/* Process model C form file: peg.pr.c */
/* Portions of this file copyright 1986-2008 by OPNET Technologies, Inc. */
/* This variable carries the header into the object file */
const char peg_pr_c [] = "MIL_3_Tfile_Hdr_ 145A 30A modeler 7 5851CC00 5851CC00 1 AHMED Ahmed@Ramadhan 0 0 none none 0 0 none 0 0 0 0 0 0 0 0 1e80 8 ";
#include <string.h>
/* OPNET system definitions */
#include <opnet.h>
/* Header Block */
#include <nato.h>
#include <oms_pr.h>
#include <oms_tan.h>
#include <ip_addr_v4.h>
#include <ip_rte_v4.h>
#include <ip_dgram_sup.h>
#include <tcp_seg_sup.h>
// added by Renju to include the
#include <oms_dt.h>
#include <tcp_v3.h>
/* Function Return Constants */
#define SN_FUNC_SUCCESS 0
#define SN_FUNC_FAILED 1
#define SN_FUNC_CACHE_FULL 10
/* Define a transition condition corresponding */
/* to the IP datagram arrival */
#define HOST_PKT_ARRIVAL (intrpt_type == OPC_INTRPT_STRM && intrpt_strm == 2)
#define MOB_PKT_ARRIVAL (intrpt_type == OPC_INTRPT_STRM && intrpt_strm == 1)
/* Function return code */
#define PEG_FUNC_SUCCESS 0
#define PEG_FUNC_FAILED 1
/* TCP Information Structure */
typedef struct
{
unsigned int src_ip;
unsigned int dest_ip;
int src_port;
int dest_port;
unsigned int seq_num;
unsigned int ack_num;
unsigned int rcv_win;
int urgent_pointer;
int data_len;
//int urg;
//int ack;
//int push;
//int rst;
//int syn;
//int fin;
// added by Renju for opnet ver 9.1.A
TcpT_Flag flags;
/* these are the possible values for the flags:
#define TCPC_FLAG_NONE 0x00
#define TCPC_FLAG_FIN 0x01
#define TCPC_FLAG_SYN 0x02
#define TCPC_FLAG_RST 0x04
#define TCPC_FLAG_PSH 0x08
#define TCPC_FLAG_ACK 0x10
#define TCPC_FLAG_URG 0x20*/
OmsT_Dt_Key local_key;
OmsT_Dt_Key remote_key;
} TcpInfo;
int pegGetTcpInfo (Packet *pkp, TcpInfo *tcp_infop);
/* End of Header Block */
#if !defined (VOSD_NO_FIN)
#undef BIN
#undef BOUT
#define BIN FIN_LOCAL_FIELD(_op_last_line_passed) = __LINE__ - _op_block_origin;
#define BOUT BIN
#define BINIT FIN_LOCAL_FIELD(_op_last_line_passed) = 0; _op_block_origin = __LINE__;
#else
#define BINIT
#endif /* #if !defined (VOSD_NO_FIN) */
/* State variable definitions */
typedef struct
{
/* Internal state tracking for FSM */
FSM_SYS_STATE
/* State Variables */
Ici* send_iciptr ;
int send_total_pkcnt ;
int recv_total_pkcnt ;
int send_drop_pkcnt ;
int recv_drop_pkcnt ;
Stathandle send_total_pkcnt_stathandle ;
Stathandle send_drop_pkcnt_stathandle ;
Stathandle recv_total_pkcnt_stathandle ;
Stathandle recv_drop_pkcnt_stathandle ;
Ici* recv_iciptr ;
int send_drop_rate ;
int recv_drop_rate ;
} peg_state;
#define send_iciptr op_sv_ptr->send_iciptr
#define send_total_pkcnt op_sv_ptr->send_total_pkcnt
#define recv_total_pkcnt op_sv_ptr->recv_total_pkcnt
#define send_drop_pkcnt op_sv_ptr->send_drop_pkcnt
#define recv_drop_pkcnt op_sv_ptr->recv_drop_pkcnt
#define send_total_pkcnt_stathandle op_sv_ptr->send_total_pkcnt_stathandle
#define send_drop_pkcnt_stathandle op_sv_ptr->send_drop_pkcnt_stathandle
#define recv_total_pkcnt_stathandle op_sv_ptr->recv_total_pkcnt_stathandle
#define recv_drop_pkcnt_stathandle op_sv_ptr->recv_drop_pkcnt_stathandle
#define recv_iciptr op_sv_ptr->recv_iciptr
#define send_drop_rate op_sv_ptr->send_drop_rate
#define recv_drop_rate op_sv_ptr->recv_drop_rate
/* These macro definitions will define a local variable called */
/* "op_sv_ptr" in each function containing a FIN statement. */
/* This variable points to the state variable data structure, */
/* and can be used from a C debugger to display their values. */
#undef FIN_PREAMBLE_DEC
#undef FIN_PREAMBLE_CODE
#define FIN_PREAMBLE_DEC peg_state *op_sv_ptr;
#define FIN_PREAMBLE_CODE \
op_sv_ptr = ((peg_state *)(OP_SIM_CONTEXT_PTR->_op_mod_state_ptr));
/* Function Block */
#if !defined (VOSD_NO_FIN)
enum { _op_block_origin = __LINE__ + 2};
#endif
int pegGetTcpInfo (Packet *pkp, TcpInfo *tcp_infop)
{
Packet *encap_tcp_pktp;
TcpT_Seg_Fields *tcp_fdp;
IpT_Dgram_Fields *ip_fdp;
op_pk_nfd_access (pkp, "fields", &ip_fdp);
//tcp_infop->src_ip = ip_fdp->src_addr;
//tcp_infop->dest_ip = ip_fdp->dest_addr;
tcp_infop->src_ip = ip_fdp->src_addr.address.ipv4_addr;
tcp_infop->dest_ip = ip_fdp->dest_addr.address.ipv4_addr;
/* Check if the packet is TCP packet */
/* Get TCP Packet contained inside the IP packet */
if (op_pk_nfd_get (pkp, "data", &encap_tcp_pktp) == OPC_COMPCODE_FAILURE)
{
printf ("Unable to extract TCP packet from ip");
return PEG_FUNC_FAILED;
}
else
{
/* Extract Tcp Info */
op_pk_nfd_access (encap_tcp_pktp, "fields", &tcp_fdp);
tcp_infop->src_port = tcp_fdp->src_port;
tcp_infop->dest_port = tcp_fdp->dest_port;
tcp_infop->seq_num = tcp_fdp->seq_num;
tcp_infop->ack_num = tcp_fdp->ack_num;
tcp_infop->rcv_win = tcp_fdp->rcv_win;
tcp_infop->urgent_pointer = tcp_fdp->urgent_pointer;
tcp_infop->data_len = tcp_fdp->data_len;
/*tcp_infop->urg = tcp_fdp->urg;
tcp_infop->ack = tcp_fdp->ack;
tcp_infop->push = tcp_fdp->push;
tcp_infop->rst = tcp_fdp->rst;
tcp_infop->syn = tcp_fdp->syn;
tcp_infop->fin = tcp_fdp->fin;*/
// added by Renju as per the changes in the TCP segment in OPnet
//in 9.1.A
tcp_infop->flags = tcp_fdp->flags;
tcp_infop->local_key = tcp_fdp->local_key;
tcp_infop->remote_key = tcp_fdp->remote_key;
op_pk_nfd_set (pkp, "data", encap_tcp_pktp);
return PEG_FUNC_SUCCESS;
}
}
/* End of Function Block */
/* Undefine optional tracing in FIN/FOUT/FRET */
/* The FSM has its own tracing code and the other */
/* functions should not have any tracing. */
#undef FIN_TRACING
#define FIN_TRACING
#undef FOUTRET_TRACING
#define FOUTRET_TRACING
#if defined (__cplusplus)
extern "C" {
#endif
void peg (OP_SIM_CONTEXT_ARG_OPT);
VosT_Obtype _op_peg_init (int * init_block_ptr);
void _op_peg_diag (OP_SIM_CONTEXT_ARG_OPT);
void _op_peg_terminate (OP_SIM_CONTEXT_ARG_OPT);
VosT_Address _op_peg_alloc (VosT_Obtype, int);
void _op_peg_svar (void *, const char *, void **);
#if defined (__cplusplus)
} /* end of 'extern "C"' */
#endif
/* Process model interrupt handling procedure */
void
peg (OP_SIM_CONT
peg.pr.rar_packet generator
版权申诉
149 浏览量
2022-09-14
23:10:18
上传
评论
收藏 4KB RAR 举报

邓凌佳
- 粉丝: 65
- 资源: 1万+
最新资源
- Code for the complete guide to tkinter tutorial
- 关于百货中心供应链管理系统.zip
- SimpleFolderIcon-master 修改Unity的Project下的文件夹图标
- A python Tkinter widget to display tile based maps
- A pure Python library for adding tables to a Tkinter application
- Vector资源文件.zip
- MobaXterm-Installer
- MicroMsg.xlsx
- 88-520告白(520气球).zip
- HTML+CSS+JS精品网页模板H126.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


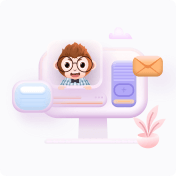