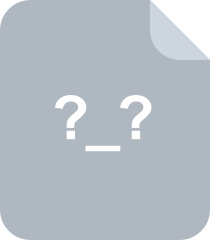
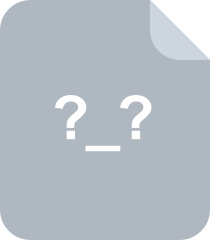
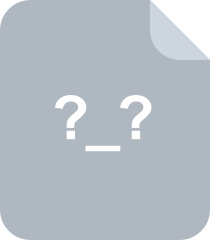
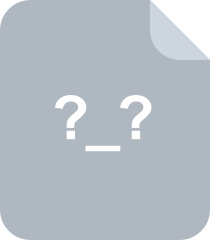
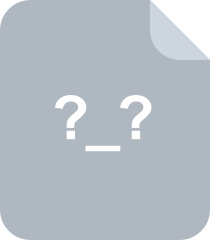
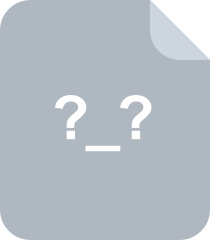
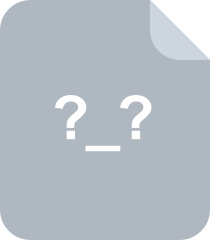
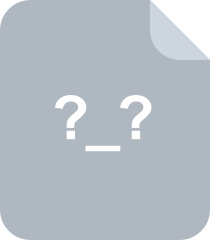
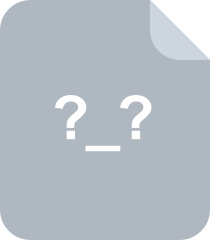
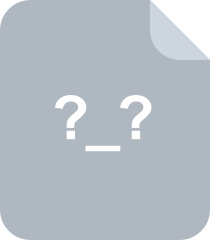
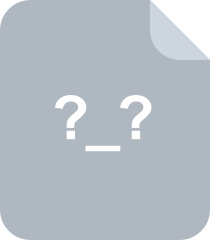
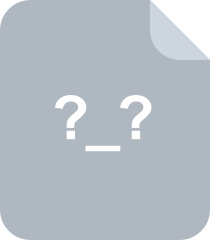
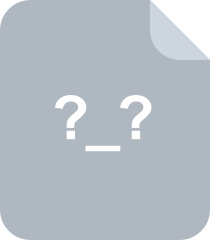
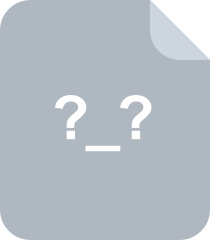
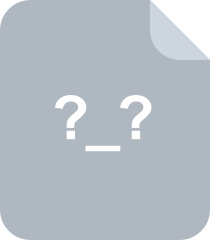
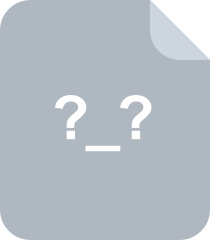
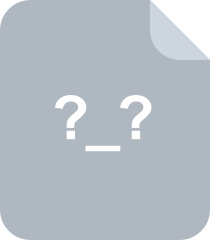
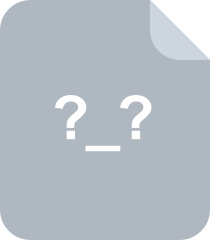
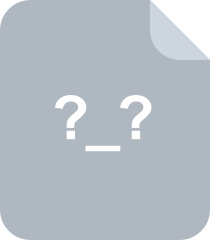
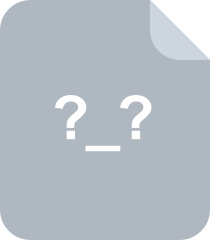
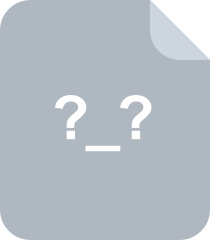
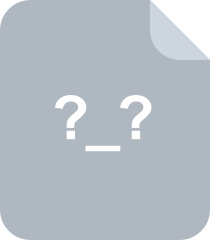
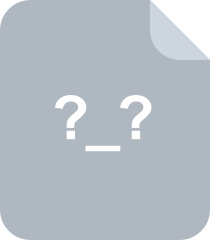
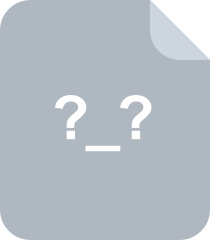
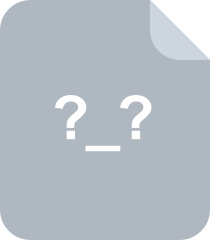
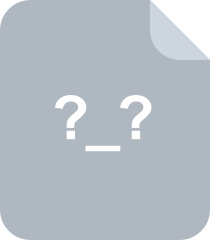
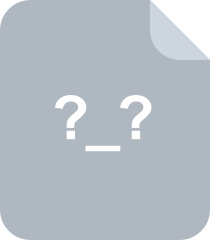
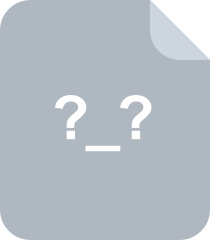
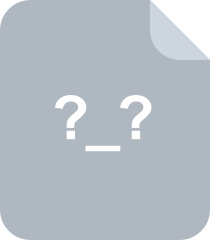
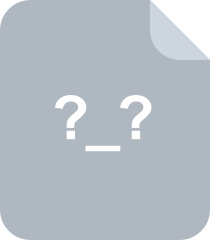
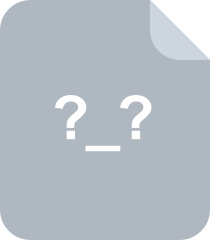
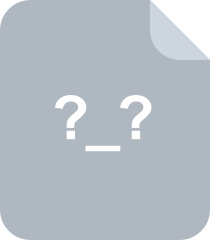
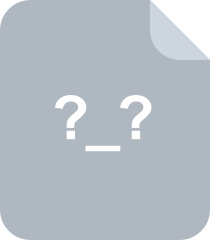
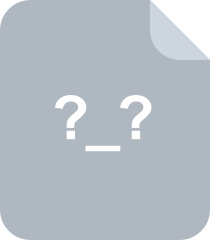
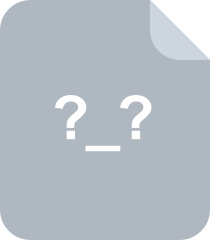
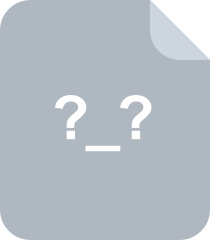
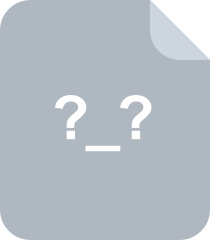
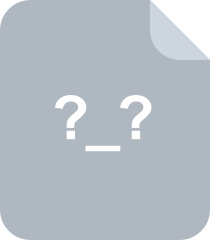
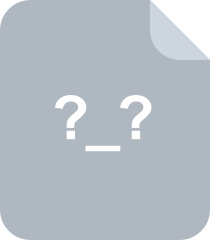
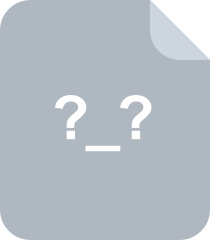
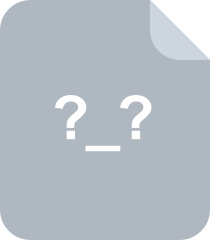
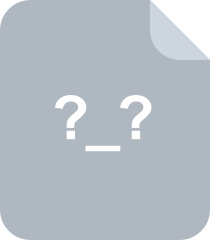
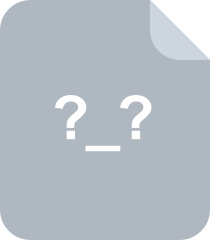
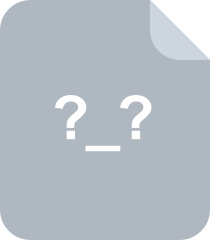
共 541 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
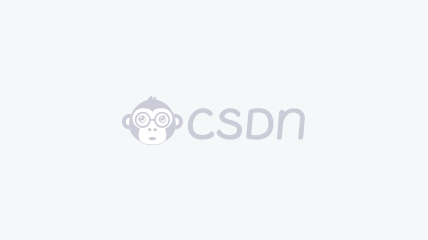

Kinonoyomeo
- 粉丝: 79
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

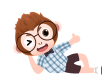
安全验证
文档复制为VIP权益,开通VIP直接复制
