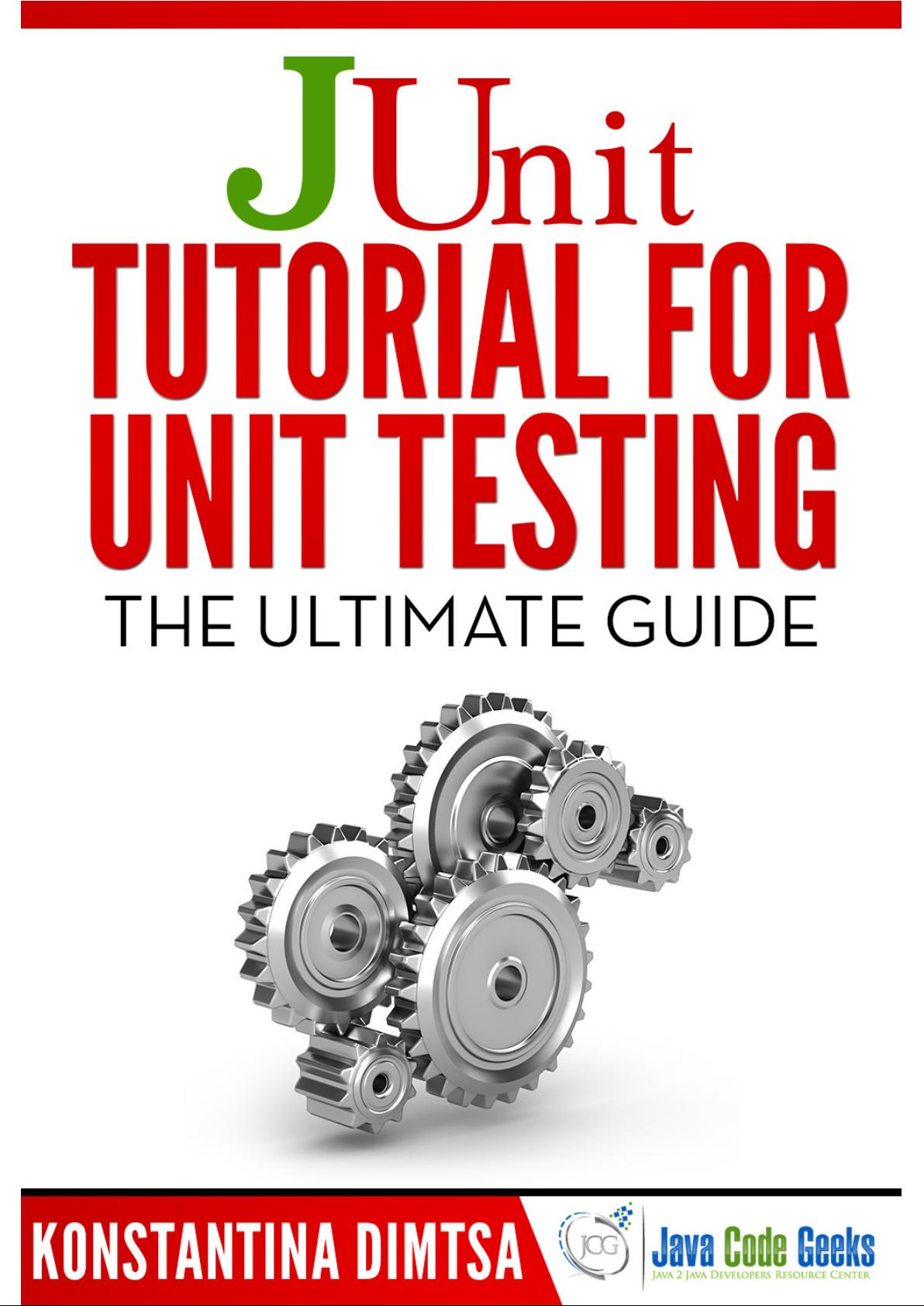
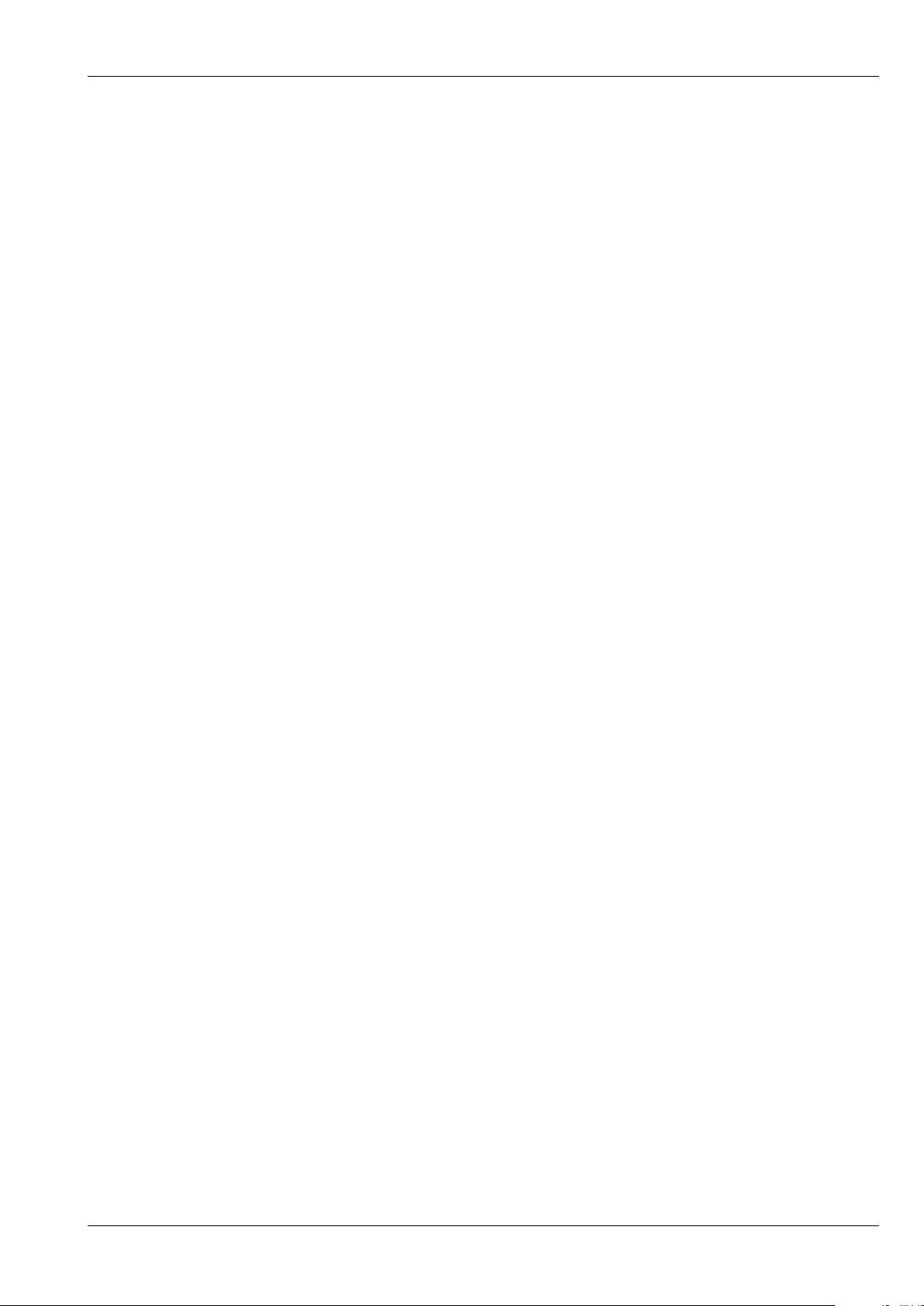
JUnit Tutorial i
JUnit Tutorial
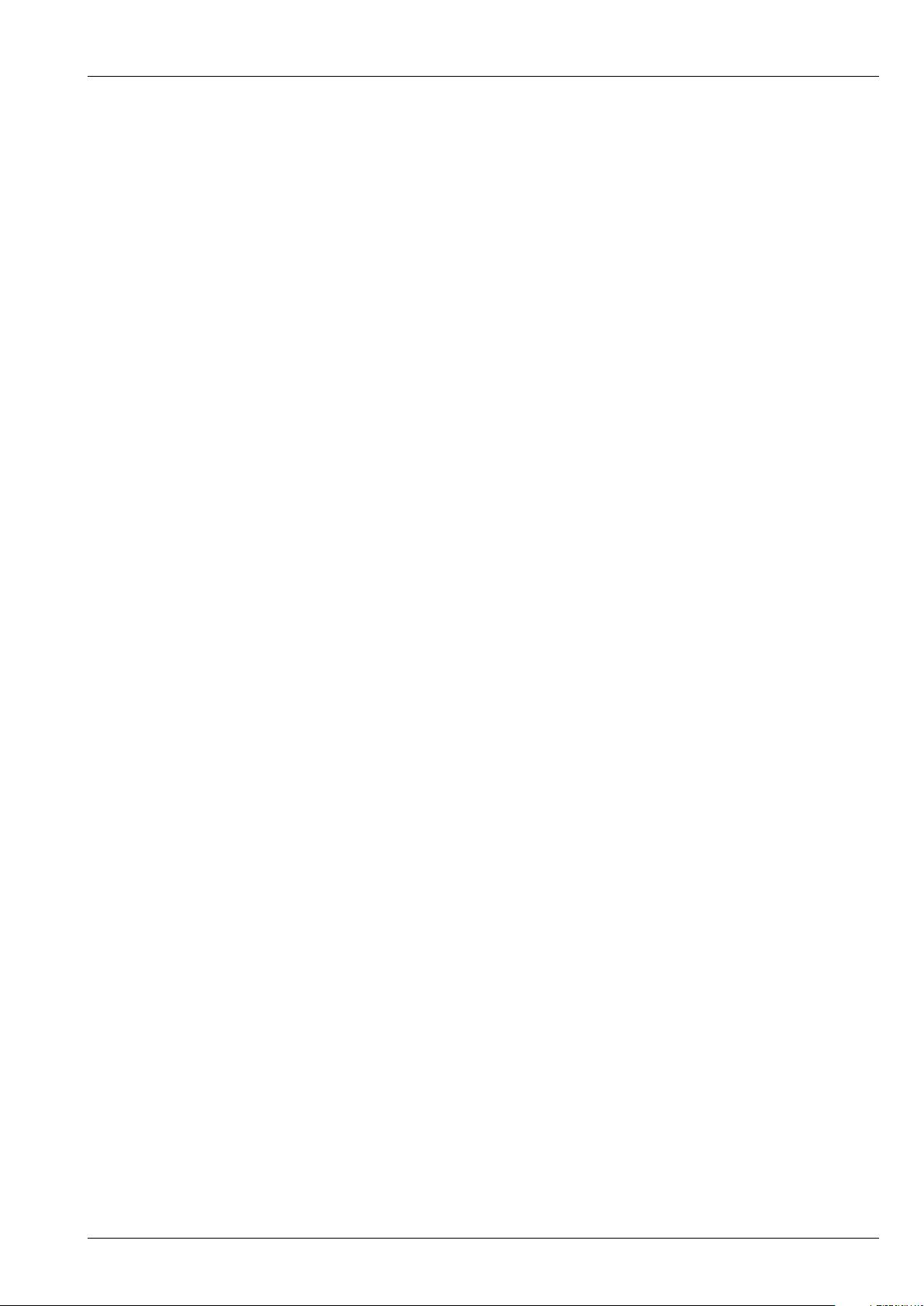
JUnit Tutorial ii
Contents
1 Unit testing introduction 1
1.1 What is unit testing? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
1.2 Test coverage . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
1.3 Unit testing in Java . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
2 JUnit introduction 2
2.1 JUnit Simple Example using Eclipse . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
2.2 JUnit annotations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
2.3 JUnit assertions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
3 JUnit complete example using Eclipse 9
3.1 Initial Steps . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
3.2 Create the java class to be tested . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
3.3 Create and run a JUnit test case . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
3.4 Using @Ignore annotation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17
3.5 Creating suite tests . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
3.6 Creating parameterized tests . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20
3.7 Rules . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
3.8 Categories . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
4 Run JUnit tests from command line 24
5 Conclusions 26
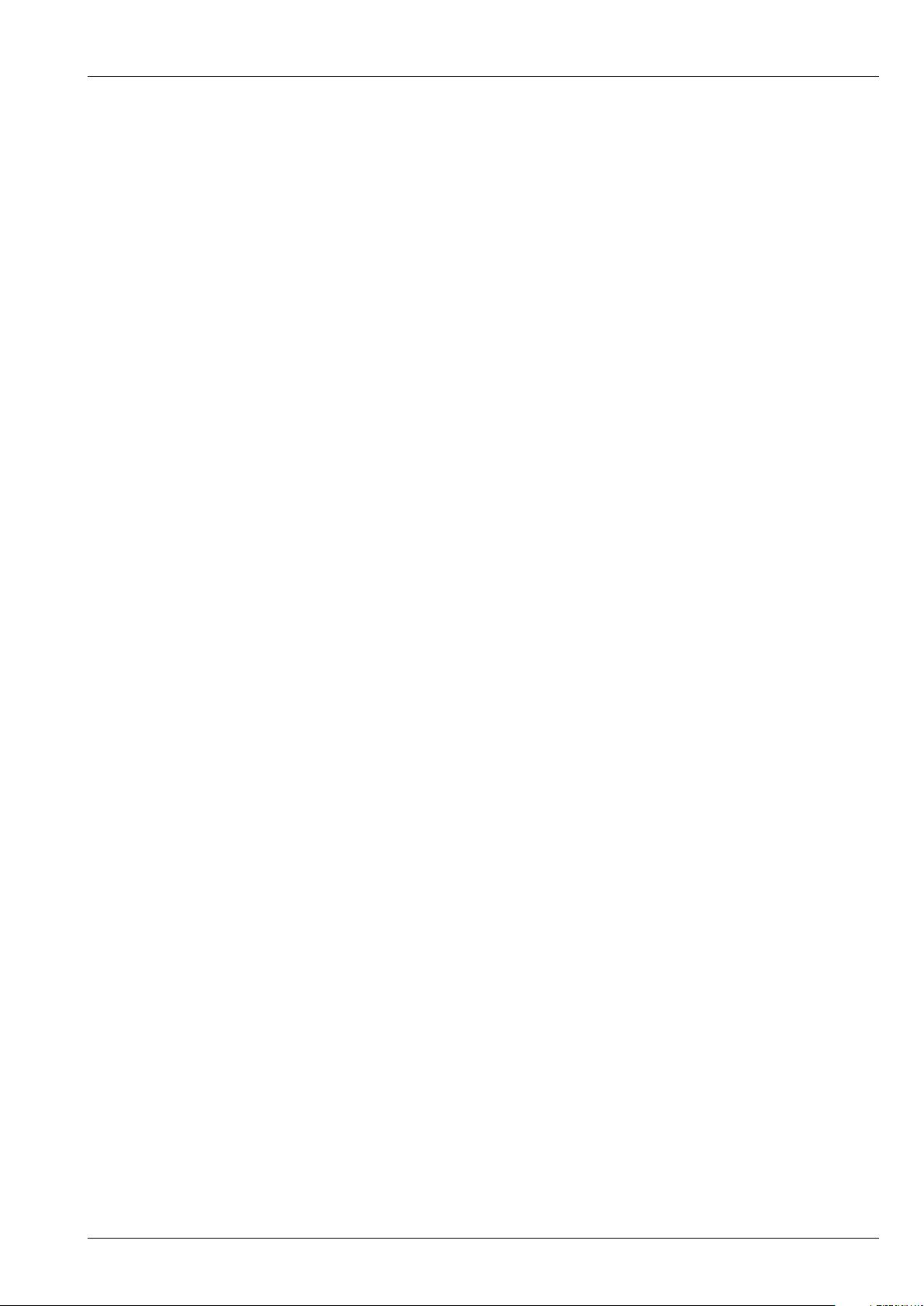
JUnit Tutorial iii
Copyright (c) Exelixis Media P.C., 2014
All rights reserved. Without limiting the rights under
copyright reserved above, no part of this publication
may be reproduced, stored or introduced into a retrieval system, or
transmitted, in any form or by any means (electronic, mechanical,
photocopying, recording or otherwise), without the prior written
permission of the copyright owner.
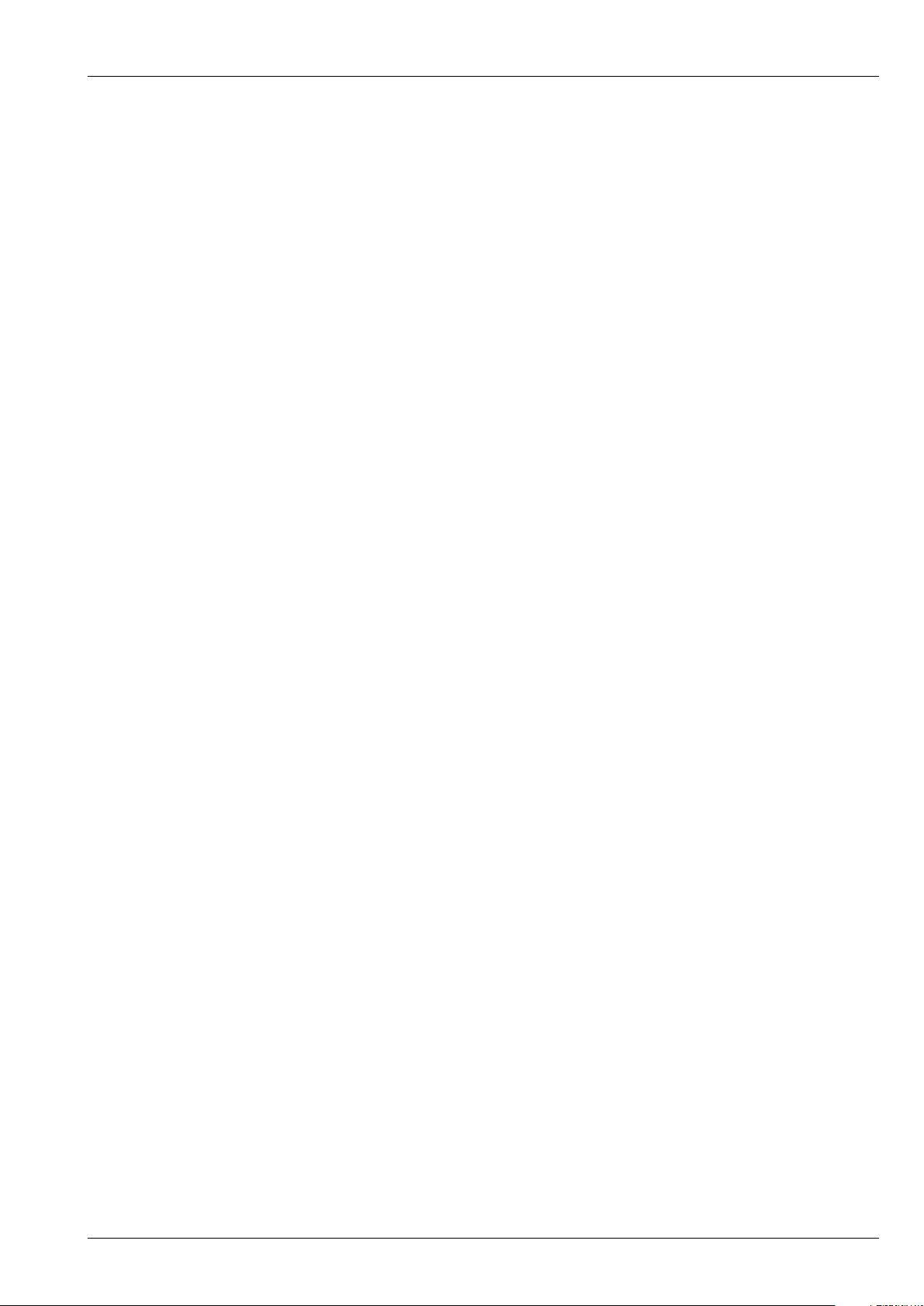
JUnit Tutorial iv
Preface
We have provided plenty of JUnit [1] tutorials here at Java Code Geeks, like JUnit Getting Started Example [2], JUnit Using
Assertions and Annotations Example [3], JUnit Annotations Example [4] and so on.
However, we prefered to gather all the JUnit features in one detailed guide for the convenience of the reader.
We hope you like it!
References:
[1] http://junit.org/
[2] http://examples.javacodegeeks.com/core-java/junit/junit-getting-started-example/
[3] http://examples.javacodegeeks.com/core-java/junit/junit-using-assertions-and-annotations-example/
[4] http://examples.javacodegeeks.com/core-java/junit/junit-annotations-example/