/*
* SNMP Package
*
* Copyright (C) 2004, Jonathan Sevy <jsevy@mcs.drexel.edu>
*
* This is free software. Redistribution and use in source and binary forms, with
* or without modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* 3. The name of the author may not be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED
* WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF
* MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO
* EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT
* OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT,
* STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY
* OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
*/
package snmp;
import java.io.*;
import java.net.*;
/**
* The class SNMPv1CommunicationInterface defines methods for communicating with SNMP entities.
* The approach is that from version 1 of SNMP, using no encryption of data. Communication occurs
* via UDP, using port 161, the standard SNMP port, unless an alternate (non-standard) port is
* supplied in the constructor.
*/
public class SNMPv1CommunicationInterface
{
public static final int SNMP_PORT = 161;
// port to which requests will be sent
private int remotePort = SNMP_PORT;
// largest size for datagram packet payload; based on
// RFC 1157, need to handle messages of at least 484 bytes
private int receiveBufferSize = 512;
private int version;
private InetAddress hostAddress;
private String community;
DatagramSocket dSocket;
public int requestID = 1;
/**
* Construct a new communication object to communicate with the specified host using the
* given community name. The version setting should be either 0 (version 1) or 1 (version 2,
* a la RFC 1157).
*/
public SNMPv1CommunicationInterface(int version, InetAddress hostAddress, String community)
throws SocketException
{
this(version, hostAddress, community, SNMP_PORT);
}
/**
* Construct a new communication object to communicate with the specified host using the
* given community name, and sending requests to the specified port. The version setting
* should be either 0 (version 1) or 1 (version 2, a la RFC 1157).
*/
public SNMPv1CommunicationInterface(int version, InetAddress hostAddress, String community, int remotePort)
throws SocketException
{
this.remotePort = remotePort;
this.version = version;
this.hostAddress = hostAddress;
this.community = community;
dSocket = new DatagramSocket();
dSocket.setSoTimeout(15000); //15 seconds
}
/**
* Permits setting timeout value for underlying datagram socket (in milliseconds).
*/
public void setSocketTimeout(int socketTimeout)
throws SocketException
{
dSocket.setSoTimeout(socketTimeout);
}
/**
* Close the "connection" with the device.
*/
public void closeConnection()
throws SocketException
{
dSocket.close();
}
/**
* Retrieve all MIB variable values subsequent to the starting object identifier
* given in startID (in dotted-integer notation). Return as SNMPVarBindList object.
* Uses SNMPGetNextRequests to retrieve variable values in sequence.
* @throws IOException Thrown when timeout experienced while waiting for response to request.
* @throws SNMPBadValueException
*/
public SNMPVarBindList retrieveAllMIBInfo(String startID)
throws IOException, SNMPBadValueException
{
// send GetNextRequests until receive
// an error message or a repeat of the object identifier we sent out
SNMPVarBindList retrievedVars = new SNMPVarBindList();
int errorStatus = 0;
int errorIndex = 0;
SNMPObjectIdentifier requestedObjectIdentifier = new SNMPObjectIdentifier(startID);
SNMPVariablePair nextPair = new SNMPVariablePair(requestedObjectIdentifier, new SNMPNull());
SNMPSequence varList = new SNMPSequence();
varList.addSNMPObject(nextPair);
SNMPPDU pdu = new SNMPPDU(SNMPBERCodec.SNMPGETNEXTREQUEST, requestID, errorStatus, errorIndex, varList);
SNMPMessage message = new SNMPMessage(version, community, pdu);
byte[] messageEncoding = message.getBEREncoding();
DatagramPacket outPacket = new DatagramPacket(messageEncoding, messageEncoding.length, hostAddress, remotePort);
dSocket.send(outPacket);
while (errorStatus == 0)
{
DatagramPacket inPacket = new DatagramPacket(new byte[receiveBufferSize], receiveBufferSize);
dSocket.receive(inPacket);
byte[] encodedMessage = inPacket.getData();
SNMPMessage receivedMessage = new SNMPMessage(SNMPBERCodec.extractNextTLV(encodedMessage,0).value);
//errorStatus = ((BigInteger)((SNMPInteger)((receivedMessage.getPDU()).getSNMPObjectAt(1))).getValue()).intValue();
varList = (receivedMessage.getPDU()).getVarBindList();
SNMPSequence newPair = (SNMPSequence)(varList.getSNMPObjectAt(0));
SNMPObjectIdentifier newObjectIdentifier = (SNMPObjectIdentifier)(newPair.getSNMPObjectAt(0));
SNMPObject newValue = newPair.getSNMPObjectAt(1);
retrievedVars.addSNMPObject(newPair);
if (requestedObjectIdentifier.equals(newObjectIdentifier))
break;
requestedObjectIdentifier = newObjectIdentifier;
requestID++;
nextPair = new SNMPVariablePair(requestedObjectIdentifier, new SNMPNull());
varList = new SNMPSequence();
varList.addSNMPObject(nextPair);
pdu = new SNMPPDU(SNMPBERCodec.SNMPGETNEXTREQUEST, requestID, errorStatus, errorIndex, varList);
message = new SNMPMessage(version, community, pdu);
messageEncoding = message.getBEREncoding();
outPacket = new DatagramPacket(messageEncoding, messageEncoding.length, hostAddress, remotePort);
dSocket.send(outPacket);
}
return retrievedVars;
}
private String hexByte(byte b)
{
int pos = b;
if (pos < 0)
pos += 256;
String returnString = new String();
returnString += Integer.toHexString(pos/16);
returnString += Integer.toHexString(pos%16);
return returnString;
}
没有合适的资源?快使用搜索试试~ 我知道了~
snmp.rar_SNMP set_java SNMP get _open
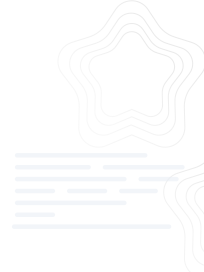
共214个文件
html:110个
java:50个
class:43个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 34 浏览量
2022-09-22
18:24:02
上传
评论
收藏 420KB RAR 举报
温馨提示
snmp inquistor Pour la communication avec les agents SNMP on utilise un package Java open source développé par Jonathan Sevy. Ce package contient notamment les différents types de données utilisés par SNMP, ainsi que des classes permettant l’envoi de requêtes GET et SET.
资源推荐
资源详情
资源评论
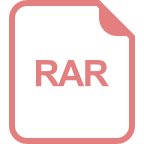
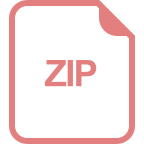
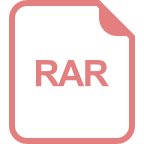
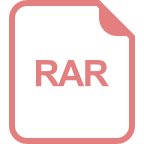
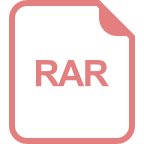
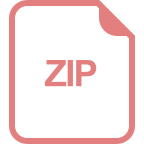
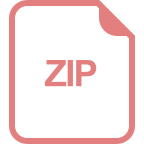
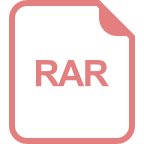
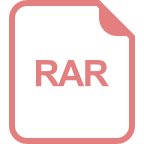
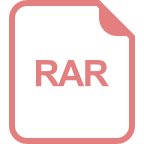
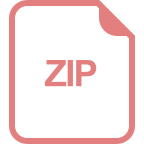
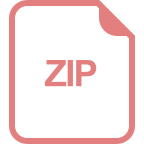
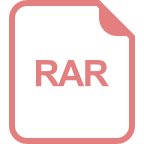
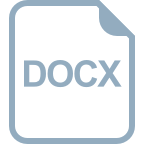
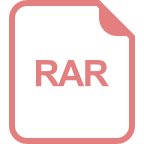
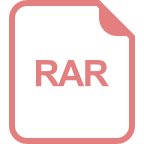
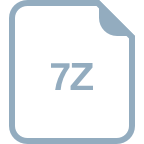
收起资源包目录

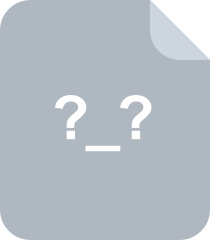
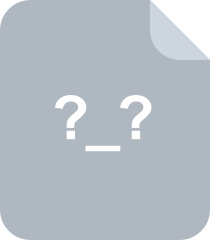
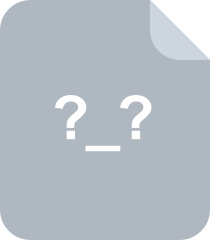
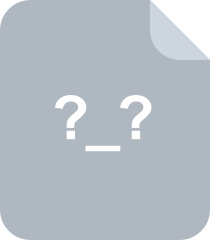
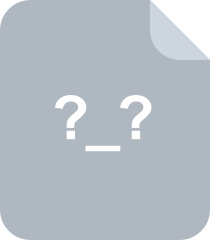
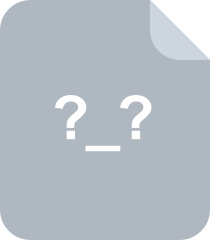
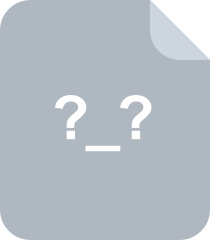
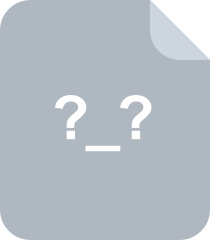
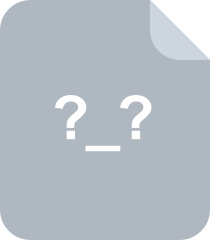
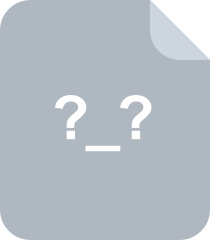
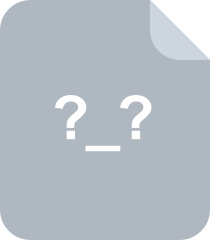
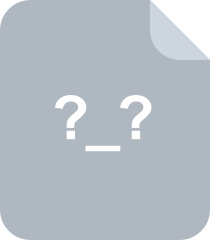
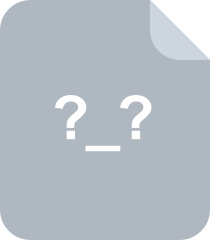
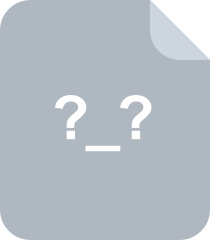
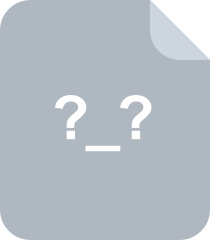
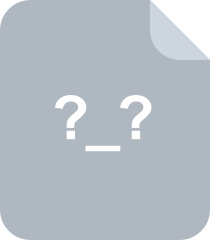
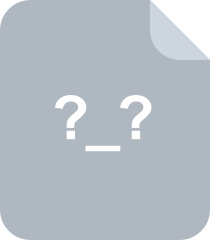
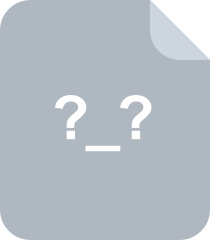
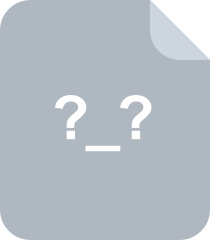
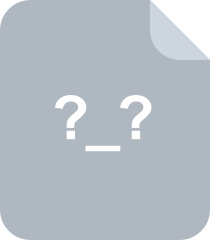
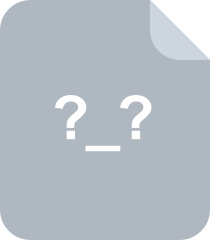
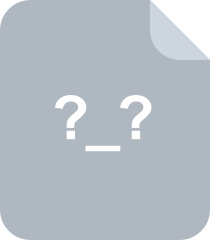
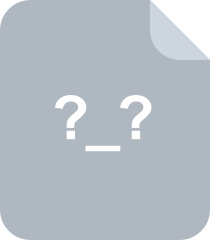
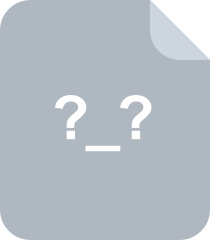
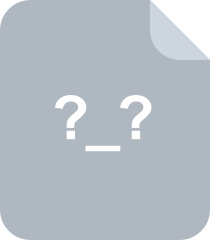
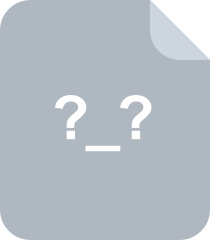
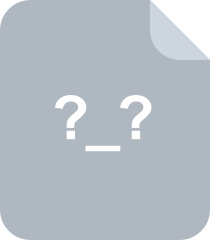
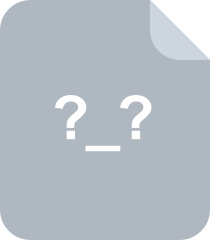
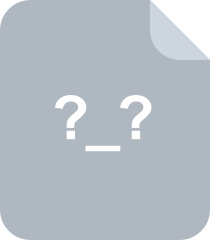
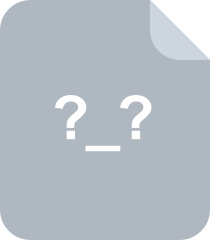
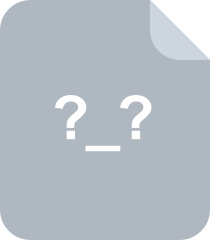
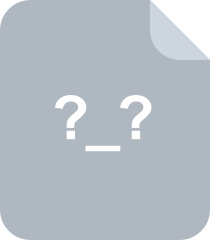
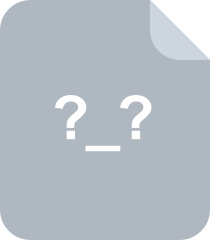
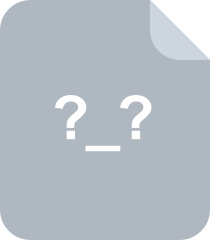
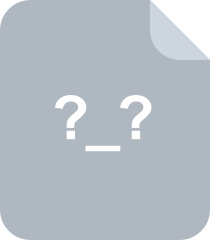
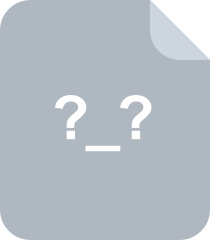
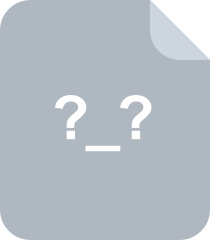
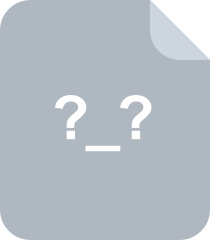
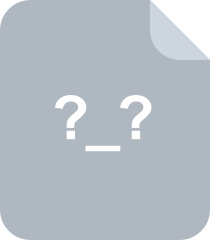
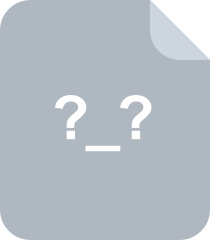
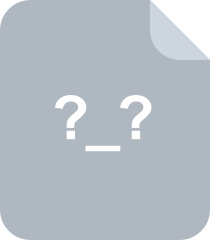
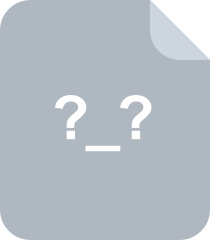
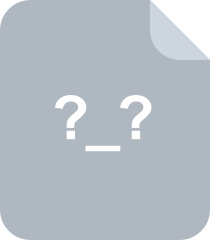
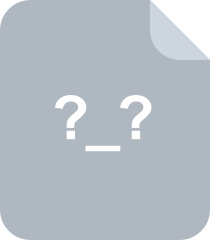
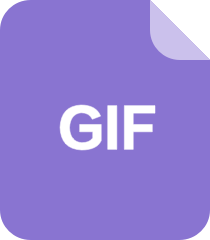
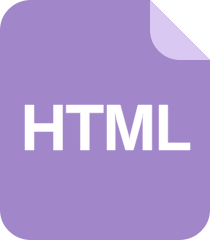
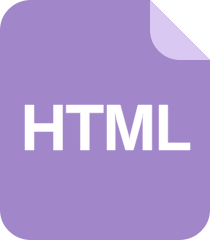
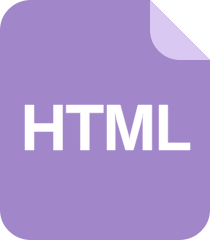
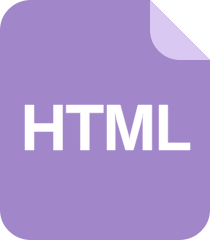
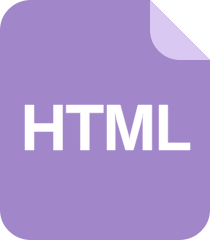
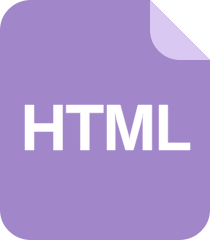
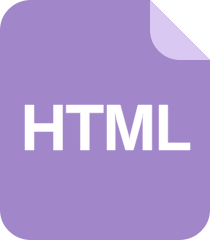
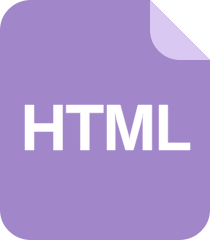
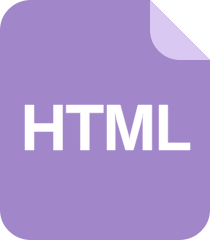
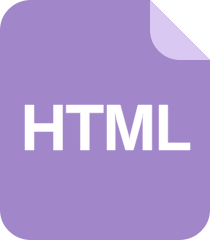
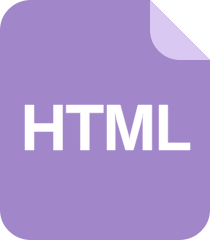
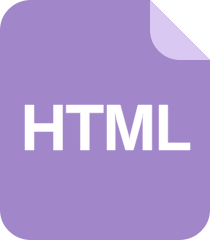
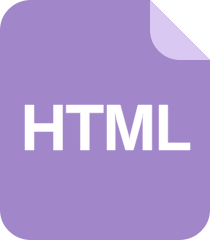
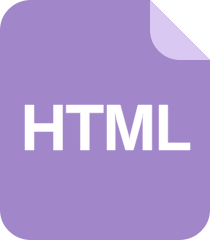
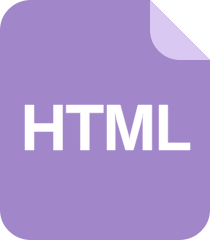
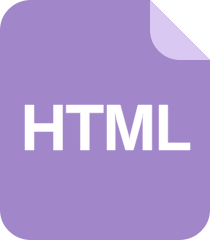
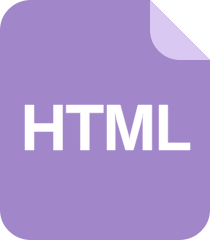
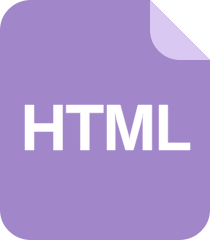
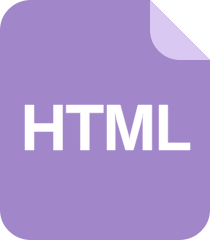
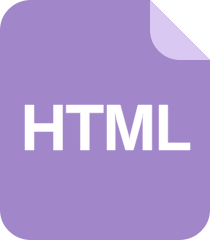
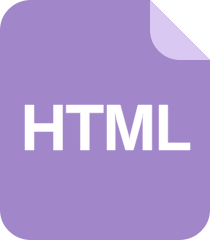
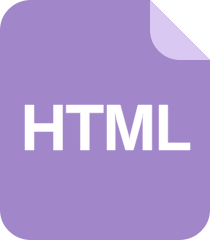
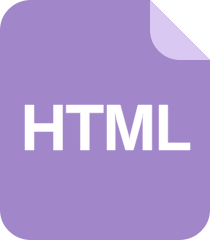
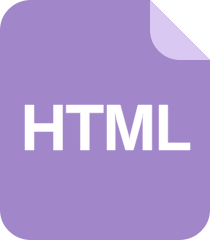
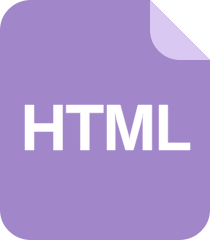
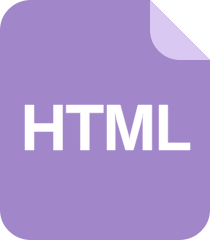
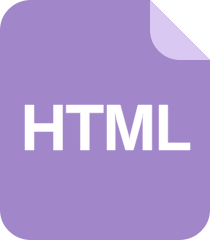
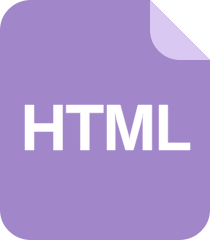
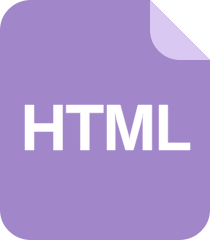
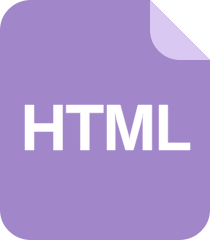
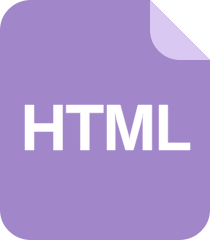
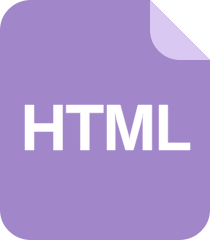
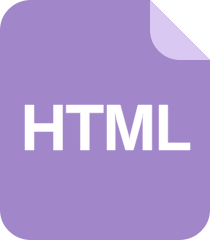
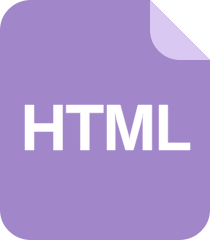
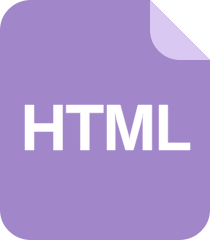
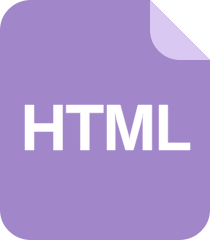
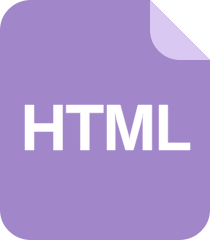
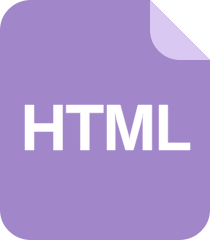
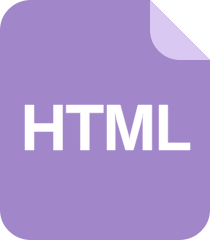
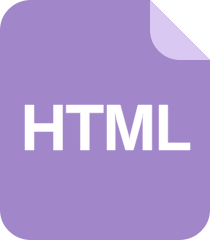
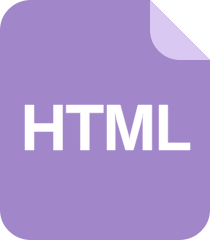
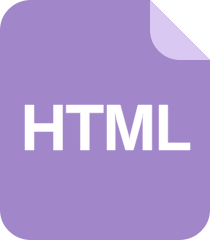
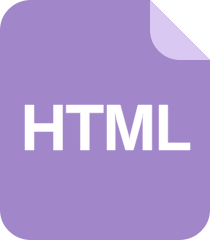
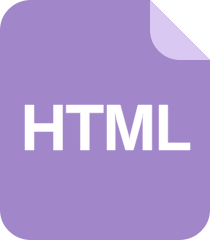
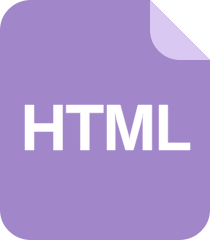
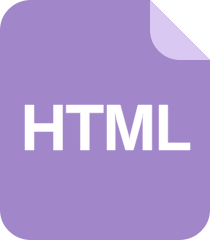
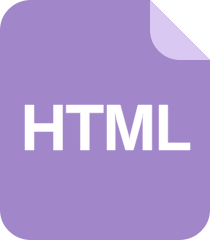
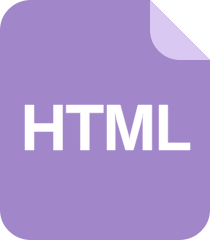
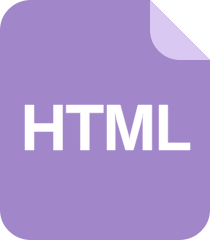
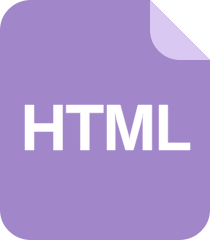
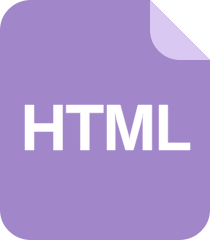
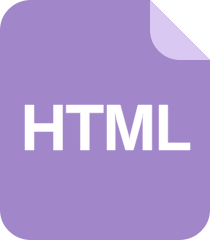
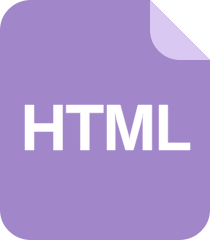
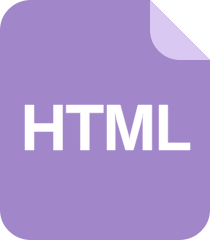
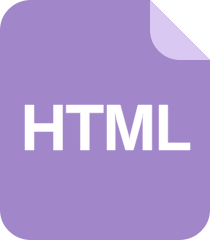
共 214 条
- 1
- 2
- 3
资源评论
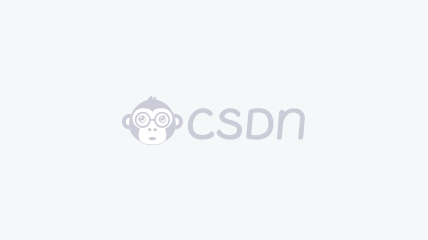

周楷雯
- 粉丝: 97
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

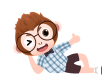
最新资源
- 每周质量安全排查报告.docx
- 排水报装接入申请表.docx
- 评估报告公示公众意见表.doc
- 评审、登记备案情况表.docx
- 墙板隐蔽前监理检查记录.docx
- 抢救室、输液室周带教计划表.docx
- 人防工程主体结构验收前监理人员检查记录表.docx
- 人防工程竣工验收前监理人员检查记录.docx
- 人防门框及临战封堵框常规数据检查表.docx
- 人防门扇常规数据检查表.docx
- 社区工作者岗位表.docx
- 涉及消防的建筑材料、构配件和设备的进场试验报告汇总表.docx
- 涉及消防的各分部分项工程消防查验结果表.docx
- 十级伤残鉴定标准表.docx
- 市标化优良工地检查自评表(施工、监理企业用表).docx
- 输液结束(拔针)流程表.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


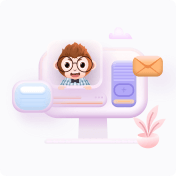
安全验证
文档复制为VIP权益,开通VIP直接复制
