/*
* hydra (c) 2001-2014 by van Hauser / THC <vh@thc.org>
* http://www.thc.org
*
* Parallized network login hacker.
* Don't use in military or secret service organizations, or for illegal purposes.
*
* License: GNU AFFERO GENERAL PUBLIC LICENSE v3.0, see LICENSE file
*/
#include "hydra.h"
#include "bfg.h"
extern void service_asterisk(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_telnet(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_ftp(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_ftps(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_pop3(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_vmauthd(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_imap(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_ldap2(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_ldap3(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_ldap3_cram_md5(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_ldap3_digest_md5(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_cisco(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_cisco_enable(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_vnc(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_socks5(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_rexec(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_rlogin(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_rsh(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_nntp(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_http_head(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_http_get(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_http_get_form(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_http_post_form(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_icq(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_pcnfs(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_mssql(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_cvs(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_snmp(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_smtp(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_smtp_enum(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_teamspeak(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_pcanywhere(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_http_proxy(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_xmpp(char *target, char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_irc(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_http_proxy_urlenum(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_s7_300(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
// ADD NEW SERVICES HERE
#ifdef HAVE_MATH_H
extern void service_mysql(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_mysql_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBPOSTGRES
extern void service_postgres(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_postgres_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBOPENSSL
extern void service_smb(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_smb_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_oracle_listener(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_oracle_listener_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_oracle_sid(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_oracle_sid_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_sip(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_sip_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_rdp(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_rdp_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBSAPR3
extern void service_sapr3(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_sapr3_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBFIREBIRD
extern void service_firebird(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_firebird_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBAFP
extern void service_afp(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_afp_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBNCP
extern void service_ncp(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_ncp_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBSSH
extern void service_ssh(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_ssh_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern void service_sshkey(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_sshkey_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBSVN
extern void service_svn(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_svn_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
#ifdef LIBORACLE
extern void service_oracle(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_oracle_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
#endif
extern int service_cisco_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_cisco_enable_init(char *ip, int sp, unsigned char options, char *miscptr, FILE * fp, int port);
extern int service_cvs_init(char *ip, int sp, unsigned char options
没有合适的资源?快使用搜索试试~ 我知道了~
hydra-8.1.tar.gz_Network_bruteforce_hydra-8.1_hydra-8.1.tar.gz_p
1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
hydra - a very fast network logon cracker which support many different services
资源详情
资源评论
资源推荐
收起资源包目录

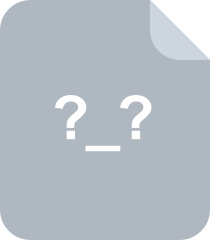
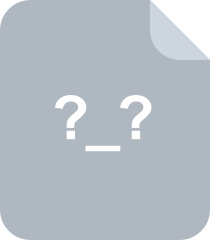
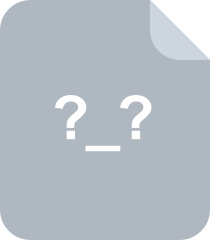
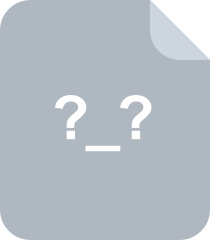
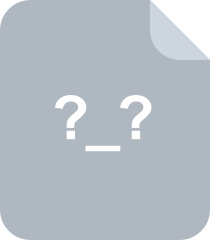
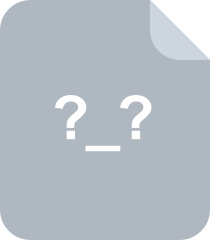
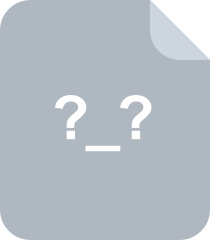
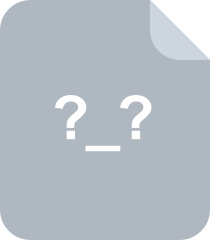
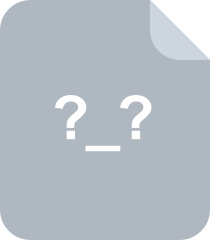
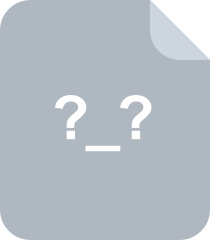
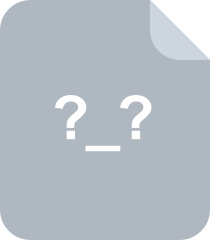
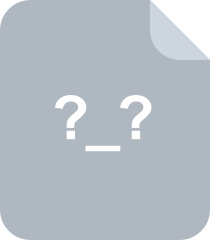
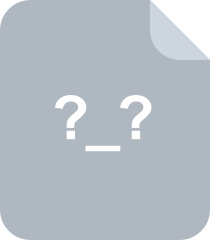
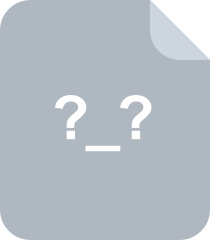
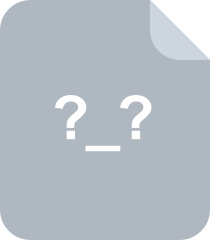
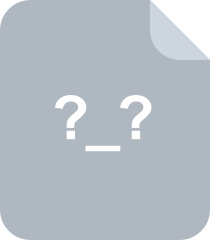
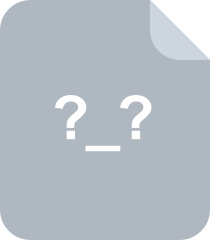
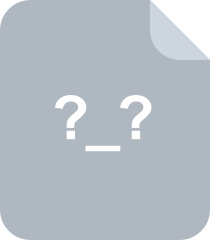
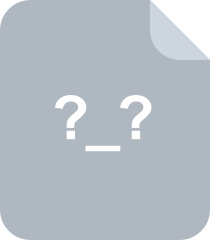
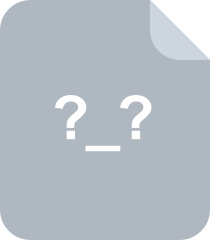
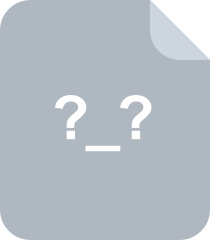
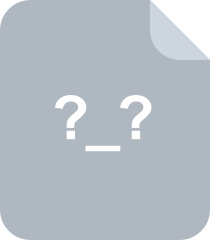
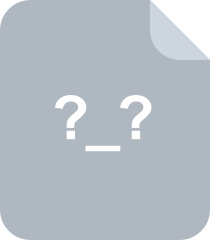
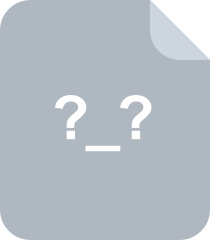
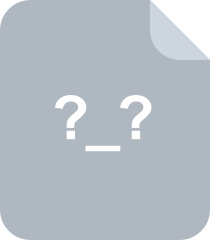
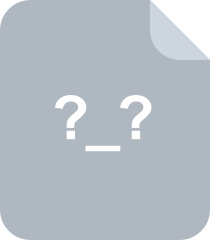
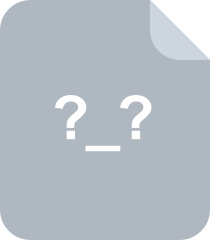
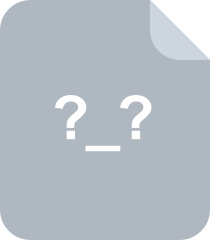
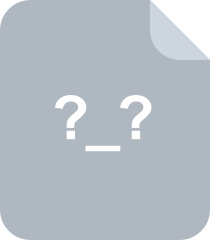
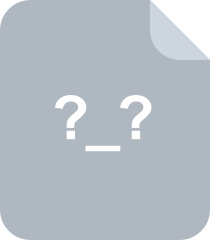
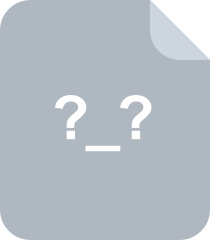
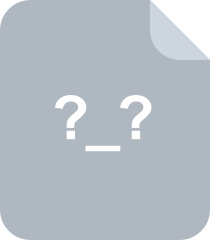
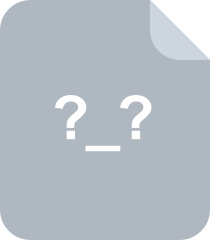
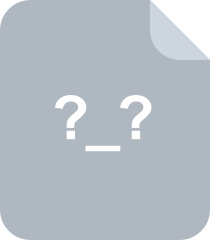
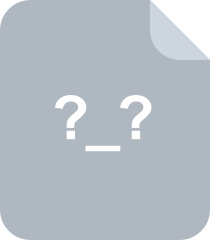
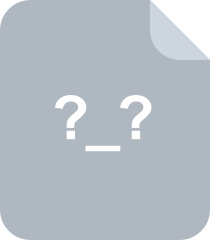
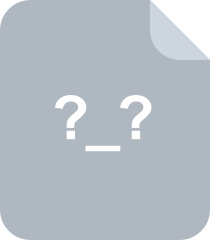
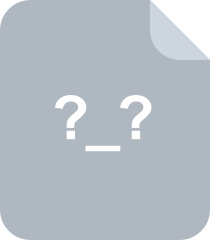
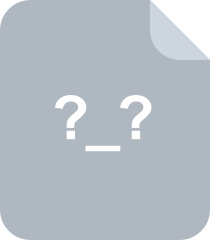
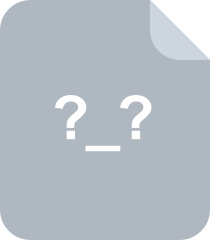
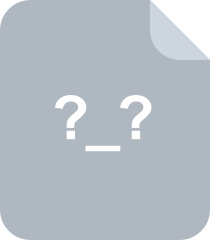
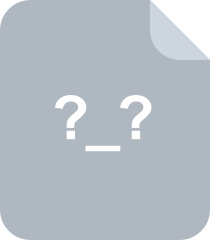
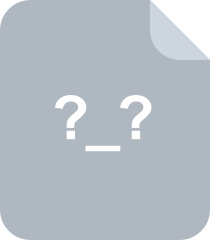
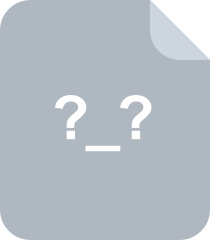
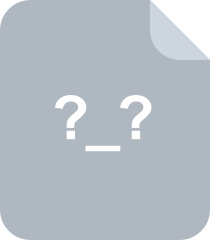
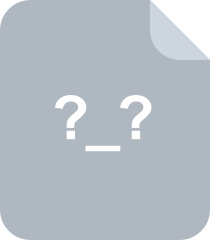
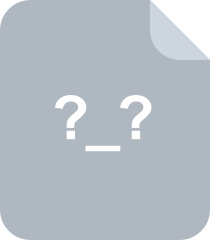
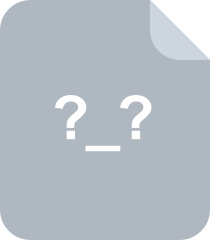
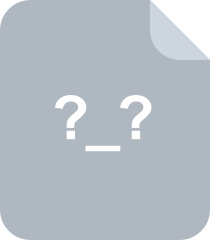
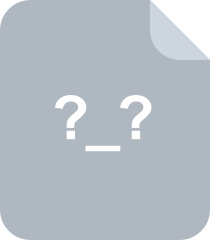
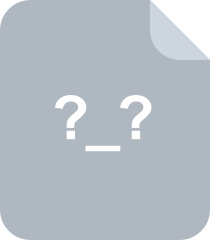
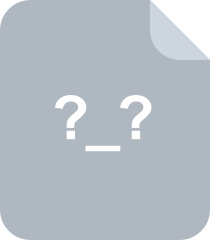
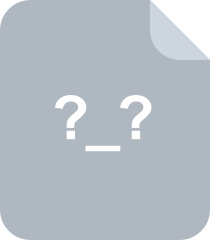
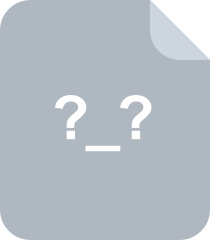
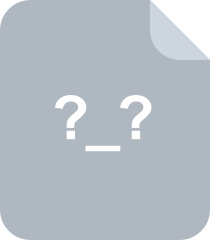
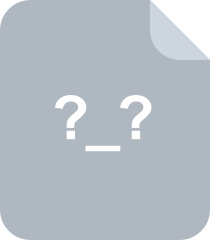
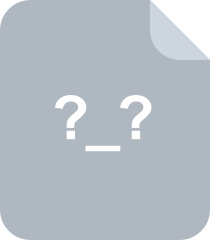
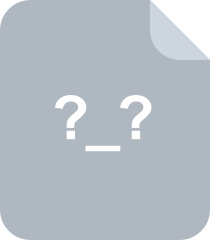
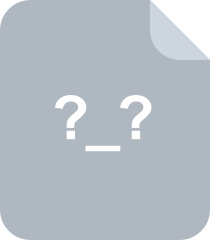
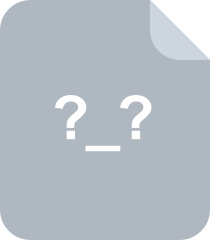
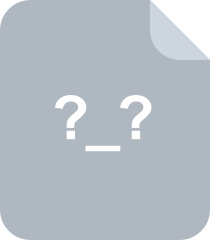
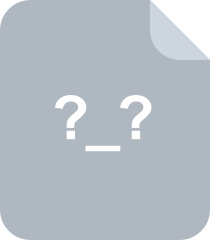
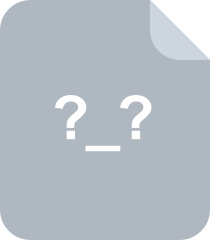
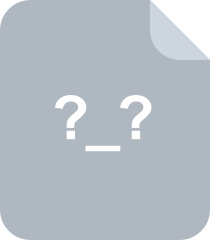
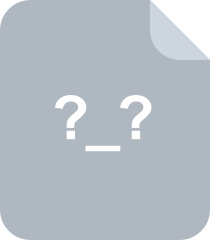
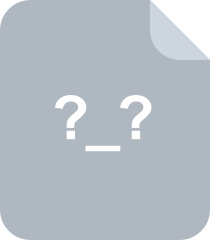
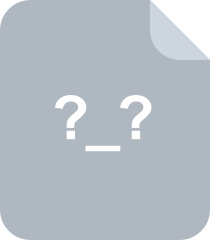
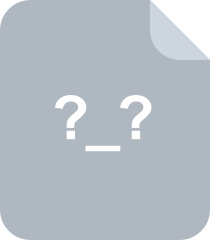
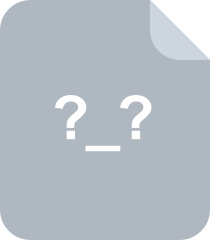
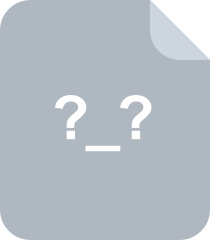
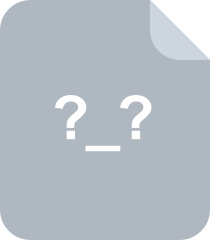
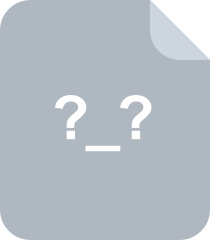
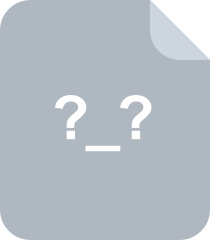
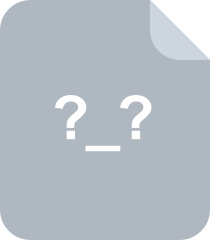
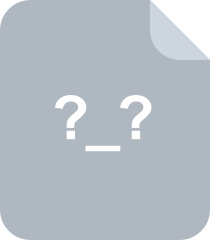
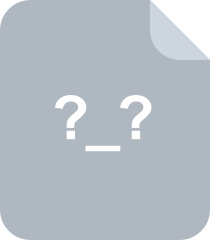
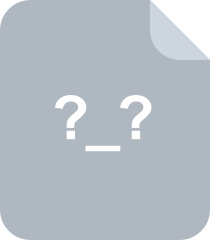
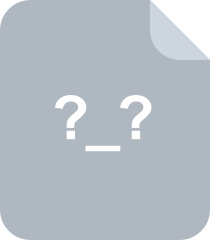
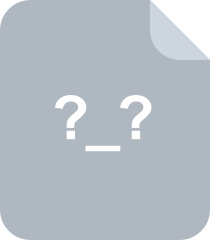
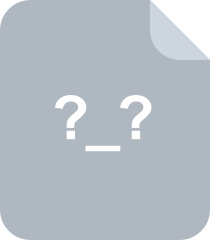
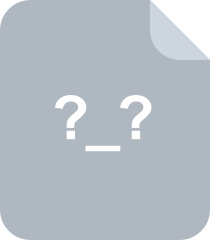
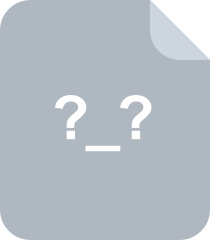
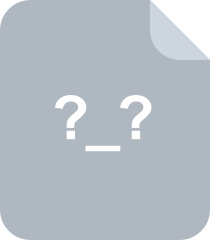
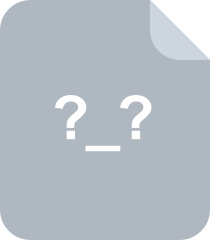
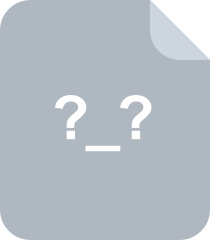
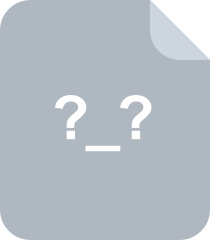
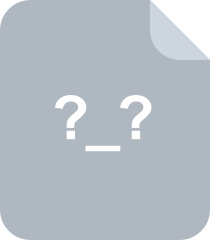
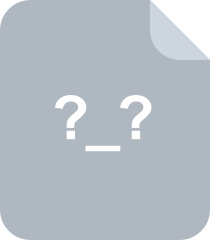
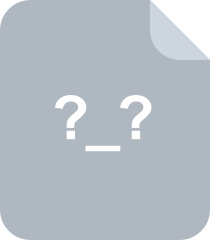
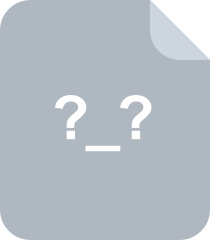
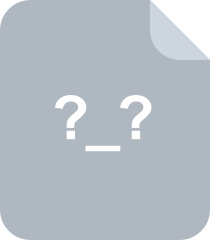
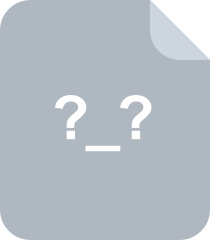
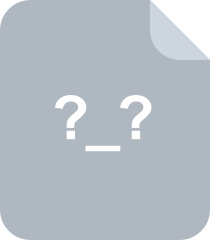
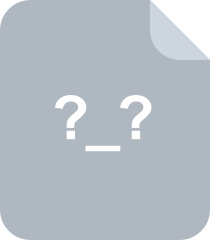
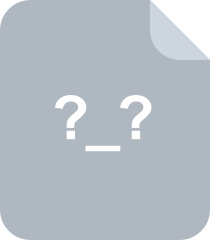
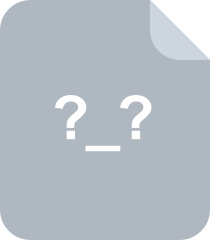
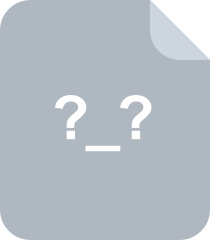
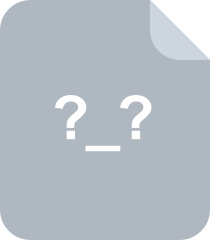
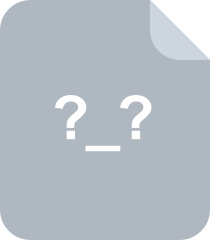
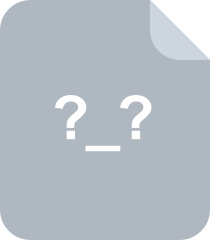
共 123 条
- 1
- 2
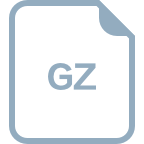
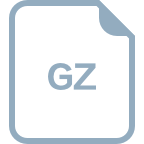
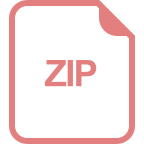
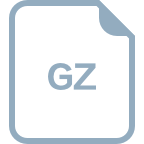
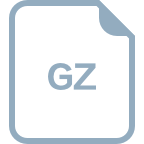
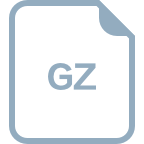
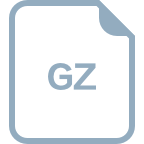
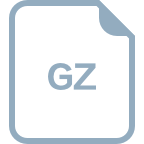
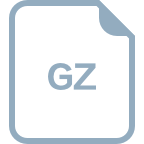
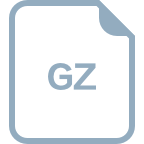
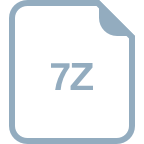
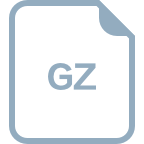
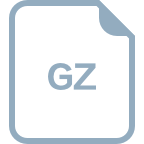

周楷雯
- 粉丝: 93
- 资源: 1万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

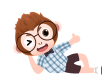
安全验证
文档复制为VIP权益,开通VIP直接复制

评论2