//###########################################################################
//
// FILE: F2837xS_DefaultISR.c
//
// TITLE: F2837xS Device Default Interrupt Service Routines
//
//###########################################################################
// $TI Release: F2837xS Support Library v180 $
// $Release Date: Fri Nov 6 16:27:58 CST 2015 $
// $Copyright: Copyright (C) 2014-2015 Texas Instruments Incorporated -
// http://www.ti.com/ ALL RIGHTS RESERVED $
//###########################################################################
#include "F2837xS_device.h" // F2837xS Header File Include File
#include "F2837xS_Examples.h" // F2837xS Examples Include File
//---------------------------------------------------------------------------
// CPU Timer 1 Interrupt
interrupt void TIMER1_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// CPU Timer 2 Interrupt
interrupt void TIMER2_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// Datalogging Interrupt
interrupt void DATALOG_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// RTOS Interrupt
interrupt void RTOS_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// Emulation Interrupt
interrupt void EMU_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// Non-Maskable Interrupt
interrupt void NMI_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// Illegal Operation Trap
interrupt void ILLEGAL_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 1
interrupt void USER1_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 2
interrupt void USER2_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 3
interrupt void USER3_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 4
interrupt void USER4_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 5
interrupt void USER5_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 6
interrupt void USER6_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 7
interrupt void USER7_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 8
interrupt void USER8_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 9
interrupt void USER9_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 10
interrupt void USER10_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 11
interrupt void USER11_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// User Defined Trap 12
interrupt void USER12_ISR(void)
{
// Insert ISR Code here
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// 1.1 - ADCA Interrupt 1
interrupt void ADCA1_ISR(void)
{
// Insert ISR Code here
// To receive more interrupts from this PIE group,
// acknowledge this interrupt.
// PieCtrlRegs.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// 1.2 - ADCB Interrupt 1
interrupt void ADCB1_ISR(void)
{
// Insert ISR Code here
// To receive more interrupts from this PIE group,
// acknowledge this interrupt.
// PieCtrlRegs.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// 1.3 - ADCC Interrupt 1
interrupt void ADCC1_ISR(void)
{
// Insert ISR Code here
// To receive more interrupts from this PIE group,
// acknowledge this interrupt.
// PieCtrlRegs.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// 1.4 - XINT1 Interrupt
interrupt void XINT1_ISR(void)
{
// Insert ISR Code here
// To receive more interrupts from this PIE group,
// acknowledge this interrupt.
// PieCtrlRegs.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// 1.5 - XINT2 Interrupt
interrupt void XINT2_ISR(void)
{
// Insert ISR Code here
// To receive more interrupts from this PIE group,
// acknowledge this interrupt.
// PieCtrlRegs.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// 1.6 - ADCD Interrupt 1
interrupt void ADCD1_ISR(void)
{
// Insert ISR Code here
// To receive more interrupts from this PIE group,
// acknowledge this interrupt.
// PieCtrlRegs.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only to halt the processor here
// Remove after inserting ISR Code
asm (" ESTOP0");
for(;;);
}
// 1.7 - Timer 0 Interrupt
interrupt void TIMER0_ISR(void)
{
// Insert ISR Code here
// To receive more interrupts from this PIE group,
// acknowledge this interrupt.
// PieCtrlRegs.PIEACK.all = PIEACK_GROUP1;
// Next two lines for debug only to halt the processor here
没有合适的资源?快使用搜索试试~ 我知道了~
tms320f28377d_timer.rar_tms320f28377d_定时器
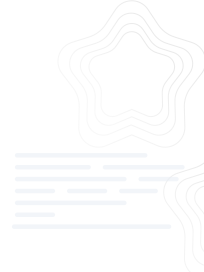
共114个文件
h:64个
obj:10个
pp:8个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
定时器中断典型例程,介绍了定时器中断的寄存器配置,编写理定时器中断代码,供程序设计参考。
资源详情
资源评论
资源推荐
收起资源包目录

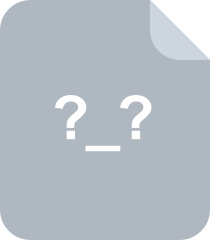
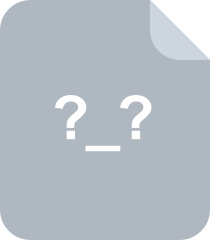
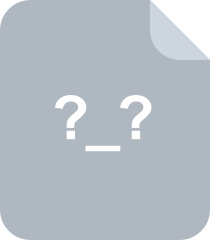
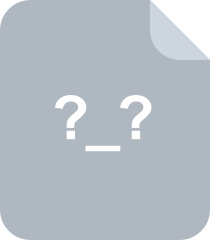
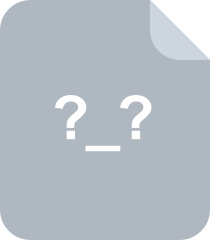
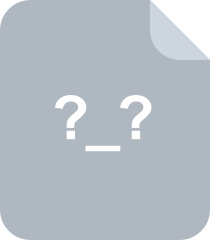
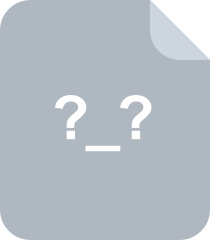
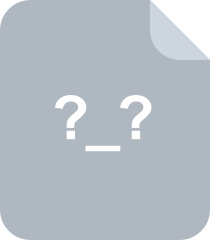
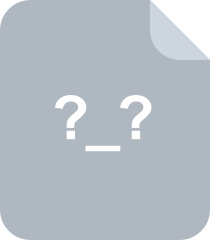
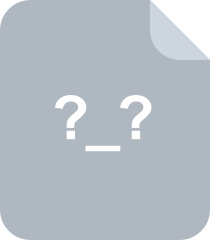
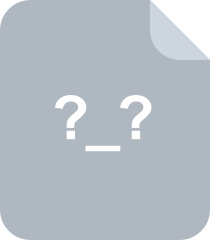
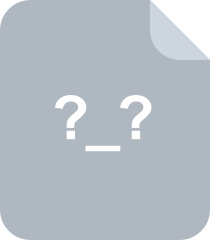
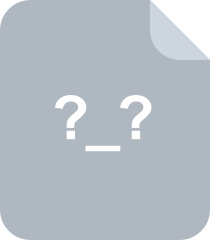
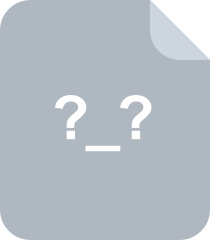
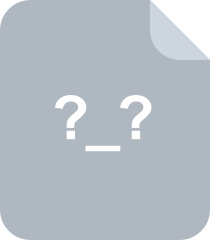
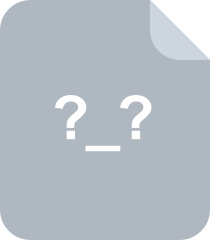
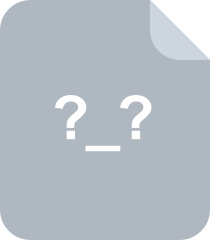
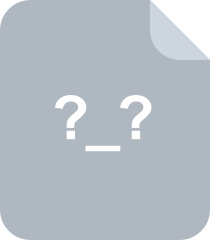
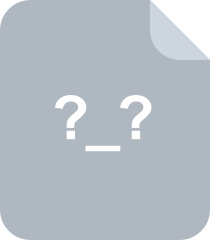
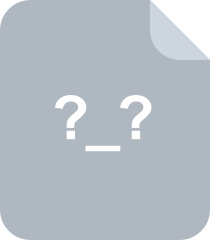
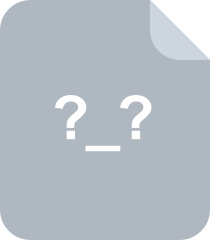
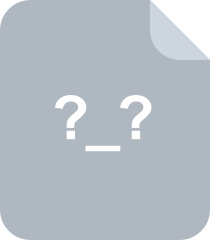
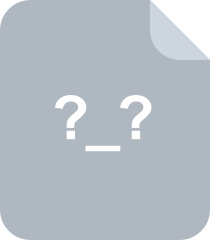
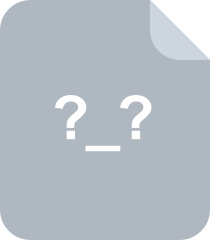
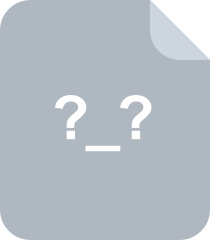
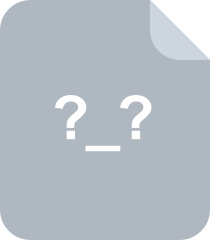
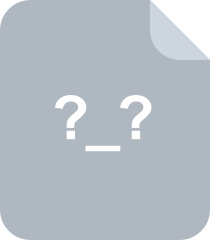
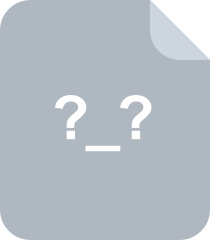
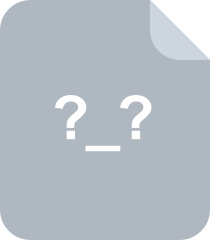
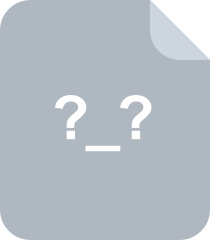
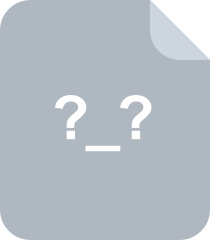
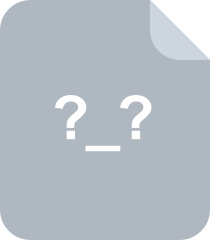
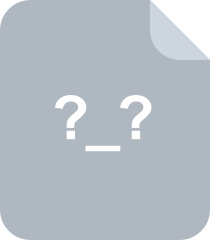
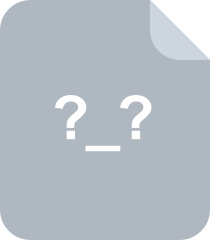
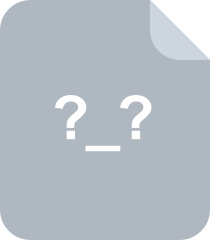
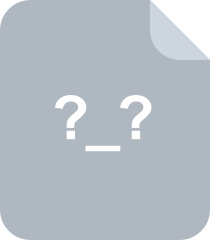
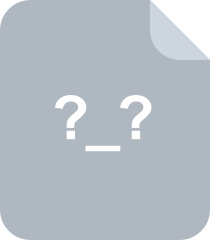
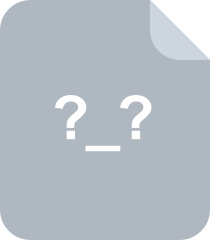
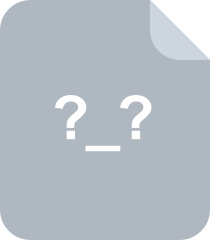
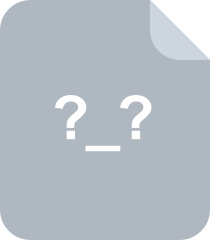
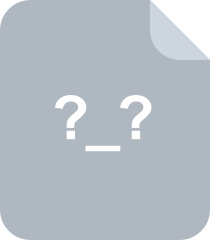
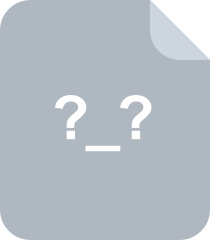
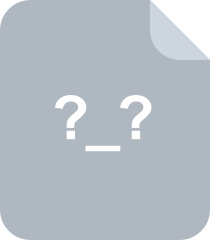
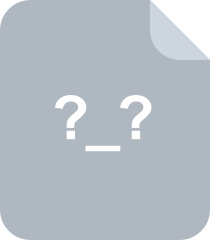
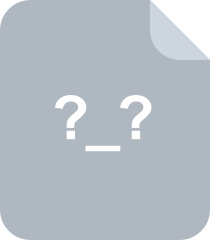
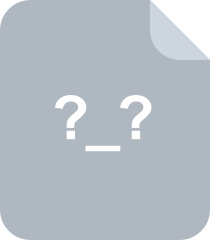
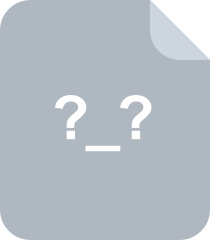
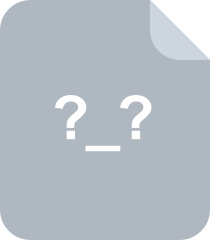
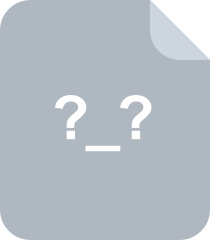
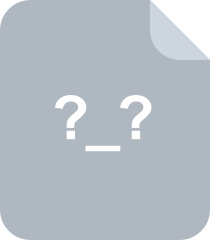
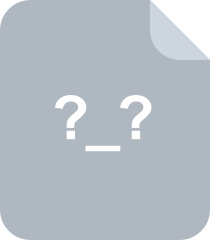
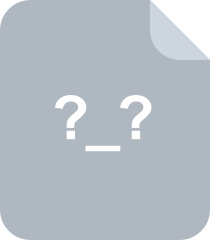
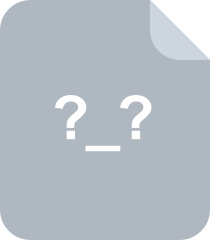
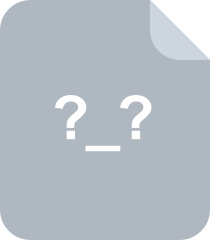
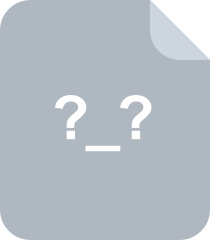
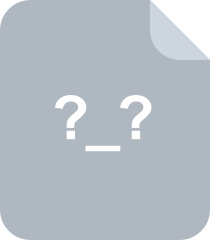
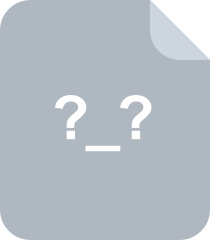
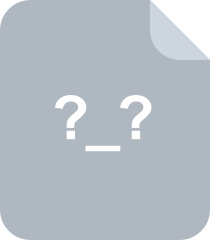
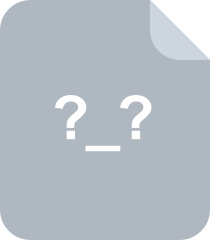
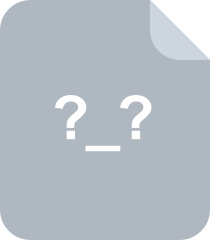
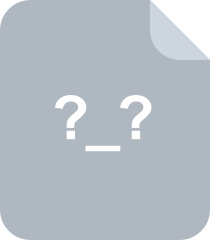
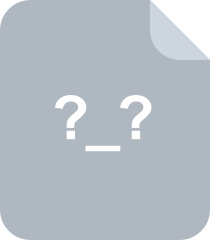
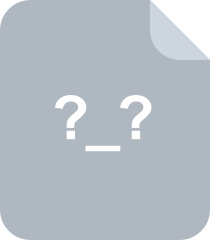
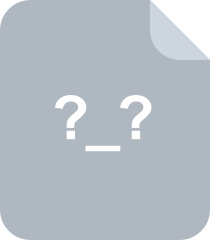
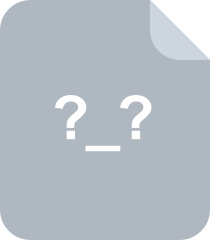
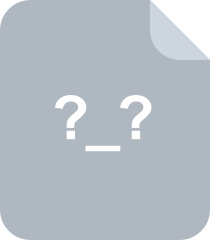
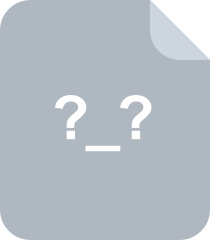
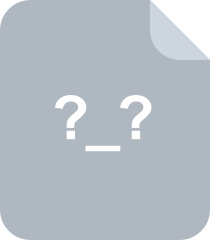
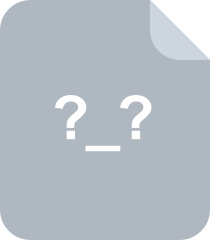
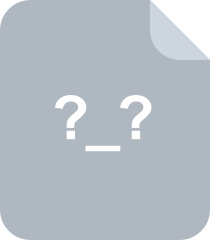
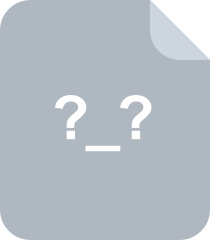
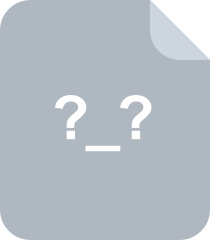
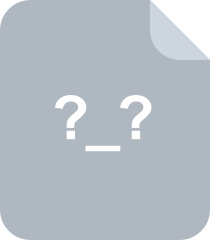
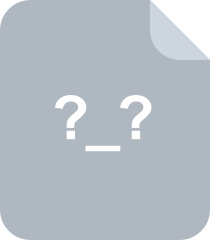
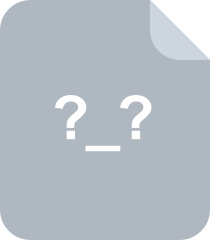
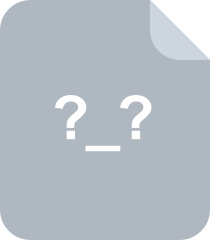
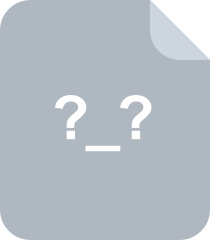
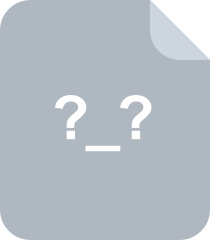
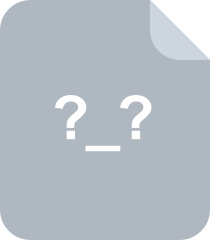
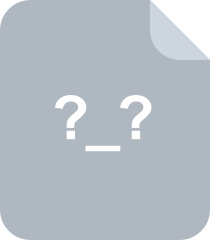
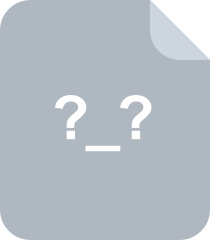
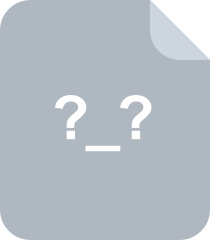
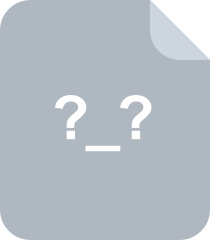
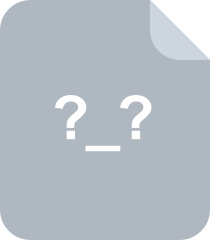
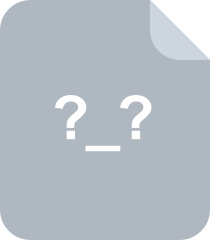
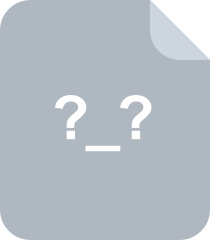
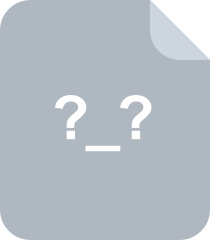
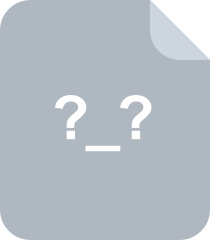
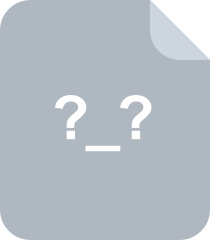
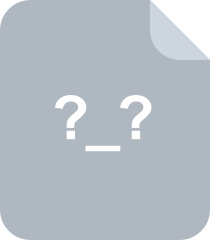
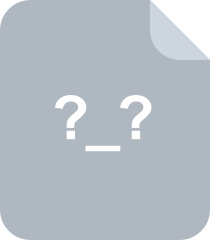
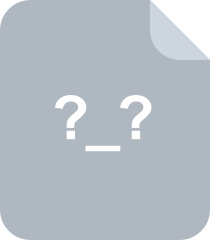
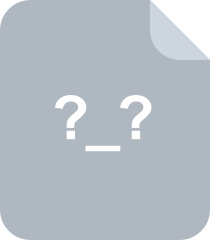
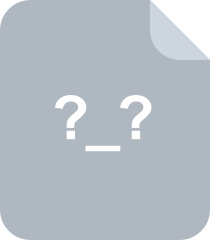
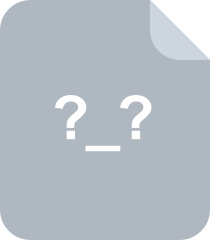
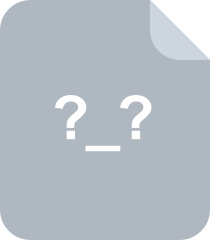
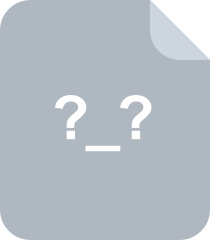
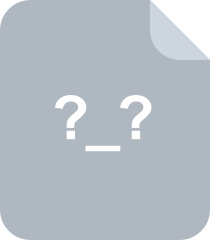
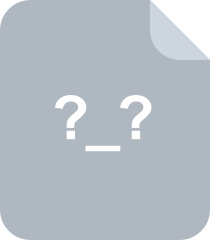
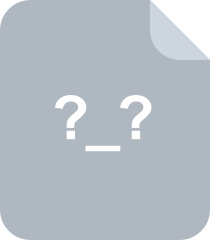
共 114 条
- 1
- 2
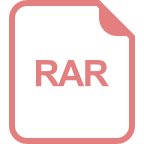
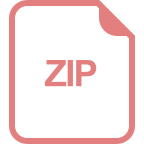
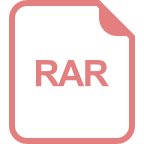
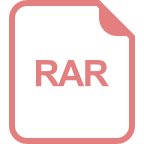
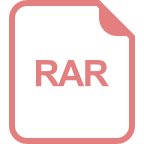
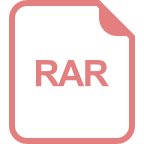
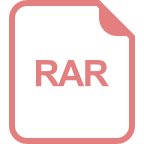
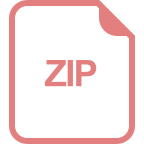
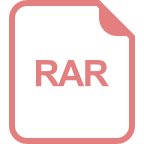
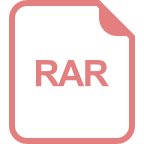

邓凌佳
- 粉丝: 65
- 资源: 1万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

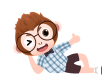
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1