/*
tsc.c
Assembly-level routines to read the processor timestamp counter.
*/
#include <stdio.h>
#include "tsc.h"
int tsc_valid( void ) {
int is_valid = 0;
/* presumably Visual C++ specific */
#ifdef _MSC_VER
/* the "ID" bit on CFLAGS */
#define ID_BIT 21
/* the "supports processor counter" bitmask */
#define TSC_BITMASK 0x10
__asm {
/* alright, first we should check for the CPUID instruction */
pushfd /* put EFLAGS on the stack */
mov eax, [esp] /* copy over to eax */
btc [esp], ID_BIT /* flip the bit on the stack */
popfd /* put the stack back in EFLAGS */
pushfd /* and take it out again */
test eax, [esp] /* test for similarity */
jz done /* identical: flip didn't take */
mov eax, [esp] /* copy over to eax */
btc [esp], ID_BIT /* flip the bit again */
popfd /* put the stack back in EFLAGS */
pushfd /* and take it out again again */
test eax, [esp] /* test for similarity */
jz done /* identical: flip didn't take */
mov eax, 0x1 /* the code for what we want */
cpuid /* get it */
and edx, TSC_BITMASK /* check only the bit we want */
jz done /* zero if it wasn't set, i.e. no capability */
mov is_valid, 1 /* set is_valid */
jmp done /* goto done */
done:
add esp, 4 /* clean up the stack */
}
/* no other cases: note that we "cleverly" compile to constant-zero */
#endif
return is_valid;
}
tsc_t tsc_get( void ) {
/*
union {
UINT64_T whole;
struct {
UINT32_T high;
UINT32_T low;
} split;
} store;
*/
#ifdef _MSC_VER
__asm {
rdtsc
/* This nicely puts our returned value in storage, from which
we can conveniently return. Note that standard calling
conventions have us returning 64 bit quantities in eax and
edx anyway, so we might as well skip the middle step.
mov store.split.high, eax
mov store.split.low, edx
*/
}
#endif
/* fprintf( stderr, "dbg_ts: got %lx, %lx: %I64x\n", store.split.high,
store.split.low, store.whole ); */
/* return store.whole; */
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Timestamp程序会创建用户指定数量的线程,每一个线程都将执行一个简单的for循环。每次循环都会读取时钟计数器的值并将其保存到一个数组中。每个线程将这些值保存到各自的数组中。 通过观察timestamp的值,程序可以找出当前线程被执行的时刻。如果一个线程在执行,那就意味着它在执行时,每次循环保存的结果都是连续的值,而且相邻值的差距很小。因为处理器完成一次循环不会花费很长的时间。 我们认为,如果相邻值的差距在1到20个时钟周期那是一次普通的循环。如果差距很大,比如20000000个时钟周期就意味着在那段时间,该线程并没有被执行。 程序循环把从时钟计数器读取到的值填充到一个指定大小的数组。循环完成之后会生成一个报告,通过分析数组里面相邻数据的差距,如果差距超过某一个极限值则被认为线程被移出了CPU,报告包含了线程在什么时候被移出CPU的记录。 这个极限值默认被设置为一次循环所需要的最小的时钟周期数。数组里面任何一次相邻值差距超过这个极限值则被视作一次中断。
资源推荐
资源详情
资源评论
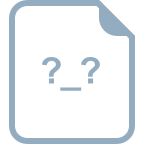
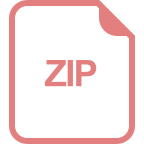
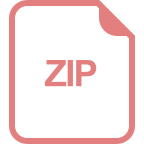
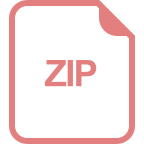
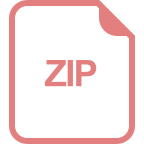
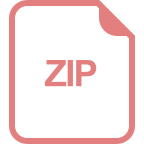
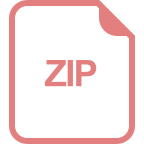
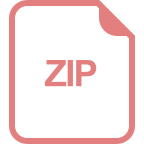
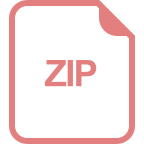
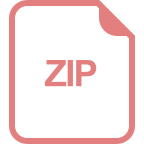
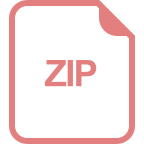
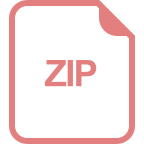
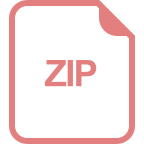
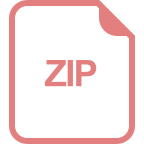
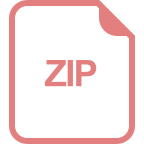
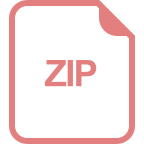
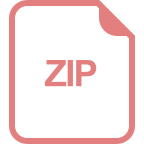
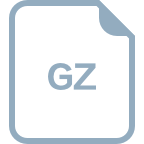
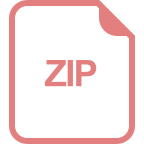
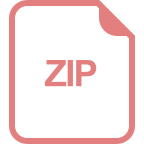
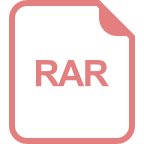
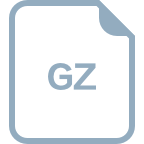
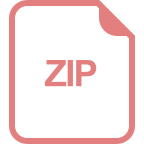
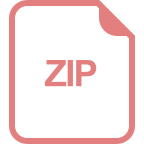
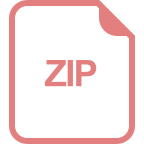
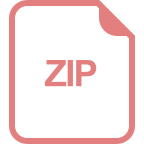
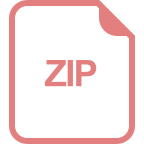
收起资源包目录

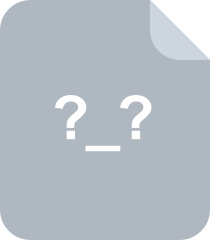
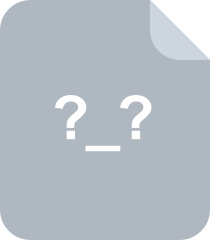
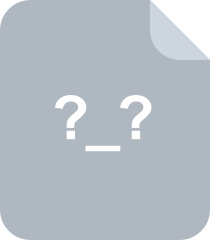
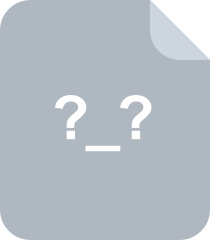
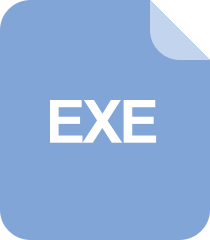
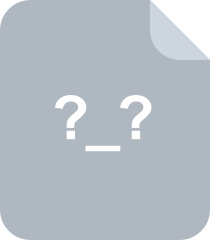
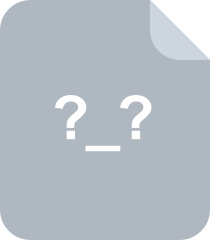
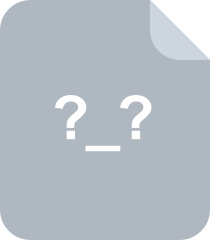
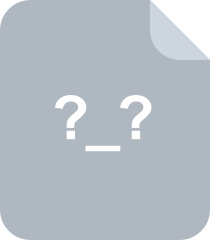
共 9 条
- 1
资源评论
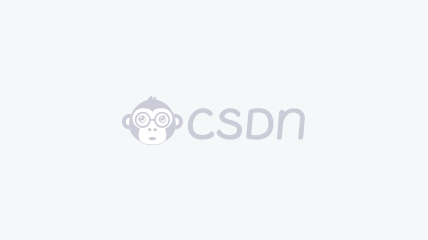

朱moyimi
- 粉丝: 75
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

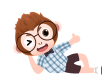
最新资源
- asp.net 原生js代码及HTML实现多文件分片上传功能(自定义上传文件大小、文件上传类型)
- whl@pip install pyaudio ERROR: Failed building wheel for pyaudio
- Constantsfd密钥和权限集合.kt
- 基于Java的财务报销管理系统后端开发源码
- 基于Python核心技术的cola项目设计源码介绍
- 基于Python及多语言集成的TSDT软件过程改进设计源码
- 基于Java语言的歌唱比赛评分系统设计源码
- 基于JavaEE技术的课程项目答辩源码设计——杨晔萌、李知林、岳圣杰、张俊范小组作品
- 基于Java原生安卓开发的蔚蓝档案娱乐应用设计源码
- 基于Java、Vue、JavaScript、CSS、HTML的毕设设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


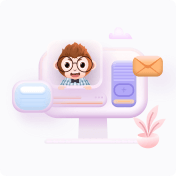
安全验证
文档复制为VIP权益,开通VIP直接复制
