网格划分在计算机科学和工程计算领域,特别是在模拟和数值计算中扮演着至关重要的角色。它是一种将三维空间或二维平面划分为多个互不重叠的子区域,也称为“单元”或“元素”的过程。这些子区域构成了一个网格,允许我们将复杂的物理问题转化为在每个单元上独立解决的简单问题,然后通过边界条件和单元之间的相互作用来获得全局解决方案。"lianxi.rar_Mesh_区域划分_网格划分"这个压缩包文件,很可能包含了用于进行网格划分的源代码或工具,名为"lianxi.m"的文件可能是MATLAB或其他类似编程环境中的脚本或函数。 我们来了解一下网格划分的基本概念。网格划分通常有三种类型:结构网格、非结构网格和混合网格。结构网格的单元形状规则,如矩形或正六边形,适用于规则几何形状的问题。非结构网格则更为灵活,单元形状可以是任意多边形或四面体,适用于复杂几何形状和不规则边界。混合网格结合了两者,可以在不同区域使用不同类型的网格,以优化计算效率和精度。 在“巨星区域内划分”这个场景下,可能是指天体物理或者流体动力学模拟中的网格布置。巨星通常指的是质量极大、体积庞大的恒星,其内部的物理过程非常复杂,如核反应、对流等,这些都需要通过数值模拟来研究。网格划分在这里的任务就是将巨星的内部空间合理地分割,使得每个网格能准确反映局部的物理状态,同时保持整体的计算效率。 网格划分的步骤通常包括: 1. **定义域划分**:确定计算域的边界,并根据问题的特性选择合适的网格类型。 2. **细化处理**:在需要更高分辨率的区域(如边界层、热点等)增加网格密度,以提高计算精度。 3. **生成网格**:使用算法(如Delaunay三角化、Advancing Front方法等)生成网格。 4. **质量检查与优化**:确保网格的质量参数(如形状因子、面积比等)满足一定的标准,以保证数值稳定性。 5. **接口处理**:处理单元间的连接,确保信息传递的正确性。 在MATLAB环境中,进行网格划分可能涉及的函数或工具箱有PDE Toolbox、FEM Toolbox或自定义的脚本。"lianxi.m"可能包含用于读取几何数据、定义网格属性、调用网格生成函数以及后处理的代码。用户可能需要根据输入的巨星模型数据,运行这个脚本来生成所需的网格。 网格划分的结果将直接影响数值模拟的精度和计算效率。因此,选择合适的网格划分策略和技术,以及优化网格质量,是数值模拟中至关重要的一步。对于"lianxi.rar"这个文件的具体内容,我们需要解压并查看代码才能进一步分析其工作原理和应用。
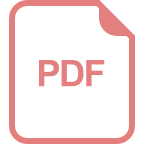
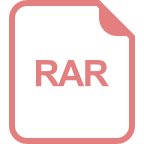
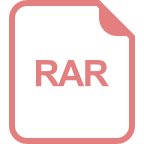
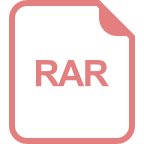
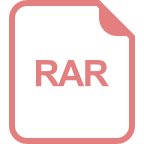
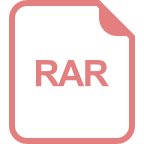
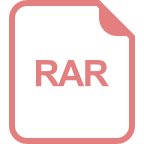
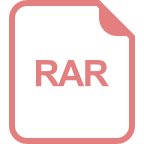
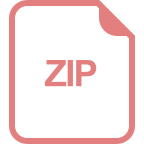
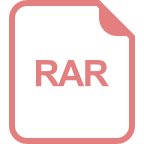
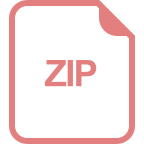
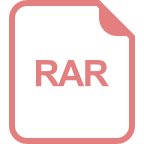
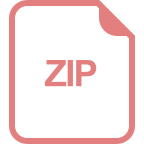
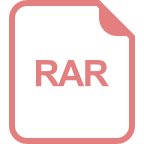

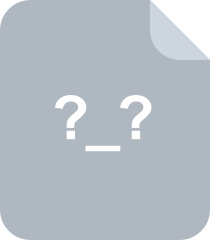
- 1
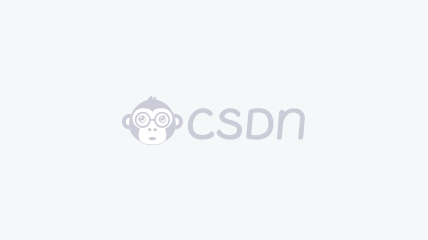

- 粉丝: 97
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

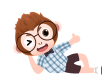
最新资源
- 基于Java实现的MapReduce分布式计算框架设计源码
- Qwen2.5 Technical Report 详细技术报告
- 基于ThinkGms v2.0.1框架的旧快马配送系统设计源码
- 基于Java编程语言的俄罗斯方块游戏设计源码
- 套膜封切机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 小麦联合收割机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 小型全自动卷烟机构图纸工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 线体牵引力测试机(含bom)sw17可编辑工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 前端入门day1的文件记录
- 型钢校正机矫直机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 旋转停车系统工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 数仓构造与多维分析大作业
- 【图像融合】基于matlab结合contourlet与压缩感知图像融合【含Matlab源码 9741期】.zip
- 【坐标转换】基于matlab GUI大地坐标和空间直角坐标相互转换【含Matlab源码 9227期】.zip
- 【迷宫路径规划】基于matlab SARSA和强化学习迷宫路径规划解决迷宫问题【含Matlab源码 8857期】.mp4
- 【语音去噪】基于matlab GUI切比雪夫+椭圆形低通滤波器语音去噪【含Matlab源码 2198期】.mp4

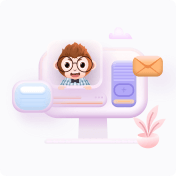
