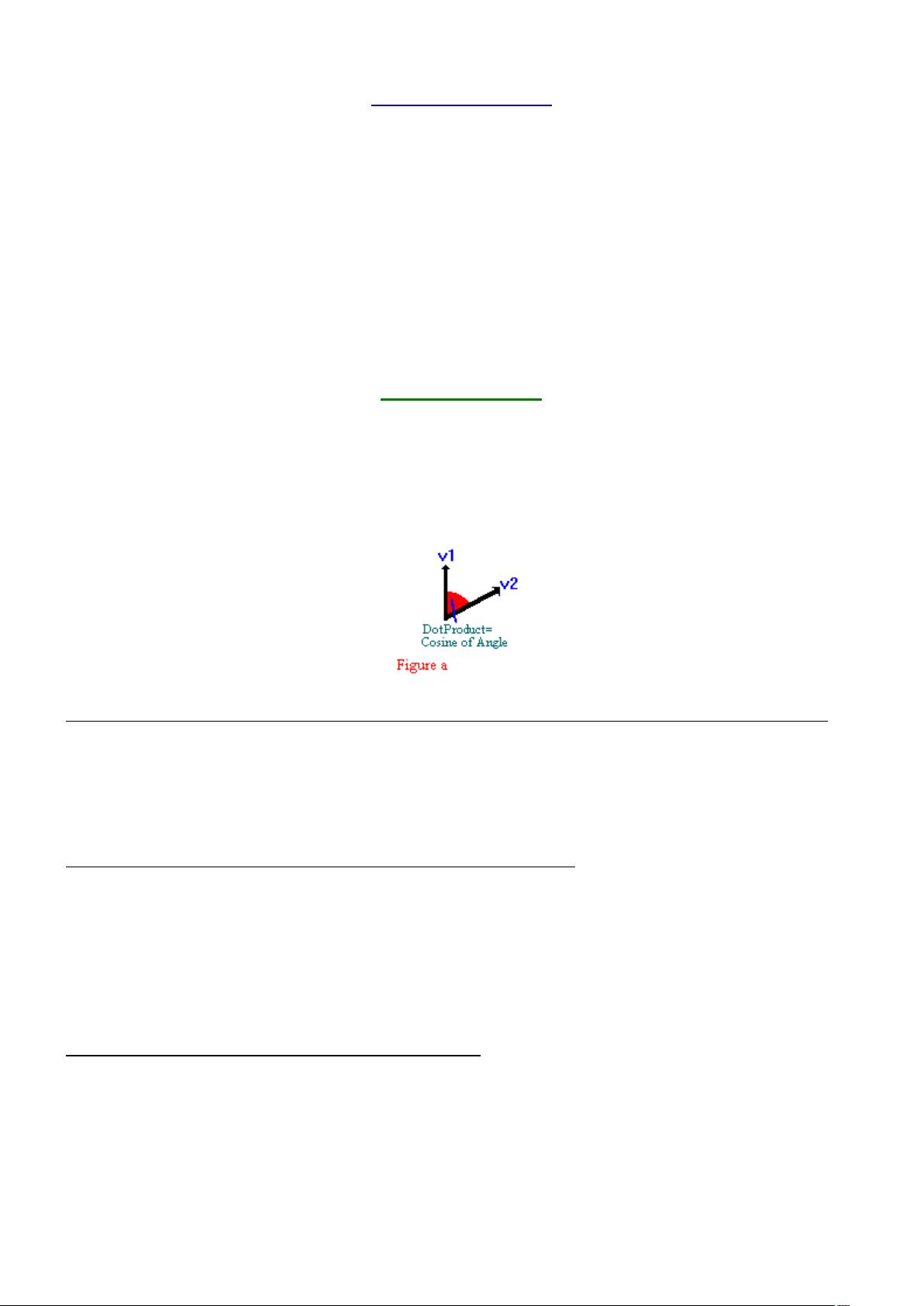
ogl_basic_collision.rar_visual c
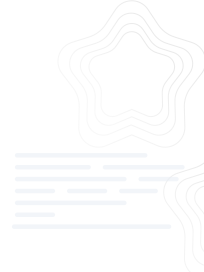

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
OpenGL基础碰撞检测——Visual C++ 实现 OpenGL是一种开放源代码的图形库,广泛用于创建2D和3D图形应用程序。在游戏开发、科学可视化等领域,碰撞检测是至关重要的功能,它能帮助我们识别和处理两个或多个物体之间的碰撞事件。在"ogl_basic_collision.rar_visual c"这个压缩包中,我们很显然会看到一个使用C++和OpenGL实现的基础碰撞检测的示例。 我们要理解碰撞检测的基本概念。碰撞检测分为两个主要部分:包围体(Bounding Volumes)和碰撞算法。包围体是物体的一种简化表示,通常是一个能够完全包围物体的几何形状,如球体、立方体或包围盒。碰撞算法则用于检查这些包围体之间是否发生交集,从而判断是否存在碰撞。 在OpenGL中,我们可以使用各种方法来实现碰撞检测。一种常见的方式是使用GLU库中的quadrics对象(如gluSphere)创建球体,并使用射线-球体碰撞检测算法。当用户与屏幕交互时,可以生成射线并检测该射线是否与场景中的球体相交。 Visual C++是微软提供的一个集成开发环境(IDE),支持C++编程语言,包括对OpenGL的支持。在Visual C++中编写OpenGL程序,通常需要包含适当的头文件(如<GL/glut.h>或<GLFW/glfw3.h>),设置OpenGL上下文,并在主循环中处理渲染和用户输入。 在"ogl_basic_collision"的示例中,我们可能可以看到以下关键步骤: 1. 初始化OpenGL上下文:这通常涉及设置窗口大小、颜色模式以及启用深度测试等。 2. 创建和设置包围体:为每个物体创建一个包围体,如球体或包围盒,并将其位置、尺寸等信息存储在数据结构中。 3. 定义碰撞检测函数:编写一个函数来处理射线-包围体的碰撞检测,通常会用到数学几何知识,如向量运算、距离计算等。 4. 用户输入处理:监听用户的鼠标或键盘输入,生成射线并调用碰撞检测函数。 5. 渲染场景:根据碰撞检测结果更新物体状态,例如改变颜色、位置等,然后绘制整个场景。 6. 主循环:不断地重绘场景,直到程序退出。 7. 错误处理和资源释放:确保在程序结束时正确地清理和释放分配的资源。 通过分析和学习"ogl_basic_collision.rar_visual c"中的代码,你可以深入理解如何在实际项目中集成和应用基础的碰撞检测技术,这对于任何使用OpenGL进行图形编程的人来说都是一个宝贵的起点。同时,这也是提升你的C++编程技巧和理解3D图形学的好机会。
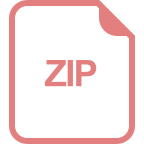
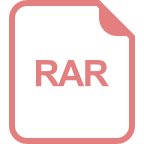
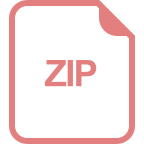
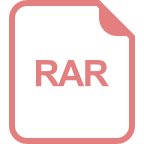
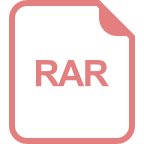
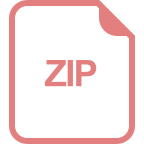
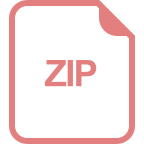
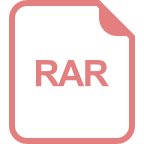
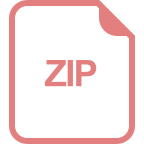
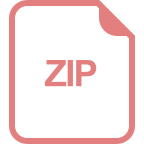
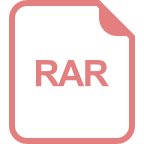
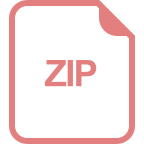
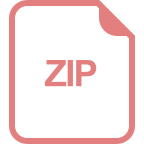
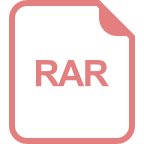


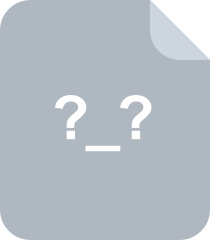
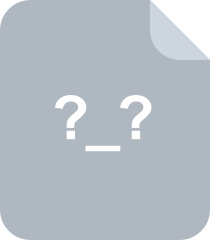
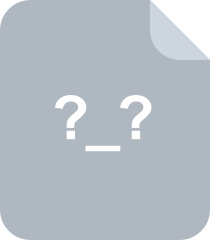
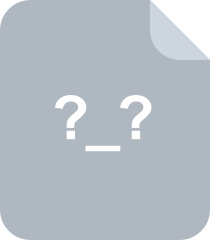
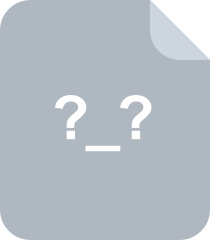
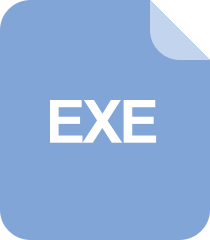
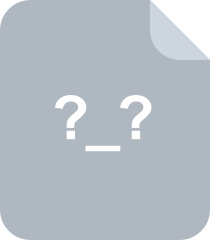
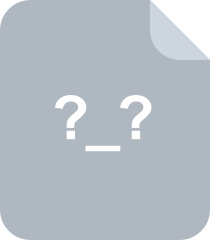
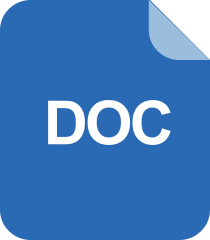
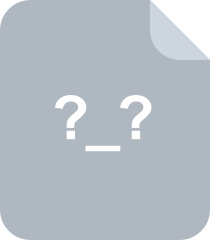
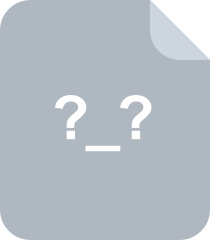
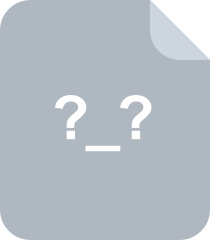

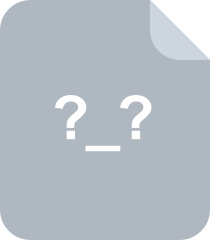
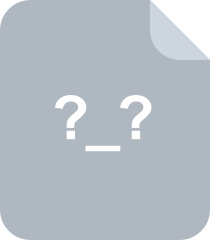
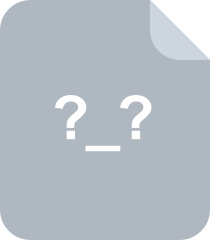
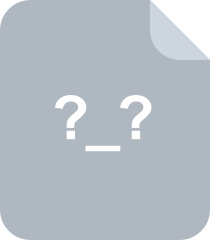
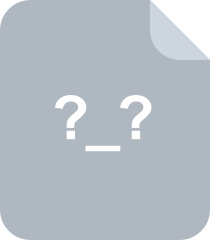
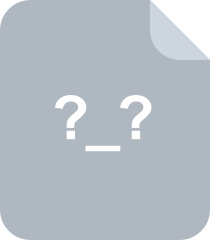
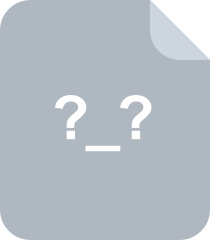
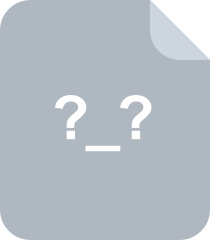
- 1
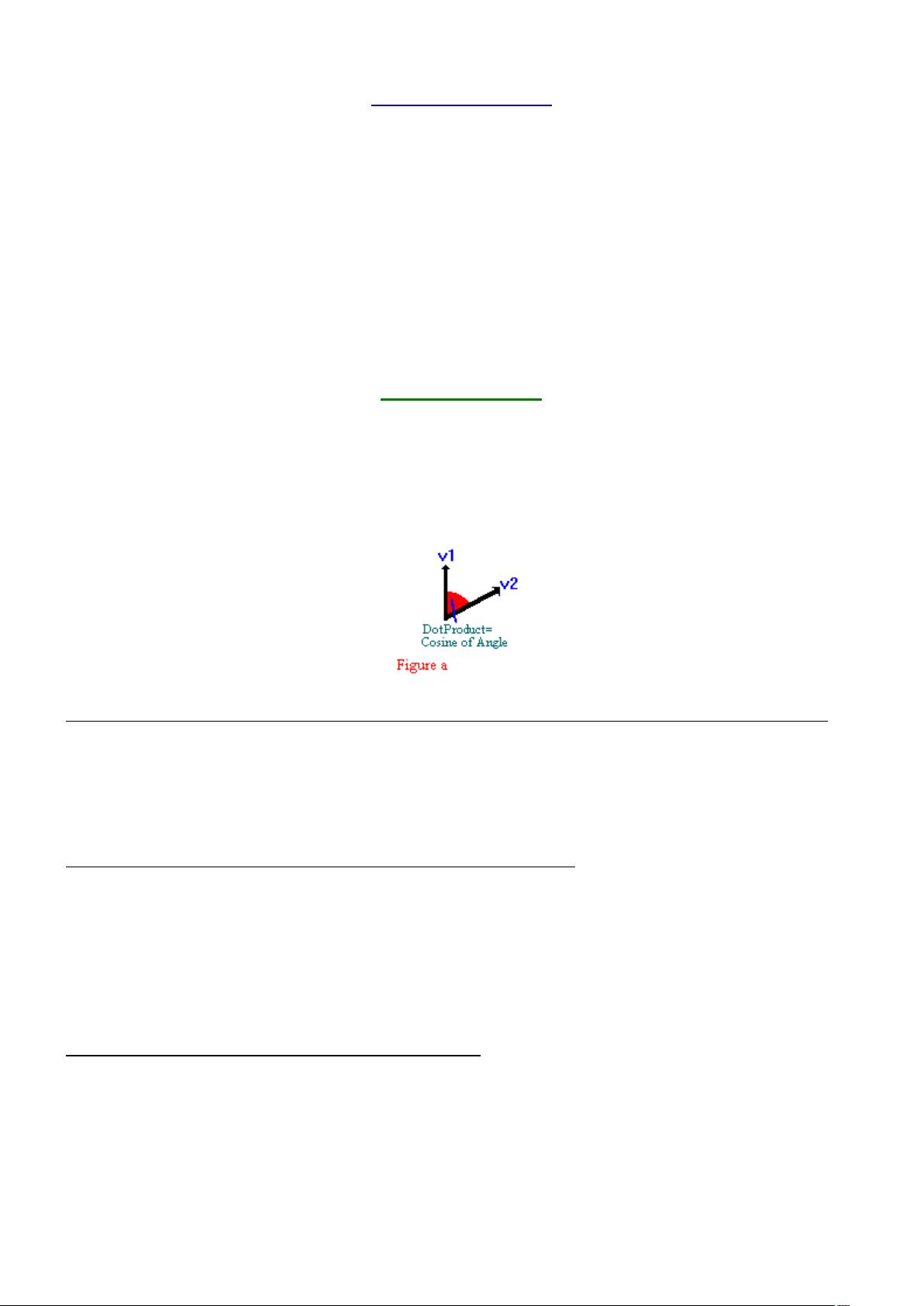
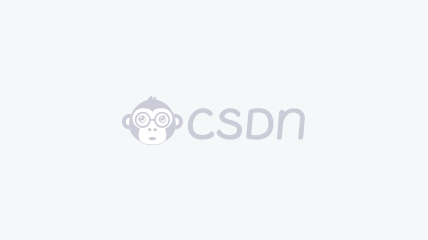

- 粉丝: 79
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

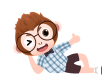
最新资源
- 基于51单片机开发板设计的六位密码锁
- course_s5_linux应用程序开发篇.pdf
- course_s4_ALINX_ZYNQ_MPSoC开发平台Linux驱动教程V1.04.pdf
- 核间ipcf示例,NXP的解决方案
- course_s0_Xilinx开发环境安装教程.pdf
- 多边形框架物体检测20-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- course_s1_ALINX_ZYNQ_MPSoC开发平台FPGA教程V1.01.pdf
- course_s3_ALINX_ZYNQ_MPSoC开发平台Linux基础教程V1.05.pdf
- rwer456456567567
- AXU2CGB-E开发板用户手册.pdf

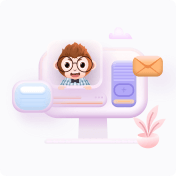
