支持发附件功能(11KB)smtp.rar_java smtp_smtp java_smtp 附件_邮件 附件
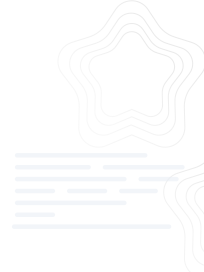

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
Java SMTP邮件发送是Java开发中常见的一项功能,用于通过简单邮件传输协议(SMTP)发送电子邮件。在标题和描述中提到的“支持发附件功能(11KB)smtp.rar”,这表明我们关注的是一个实现了SMTP邮件服务并且具备附件发送功能的Java程序。这个程序可能是一个小型的实用工具或者代码示例,帮助开发者理解如何在Java应用中添加邮件发送及附件处理的逻辑。 让我们了解SMTP协议。SMTP是Internet上用于发送电子邮件的标准协议,它定义了邮件服务器之间通信的规则。JavaMail API是Java平台上的一个库,提供了与多种邮件协议(包括SMTP)交互的接口和类,使得开发者能够轻松地在Java应用中实现邮件发送。 在JavaMail API中,`javax.mail.Transport` 类用于实际的邮件发送,而`javax.mail.internet.MimeMessage` 类则用于构建邮件内容,包括主题、正文、发件人、收件人等信息,以及附件。为了添加附件,我们需要使用`MimeBodyPart` 和 `MimeMultipart` 类。`MimeBodyPart` 用于创建包含附件的邮件部分,`MimeMultipart` 则可以将多个邮件部分组合成一个邮件消息。 下面是一个基本的Java SMTP邮件发送带附件的示例: ```java import javax.mail.*; import javax.mail.internet.*; import java.util.Properties; public class MailSender { public static void main(String[] args) { String from = "youremail@example.com"; String to = "recipient@example.com"; String subject = "邮件主题"; String body = "邮件正文"; Properties props = System.getProperties(); props.put("mail.smtp.host", "smtp.example.com"); props.put("mail.smtp.port", "587"); props.put("mail.smtp.auth", "true"); Session session = Session.getDefaultInstance(props, new Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(from, "yourpassword"); } }); try { MimeMessage message = new MimeMessage(session); message.setFrom(new InternetAddress(from)); message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(to)); message.setSubject(subject); message.setText(body); // 添加附件 MimeBodyPart attachmentPart = new MimeBodyPart(); DataSource source = new FileDataSource("path_to_your_file"); attachmentPart.setDataHandler(new DataHandler(source)); attachmentPart.setFileName(source.getName()); MimeMultipart multipart = new MimeMultipart(); multipart.addBodyPart(messagePart); message.setContent(multipart); Transport.send(message); System.out.println("邮件已发送"); } catch (MessagingException e) { e.printStackTrace(); } } } ``` 在描述中提到了“在分析以往一些排课软件的基础上,提出一个通用的排课管理信息系统的设计方法”。这暗示了这个SMTP邮件程序可能被用作排课系统的一部分,用于向教师或学生发送课程安排、通知或其他相关文件。排课系统通常涉及到复杂的算法,如回溯法、贪心算法或遗传算法,以优化课程安排,满足各种约束条件,如教室容量、教师时间表等。 压缩包中的“www.pudn.com.txt”可能是提供了一些关于SMTP邮件服务的说明或参考资料,而“smtp”可能是一个包含SMTP邮件发送代码的Java类。要深入了解这个程序的工作原理,你需要解压rar文件并查看这些文件的内容。 这个Java SMTP邮件程序是一个利用JavaMail API实现的工具,允许用户通过SMTP发送带有附件的电子邮件。它在排课管理信息系统中可能扮演着通知和信息传递的角色,帮助系统管理员便捷地分发课程安排和其他重要文件。对于开发者来说,理解和掌握这样的功能有助于提升他们在构建类似系统时的能力。
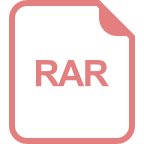
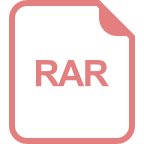
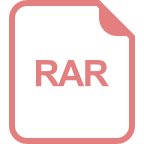
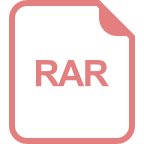
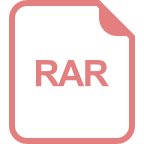
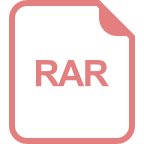
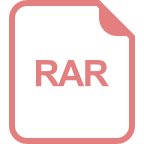
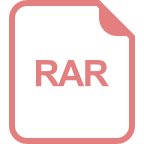
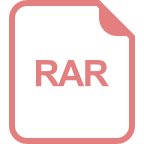
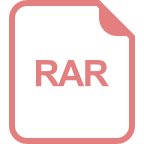
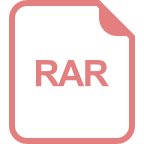
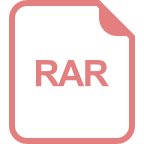
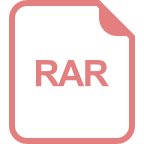
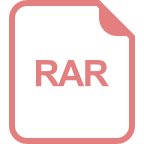
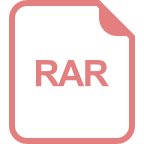
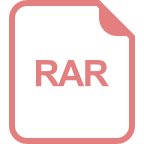
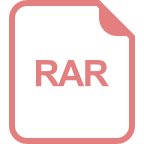
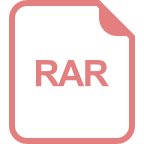
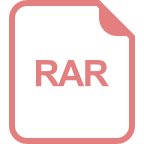
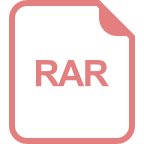
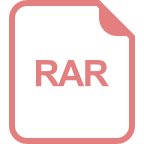
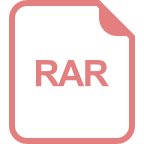
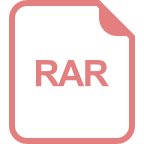


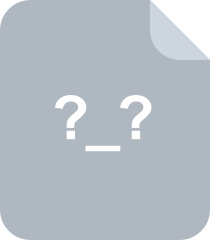
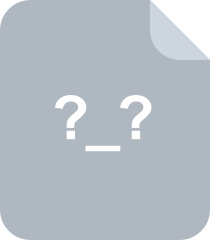
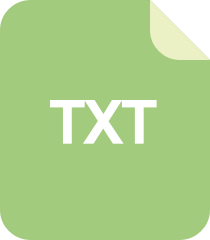
- 1
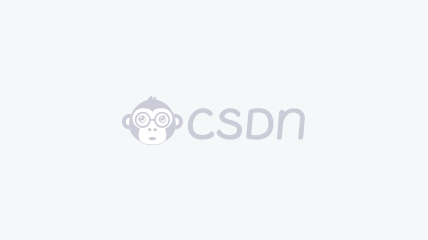

- 粉丝: 104
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

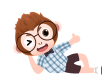
最新资源
- 2024年下半年软考中级网络工程师手工负载分担模式链路聚合配置实验
- java二手车销售管理系统源码(前台+后台)数据库 MySQL源码类型 WebForm
- VC++2019 访问和操作SQLite数据的例子
- 2024年下半年软考中级网络工程师lacp模式链路聚合配置实验
- 使用JS脚本实现spotfire分析弹出窗口demo,自用
- 2024年下半年软考中级网络工程师lacp配置实验
- 基于MATLAB的车牌识别实现车牌定位系统【GUI含界面】.zip
- 基于MATLAB的车牌识别实现车牌定位代码【含界面GUI】.zip
- 基于MATLAB的车牌识别实现车牌定位代码【含界面GUI】(1).zip
- 2024年下半年软考中级网络工程师小型园区组网配置实验

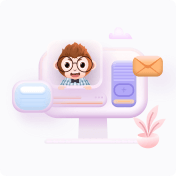
