(java)excel-to-sql.zip_Excel导入sql_excel_excel sql_excel 导入 SQL
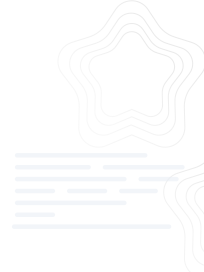

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
在IT行业中,数据处理是一项常见的任务,特别是在大数据和企业级应用中。本示例通过Java技术,演示了如何将Excel文件中的数据有效地导入到SQL数据库中,这对于数据管理、数据分析和自动化测试等领域非常实用。下面将详细介绍这个过程涉及的关键知识点。 1. **Java编程**:Java是一种广泛使用的面向对象的编程语言,它具有跨平台性,使得开发的程序可以在不同的操作系统上运行。在这个项目中,Java被用来编写处理Excel和数据库操作的代码。 2. **Apache POI库**:`ExcelReader.java`文件可能使用了Apache POI库来读取Excel文件。Apache POI是Java中处理Microsoft Office格式文件的开源库,它提供了读取和写入Excel文件的功能。通过POI,我们可以访问Excel工作簿、工作表和单元格,从而提取数据。 3. **Excel数据解析**:在`ExcelReader.java`中,可能包含了对Excel文件中数据的解析逻辑。这通常涉及到遍历工作表的行和列,提取出数据,并将其转换为适合数据库存储的格式。 4. **JDBC(Java Database Connectivity)**:为了将数据导入数据库,项目可能使用了JDBC API。JDBC是Java连接数据库的标准接口,可以支持多种数据库,如MySQL、Oracle、PostgreSQL等。开发者可以通过JDBC创建数据库连接,执行SQL语句以及处理结果集。 5. **SQL语句构造**:在DataInsert.java文件中,可能包含了根据Excel数据构造SQL插入语句的逻辑。数据从Excel中读取后,会拼接成一个个INSERT INTO语句,用于向数据库中添加新记录。 6. **批量处理**:为了提高效率,通常会采用批量插入的方式将大量数据导入数据库。Java的Statement或PreparedStatement接口提供了批处理的方法,可以一次性提交多条SQL语句。 7. **异常处理**:在实际编程中,必须考虑到可能出现的错误和异常,例如文件读取错误、数据库连接问题或者SQL执行失败等。因此,代码中应包含适当的try-catch块来捕获和处理这些异常。 8. **性能优化**:在处理大量数据时,优化代码以减少内存占用和提高处理速度是必要的。例如,使用流式处理(Streaming API)读取Excel,以及合理设置数据库的批处理大小等。 9. **数据库设计**:导入数据前,需要有与Excel数据结构匹配的数据库表结构。表的设计应该考虑字段类型、主键、外键等关系数据库概念,以确保数据的一致性和完整性。 10. **测试与调试**:在完成代码编写后,需要进行单元测试和集成测试,确保数据导入功能的正确性和性能。可以使用JUnit等测试框架进行测试,并使用日志工具(如Log4j)记录程序运行状态,便于调试和问题定位。 以上就是从标题、描述和标签中提炼出的关于Java将Excel数据导入SQL数据库的关键知识点。了解并掌握这些知识,将有助于在实际项目中实现类似的数据处理任务。
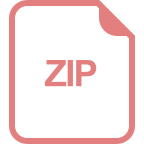
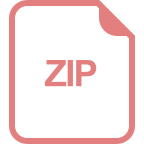
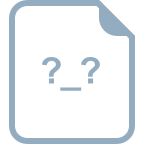
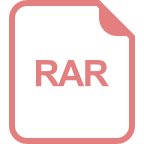
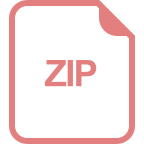
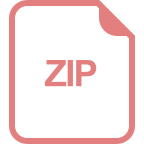
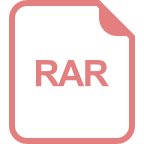
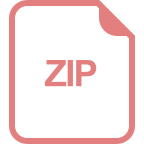
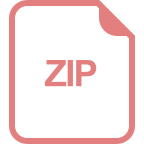
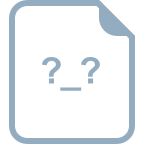
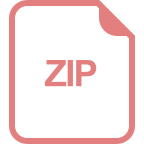
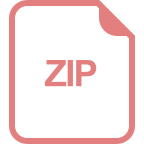
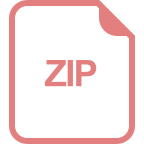
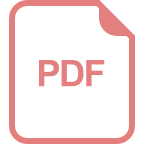
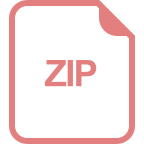
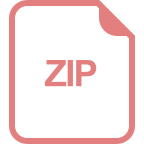

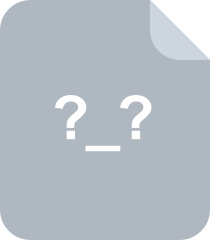
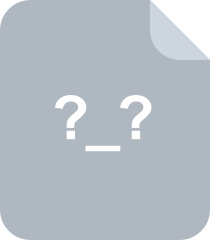
- 1
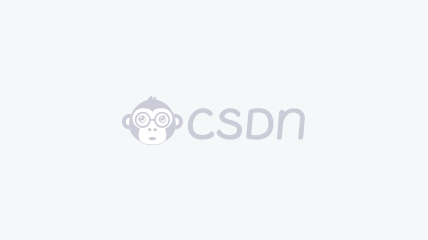

- 粉丝: 99
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

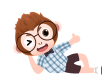
最新资源
- 基于java+springboot+vue+mysql的线上医院挂号系统设计与实现.docx
- 基于java+springboot+vue+mysql的箱包存储系统设计与实现.docx
- 基于java+springboot+vue+mysql的校园二手书交易平台设计与实现.docx
- 基于java+springboot+vue+mysql的校园健康驿站管理系统设计与实现.docx
- 02-【名企案例】-08-微软员工手册.doc
- 基于java+springboot+vue+mysql的校园竞赛管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的校园生活服务平台设计与实现.docx
- 02-【名企案例】-15-龙湖地产员工手册.doc
- 基于java+springboot+vue+mysql的校园外卖服务系统设计与实现.docx
- 基于java+springboot+vue+mysql的校园新闻管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的校园食堂订餐系统设计与实现.docx
- 06-【制造企业】-03-员工手册.doc
- 11-【管桩公司】-01-员工手册.doc
- 10-【装饰公司】-01-员工手册.doc
- 18-【餐饮公司】-02-员工手册.doc
- 基于java+springboot+vue+mysql的学生选课系统设计与实现.docx

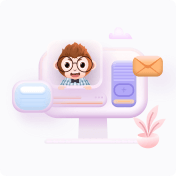
