package dot.junit.opcodes.div_float;
import dot.junit.DxTestCase;
import dot.junit.DxUtil;
import dot.junit.opcodes.div_float.d.T_div_float_1;
import dot.junit.opcodes.div_float.d.T_div_float_5;
public class Test_div_float extends DxTestCase {
/**
* @title Arguments = 2.7f, 3.14f
*/
public void testN1() {
T_div_float_1 t = new T_div_float_1();
assertEquals(0.8598726f, t.run(2.7f, 3.14f));
}
/**
* @title Dividend = 0
*/
public void testN2() {
T_div_float_1 t = new T_div_float_1();
assertEquals(0f, t.run(0, 3.14f));
}
/**
* @title Dividend is negative
*/
public void testN3() {
T_div_float_1 t = new T_div_float_1();
assertEquals(-1.162963f, t.run(-3.14f, 2.7f));
}
/**
* @title Types of arguments - int, float. Dalvik doens't distinguish 32-bits types internally,
* so this division of float and int makes no sense but shall not crash the VM.
*/
public void testN4() {
T_div_float_5 t = new T_div_float_5();
try {
t.run(1, 3.14f);
} catch (Throwable e) {
}
}
/**
* @title Arguments = Float.MAX_VALUE, Float.NaN
*/
public void testB1() {
T_div_float_1 t = new T_div_float_1();
assertEquals(Float.NaN, t.run(Float.MAX_VALUE, Float.NaN));
}
/**
* @title Arguments = Float.POSITIVE_INFINITY,
* Float.NEGATIVE_INFINITY
*/
public void testB2() {
T_div_float_1 t = new T_div_float_1();
assertEquals(Float.NaN, t.run(Float.POSITIVE_INFINITY,
Float.NEGATIVE_INFINITY));
}
/**
* @title Arguments = Float.POSITIVE_INFINITY, -2.7f
*/
public void testB3() {
T_div_float_1 t = new T_div_float_1();
assertEquals(Float.NEGATIVE_INFINITY, t.run(Float.POSITIVE_INFINITY,
-2.7f));
}
/**
* @title Arguments = -2.7f, Float.NEGATIVE_INFINITY
*/
public void testB4() {
T_div_float_1 t = new T_div_float_1();
assertEquals(0f, t.run(-2.7f, Float.NEGATIVE_INFINITY));
}
/**
* @title Arguments = 0, 0
*/
public void testB5() {
T_div_float_1 t = new T_div_float_1();
assertEquals(Float.NaN, t.run(0, 0));
}
/**
* @title Arguments = 0, -2.7
*/
public void testB6() {
T_div_float_1 t = new T_div_float_1();
assertEquals(-0f, t.run(0, -2.7f));
}
/**
* @title Arguments = -2.7, 0
*/
public void testB7() {
T_div_float_1 t = new T_div_float_1();
assertEquals(Float.NEGATIVE_INFINITY, t.run(-2.7f, 0));
}
/**
* @title Arguments = 1, Float.MAX_VALUE
*/
public void testB8() {
T_div_float_1 t = new T_div_float_1();
assertEquals(Float.POSITIVE_INFINITY, t.run(1, Float.MIN_VALUE));
}
/**
* @title Arguments = Float.MAX_VALUE, -1E-9f
*/
public void testB9() {
T_div_float_1 t = new T_div_float_1();
assertEquals(Float.NEGATIVE_INFINITY, t.run(Float.MAX_VALUE, -1E-9f));
}
/**
* @constraint B1
* @title types of arguments - float / double
*/
public void testVFE1() {
try {
Class.forName("dot.junit.opcodes.div_float.d.T_div_float_2");
fail("expected a verification exception");
} catch (Throwable t) {
DxUtil.checkVerifyException(t);
}
}
/**
* @constraint B1
* @title types of arguments - long / float
*/
public void testVFE2() {
try {
Class.forName("dot.junit.opcodes.div_float.d.T_div_float_3");
fail("expected a verification exception");
} catch (Throwable t) {
DxUtil.checkVerifyException(t);
}
}
/**
* @constraint B1
* @title types of arguments - reference / float
*/
public void testVFE3() {
try {
Class.forName("dot.junit.opcodes.div_float.d.T_div_float_4");
fail("expected a verification exception");
} catch (Throwable t) {
DxUtil.checkVerifyException(t);
}
}
/**
* @constraint A23
* @title number of registers
*/
public void testVFE4() {
try {
Class.forName("dot.junit.opcodes.div_float.d.T_div_float_6");
fail("expected a verification exception");
} catch (Throwable t) {
DxUtil.checkVerifyException(t);
}
}
}

周楷雯
- 粉丝: 97
- 资源: 1万+
最新资源
- hadoop ipc-hadoop
- bootshiro-springboot
- 微信文章爬虫 Reptile-爬虫
- AwesomeUnityTutorial-unity
- STM32多功能小车-stm32
- blog-vscode安装
- ultralytics-yolov11
- Image processing based on matlab-matlab下载
- 即用即查XML数据标记语言参考手册pdf版最新版本
- XML轻松学习教程chm版最新版本
- 《XMLHTTP对象参考手册》CHM最新版本
- 单机版锁螺丝机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 注册程序示例示例示例示例示例
- 网络实践2222222
- kotlin coroutine blogs
- Windchill前端测试工具class文件
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


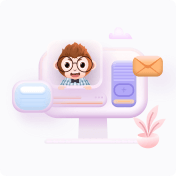