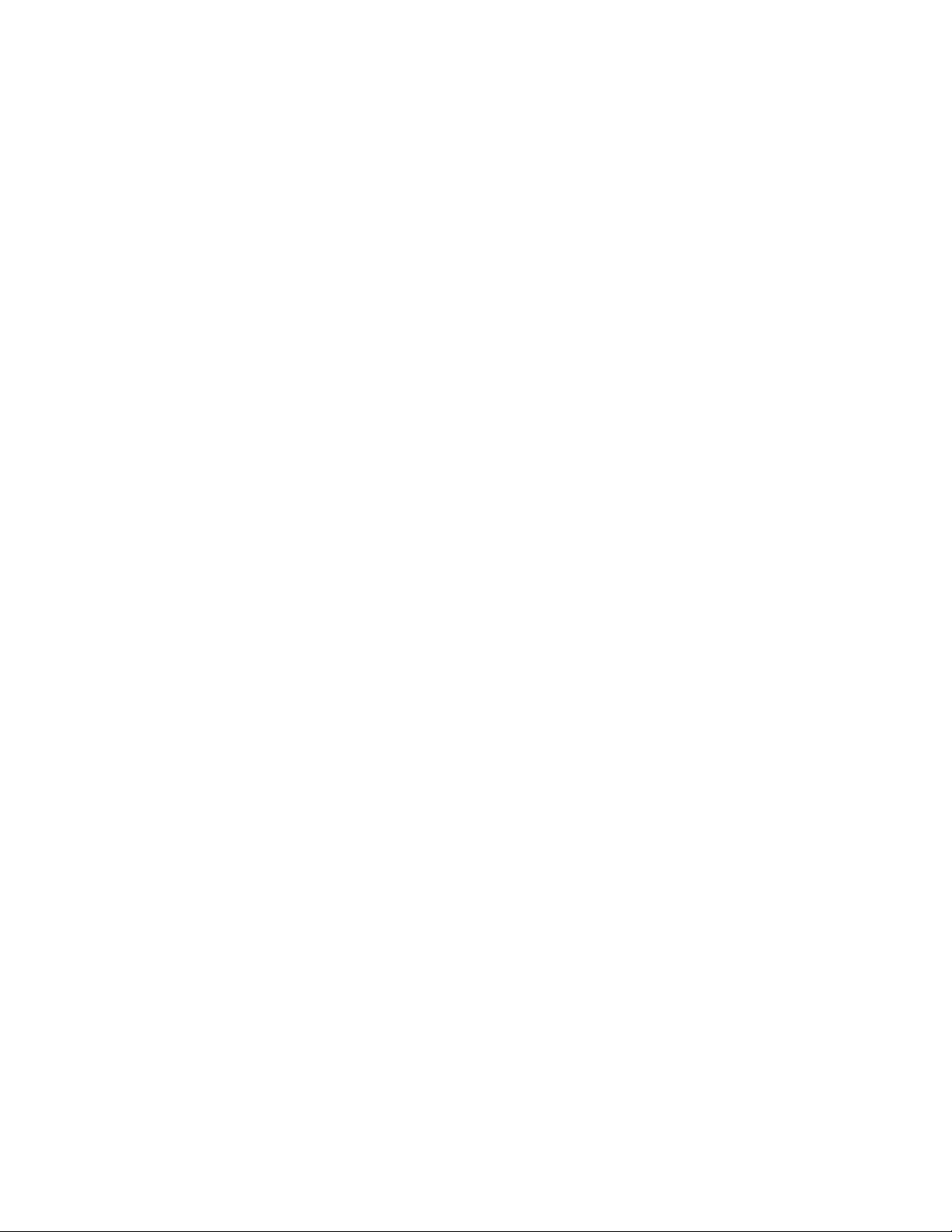
First we will create a class that handles all the operations required to deal with the
database such as creating the database, creating tables, inserting and deleting records and
so on.
The first step is to create a class that inherits from SQLiteOpenHelper class. this class
provides two methods to override to deal with the database:
1. onCreate(SQLiteDatabase db): invoked when the database is created, this is
where we can create tables and columns to them, create views or triggers.
2. onUpgrade(SQLiteDatabse db, int oldVersion, int newVersion): invoked
when we make a modification to the database such as altering, dropping , creating
new tables.
our class will have the following members
public class DatabaseHelper extends SQLiteOpenHelper {
static final String dbName="demoDB";
static final String employeeTable="Employees";
static final String colID="EmployeeID";
static final String colName="EmployeeName";
static final String colAge="Age";
static final String colDept="Dept";
static final String deptTable="Dept";
static final String colDeptID="DeptID";
static final String colDeptName="DeptName";
static final String viewEmps="ViewEmps";
The Constructor:
public DatabaseHelper(Context context) {
super(context, dbName, null,33);
}
The constructor of the super class has the following parameters:
Context con: the context attached to the database.
dataBaseName: the name of the database.
CursorFactory: some times we may use a class that extends the Cursor class to
implement some extra validations or operations on the queries run on the database. In this
case we pass an instance of CusrsorFactory to return a reference to our derived class to be
used instead of the default cursor,
In this example we are going to use the standard Cursor Interface to retrieve results, so
the CursorFactory parameter is going to be null.
Version: the version of the schema of the database.