// ecctest4.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
// Runtime Includes
#include <iostream>
#include <string>
// Crypto++ Library
#ifdef _DEBUG
# pragma comment ( lib, "cryptlibd" )
#else
# pragma comment ( lib, "cryptlib" )
#endif
// Crypto++ Includes
#include "cryptlib.h"
#include "osrng.h" // Random Number Generator
#include "eccrypto.h" // Elliptic Curve
#include "ecp.h" // F(p) EC
#include "integer.h" // Integer Operations
#include "base64.h" // Encodeing-Decoding
// The Features Available...
const unsigned int FEATURE_EVALUATION = 0x01; // 0000 0001
const unsigned int FEATURE_USE_SQUARES = 0x02; // 0000 0010
const unsigned int FEATURE_USE_CIRCLES = 0x04; // 0000 0100
const unsigned int FEATURE_USE_WIDGETS = 0x08; // 0000 1000
const unsigned int FEATURE_MAGIC = 0xAAAA << 16; // 1010 1010 1010 1010 0000 0000 0000 0000
const unsigned int FEATURE_MAGIC_MASK = 0xFFFF << 16; // 1111 1111 1111 1111 0000 0000 0000 0000
//
// The following parameters were generated using Elliptic Curve Builder
// ECB can be downloaded at http://www.ellipsa.net/
//
// Curve of Order R*S over GF(P)
// -----------------------------
// P = 4727818631 (33 bits)
// R = 1575934013
// S = 3
// A = 3145529313
// B = -1347526597
//
// Point of Order R
// ----------------
// X = -1556174464
// Y = -2217668713
int main(int argc, char* argv[]) {
byte* CipherText = NULL;
byte* EncodedText = NULL;
byte* DecodedText = NULL;
try {
CryptoPP::AutoSeededRandomPool rng;
// User Defined Domain Parameters
CryptoPP::Integer p("4727818631");
CryptoPP::Integer n("1575934013"); // R from ECB
CryptoPP::Integer h("3"); // S from ECB, must be <= 4
CryptoPP::Integer a("3145529313");
CryptoPP::Integer b("-1347526597");
CryptoPP::Integer x("-1496737238");
CryptoPP::Integer y("-1055837300");
CryptoPP::ECP ec( p, a, b );
CryptoPP::ECIES< CryptoPP::ECP >::PrivateKey PrivateKey;
CryptoPP::ECIES< CryptoPP::ECP >::PublicKey PublicKey;
//
// Curve Initialization and Key Generation
//
PrivateKey.Initialize( ec, CryptoPP::ECP::Point( x, y ), n, h );
PrivateKey.MakePublicKey( PublicKey );
//
// Key Validation
//
// User Defined Domain Parameters always fail, even at level 0???
//
// From cryptlib.h, Validate(...):
//
// 0 - using this object won't cause a crash or exception (rng is ignored)
// 1 - this object will probably function (encrypt, sign, etc.) correctly
// (but may not check for weak keys and such)
// 2 - make sure this object will function correctly, and do reasonable
// security checks
// 3 - do checks that may take a long time
//
// if( false == PrivateKey.Validate( rng, 0 ) )
// { throw std::string( "Private Key Validation" ); }
// if( false == PublicKey.Validate( rng, 0 ) )
// { throw std::string( "Public Key Validation" ); }
// Encryptor and Decryptor
CryptoPP::ECIES< CryptoPP::ECP >::Encryptor Encryptor( PublicKey );
CryptoPP::ECIES< CryptoPP::ECP >::Decryptor Decryptor( PrivateKey );
// Message
unsigned int Features = 0;
Features |= FEATURE_MAGIC;
Features |= FEATURE_USE_WIDGETS;
Features |= FEATURE_USE_SQUARES;
// Runtime Sizes...
unsigned int PlainTextLength = sizeof( Features );
unsigned int CipherTextLength = Encryptor.CiphertextLength( PlainTextLength );
if( 0 == CipherTextLength )
{ throw std::string("plaintextLength is not valid (too long)"); }
// Scratch for Encryption
CipherText = new byte[ CipherTextLength ];
if( NULL == CipherText )
{ throw std::string( "CipherText Allocation Failure" ); }
// Encryption
Encryptor.Encrypt( rng, reinterpret_cast<const byte*>( &Features ),
PlainTextLength, CipherText );
// Base 64 Encoding
CryptoPP::Base64Encoder Encoder;
Encoder.Put( CipherText, CipherTextLength );
Encoder.MessageEnd();
// Scratch for Base 64 Encoded Ciphertext
unsigned int EncodedTextLength = Encoder.MaxRetrievable();
EncodedText = new byte[ EncodedTextLength + 1 ];
if( NULL == EncodedText )
{ throw std::string( "Base64 EncodedText Allocation Failure" ); }
EncodedText[ EncodedTextLength ] = '\0';
// Fetch Base 64 Encoded Ciphertext
Encoder.Get( EncodedText, EncodedTextLength );
//
// Break the Product Key Here
//
// Diagnostics...
std::cout << "Encoded Text Before Tampering:" << std::endl << EncodedText << std::endl;
// Output
if( FEATURE_EVALUATION == (Features & FEATURE_EVALUATION ) )
{ std::cout << "Evaluation Edition." << std::endl; }
if( FEATURE_USE_SQUARES == (Features & FEATURE_USE_SQUARES) )
{ std::cout << "Operations are permitted on Squares." << std::endl; }
if( FEATURE_USE_CIRCLES == (Features & FEATURE_USE_CIRCLES) )
{ std::cout << "Operations are permitted on Circles." << std::endl; }
if( FEATURE_USE_WIDGETS == (Features & FEATURE_USE_WIDGETS) )
{ std::cout << "Operations are permitted on Widgets." << std::endl; }
std::cout << std::endl << "********************" << std::endl << std::endl;
// The folllowing introduces 1 random error
EncodedText[ 1 ] = 'W';
// The folllowing introduces multiple random errors
// Guessing at a Product Key
/*
char ch = 'A';
for( unsigned int i = 0; i < EncodedTextLength - 1; i += 3 )
{
EncodedText[ i ] = ch++;
}
*/
// Diagnostics...
std::cout << "Encoded Text After Tampering:" << std::endl << EncodedText << std::endl;
// Base 64 Decoding
CryptoPP::Base64Decoder Decoder;
Decoder.Put( EncodedText, EncodedTextLength );
Decoder.MessageEnd();
// Scratch for Base 64 Decoded Ciphertext
unsigned int DecodedTextLength = Decoder.MaxRetrievable();
DecodedText = new byte[ DecodedTextLength ];
if( NULL == DecodedText )
{ throw std::string( "Base64 DecodedText Allocation Failure" ); }
// Fetch Base 64 Decoded Ciphertext
Decoder.Get( DecodedText, DecodedTextLength );
// Scratch for Decryption
unsigned int RecoveredTextLength = Decryptor.MaxPlaintextLength( DecodedTextLength );
if( 0 == RecoveredTextLength )
{ throw std::string("ciphertextLength is not valid (too long or too short)"); }
// Decryption Buffer
unsigned int RecoveredText = static_cast<int>( -1 ); // 1111 ... 1111
if( NULL == RecoveredText )
{ throw std::string( "RecoveredText CipherText Allocation Failure" ); }
// Decryption
Decryptor.Decrypt( rng, DecodedText, DecodedTextLength,
reinterpret_cast<byte *>( &RecoveredText ) );
// Output
if( FEATURE_MAGIC != (RecoveredText & FEATURE_MAGIC_MASK ) )
{
std::cout << "Invalid Product Key!" << std::endl;
}
else
{
if( FEATURE_EVALUATION == (RecoveredText & FEATURE_EVALUATION ) )
{ std::cout << "Evaluation Edition." << std::endl; }
if( FEATURE_USE_SQUARES == (RecoveredText & FEATURE_USE_SQUARES) )

邓凌佳
- 粉丝: 79
- 资源: 1万+
最新资源
- java毕设项目之ssm基于Java的共享客栈管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于java的健身房管理系统的设计与实现+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于java和mysql的多角色学生管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于Java的图书管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于java的少儿编程网上报名系统+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于Java语言校园快递代取系统的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于jsp的精品酒销售管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于JSP的乡镇自来水收费系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于SSM的高校共享单车管理系统的设计与实现+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于ssm的人才招聘网站+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于SSM框架的购物商城系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于SSM框架的个人博客网站的设计与实现+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于ssm的新能源汽车在线租赁管理系统+vue(完整前后端+说明文档+mysql+lw).zip
- 小目标尺寸下的地表信息图像分类数据集【已标注,约30,000张数据】
- java毕设项目之ssm家政服务网站设计+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于Web的智慧城市实验室主页系统设计与实现+vue(完整前后端+说明文档+mysql+lw).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


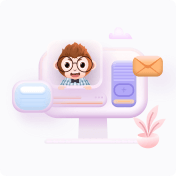