TS.rar_TS Fuzzy_Takagi Sugeno_Takagi-Sugeno_fuzzy sugeno_sugeno
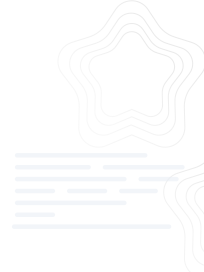

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
标题中的"TS.rar"可能指的是Takagi-Sugeno(TS)模糊系统相关的压缩文件,而"TS Fuzzy"进一步确认了这是关于模糊逻辑领域的学习资料。Takagi-Sugeno(TS)模糊系统是一种广泛应用的模糊推理模型,由Takagi和Sugeno在1985年提出,它在控制理论、人工智能和数据分析等领域有广泛的应用。 描述中的"training Takagi-Sugeno fuzzy"暗示这可能是关于训练或学习如何构建和应用Takagi-Sugeno模糊系统的教程或资料。学习TS模糊系统通常涉及理解模糊集合理论、模糊规则构造、模糊推理过程以及如何将这些概念应用于实际问题解决。 标签中的关键词进一步强调了主题:"ts_fuzzy"指的是TS模糊系统,"takagi_sugeno"是两位创始人,"takagi-sugeno"是其名字的连接形式,"fuzzy_sugeno"和"sugeno_fuzzy"则重申了Sugeno模糊系统或与Sugeno相关的模糊概念。 压缩包中的唯一文件"TS.txt"可能是文本格式的教程或论文,详细介绍了Takagi-Sugeno模糊系统的基本概念、数学模型、设计方法、控制算法以及可能的应用案例。通常,这样的文档会涵盖以下内容: 1. **模糊集合理论**:模糊集合是经典集合论的扩展,允许元素具有不确定性的隶属度。TS模糊系统基于模糊集合来描述输入和输出变量的不确定性。 2. **模糊规则**:TS模糊系统由一系列模糊规则构成,每个规则都以“如果...那么...”的形式表述,其中“如果”部分是模糊条件,而“那么”部分是模糊结论。 3. **模糊推理**:通过模糊化、模糊推理和去模糊化这三个步骤,TS模糊系统能够处理输入的模糊数据并产生清晰的输出。 4. **Takagi-Sugeno模型**:这个模型的特点在于它的规则输出是线性函数的加权和,这使得它在处理非线性问题时表现出优良的性能。 5. **系统设计**:如何构建模糊集、定义规则库、选择合适的模糊化和去模糊化方法,以及参数调整等步骤。 6. **应用实例**:可能包括控制系统设计、故障诊断、预测模型、图像处理等领域的实际应用案例。 7. **软件工具**:介绍使用模糊逻辑工具箱(如MATLAB的Fuzzy Logic Toolbox)进行TS模糊系统建模和仿真。 8. **性能评估**:如何衡量TS模糊系统的性能,比如稳定性、鲁棒性、精确度等。 学习TS模糊系统需要对模糊逻辑有一定的了解,并通过实践案例加深理解。"TS.txt"文件很可能是帮助读者深入理解和应用这一理论的重要资源。
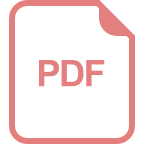
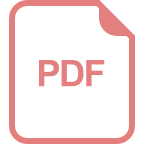
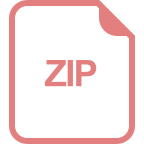
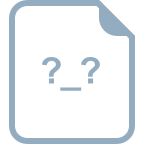
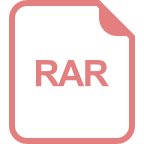
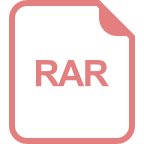
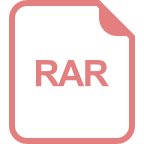
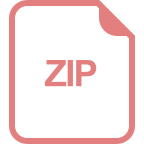
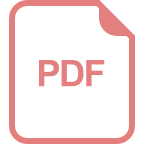
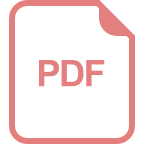
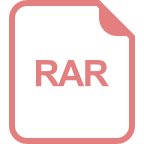
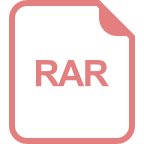
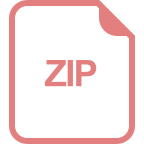
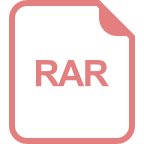
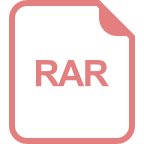
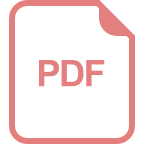
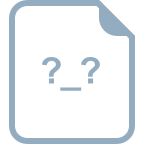
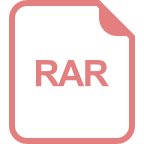
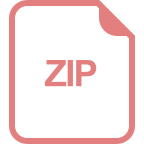
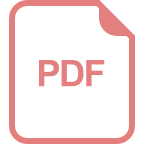
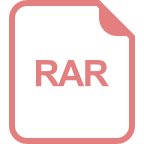
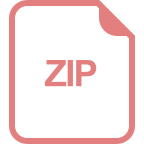
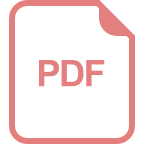

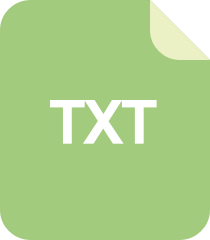
- 1
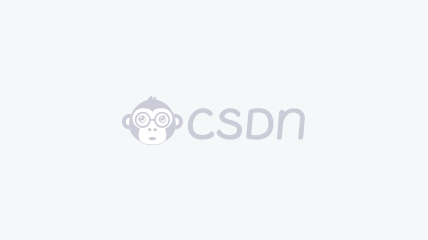

- 粉丝: 98
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

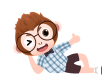
最新资源
- ccceeeeee,ukytkyk/liyihm
- 考虑新能源消纳的火电机组深度调峰策略 摘要:本代码主要做的是考虑新能源消纳的火电机组深度调峰策略,以常规调峰、不投油深度调峰、投油深度调峰三个阶段,建立了火电机组深度调峰成本模型,并以风电全额消纳为前
- PROGPPCNEXUS读写烧录刷写软件 飞思卡尔MPC55xx 56xx 57xx 58xx 没有次数限制
- 含光伏的储能选址定容模型 14节点 程序采用改进粒子群算法,对分析14节点配网系统中的储能选址定容方案,并得到储能的出力情况,有相关参考资料 这段程序是一个粒子群算法(Particle Swarm O
- P6ProfessionalSetup R24.12 安装包
- SQLServer2012数据库配置及网络连接设置WORD文档doc格式最新版本
- 中大型三相异步电机电磁设计软件
- DSP28335 PMSM电机控制程序
- 四足机器人技术发展及其应用场景概述
- linux常用命令大全.txt

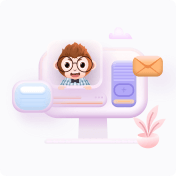
