**--------------文件信息-------------------------------------------------------------------------------
** 文件名称: i2c.c
** 创建日期: 2008-05-10
** 最终版本: 1.0
** 描 述: I2C操作函数库
********************************************************************************************************/
#include "config.h"
uint8 xdata i2c_buf[EEP_MAX_BYTES];
/*******************************************************************************************
** 函数名称: I2C_Start
** 函数描述: 模拟I2C发送起始条件
** 输入参数: 无
** 输出参数: 无
*******************************************************************************************/
void I2C_Start(void)
{
SDA_OUT;
SCL_OUT;
SDA_HI;
SCL_HI;
Delay_us(5);
SDA_LO;
Delay_us(5);
SCL_LO;
Delay_us(5);
}
/*******************************************************************************************
** 函数名称: I2C_Stop
** 函数描述: 模拟I2C发送结束条件
** 输入参数: 无
** 输出参数: 无
*******************************************************************************************/
void I2C_Stop(void)
{
SDA_OUT;
SCL_OUT;
SDA_LO;
SCL_LO;
Delay_us(5);
SCL_HI;
Delay_us(5);
SDA_HI;
Delay_us(5);
}
/*******************************************************************************************
** 函数名称: I2C_Ack
** 函数描述: 模拟I2C发送确认条件
** 输入参数: 无
** 输出参数: 无
*******************************************************************************************/
void I2C_Ack(uint8 ack)
{
SDA_OUT;
SCL_OUT;
if(ack)
{
SDA_HI;
}
else
{
SDA_LO;
}
Delay_us(5);
SCL_HI;
Delay_us(5);
SCL_LO;
Delay_us(5);
}
/*******************************************************************************************
** 函数名称: I2C_SendByte
** 函数描述: 模拟I2C发送一字节数据
** 输入参数: tmp:需要发送的数据
** 输出参数: ack :总线确认信号
*******************************************************************************************/
uint8 I2C_SendByte(uint8 tmp)
{
uint8 i;
uint8 ack;
SDA_OUT;
SCL_OUT;
for(i=0;i<8;i++)
{
if((tmp<<i)&0x80)
{
SDA_HI;
}
else
{
SDA_LO;
}
Delay_us(5);
SCL_HI;
Delay_us(5);
SCL_LO;
Delay_us(5);
}
Delay_us(5);
SDA_IN;
Delay_us(5);
SCL_HI;
Delay_us(5);
if(SDA)
{
ack=0;
}
else
{
ack=1;
}
Delay_us(5);
SCL_LO;
Delay_us(5);
return(ack);
}
/*******************************************************************************************
** 函数名称: I2C_ReceiveByte
** 函数描述: 模拟I2C接收一字节数据
** 输入参数:
** 输出参数: 接收到的数据
*******************************************************************************************/
uint8 I2C_ReceiveByte(void)
{
uint8 i;
uint8 tmp;
SDA_IN;
tmp=0;
for(i=0;i<8;i++)
{
Delay_us(5);
SCL_LO;
Delay_us(5);
SCL_HI;
Delay_us(5);
tmp<<=1;
if(SDA) tmp|=0x01;
Delay_us(5);
}
SCL_LO;
Delay_us(5);
return(tmp);
}
/******************************************************************************************
** 函数名称: EEP_WriteBytes
** 函数描述: 模拟I2C向EEPROM写入N个字节
** 输入参数:
** 输出参数:
*******************************************************************************************/
uint8 I2C_PageWrite(uint8 adr,uint8 *buf,uint8 cnt)
{
uint8 ack,i;
// WP_LO;
I2C_Start();
ack=I2C_SendByte(ADDR_24C02);
if(ack==0) return FALSE;
ack=I2C_SendByte(adr);
if(ack==0) return FALSE;
for(i=0;i<cnt;i++)
{
ack=I2C_SendByte(*(buf+i));
if(ack==0) return FALSE;
}
I2C_Stop();
// WP_HI;
return TRUE;
}
/******************************************************************************************
** 函数名称: EEP_ReadBytes
** 函数描述: 模拟I2C向EEPROM读取N个字节
** 输入参数:
** 输出参数:
*******************************************************************************************/
uint8 I2C_ReadBytes(uint8 adr,uint8 *buf,uint8 cnt)
{
uint8 ack,i;
I2C_Start();
ack=I2C_SendByte(ADDR_24C02);
if(ack==0) return(FALSE);
ack=I2C_SendByte(adr);
if(ack==0) return(FALSE);
I2C_Start();
ack=I2C_SendByte(ADDR_24C02|0x01);
if(ack==0) return(FALSE);
for(i=0;i<cnt-1;i++)
{
*(buf+i)=I2C_ReceiveByte();
I2C_Ack(0);
}
*(buf+cnt-1)=I2C_ReceiveByte();
I2C_Ack(1);
I2C_Stop();
return(TRUE);
}
///******************************************************************************************
//** 函数名称: EEP_ReadBytes
//** 函数描述: 不带校验读EEPROM
//** 输入参数: page:要操作的页
//** offset:数据在页内的偏移地址
//** *buf:数据地址
//** len:数据长度
//** 输出参数: TRUE/FALSE
//*******************************************************************************************/
//uint8 EEP_ReadBytes(uint8 page,uint8 offset,uint8 *buf,uint8 len)
//{
// uint8 i;
// uint8 adr;
// uint8 xdata tmpbuf[EEP_PAGESIZE];
//
// if(page>=EEP_MAX_PAGE) return FALSE;
// if((offset+len)>EEP_PAGESIZE ) return FALSE; //空间溢出
//
// for(i=0;i<3;i++) //最多读3次
// {
// adr=page*EEP_PAGESIZE;
// I2C_ReadBytes(adr+offset,buf,len);
// I2C_ReadBytes(adr+offset,tmpbuf,len);
// if(memcmp(buf,tmpbuf,len)!=0) continue;
// else return TRUE;
// }
// return FALSE;
//}
//
///******************************************************************************************
//** 函数名称: EEP_ReadBytes_WithCS
//** 函数描述: 带校验读EEPROM
//** 输入参数: page:要操作的页
//** offset:数据在页内的偏移地址
//** *buf:数据地址
//** len:数据长度
//** 输出参数: TRUE/FALSE
//*******************************************************************************************/
//uint8 EEP_ReadBytes_WithCS(uint8 page,uint8 offset,uint8 *buf,uint8 len)
//{
// uint8 i;
// uint8 adr;
// uint8 xdata tmpbuf[EEP_PAGESIZE];
// __U16_U08 crc;
//
// if(page>=EEP_MAX_PAGE) return FALSE;
// if((offset+len)>EEP_PAGESIZE ) return FALSE; //空间溢出
//
// for(i=0;i<3;i++) //最多读3次
// {
// adr=page*EEP_PAGESIZE;
// I2C_ReadBytes(adr,tmpbuf,EEP_PAGESIZE);
//
// crc.TBuf.buf0=tmpbuf[EEP_PAGESIZE-1];
// crc.TBuf.buf1=tmpbuf[EEP_PAGESIZE-2];
// if(Cal_CRC16(tmpbuf,EEP_PAGESIZE-2)==crc.val)
// {
// memcpy(buf,tmpbuf+offset,len);
// return TRUE;
// }
// }
// return FALSE;
//}
//
///******************************************************************************************
//** 函数名称: EEP_WriteBytes
//** 函数描述: 写EEPROM
//** 输入参数: page:要操作的页
//** offset:数据在页内的偏移地址
//** *buf:数据地址
//** len:数据长度
//** readchk:回读校验 0不回读校验,1使能回读校验
//** 输出参数: TRUE/FALSE
//*******************************************************************************************/
//uint8 EEP_WriteBytes(uint8 page,uint8 offset,uint8 *buf,uint8 len)
//{
// uint8 i;
// uint8 adr;
// __U16_U08 crc;
// uint8 xdata tmpbuf[EEP_PAGESIZE];
//
// if(page>=EEP_MAX_PAGE) return FALSE;
// if((offset+len)>EEP_PAGESIZE) return FALSE; //空间溢出
//
//
// EEP_ReadBytes(page,0,tmpbuf,EEP_PAGESIZE); //将一页数据取回
// for(i=0;i<len;i++) tmpbuf[offset+i]=buf[i]; //更新数据
// crc.val=Cal_CRC16(tmpbuf,EEP_PAGESIZE-2); //重新计算CRC
// tmpbuf[EEP_PAGESIZE-2]=crc.TBuf.buf1;
// tmpbuf[EEP_PAGESIZE-1]=crc.TBuf.buf0;
//
// adr=page*EEP_PAGESIZE;
//
// for(i=0;i<3;i++) //最多写3次
// {
// memcpy(i2c_buf,tmpbuf,EEP_PAGESIZE);
// I2C_PageWrit

周楷雯
- 粉丝: 94
- 资源: 1万+
最新资源
- Android开发笔记模拟器、应用教程pdf版最新版本
- <项目代码>YOLOv8 集装箱缺陷识别<目标检测>
- 【python毕业设计】基于Django的校园疫情监控平台源码(完整前后端+mysql+说明文档+LW).zip
- Android开发教程与笔记(文件存取与数据库编程知识)pdf版最新版本
- PHP客户关系CRM管理系统源码带文字搭建教程数据库 MySQL源码类型 WebForm
- 社区饮水机控制系统项目源码
- AndroidGoogle手机程序设计教程PDF版最新版本
- Android程序员向导(全面、基础型的Android编程教程)chm版最新版本
- 安然chuxing-12.01
- 海鹰HY1601超声波测深仪电源板详细电路图
- Delphi 12 控件之Delphi12htmx&webstencils-fastwebdevelopmentwithradstudio-1-69-translate.rar
- Delphi 12 控件之易语言百度网盘一刻相册上传文件+下载文件例子.rar
- JAVA的SpringBoot基于若依项目工时统计成本核算管理源码带教程数据库 MySQL源码类型 WebForm
- Delphi 12 控件之openssl-1.0.2q-x64-86-win64.rar
- Delphi 12 控件之DelphiXEIdHTTPServerUTF-8转换JSON解析源码测试可用89097-main.rar
- Delphi 12 控件之dotConnect-for-MySQL-v9.3.104-Professional.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


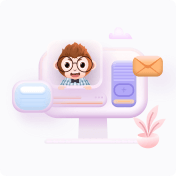