%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
%
% Simulator for Attentional Strategies
%
% By: Kevin Passino
% Version: 4/19/01
%
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
clear
Tmax=100; % Length of simulation time (real time, in seconds)
Tsample=0.01; % Sampling period (used to discretize)
Nsteps=(1/Tsample)*Tmax; % Number of steps to run the simulation
N=4; % Number of predators/prey
T=0*ones(N,Nsteps+1); % T(i,k) will denote the number of steps that predator/prey i has
% been ignored as of step k. This simply allocates memory
% for it.
%-------------------------
% Predator/prey characteristics:
%-------------------------
e=0*ones(N,Nsteps+1); % Allocate memory for predators/prey signals
% Define predators/prey appearance instants (note that f(i,1) refers to predator/prey i, and that predator/prey i
% has priority i, where higher values of i indicate higher priority predators/prey)
f(:,1)=0*ones(N,1); % Allocate memory
f(1,1)=1.2*(1/Tsample); % Sets that we want an appearance from predator/prey 1 every f(1,1) steps (1.2 sec.)
% (this sets the frequency of appearance)
f(2,1)=1.5*(1/Tsample);
f(3,1)=2*(1/Tsample);
f(4,1)=1*(1/Tsample);
%f(5,1)=1.7*(1/Tsample);
fcounter(:,1)=ones(N,1); % Allocate memory for counters
% Next, define the bounds delta^i on the maximum amount of time between the appearances of each predator/prey
% (note that these must be consistent with the choice for the f(;,1) parameters above that define when the
% appearances occur). These are given in real time, not steps.
deltai(1,1)=1.3; % Overbounds a choice of 1.2 above
deltai(2,1)=1.6;
deltai(3,1)=2.3;
deltai(4,1)=1.1;
%deltai(5,1)=1.75;
%---------------------------
% Organism characteristics:
%---------------------------
% Pick attentional strategy (scheduler) type
sched=1; % Chooses the policy of choosing the predator/prey that has been ignored for the longest time
%sched=2; % Chooses the policy of choosing the predator/prey that is the highest priority one that has
% been ignored longer than the average one
%sched=3; % Chooses the policy of choosing the predators/prey that may be the most difficult to find
% (of course if the a(:,1) are all the same then this is the same as sched=1)
%sched=4; % Chooses the policy of choosing the predators/prey that may be most difficult to detect
% For sched=4, need weighting factors
w(1,1)=2;
w(2,1)=1;
w(3,1)=1;
w(4,1)=1;
peakest=0*ones(N,1); % Allocate memory for computation of estimate of peak for sched=4
% Set the a_i parameters so that the capacity condition is met:
epsilon=0.1; % Used to define a_i parameters next
a(:,1)=epsilon*(1/N)*ones(N,1); % Picks so will meet capacity condition - scale it
% (other choices are possible)
% Another choice:
%a(1,1)=0.1;
%a(2,1)=0.2;
%a(3,1)=0.3;
%a(4,1)=0.399;
deltas=1*Tsample; % Sets the delay (in steps) that it takes before the organism can
% switch from focusing on one predator/prey to another.
% NOTE: This only accounts for part of the "delta" used in the book.
% This is the part due to switching from one predator/prey to another.
deltacounter=0; % Used to implement the deltas delay
decisiontime=1; % Signals that it is a time (if =1) that pick a new predators/prey
% to focus on (initially pick one to focus on)
predpreyfocusedon=0*ones(Nsteps+1,1); % Initialize variable that indicates which predators/prey is
% currently being focused on (if in a delay period, means
% the one that it is trying to focus on)
predpreyprocess=0; % Flag to indicate that switch delay is over
detectedpredprey=0; % Flag that indicates that the predators/prey that is focused on is detected
% (=0 means not detected, =1 means detected)
%-------------------------------------------------
% Variables for measuring performance:
%-------------------------------------------------
avgT=0*ones(Nsteps+1,1); % avgT(k,1) denotes the average size of T at each step
% (average of N values)
maxT=0*ones(Nsteps+1,1); % maxT(k,1) denotes the maximum size of any T at each step
% (maximum of N values)
avgP=0; % Time average of priorities
%-----------------------------------
% Start simulation loop:
%-----------------------------------
for k=1:Nsteps
for i=1:N % First generate the appearance signals at step k
if fcounter(i,1)>=f(i,1); % Check if have waited long enough to appear
%(notice here have >= so since due to how the frequency of
% occurences of appearances are defined so that f can be fraction)
e(i,k+1)=1; % Appear
fcounter(i,1)=1; % Reset counter
else
e(i,k+1)=0; % Do not appear
fcounter(i,1)=fcounter(i,1)+1; % Increment counter
end
end
% Compute some performance measures that can be used in decision making:
% (and for plotting later)
avgT(k,1)=(1/N)*sum(T(:,k));
maxT(k,1)=max(T(:,k));
if decisiontime~=1;
predpreyfocusedon(k,1)=predpreyfocusedon(k-1,1); % If not a decision time then same focus as last time
end
% Decide which predators/prey to focus on:
if decisiontime==1; % Test if it is a decision time
% Policy of choosing the predator/prey that has been ignored for the longest time
if sched==1
[val,predpreyfocusedon(k,1)]=max(T(:,k)); % Pick predator/prey that has largest T at time k
end
% Policy of choosing the predator/prey that is the highest priority one that has
% been ignored longer than the average one
if sched==2
for j=1:N % Consider predators/prey numbered 1,..,N with N being the highest priority predator/prey
if T(j,k)>=avgT(k,1) % Pick the predator/prey encountered that is above the average (so
% this picks the highest priority one that is above the average)
% Of course there are some wasted computations here...
predpreyfocusedon(k,1)=j;
end
end
end
% Policy of choosing the predator/prey that may be the most difficult to find
if sched==3
[val,predpreyfocusedon(k,1)]=max(a(:,1).*T(:,k)); % Scales the T(i,k)
% elements by a(i,1) in making choice
end
% Policy of choosing the predators/prey that may be most difficult to detect
if sched==4
for j=1:N
peakest(j,1)=w(j,1)*(a(j,1)/(1-a(j,1)))*(T(j,k)+deltas+deltai(j,1));
end
[val,predpreyfocusedon(k,1)]=max(peakest); % Picks the predator/prey expected to have the highest peak
end
decisiontime=0; % Do not decide again until detect that predators/prey (consider it
% "detected" when T(i,.) goes to zero)
end
if deltacounter>=deltas % First, implement delay due to switching from one predator/prey to another
predpreyprocess=1; % Flag to indicate that we are now going to focus on the predator/prey
else
deltacounter=deltacounter+Tsample; % Increment delay since computing T(i,k+1) (note that
% at every time we are focusing on something so
% at every time we need to compute this delay)
% Also, note that below the test for the delay
% accounts for incrementing the counter past delta
end
if predpreyprocess==1 % If switch delay is over, then can start trying to detect predator/prey
if e(predpreyfocusedon(k,1),k)==1 % Consider this test for "detection" to only indicate one pulse
detectedpredprey=1; % If detect predators/prey set flag
% D=k; % The time that an predators/prey pulse was found
end
end
if predpreyprocess==1 & detectedpredprey==1
deltaT=((1-a(predpreyfocusedon(k,1),1))/a(predpreyfocusedon(k,1),1))*Tsample;
if T(predpreyfocusedon(k,1),k)-deltaT>0; % Must test if will hit zero (to keep nonneg)
T(predpreyfocusedon(k,1),k+1)=T(predpreyfocusedon(k,1),k)-deltaT;
else
T(predpreyfocusedon(k,1),k+1)=0; % Set it
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
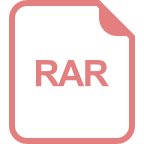
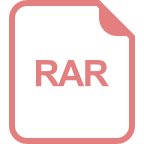
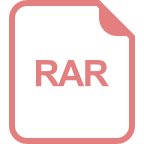
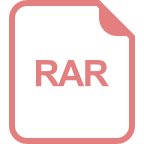
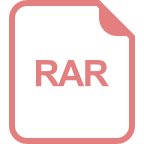
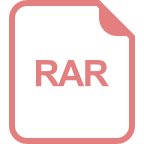
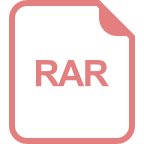
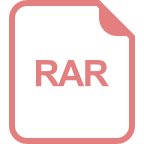
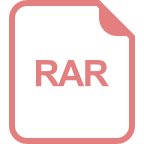
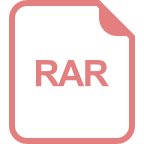
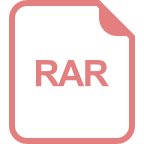
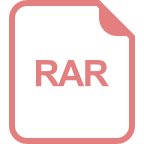
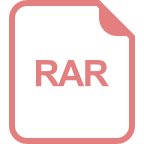
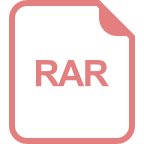
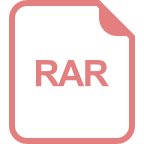
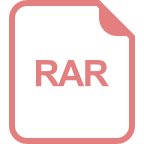
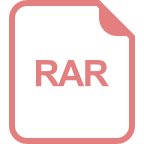
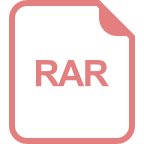
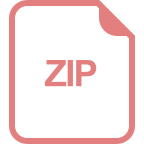
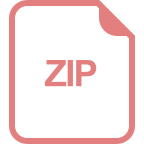
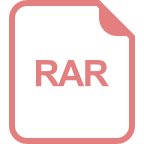
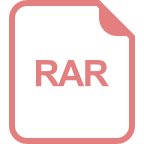
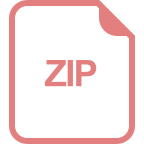
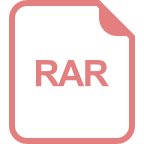
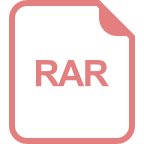
收起资源包目录

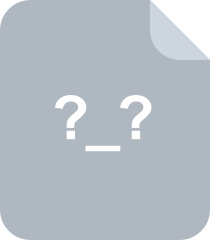
共 1 条
- 1
资源评论
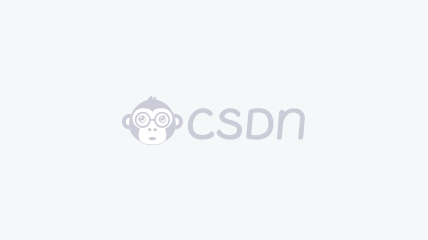

刘良运
- 粉丝: 77
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

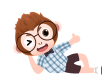
最新资源
- 质量安全排查报告.docx
- 职业中专技工学校专业评估表.docx
- 质量控制资料核查表:建筑保温工程.docx
- 质量目标统计数据表.docx
- 质量内审方案.docx
- 中国古今地名对照表.docx
- 智力残疾评定标准一览表.docx
- 中央造林补助实施方案小班一览表.docx
- 肘关节功能丧失程度评定表.docx
- 重要神经及血管损伤评定.docx
- 自建房安全整治和农村住房建设考评内容和评分标准.docx
- 走访服务企业登记表.doc
- 智能车开发技术的多领域深度解析及应用
- 西红柿叶片图像目标检测数据【已标注,约700张数据,YOLO 标注格式】
- 蓝桥杯开发技术的全面解析与备赛建议
- 相当于去中心化的QQ版本了
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


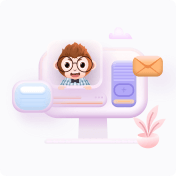
安全验证
文档复制为VIP权益,开通VIP直接复制
