#include <windows.h>
#include <math.h>
#include "ca.h"
#include"resource.h"
void Number(int n)
{
if (dot == false) {
first = first * 10 + n;
}
else {
dotnum++;
first = first + n / pow(10, dotnum);
}
_gcvt(first,10,output);
SetWindowText(Editbox,output);
}
void dotB()
{
if (!dot) {
dot = true;
}
}
void Add()
{
cmd = '+';
last = first;
first=0;
dot=false;
dotnum=0;
}
void Sub()
{
cmd = '-';
last = first;
first=0;
dot=false;
dotnum=0;
}
void Mul()
{
cmd = '*';
last = first;
first=0;
dot=false;
dotnum=0;
}
void Div()
{
cmd = '/';
last = first;
first=0;
dot=false;
dotnum=0;
}
void Sin()
{
first=sin(first);
_gcvt(first,10,output);
SetWindowText(Editbox,output);
}
void Equ()
{
switch (cmd) {
case '+':
first += last;
break;
case '-':
first = last-first;
break;
case '*':
first *= last;
break;
case '/':
first = last/first;
break;
}
dot = false;
_gcvt(first,10,output);
SetWindowText(Editbox,output);
}
void Clear()
{
first = 0;
last= 0;
dot=false;
dotnum=0;
SetWindowText(Editbox,"");
}
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance,
HINSTANCE hPrevInst,
LPSTR lpszCmdLine,
int nCmdShow)
{
HWND hwnd;
MSG Msg;
WNDCLASS wndclass;
char lpszClassName[] = "Calculator";
char lpszTitle[] = "Calculator";
wndclass.style = 0;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(hInstance, MAKEINTRESOURCE(IDI_ICON1));
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(BLACK_BRUSH);
wndclass.lpszClassName = lpszClassName;
wndclass.lpszMenuName = NULL;
if (!RegisterClass(&wndclass)) {
MessageBeep(0);
return false;
}
hwnd = CreateWindow(
lpszClassName,
lpszTitle,
WS_OVERLAPPEDWINDOW,
200, 200, 220, 280,
NULL, NULL,
hInstance,
NULL
);
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&Msg, NULL, 0, 0)) {
TranslateMessage(&Msg);
DispatchMessage(&Msg);
}
return Msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hWnd,
UINT Message,
WPARAM wParam,
LPARAM lParam)
{
HWND Button[22];
HWND hclear;
int i;
HINSTANCE hInst = GetModuleHandle(NULL);
switch(Message) {
case WM_CREATE:
for ( i = 1; i <= 20; i++) {
Button[i] = CreateWindow(
"BUTTON",
Caption[i-1],
WS_CHILD | WS_VISIBLE,
pos_x[i-1], pos_y[i-1],
35, //long
35, //height
hWnd,
(HMENU)ID[i-1],
hInst,
NULL);
}
Editbox=CreateWindow(
"Edit",
NULL,
WS_CHILD|WS_VISIBLE,
10,5,
195,25,
hWnd,
(HMENU)1000,
hInst,
NULL);
hclear=CreateWindow(
"BUTTON",
"CLEAR",
WS_CHILD|WS_VISIBLE,
10,200,
195,30,
hWnd,
(HMENU)100,
hInst,
NULL);
break;
case WM_COMMAND:
switch (wParam) {
case 0:
Number(0);
break;
case 1:
Number(1);
break;
case 2:
Number(2);
break;
case 3:
Number(3);
break;
case 4:
Number(4);
break;
case 5:
Number(5);
break;
case 6:
Number(6);
break;
case 7:
Number(7);
break;
case 8:
Number(8);
break;
case 9:
Number(9);
break;
case 10:
Add();
break;
case 11:
Sub();
break;
case 12:
Mul();
break;
case 13:
Div();
break;
case 15:
Equ();
break;
case 14:
dotB();
break;
case 100:
Clear();
break;
case 16:
Sin();
break;
}
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hWnd, Message, wParam, lParam);
}
return 0;
}
caishuzi.rar_VC 游戏_猜数字游戏
版权申诉
70 浏览量
2022-09-24
12:22:17
上传
评论 1
收藏 16KB RAR 举报

四散
- 粉丝: 52
- 资源: 1万+
最新资源
- ISOSAE21434.D1-2020SAE美国汽车标准
- 奥比中光RGBD在JETSON ORIN NX的ROS程序
- SerialNumberUtil.java
- autojspro写的木鱼小软件,模拟木鱼的敲击声,提供源代码
- 修改windows服务器远程桌面端口批处理
- 黑马Java八股文面试题视频教程,Java面试八股文宝典(含阿里、腾迅大厂java面试真题,java数据结构,java并发
- java调用科大讯飞在线语音合成API -完整代码
- Python爬虫基础知识.zip
- Java八股文和面试项目介绍-春招秋招校招社招
- 其他类别JSP网页HTML编辑器 v1.0 beat-jsphtmleditor.7z
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


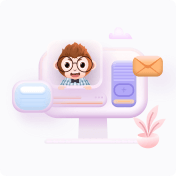