// JXL File $Revision: /main/8 $
// Checkin $Date: April 20, 2008 14:40:00 $
//###########################################################################
//
// FILE: MPPT.c
//
// TITLE: DSP2808 MPPT Program.
//
// ASSUMPTIONS:
//
// This program requires the DSP280x header files.
//
// Make sure the CPU clock speed is properly defined in
// DSP280x_Examples.h before compiling this example.
//
// Connect signals to be converted to A2 and A3.
//
// As supplied, this project is configured for "boot to SARAM"
// operation. The 280x Boot Mode table is shown below.
// For information on configuring the boot mode of an eZdsp,
// please refer to the documentation included with the eZdsp,
//
// Boot GPIO18 GPIO29 GPIO34
// Mode SPICLKA SCITXDA
// SCITXB
// -------------------------------------
// Flash 1 1 1
// SCI-A 1 1 0
// SPI-A 1 0 1
// I2C-A 1 0 0
// ECAN-A 0 1 1
// SARAM 0 1 0 <- "boot to SARAM"
// OTP 0 0 1
// I/0 0 0 0
//
//
// DESCRIPTION:
//
// This Example sets up the PLL in x10/2 mode (for 100 Mhz devices)
// or x6/2 mode (for 60 Mhz devices), divides SYSCLKOUT
// by eight to reach a 12.5Mhz (100 Mhz devices) or a 7.5 Mhz (60 Mhz
// devices) HSPCLK (assuming a 20Mhz XCLKIN)
// Interrupts are enabled and the ePWM1 is setup to generate a periodic
// ADC SOC on SEQ1. Two channels are converted, ADCINA3 and ADCINA2.
//
// Watch Variables:
//
// Voltage1[10] Last 10 ADCRESULT0 values
// Voltage2[10] Last 10 ADCRESULT1 values
// ConversionCount Current result number 0-9
// LoopCount Idle loop counter
//
//
//###########################################################################
//
// Original Author: D.F.
//
// $TI Release: DSP280x Header Files V1.60 $
// $Release Date: December 3, 2007 $
//###########################################################################
#include "DSP280x_Device.h" // DSP280x Headerfile Include File
#include "DSP280x_Examples.h" // DSP280x Examples Include File
#pragma DATA_SECTION(buffer1, ".saram");
#pragma DATA_SECTION(buffer2, ".saram");
int buffer1[512];
int buffer2[512];
unsigned int buffer_index = 0;
unsigned pv_time = 0, RST_SEQ_flag = 0, Duty1 = 500, Duty2 = 500, Duty3 = 500;
unsigned long Vold_pv1 = 0, Pnew1 = 0, Pold1 = 0, Vpv1_sum = 0, Vpv1 = 0, Ipv1 = 0, Ipv1_sum = 0, Vold_pv2 = 0, Pnew2 = 0, Pold2 = 0, Vpv2_sum = 0, Vpv2 = 0, Ipv2 = 0, Ipv2_sum = 0;
typedef struct
{
volatile struct EPWM_REGS *EPwmRegHandle;
Uint16 EPwm_CMPA_Direction;
Uint16 EPwm_CMPB_Direction;
Uint16 EPwmTimerIntCount;
Uint16 EPwmMaxCMPA;
Uint16 EPwmMinCMPA;
Uint16 EPwmMaxCMPB;
Uint16 EPwmMinCMPB;
}EPWM_INFO;
static void decDuty1(void)
{
if (EPwm1Regs.CMPA.half.CMPA < 1950)
{
EPwm1Regs.CMPA.half.CMPA = Duty1++;
EPwm1Regs.CMPA.half.CMPA = Duty1++;
// EPwm2Regs.CMPA.half.CMPA = Duty2++;
// EPwm2Regs.CMPA.half.CMPA = Duty2++;
}
}
static void decDuty2(void)
{
if (EPwm2Regs.CMPA.half.CMPA < 1950)
{
EPwm2Regs.CMPA.half.CMPA = Duty2++;
EPwm2Regs.CMPA.half.CMPA = Duty2++;
// EPwm2Regs.CMPA.half.CMPA = Duty2++;
// EPwm2Regs.CMPA.half.CMPA = Duty2++;
}
}
static void decDuty3(void)
{
if (EPwm3Regs.CMPA.half.CMPA < 950)
{
EPwm3Regs.CMPA.half.CMPA = Duty3++;
EPwm3Regs.CMPA.half.CMPA = Duty3++;
// EPwm3Regs.CMPA.half.CMPA = Duty3++;
// EPwm3Regs.CMPA.half.CMPA = Duty3++;
}
}
static void incDuty1(void)
{
if (EPwm1Regs.CMPA.half.CMPA > 50)
{
EPwm1Regs.CMPA.half.CMPA = Duty1--;
EPwm1Regs.CMPA.half.CMPA = Duty1--;
// EPwm2Regs.CMPA.half.CMPA = Duty2--;
// EPwm2Regs.CMPA.half.CMPA = Duty2--;
}
}
static void incDuty2(void)
{
if (EPwm2Regs.CMPA.half.CMPA > 50)
{
EPwm2Regs.CMPA.half.CMPA = Duty2--;
EPwm2Regs.CMPA.half.CMPA = Duty2--;
// EPwm2Regs.CMPA.half.CMPA = Duty2--;
// EPwm2Regs.CMPA.half.CMPA = Duty2--;
}
}
static void incDuty3(void)
{
if (EPwm3Regs.CMPA.half.CMPA > 50)
{
EPwm3Regs.CMPA.half.CMPA = Duty3--;
EPwm3Regs.CMPA.half.CMPA = Duty3--;
// EPwm3Regs.CMPA.half.CMPA = Duty3--;
// EPwm3Regs.CMPA.half.CMPA = Duty3--;
}
}
// Prototype statements for functions found within this file.
interrupt void adc_isr(void);
void InitEPwm1Example(void);
void InitEPwm2Example(void);
void InitEPwm3Example(void);
interrupt void epwm1_isr(void);
interrupt void epwm2_isr(void);
void update_compare(EPWM_INFO*);
// Global variables used in this example
EPWM_INFO epwm1_info;
EPWM_INFO epwm2_info;
EPWM_INFO epwm3_info;
// Configure the period for each timer
#define EPWM1_TIMER_TBPRD 2000 // Period register
#define EPWM1_MAX_CMPA 950
#define EPWM1_MIN_CMPA 50
#define EPWM1_MAX_CMPB 950
#define EPWM1_MIN_CMPB 50
// Global variables used in this example:
//Uint16 LoopCount;
main()
{
// Step 1. Initialize System Control:
// PLL, WatchDog, enable Peripheral Clocks
// This example function is found in the DSP280x_SysCtrl.c file.
InitSysCtrl();
// For this example, set HSPCLK to SYSCLKOUT / 8 (12.5Mhz assuming 100Mhz SYSCLKOUT or 7.5 MHz assuming 60 MHz SYSCLKOUT)
EALLOW;
SysCtrlRegs.HISPCP.all = 0x4; // HSPCLK = SYSCLKOUT/8
EDIS;
// Step 2. Initialize GPIO:
// This example function is found in the DSP280x_Gpio.c file and
// illustrates how to set the GPIO to it's default state.
// InitGpio(); // Skipped for this example
// For this case just init GPIO pins for ePWM1, ePWM2, ePWM3
// These functions are in the DSP280x_EPwm.c file
InitEPwm1Gpio();
InitEPwm2Gpio();
//InitEPwm3Gpio();
// Step 3. Clear all interrupts and initialize PIE vector table:
// Disable CPU interrupts
DINT;
// Initialize the PIE control registers to their default state.
// The default state is all PIE interrupts disabled and flags
// are cleared.
// This function is found in the DSP280x_PieCtrl.c file.
InitPieCtrl();
// Disable CPU interrupts and clear all CPU interrupt flags:
IER = 0x0000;
IFR = 0x0000;
// Initialize the PIE vector table with pointers to the shell Interrupt
// Service Routines (ISR).
// This will populate the entire table, even if the interrupt
// is not used in this example. This is useful for debug purposes.
// The shell ISR routines are found in DSP280x_DefaultIsr.c.
// This function is found in DSP280x_PieVect.c.
InitPieVectTable();
// Interrupts that are used in this example are re-mapped to
// ISR functions found within this file.
EALLOW; // This is needed to write to EALLOW protected register
PieVectTable.ADCINT = &adc_isr;
PieVectTable.EPWM1_INT = &epwm1_isr;
PieVectTable.EPWM2_INT = &epwm2_isr;
//PieVectTable.EPWM3_INT = &epwm3_isr;
EDIS; // This is needed to disable write to EALLOW protected registers
// Step 4. Initialize all the Device Peripherals:
// This function is found in DSP280x_InitPeripherals.c
// InitPeripherals(); // Not required for this example
InitAdc(); // For this example, init the ADC
// For this example, only initialize the ePWM
EALLOW;
SysCtrlRegs.PCLKCR0.bit.TBCLKSYNC = 0;
EDIS;
InitEPwm1Example();
InitEPwm2Example();
//InitEPwm3Example();
EALLOW;
SysCtrlRegs.PCLKCR0.bit.TBCLKSYNC = 1;
EDIS;
// Step 5. User specific code, enable interrupts:
IER |= M_INT1; // Enable CPU Interrupt 1
// Enable ADCINT in PIE
PieCtrlRegs.PIEIER1.bit.INTx6 = 1;
// Enable CPU INT3 which is connected to EPWM1-3 INT:
IER |= M_INT3;
// Enable EPWM INTn in the PIE: Group 3 interrupt 1-3
PieCtrlRegs.PIEIER3.bit.INTx
boost_mppt.rar_BOOST MPPT_BOOST MPPT_MPPT 单片机_光伏 boost_光伏 mppt
版权申诉
195 浏览量
2022-07-14
01:48:09
上传
评论 2
收藏 254KB RAR 举报

Kinonoyomeo
- 粉丝: 75
- 资源: 1万+
最新资源
- 基于esp8266和dht11温湿度传感器制作的远程温湿度监控程序,温度、湿度通过mqtt协议方式上传OneNet平台
- 人染色体长度表(数据来自bilibili:基因学苑)
- 基于ASP.NET简易博客网站的设计与实现(源代码+论文).rar
- 在PyCharm中配置Python环境步骤
- 在PyCharm中配置Python环境步骤
- Lightroom-Premium-v9.2.2_build_710902200-Mod.apk
- 拾放机构3D 拾放机构3D
- Spring整合Mybatis+Spring事务快速入门(纯注解)
- 1990-2024年1月上证 深证指数日线行情
- html/css练习作业摇晃的桃子
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


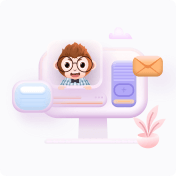