//*----------------------------------------------------------------------------
//* ATMEL Microcontroller Software Support - ROUSSET -
//*----------------------------------------------------------------------------
//* The software is delivered "AS IS" without warranty or condition of any
//* kind, either express, implied or statutory. This includes without
//* limitation any warranty or condition with respect to merchantability or
//* fitness for any particular purpose, or against the infringements of
//* intellectual property rights of others.
//*----------------------------------------------------------------------------
//* File Name : main.c
//* Object : main application written in C
//* 1.0 24/Jun/04 JPP : Creation
//* 1.1 21/Feb/05 JPP : Update AT91C_RSTC_URSTEN
//* 1.2 29/Aug/05 JPP : Update AIC definion
//*----------------------------------------------------------------------------
// Include Standard LIB files
#include "Board.h"
//* Waiting time between LED1 and LED2
#define WAIT_TIME MCK
#define PIO_INTERRUPT_LEVEL 6
#define IRQ0_INTERRUPT_LEVEL 2
#define SOFT_INTERRUPT_LEVEL 5
#define FIQ_INTERRUPT_LEVEL 0
//* Global variable
int count_timer0_interrupt;
int count_timer1_interrupt;
// Use the Library Handler defined in file periph/pio/pio_irq/irq_pio.s
extern void FIQ_init_handler(void);
extern void at91_IRQ0_handler(void);
// External Function Prototype
extern void timer_init (void );
extern void Usart_init (void);
//*----------------------------------------------------------------------------
//* Function Name : aic_software_interrupt
//* Object : Software interrupt function
//* Input Parameters : none
//* Output Parameters : none
//* Functions called : at91_pio_write
//*----------------------------------------------------------------------------
__ramfunc void aic_software_interrupt(void)
{
//* Read the output state
if ( (AT91F_PIO_GetInput(AT91C_BASE_PIOA) & LED2 ) == LED2 )
{
AT91F_PIO_ClearOutput( AT91C_BASE_PIOA, LED2 );
}
else
{
AT91F_PIO_SetOutput( AT91C_BASE_PIOA, LED2 );
}
}
//*----------------------------------------------------------------------------
//* Function Name : pio_c_irq_handler
//* Object : Irq Handler called by the irq_pio.s
//* Input Parameters : none
//* Output Parameters : none
//* Functions called : at91_pio_read, at91_pio_write
//*----------------------------------------------------------------------------
__ramfunc void pio_c_irq_handler ( void )
{
int dummy;
//* Read the output state
if ( (AT91F_PIO_GetInput(AT91C_BASE_PIOA) & LED2 ) == LED2 )
{
AT91F_PIO_ClearOutput( AT91C_BASE_PIOA, LED2);
}
else
{
AT91F_PIO_SetOutput( AT91C_BASE_PIOA, LED2);
}
//* enable the next PIO IRQ
dummy =AT91C_BASE_PIOA->PIO_ISR;
//* suppress the compilation warning
dummy =dummy;
//* while SW3 is push wait
while ( (AT91F_PIO_GetInput(AT91C_BASE_PIOA) & SW3_MASK ) != SW3_MASK );
}
//*----------------------------------------------------------------------------
//* Function Name : delay
//* Object : Wait
//* Input Parameters : none
//* Output Parameters : none
//* Functions called : none
//*----------------------------------------------------------------------------
void delay ( void )
{
//* Set in Volatile for Optimisation
volatile unsigned int i ;
//* loop delay
for ( i = 0 ;(i < WAIT_TIME/100 );i++ ) ;
}
//*----------------------------------------------------------------------------
//* Function Name : main
//* Object : Main interrupt function
//* level timer 0 => 1
//* SW2 level Irq0 => 2
//* level timer 1 => 4
//* SW4 level PIOA => 6
//* level USART => 7
//* LEVEL FIQ => MAX
//* Input Parameters : none
//* Output Parameters : TRUE
//*----------------------------------------------------------------------------
int main( void )
//* Begin
{
unsigned int loop_count ;
AT91PS_AIC pAic;
//* Load System pAic Base address
pAic = AT91C_BASE_AIC;
//* Enable User Reset and set its minimal assertion to 960 us
AT91C_BASE_RSTC->RSTC_RMR = AT91C_RSTC_URSTEN | (0x4<<8) | (unsigned int)(0xA5<<24);
//* Init
loop_count = 0 ;
// First, enable the clock of the PIOB
AT91F_PMC_EnablePeriphClock ( AT91C_BASE_PMC, 1 << AT91C_ID_PIOA ) ;
//* then, we configure the PIO Lines corresponding to LED1 to LED8
//* to be outputs. No need to set these pins to be driven by the PIO because it is GPIO pins only.
AT91F_PIO_CfgOutput( AT91C_BASE_PIOA, LED_MASK ) ;
//* Clear the LED's. On the EB55 we must apply a "1" to turn off LEDs
AT91F_PIO_SetOutput( AT91C_BASE_PIOA, LED_MASK ) ;
//* open external PIO interrupt
//* define switch SW3 at PIO input for interrupt IRQ loop
AT91F_PIO_CfgInput(AT91C_BASE_PIOA, SW3_MASK | SW4_MASK);
AT91F_AIC_ConfigureIt ( pAic, AT91C_ID_PIOA, PIO_INTERRUPT_LEVEL,AT91C_AIC_SRCTYPE_INT_HIGH_LEVEL, pio_c_irq_handler);
AT91F_PIO_InterruptEnable(AT91C_BASE_PIOA,SW4_MASK);
//* set the interrupt by software
AT91F_AIC_EnableIt (pAic, AT91C_ID_PIOA);
//* open external IRQ interrupt
AT91F_PIO_CfgPeriph(AT91C_BASE_PIOA,SW2_MASK,0);
//* open external IRQ0 interrupt
AT91F_AIC_ConfigureIt ( pAic, AT91C_ID_IRQ0, IRQ0_INTERRUPT_LEVEL,AT91C_AIC_SRCTYPE_EXT_NEGATIVE_EDGE, at91_IRQ0_handler);
AT91F_AIC_EnableIt (pAic, AT91C_ID_IRQ0);
//* Open the software interrupt on the AIC
AT91F_AIC_ConfigureIt ( pAic, AT91C_ID_SYS, SOFT_INTERRUPT_LEVEL, AT91C_AIC_SRCTYPE_INT_POSITIVE_EDGE, aic_software_interrupt);
AT91F_AIC_EnableIt (pAic, AT91C_ID_SYS);
//* open FIQ interrupt
AT91F_PIO_CfgPeriph(AT91C_BASE_PIOA,SW1_MASK,0);
AT91F_AIC_ConfigureIt ( pAic, AT91C_ID_FIQ, FIQ_INTERRUPT_LEVEL,AT91C_AIC_SRCTYPE_EXT_NEGATIVE_EDGE, FIQ_init_handler);
AT91F_AIC_EnableIt (pAic, AT91C_ID_FIQ);
//* generate FIQ interrupt by software
AT91F_AIC_Trig (pAic,AT91C_ID_FIQ) ;
//* Init timer interrupt
timer_init();
//* Init Usart
Usart_init();
//* generate software interrupt
AT91F_AIC_Trig (pAic,AT91C_ID_SYS) ;
for (;;)
{
AT91F_PIO_ClearOutput( AT91C_BASE_PIOA, LED1 );
delay () ;
AT91F_PIO_SetOutput( AT91C_BASE_PIOA, LED1 );
delay () ;
loop_count ++ ;
//* Set LED by software interrupt
if (loop_count == 10)
{
loop_count=0;
//* Software interrupt
AT91F_AIC_Trig (pAic,AT91C_ID_SYS) ;
}
}
//* End
}
没有合适的资源?快使用搜索试试~ 我知道了~
AT91SAM7S64-Interrupt.rar___interrupt I_at91sam7s64 _at91sam7s
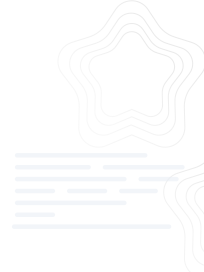
共113个文件
html:34个
r79:12个
root:9个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 164 浏览量
2022-09-23
10:52:51
上传
评论
收藏 451KB RAR 举报
温馨提示
IAR环境下AT91SAM7S64开发板例程(实现中断功能)
资源推荐
资源详情
资源评论
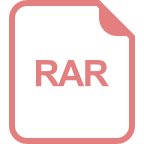
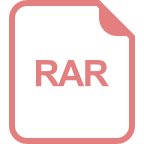
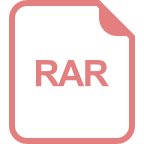
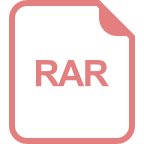
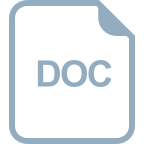
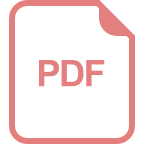
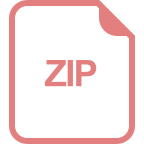
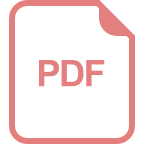
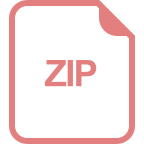
收起资源包目录

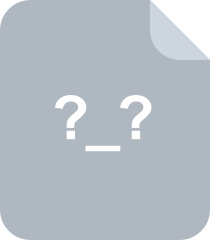
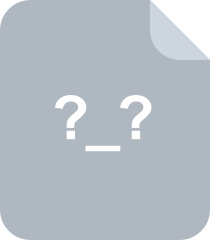
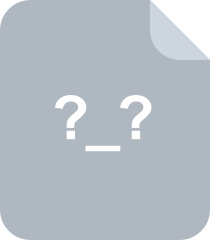
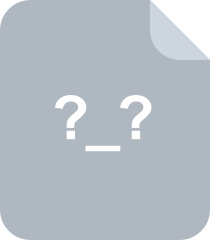
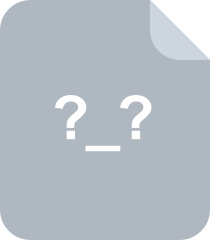
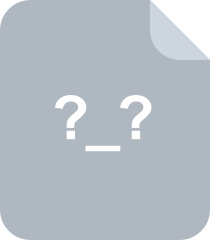
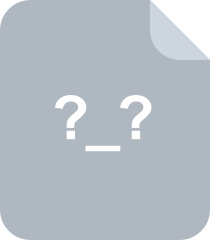
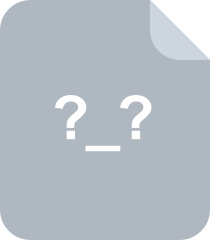
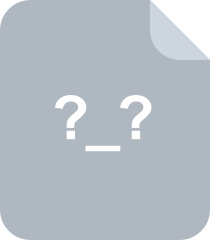
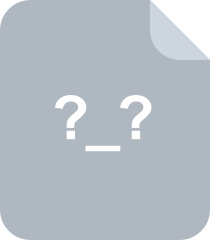
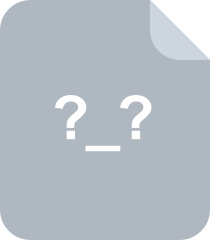
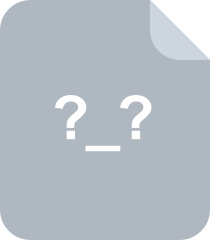
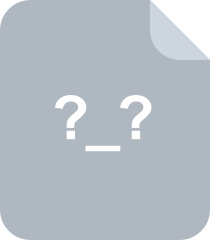
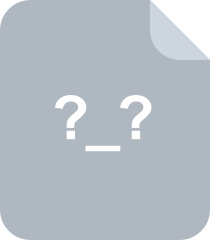
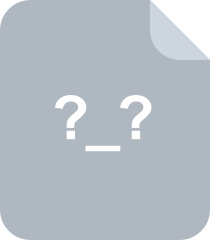
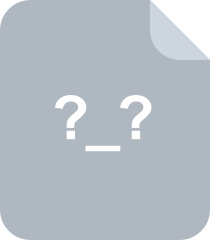
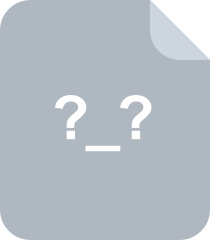
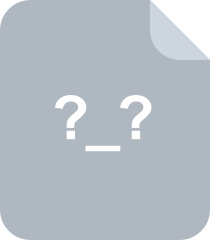
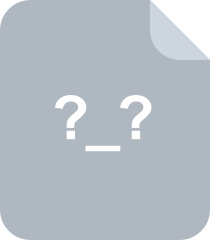
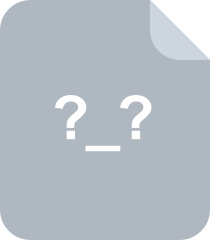
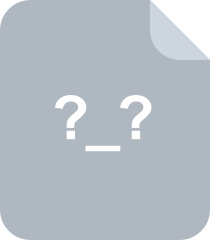
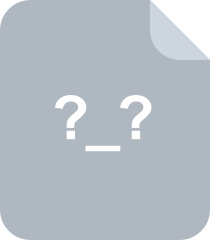
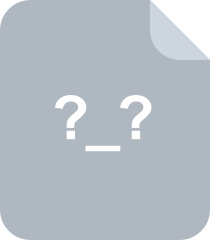
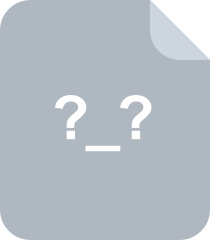
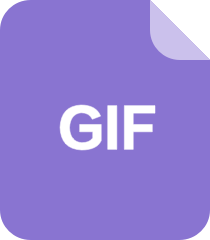
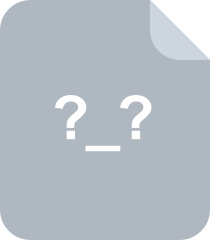
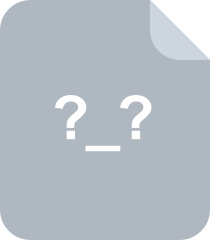
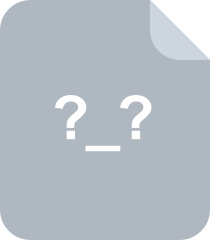
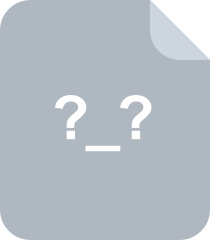
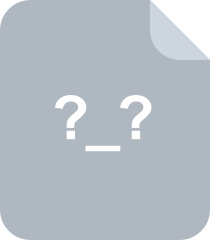
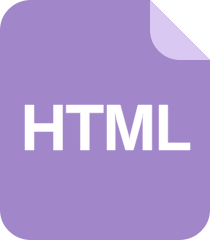
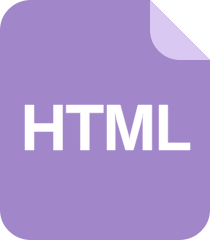
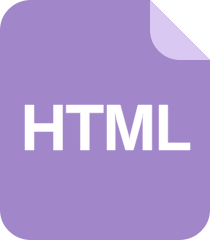
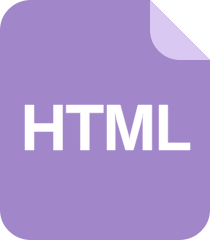
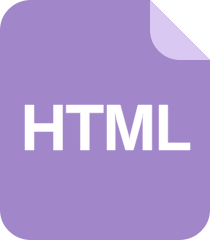
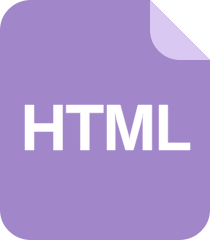
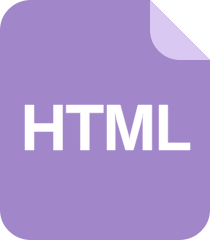
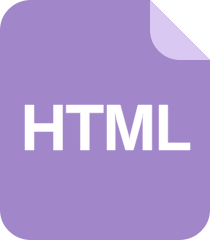
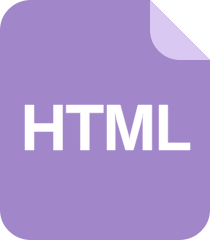
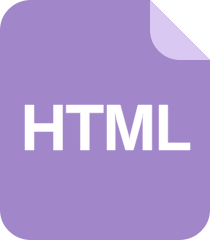
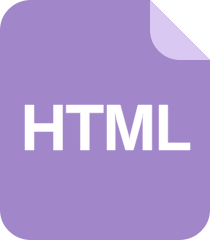
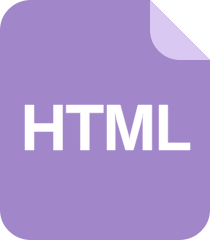
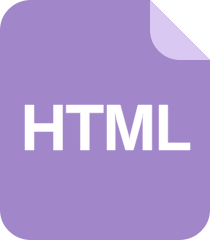
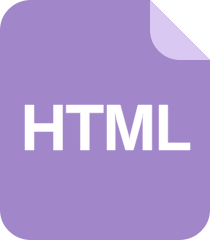
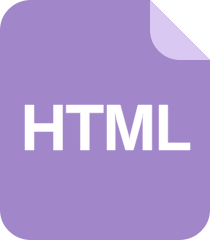
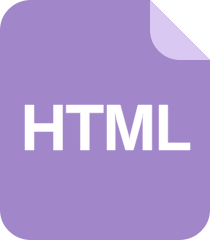
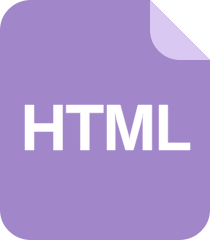
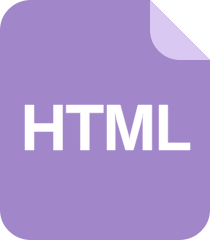
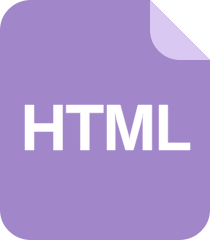
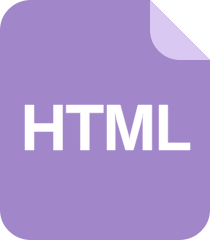
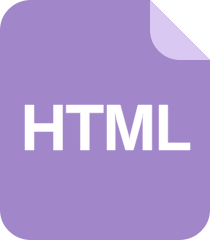
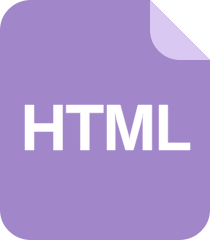
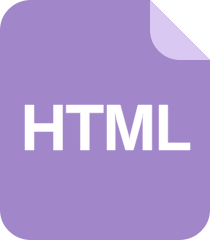
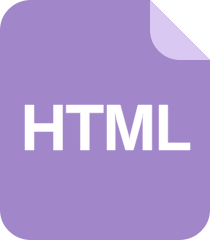
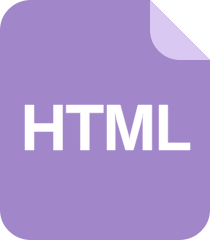
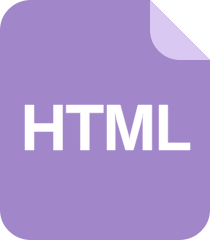
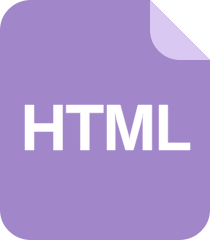
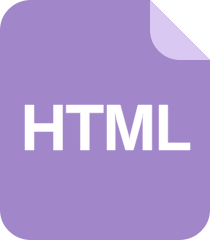
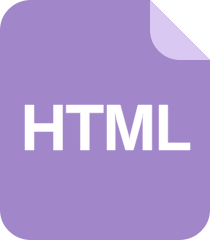
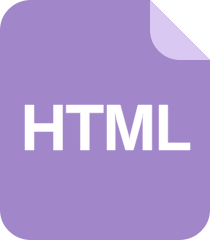
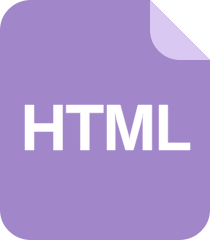
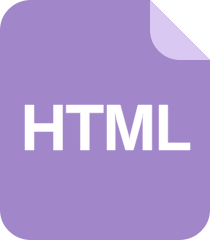
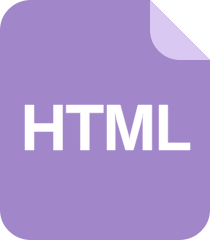
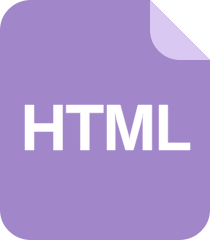
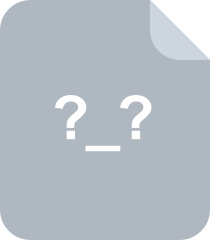
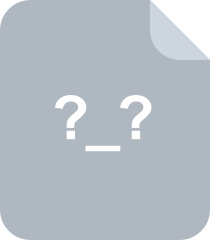
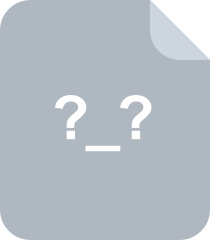
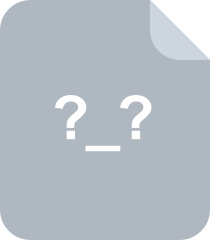
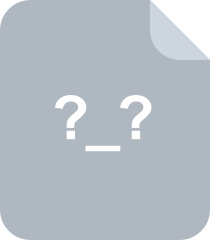
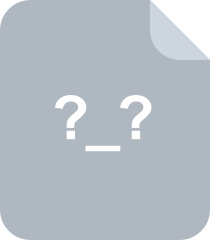
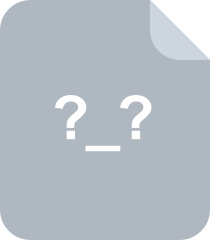
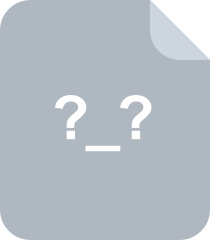
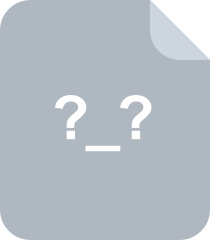
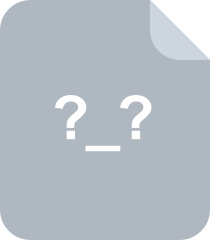
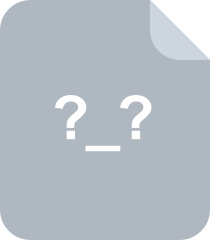
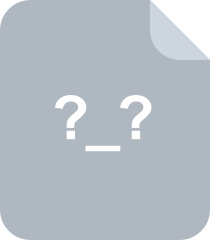
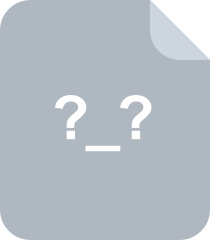
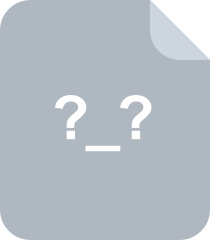
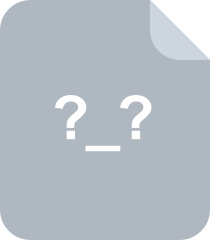
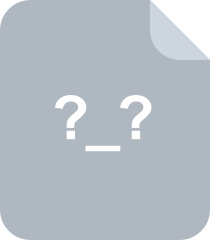
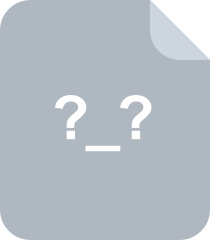
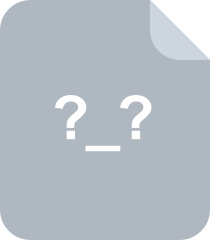
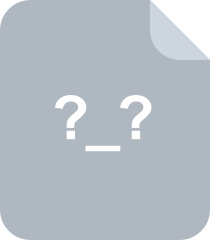
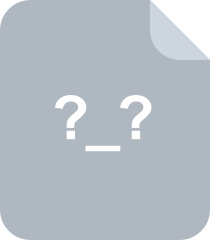
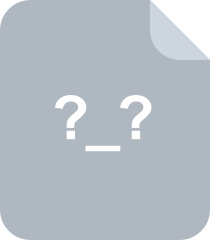
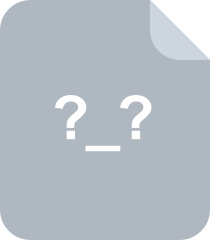
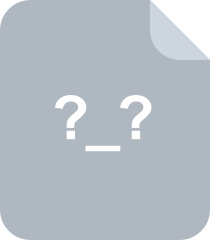
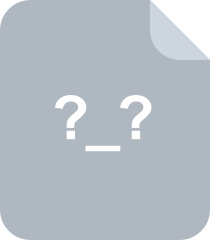
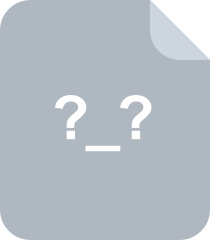
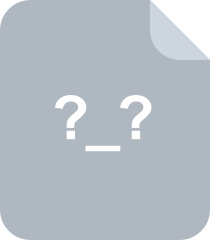
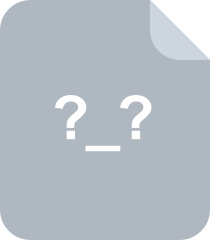
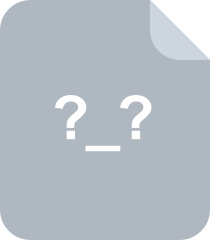
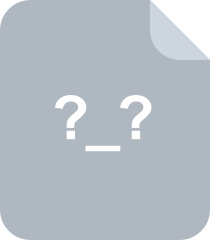
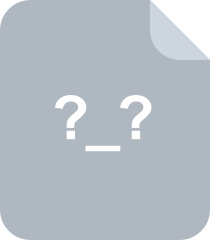
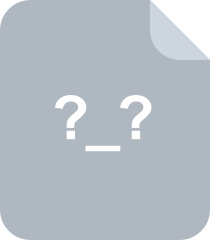
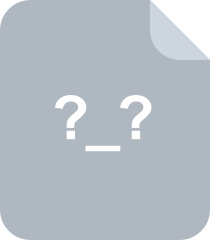
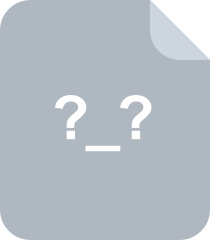
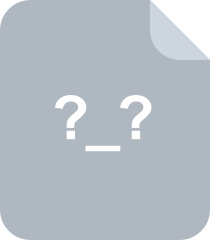
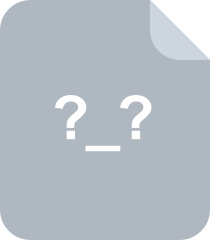
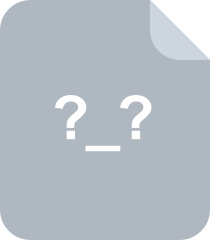
共 113 条
- 1
- 2
资源评论
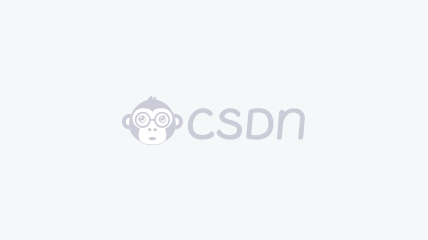

朱moyimi
- 粉丝: 61
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

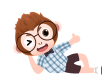
安全验证
文档复制为VIP权益,开通VIP直接复制
