BMI.zip_健康_健康表
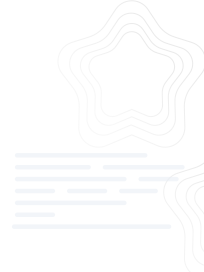

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
标题中的“BMI.zip_健康_健康表”表明这是一个与健康相关的压缩文件,主要涉及的是BMI(Body Mass Index,身体质量指数)的计算和评估。BMI是衡量人体体重与身高比例的一个标准,通常用于评估一个人是否处于健康的体重范围。在JAVA编程语言中实现这样一个健康表,意味着它可能包含了一个简单的应用程序或代码,用户可以通过输入自己的身高和体重来计算BMI,并得到相应的健康状况分析。 在描述中提到的“健康查询,利用JAVA做的简单的健康对比表”,进一步明确了这个程序的功能。这可能是一个用户友好的界面,用户可以输入信息并获得即时反馈。健康对比表可能包括不同BMI值对应的健康状态,例如过轻、正常、超重或肥胖,帮助用户了解自己的体重状况并引导他们采取适当的健康措施。 基于“健康”和“健康表”的标签,我们可以推测这个应用或代码库可能会包含以下知识点: 1. **BMI计算公式**:BMI = 体重(公斤)/ 身高²(米),这是评估人体健康的基础。 2. **JAVA编程基础**:包括数据类型、变量、条件语句、循环、函数等,这些都是编写计算BMI程序的基本元素。 3. **用户输入处理**:JAVA中的Scanner类用于获取用户输入的身高和体重数据。 4. **错误处理**:确保用户输入的是有效的数值,防止程序出错。 5. **控制台输出**:通过System.out.println()展示计算结果和健康建议。 6. **条件判断**:根据BMI值分类,判断健康状况,如使用if-else语句或switch-case结构。 7. **GUI编程**:如果程序具有图形用户界面,可能涉及Swing或JavaFX库,用于创建窗口、按钮和文本框等组件。 8. **事件监听**:当用户点击按钮时触发BMI计算,这涉及到事件处理和监听器的概念。 9. **数据存储**:可能包含将计算结果存储到文件或数据库中的功能,涉及文件操作或数据库连接技术。 10. **代码注释和文档**:良好的编程习惯,包括代码的清晰注释和可能的README文件,方便其他开发者理解和使用。 这个名为“BMI.zip”的压缩文件可能包含一个JAVA编写的健康查询应用,用户可以借此计算BMI并得到关于健康状况的反馈。其背后涉及的编程知识涵盖基础编程概念到更高级的GUI设计和数据处理。
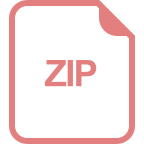
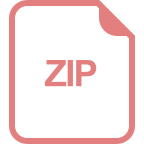
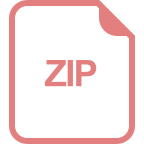
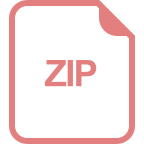
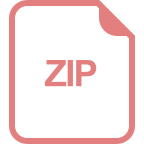
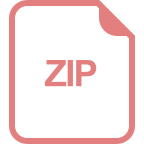
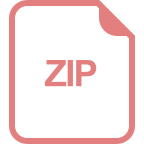
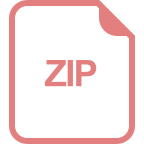
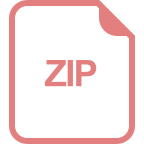
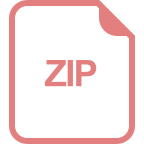
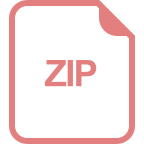
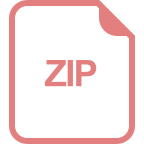
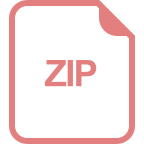
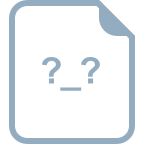
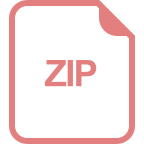
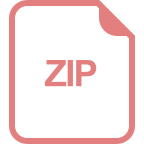
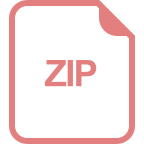
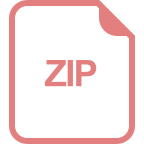




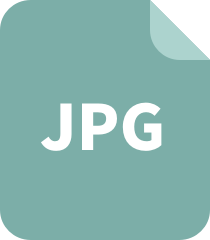
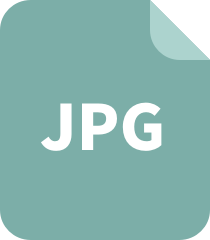
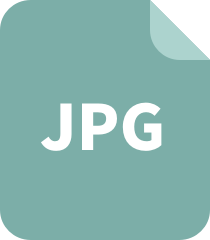
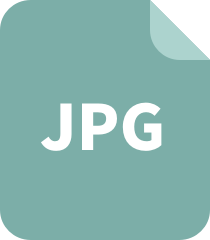
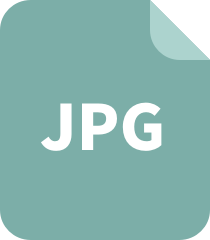
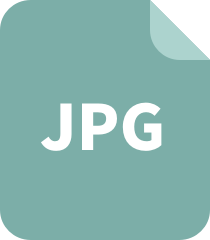
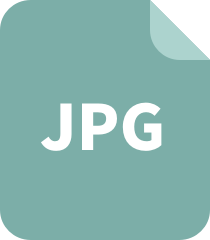
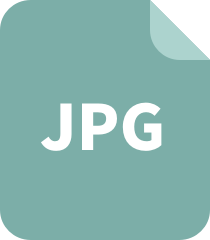

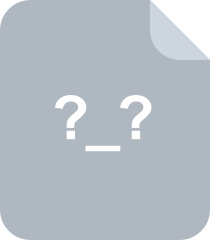

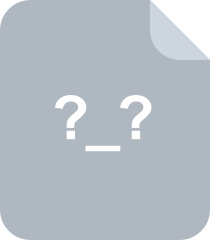

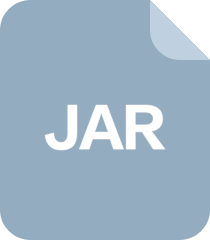

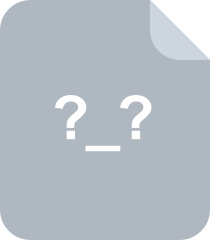
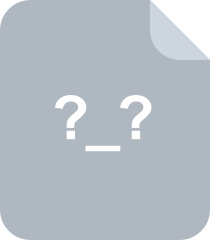

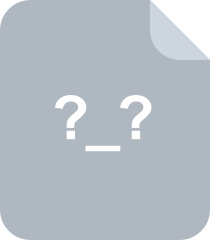
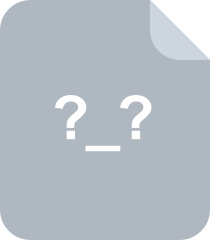

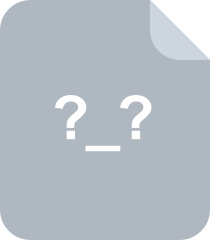
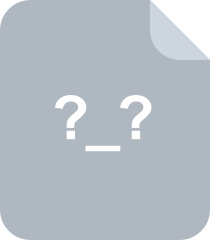
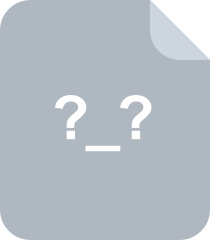
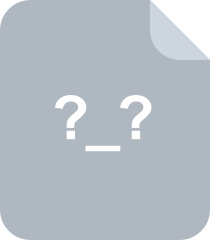
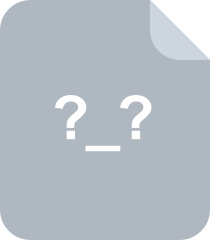
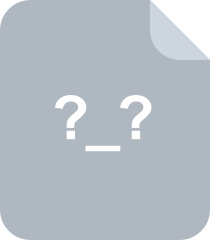
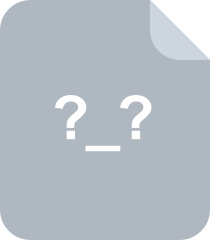




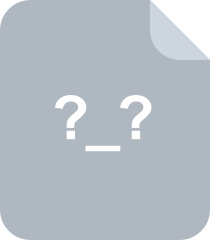

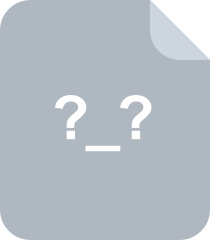
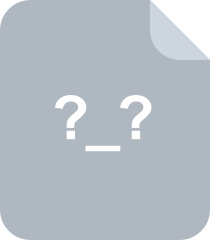
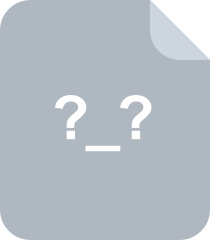
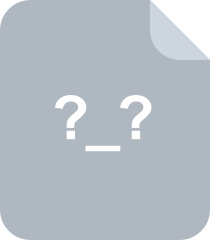





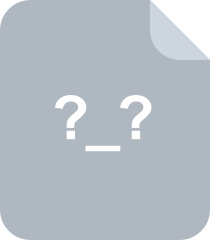

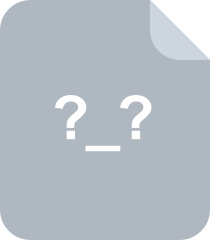
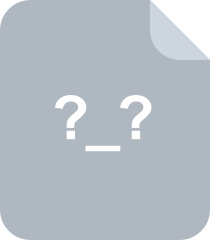
- 1
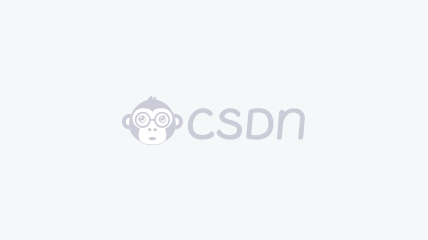

- 粉丝: 94
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

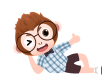
最新资源
- Python实现基于一维卷积神经网络(1D-CNN)的多元时间序列分类源码+文档说明
- java基于springBoot课程评价系统源码数据库 MySQL源码类型 WebForm
- 5G工业无线路由器说明书
- SunshineLife个人博客是基于django+mysql+layui的小型个人博客网站
- 5G终端拔号脚本(AT), 适用于MT5701
- 基于Python + Django的生鲜超市系统 调用alipay沙盒系统支付
- 登山比赛乘车安排表.xlsx
- 面试题记录11111111111111
- java基于springMVC的云音乐网站源码数据库 MySQL源码类型 WebForm
- MiniCADSee-X64(CAD看图软件)

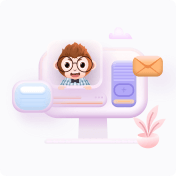
