//Graph.cpp - Version 4.0 (Brian Convery, November, 2002)
#include "stdafx.h"
#include "afxtempl.h"
#include "math.h"
#include "Graph.h"
#include "GraphDef.h"
#include "GraphDataSet.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CGraph
CGraph::CGraph()
{
dataSeries = new CObList();
legendFontSize = 12;
legendMaxText = 0;
minXvalue = 0;
minYvalue = 0;
maxXvalue = 0;
maxYvalue = 0;
quadsDisplay = 0;
minXTick = 0.0;
minYTick = 0.0;
maxXTick = 0.0;
maxYTick = 0.0;
xTickStep = 1;
yTickStep = 1;
gridLinesEnabled = FALSE;
horizontalAlign = FALSE;
xTicksEnabled = TRUE;
xTickFontSize = 12;
yTickFontSize = 12;
graphTitle = _T("Graph");
legendTitle = _T("Legend");
axisXLabel = _T("X Axis");
axisYLabel = _T("Y Axis");
doubleBufferingEnabled = FALSE;
fontType = "Arial";
}
CGraph::~CGraph()
{
if(dataSeries)
{
POSITION pos = dataSeries->GetHeadPosition();
while(pos != NULL)
{
CGraphDataSet* curDataSet = (CGraphDataSet*)dataSeries->GetNext(pos);
delete curDataSet;
}
dataSeries->RemoveAll();
delete dataSeries;
}
}
/*BEGIN_MESSAGE_MAP(CGraph, CStatic)
//{{AFX_MSG_MAP(CGraph)
// NOTE - the ClassWizard will add and remove mapping macros here.
//}}AFX_MSG_MAP
END_MESSAGE_MAP()*/
/////////////////////////////////////////////////////////////////////////////
// CGraph message handlers
void CGraph::ColorBySeries(BOOL tf)
{
}
void CGraph::ColorByGroups(BOOL tf)
{
}
void CGraph::OpenDataSet()
{
dataSet = new CGraphDataSet();
}
void CGraph::CloseDataSet()
{
dataSeries->AddTail((CObject*)dataSet);
}
void CGraph::SetData(double x, double y)
{
dataSet->AddData(x, y);
if(x > maxXvalue)
maxXvalue = x;
if(x < minXvalue)
minXvalue = x;
if(y > maxYvalue)
maxYvalue = y;
if(y < minYvalue)
minYvalue = y;
}
void CGraph::SetData(double x, double y, COLORREF color) //if ColorBySeries
{
dataSet->AddData(x, y, color);
if(x > maxXvalue)
maxXvalue = x;
if(x < minXvalue)
minXvalue = x;
if(y > maxYvalue)
maxYvalue = y;
if(y < minYvalue)
minYvalue = y;
}
void CGraph::SetData(CString xStr, double y)
{
dataSet->AddData(xStr, y);
if(xStr.GetLength() > legendMaxText)
legendMaxText = xStr.GetLength();
if(y > maxYvalue)
maxYvalue = y;
if(y < minYvalue)
minYvalue = y;
}
void CGraph::SetData(CString xStr, double y, COLORREF color) //if ColorBySeries
{
dataSet->AddData(xStr, y, color);
if(xStr.GetLength() > legendMaxText)
legendMaxText = xStr.GetLength();
if(y > maxYvalue)
maxYvalue = y;
if(y < minYvalue)
minYvalue = y;
}
void CGraph::SetDataSetLabel(CString label)
{
dataSet->SetLabel(label);
}
void CGraph::SetColor(COLORREF color) //if ColorByGroups
{
dataSet->SetColor(color);
}
void CGraph::SetDataLegend(CString legend)
{
dataSet->SetLegend(legend);
if(legend.GetLength() > legendMaxText)
legendMaxText = legend.GetLength();
}
void CGraph::SetGraphTitle(CString label)
{
graphTitle = label;
}
void CGraph::SetLegendTitle(CString legend)
{
legendTitle = legend;
if(legendTitle.GetLength() > legendMaxText)
legendMaxText = legendTitle.GetLength();
}
void CGraph::SetXAxisLabel(CString label)
{
axisXLabel = label;
}
void CGraph::SetYAxisLabel(CString label)
{
axisYLabel = label;
}
void CGraph::SetTickLimits(double minXLimit, double maxXLimit,
double minYLimit, double maxYLimit)
{
minXTick = minXLimit;
minYTick = minYLimit;
maxXTick = maxXLimit;
maxYTick = maxYLimit;
}
void CGraph::SetTickStep(double xStep, double yStep)
{
//tick steps should be positive values...correct them if not
if(xStep > 0)
xTickStep = xStep;
else
xTickStep = 0 - xStep;
if(yStep > 0)
yTickStep = yStep;
else
yTickStep = 0 - yStep;
}
void CGraph::SetHorizontalAlignment(BOOL enable)
{
horizontalAlign = enable;
}
BOOL CGraph::RemoveDataSet(int ds)
{
BOOL retVal = FALSE;
if((dataSeries) && (dataSeries->GetCount() >= ds))
{
POSITION pos;
pos = dataSeries->GetHeadPosition();
for(int i = 1; i < ds; i++)
{
dataSeries->GetNext(pos);
}
CGraphDataSet* curDataSet = (CGraphDataSet*)dataSeries->GetAt(pos);
delete curDataSet;
dataSeries->RemoveAt(pos);
retVal = TRUE;
}
return retVal;
}
void CGraph::RemoveAllData()
{
while(dataSeries->GetCount())
{
POSITION pos = dataSeries->GetHeadPosition();
CGraphDataSet* curDataSet = (CGraphDataSet*)dataSeries->GetAt(pos);
delete curDataSet;
dataSeries->RemoveAt(pos);
}
delete dataSeries;
dataSeries = new CObList();
}
void CGraph::EnableXAxisTicks(BOOL enable)
{
xTicksEnabled = enable;
}
void CGraph::EnableDoubleBuffering(BOOL enable)
{
doubleBufferingEnabled = enable;
}
void CGraph::SetFontType(CString font)
{
fontType = font;
}
void CGraph::DrawGraphBase(CDC *pDC)
{
CString tickLabel;
CWnd* graphWnd = pDC->GetWindow();
CRect graphRect;
if(doubleBufferingEnabled)
pDC->GetClipBox(&graphRect);
else
graphWnd->GetClientRect(&graphRect);
TEXTMETRIC tm;
//reset graph to be clear background
CBrush backBrush (WHITE); //replace with desired background color. format: RGB(##,##,##) if your own color is desired
CBrush* pOldBackBrush;
pOldBackBrush = pDC->SelectObject(&backBrush);
pDC->Rectangle(0, 0, graphRect.Width(), graphRect.Height());
pDC->SelectObject(pOldBackBrush);
yAxisHeight = graphRect.Height() - 20; //for frame window and status bar
xAxisWidth = graphRect.Width() - 5; //for frame window
yAxisLocation = 5;
xAxisLocation = yAxisHeight - 5;
graphBottom = yAxisHeight - 5;
//draw graph title
CFont titleFont;
int tFontSize = 24;
while((graphTitle.GetLength() * (tFontSize / 2)) > xAxisWidth)
{
tFontSize -= 2;
}
titleFont.CreateFont(tFontSize, 0, 0, 0, 700, FALSE, FALSE, 0,
ANSI_CHARSET, OUT_DEFAULT_PRECIS, CLIP_DEFAULT_PRECIS,
DEFAULT_QUALITY, DEFAULT_PITCH | FF_ROMAN,fontType);
CFont* pOldFont = (CFont*) pDC->SelectObject(&titleFont);
pDC->GetTextMetrics(&tm);
int charWidth = tm.tmAveCharWidth;
pDC->TextOut((graphRect.Width() / 2) - ((graphTitle.GetLength() / 2) * charWidth), 10, graphTitle);
pDC->SelectObject(pOldFont);
yAxisHeight -= (20 + tm.tmHeight);
}
void CGraph::DrawAxis(CDC *pDC)
{
TEXTMETRIC tm;
pDC->SetTextColor(BLACK);
CFont sideFont, axisFont, dummyFont;
CFont* pOldFont;
int charWidth;
CString tickLabel;
//first off, determine how many quadrants are being used
//there are 9 possible combinations for quadrants :
//4 regular graphs, depicting each quadrant from a 4 quad graph
//4 split quads : __|__ rotated around center point
//1 4 quad graph
//resulting types are therefore (the __ and ~~ are the x axis lines)
//I will number them in order shown below (1 - 9)
//
// |__ |~~ __| ~~|
//
// __|__ ~~|~~ |-- --|
//
// --|--
//
//in case of disabled ticks, define defaults
quadsDisplay = 1;
if((maxYvalue <= 0) && (minYvalue < 0))
quadsDisplay = 2;
if((maxXvalue > 0) && (minXvalue >= 0) && (maxYvalue > 0) && (minYvalue >= 0))
{
quadsDisplay = 1;
if(minXTick < 0)
minXTick = 0;
if(minYTick < 0)
minYTick = 0;
if(maxXTick < 0)
maxXTick = 0;
if(maxYTick < 0)
maxYTick = 0;
}
if((maxXvalue > 0) && (minXvalue >= 0) && (maxYvalue <= 0) && (minYvalue < 0))
{
quadsDisplay = 2;
if(minXTick < 0)
minXTick = 0;
if(minYTick > 0)
minYTick = 0;
if(maxXTick < 0)
maxXTick = 0;
if(maxYTick > 0)
maxYTick = 0;
}
if((maxXvalue <= 0) && (minXvalue < 0) && (maxYvalue > 0) && (minYvalue >= 0))
{
quadsDisplay = 3;
if(minXTick > 0)
minXTick = 0;
if(minYTick < 0)
minYTick = 0;
if(maxXTick

朱moyimi
- 粉丝: 75
- 资源: 1万+
最新资源
- 【java毕业设计】员工在线知识培训考试平台源码(ssm+mysql+说明文档).zip
- 【java毕业设计】演出道具租赁管理系统源码(ssm+mysql+说明文档).zip
- ScanMaster RPP3 脉冲放大器手册
- 【java毕业设计】社区医院儿童预防接种管理系统源码(ssm+mysql+说明文档).zip
- 【java毕业设计】企业台账管理平台源码(ssm+mysql+说明文档+LW).zip
- 【java毕业设计】面向品牌会员的在线商城源码(ssm+mysql+说明文档).zip
- 【java毕业设计】消防物资存储系统源码(ssm+mysql+说明文档+LW).zip
- 【java毕业设计】高校课程评价系统源码(ssm+mysql+说明文档+LW).zip
- 【java毕业设计】大健康老年公寓管理系统源码(ssm+mysql+说明文档).zip
- 【java毕业设计】小雨杂志在线投稿网站源码(ssm+mysql+说明文档+LW).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


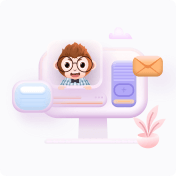