package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import bean.academyBean;
import bean.classBean;
import bean.professionBean;
import bean.sexBean;
import bean.timeBean;
import bean.typeBean;
import db.db;
public class chartsDAO {
private Connection conn;
public chartsDAO(){
this.conn=db.getConnection();
}
public ArrayList<academyBean> academyChart()
{
PreparedStatement pstmt = null;
ResultSet rst = null;
String sql = "select u_academy,count(*) from user , borrow where borrow.U_ID = user.U_ID group by u_academy";
try{
pstmt = conn.prepareStatement(sql);
rst = pstmt.executeQuery();
ArrayList<academyBean> array = new ArrayList<academyBean>();
while(rst.next())
{
academyBean ab = new academyBean();
ab.setU_academy(rst.getString("u_academy"));
ab.setNum(rst.getInt("count(*)"));
array.add(ab);
}
pstmt.close();
rst.close();
return array;
}catch(SQLException e){
return new ArrayList<academyBean>();
}finally{
try{
conn.close();
}catch(SQLException e){
System.out.println("Error occured at closing connection");
}
}
}
public ArrayList<classBean> classChart()
{
PreparedStatement pstmt = null;
ResultSet rst = null;
String sql = "select u_class,count(*) from user , borrow where borrow.U_ID = user.U_ID group by u_class";
try{
pstmt = conn.prepareStatement(sql);
rst = pstmt.executeQuery();
ArrayList<classBean> array = new ArrayList<classBean>();
while(rst.next())
{
classBean cb = new classBean();
cb.setU_class(rst.getString("u_class"));
cb.setNum(rst.getInt("count(*)"));
array.add(cb);
}
pstmt.close();
rst.close();
return array;
}catch(SQLException e){
return new ArrayList<classBean>();
}finally{
try{
conn.close();
}catch(SQLException e){
System.out.println("Error occured at closing connection");
}
}
}
public ArrayList<professionBean> professionChart()
{
PreparedStatement pstmt = null;
ResultSet rst = null;
String sql = " select u_profession,count(*) from user , borrow where borrow.U_ID = user.U_ID group by u_profession";
try{
pstmt = conn.prepareStatement(sql);
rst = pstmt.executeQuery();
ArrayList<professionBean> array = new ArrayList<professionBean>();
while(rst.next())
{
professionBean cb = new professionBean();
cb.setU_profession(rst.getString("u_profession"));
cb.setNum(rst.getInt("count(*)"));
array.add(cb);
}
pstmt.close();
rst.close();
return array;
}catch(SQLException e){
return new ArrayList<professionBean>();
}finally{
try{
conn.close();
}catch(SQLException e){
System.out.println("Error occured at closing connection");
}
}
}
public ArrayList<sexBean> sexChart()
{
PreparedStatement pstmt = null;
ResultSet rst = null;
String sql = "select u_sex,count(*) from user , borrow where borrow.U_ID = user.U_ID group by u_sex";
try{
pstmt = conn.prepareStatement(sql);
rst = pstmt.executeQuery();
ArrayList<sexBean> array = new ArrayList<sexBean>();
while(rst.next())
{
sexBean sb = new sexBean();
sb.setU_sex(rst.getString("u_sex"));
sb.setNum(rst.getInt("count(*)"));
array.add(sb);
}
pstmt.close();
rst.close();
return array;
}catch(SQLException e){
return new ArrayList<sexBean>();
}finally{
try{
conn.close();
}catch(SQLException e){
System.out.println("Error occured at closing connection");
}
}
}
public ArrayList<typeBean> typeChart()
{
PreparedStatement pstmt = null;
ResultSet rst = null;
String sql = "select u_type,count(*) from user , borrow where borrow.U_ID = user.U_ID group by u_type";
try{
pstmt = conn.prepareStatement(sql);
rst = pstmt.executeQuery();
ArrayList<typeBean> array = new ArrayList<typeBean>();
while(rst.next())
{
typeBean tb = new typeBean();
tb.setU_type(rst.getString("u_type"));
tb.setNum(rst.getInt("count(*)"));
array.add(tb);
}
pstmt.close();
rst.close();
return array;
}catch(SQLException e){
return new ArrayList<typeBean>();
}finally{
try{
conn.close();
}catch(SQLException e){
System.out.println("Error occured at closing connection");
}
}
}
public ArrayList<timeBean> timeChart()
{
PreparedStatement pstmt = null;
ResultSet rst = null;
String sql = "select MONTH(borrow_time) as month,count(*) from borrow group by MONTH(borrow_time)";
try{
pstmt = conn.prepareStatement(sql);
rst = pstmt.executeQuery();
ArrayList<timeBean> array = new ArrayList<timeBean>();
while(rst.next())
{
timeBean timebean = new timeBean();
timebean.setU_time(rst.getInt("month"));
timebean.setNum(rst.getInt("count(*)"));
array.add(timebean);
}
pstmt.close();
rst.close();
return array;
}catch(SQLException e){
return new ArrayList<timeBean>();
}finally{
try{
conn.close();
}catch(SQLException e){
System.out.println("Error occured at closing connection");
}
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
qlp.zip_echarts_handsome6ad_strus 结合echarts
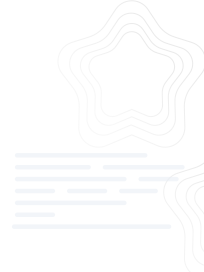
共58个文件
jar:15个
class:10个
java:10个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 70 浏览量
2022-09-20
11:21:15
上传
评论
收藏 5.98MB ZIP 举报
温馨提示
用echarts和js技术结合struts框架生成饼图和柱状图
资源推荐
资源详情
资源评论
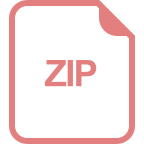
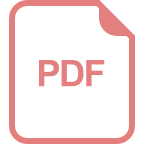
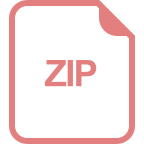
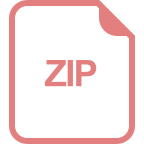
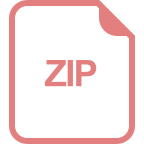
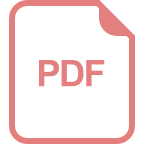
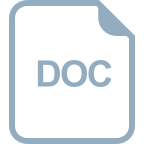
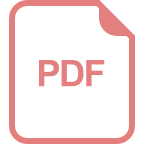
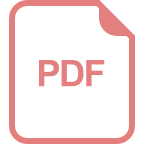
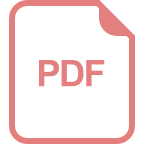
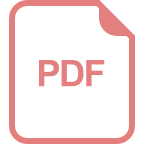
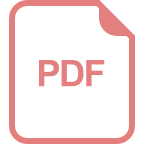
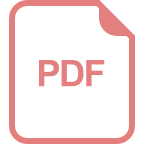
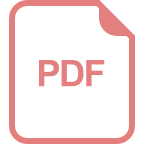
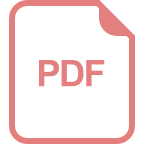
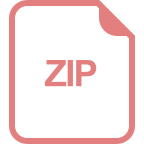
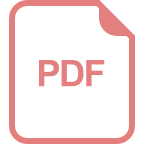
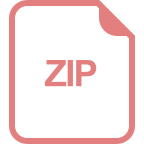
收起资源包目录



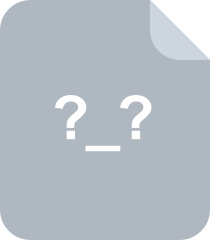
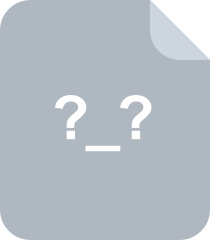
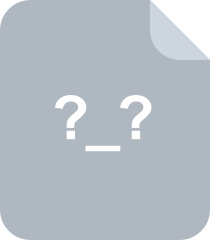
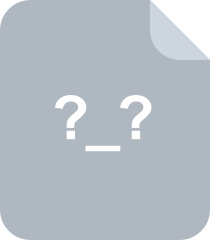
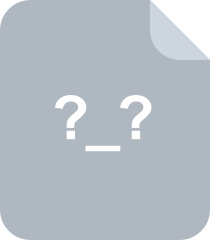
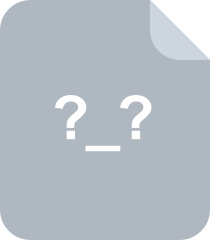
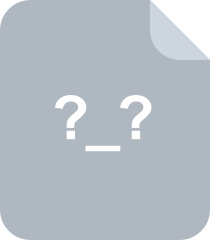


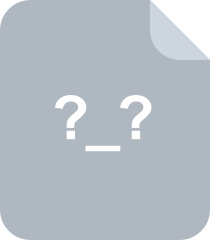
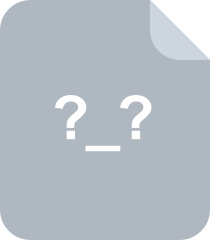

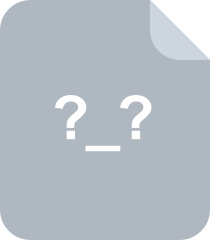

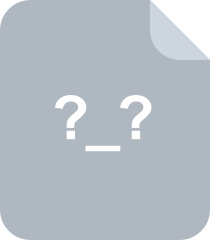

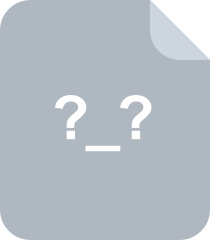
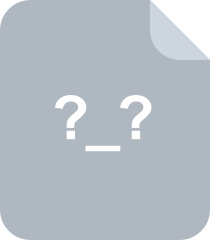
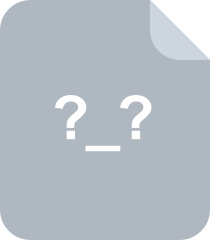
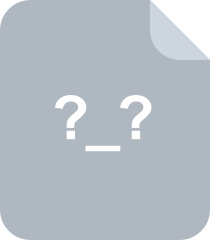
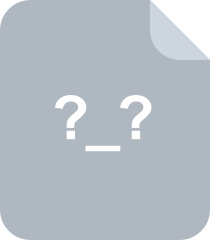
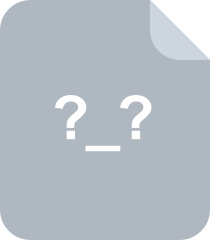
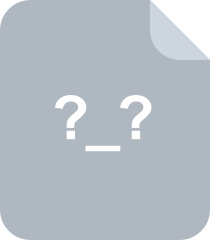
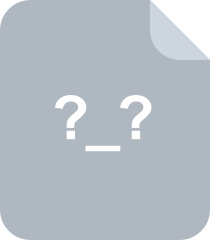

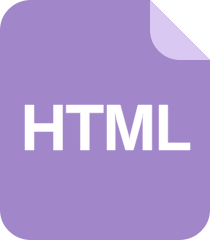
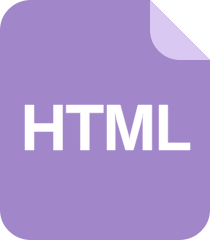

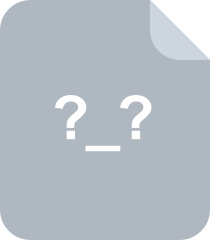

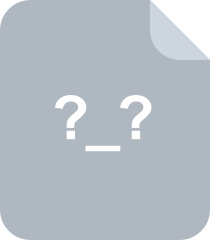


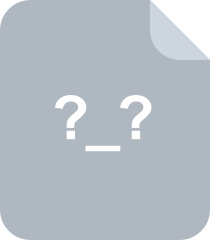
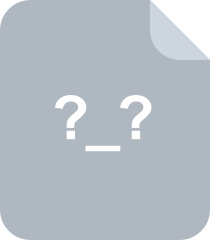

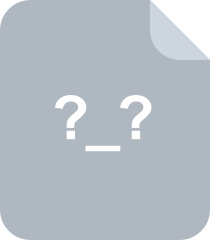

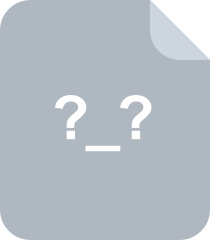

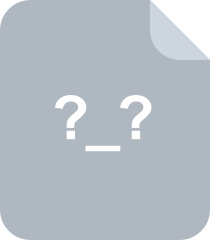
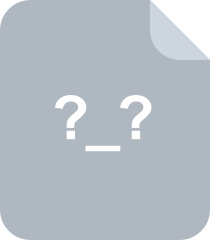
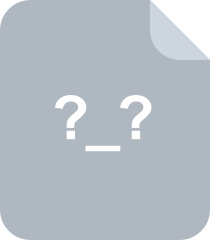
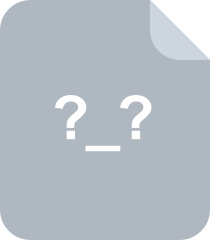
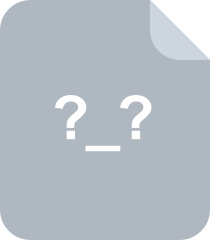
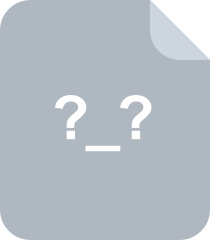
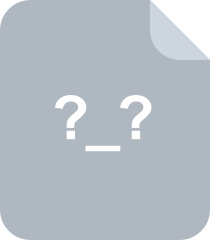

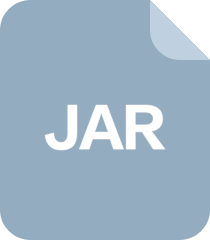
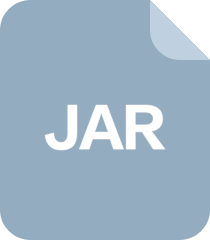
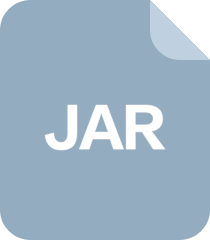
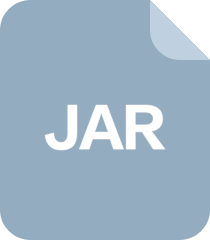
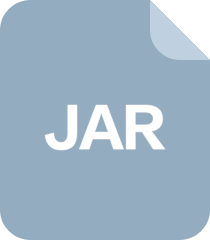
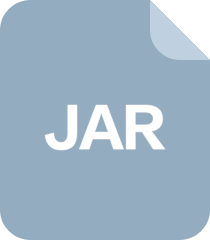
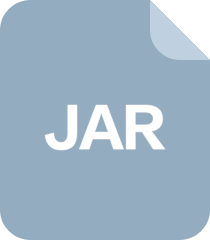
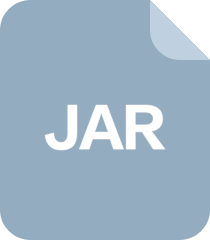
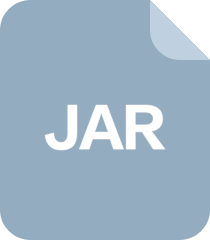
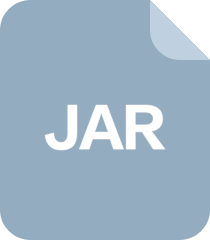
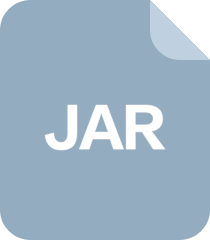
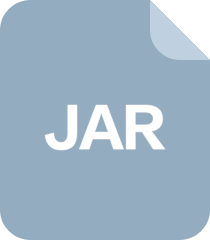
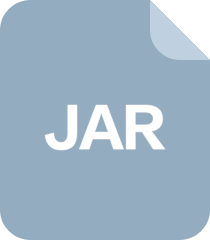
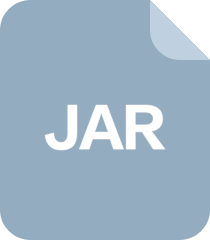
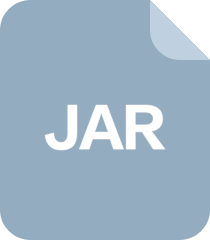

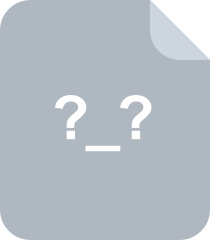
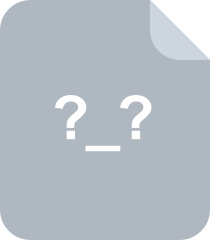
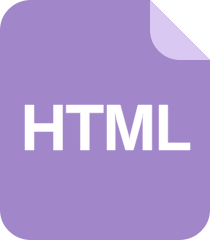
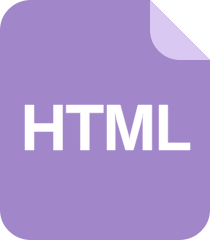
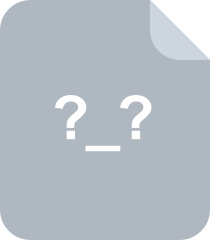
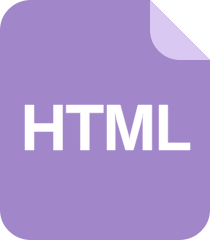
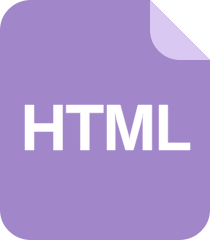
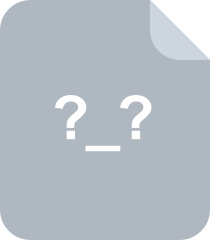
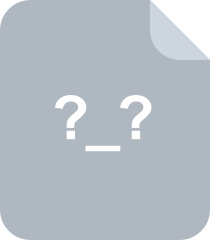

共 58 条
- 1
资源评论
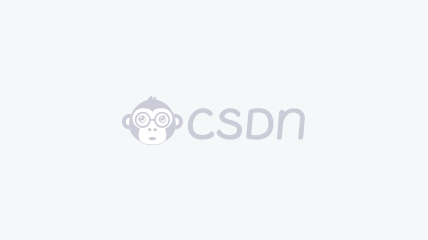

JaniceLu
- 粉丝: 78
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

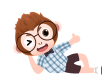
安全验证
文档复制为VIP权益,开通VIP直接复制
