#include <iostream>
#include <iomanip>
#include "SML.h"
using namespace std;
SML::SML(int *Memory,int acc,int ins,int ope,int opd,int inr ):memory(Memory)
{
accumulator=acc;
instructionCounter=ins;
operationCode=ope;
operand=opd;
instructionRegister=inr;
}
void SML::load()
{
int instruction=0;
int i = 0;
cout<<"*** Welcome to Simpletron ***"<<endl;
cout<<"*** Please enter your program one instruction ***"<<endl;
cout<<"*** ( or data word ) at a time. I will type the ***"<<endl;
cout<<"*** location number and a question mark ( ? ). ***"<<endl;
cout<<"*** You then type the word for that location. ***"<<endl;
cout<<"*** Type the sentinel -99999 to stop entering ***"<<endl;
cout<<"*** your program. ***" <<endl;
cout<<"00"<<" "<<"?"<<" "<<":";
cin>>instruction;
while ( instruction != END )
{
if (!(instruction>=-99999 &&instruction <=99999) )
{
cout<< "Number out of range. Please enter again."<<endl;
}
else
{
memory[ i++ ] = instruction;
}
cout<<setw(2)<<setfill('0')<<i<<" "<<"?"<<" :";
cin>>instruction;
}
}
void SML::execute()
{
int fatal = FALSE;
int temp=0;
cout<<endl<<"************START************"<<endl<<endl;
instructionRegister = memory[ instructionCounter ];
operationCode = instructionRegister / 100;
operand = instructionRegister % 100;
while ( operationCode != HALT || !fatal )
{
switch ( operationCode )
{
case READ:
cout<<"Enter an integer: ";
cin>>temp;
while ( !(temp>=-9999 && temp <=9999) )
{
cout<< "Number out of range. Please enter again: " ;
cin>>temp;
}
memory[operand] = temp;
++instructionCounter;
break;
case WRITE:
cout<<"Contents of"<<setw(2)<<setfill('0')<<operand<<":"<<memory[operand];
++instructionCounter;
break;
case LOAD:
accumulator = memory[ operand ];
++instructionCounter;
break;
case STORE:
memory[operand ] =accumulator;
++instructionCounter;
break;
case ADD:
temp = accumulator + memory[ operand ];
if ( !(temp>=-9999 && temp<=9999) )
{
cout<<"*** FATAL ERROR: Accumulator overflow ***"<<endl;
cout<<"*** Simpletron execution abnormally terminated ***"<<endl;
fatal = TRUE;
}
else
{
accumulator = temp;
++instructionCounter;
}
break;
case SUBTRACT:
temp = accumulator - memory[ operand ];
if (!(temp>=-9999 && temp<=9999) )
{
cout<<"*** FATAL ERROR: Accumulator overflow ***"<<endl;
cout<<"*** Simpletron execution abnormally terminated ***"<<endl;
fatal = TRUE;
}
else
{
accumulator = temp;
++instructionCounter;
}
break;
case DIVIDE:
if ( memory[ operand] == 0 )
{
cout<<"*** FATAL ERROR: Attempt to divide by zero ***"<<endl;
cout<<"*** Simpletron execution abnormally terminated ***"<<endl;
fatal = TRUE;
}
else
{
accumulator /= memory[operand ];
++instructionCounter;
}
break;
case MULTIPLY:
temp = accumulator * memory[operand ];
if ( !(temp>=-9999 && temp<=9999 ))
{
cout<< "*** FATAL ERROR: Accumulator overflow ***"<<endl;
cout<< "*** Simpletron execution abnormally terminated ***"<<endl;
fatal = TRUE;
}
else
{
accumulator = temp;
++instructionCounter;
}
break;
case BRANCH:
instructionCounter = operand;
break;
case BRANCHNEG:
if ( accumulator < 0 )
{
instructionCounter = operand;
}
else
{
++instructionCounter;
}
break;
case BRANCHZERO:
if ( accumulator == 0 )
{
instructionCounter = operand;
}
else
{
++instructionCounter;
}
break;
default:
cout<<"*** FATAL ERROR: Invalid opcode detected ***"<<endl;
cout<<"*** Simpletron execution abnormally terminated ***"<<endl;
fatal = TRUE;
break;
}
instructionRegister = memory[ instructionCounter ];
operationCode = instructionRegister / 100;
operand = instructionRegister % 100;
}
cout<<"*************END*************"<<endl;
}
void SML::dump()
{
int i;
cout<<"REGISTERS:"<<endl<< "accumulator(累加器寄存器):"<<accumulator<<endl<<"instructioncounter(正在执行指令内存位子):"
<<instructionCounter<<endl<<"instructionregister(内存转移): "<< instructionRegister<<endl
<<"operationcode(最后执行操作):"<< operationCode<<endl<< "operand(内存位子): "<< operand<<endl;
cout<<endl<<endl<<"MEMORY:"<<endl;
for ( i = 0; i <= 9; i++ )
{
cout<<" "<<setw(4)<<setfill(' ')<<i;
}
for ( i = 0; i <SIZE; i++ )
{
if ( i % 10 == 0 )
{
cout<<endl<<setw(2)<<setfill('0')<<i/10;
}
cout<<"+"<<setw(4)<<setfill('0')<<memory[i]<<" ";
}
cout<<endl;
}
没有合适的资源?快使用搜索试试~ 我知道了~
SML.rar_SML_c++sml
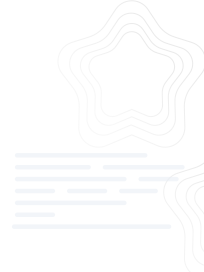
共5个文件
cpp:2个
suo:1个
h:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 34 浏览量
2022-09-20
19:32:38
上传
评论
收藏 8KB RAR 举报
温馨提示
通过C++实现得模拟SML代码,对学习C++编程的朋友有一定帮助,也能够帮助新手学习机器码在PC上执行的过程。读书是做的一个C++课程设计。
资源推荐
资源详情
资源评论
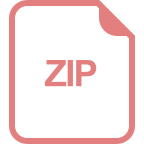
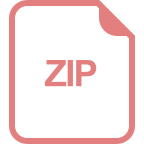
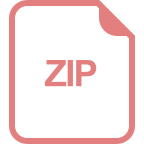
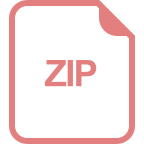
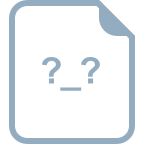
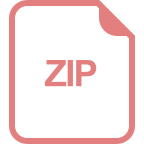
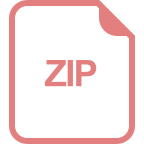
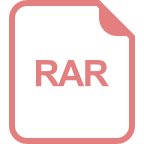
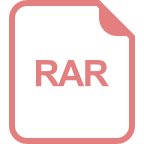
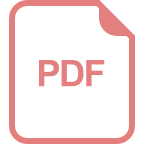
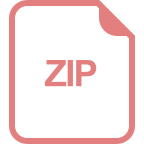
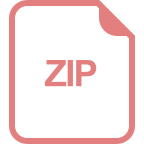
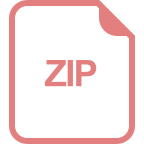
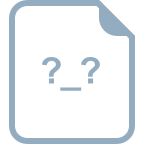
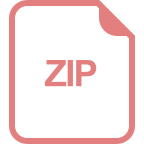
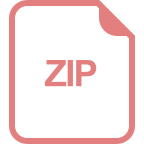
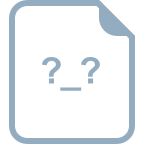
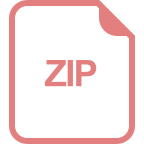
收起资源包目录




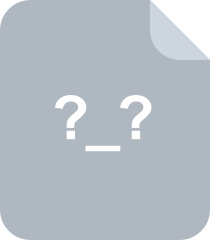
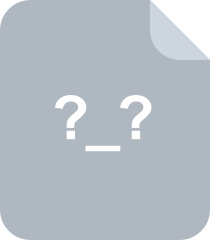
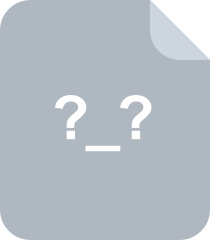
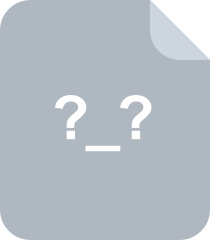
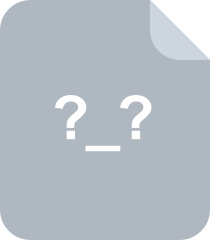
共 5 条
- 1
资源评论
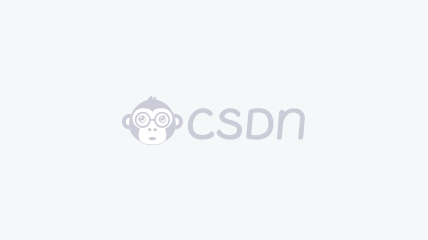

weixin_42653672
- 粉丝: 93
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

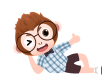
安全验证
文档复制为VIP权益,开通VIP直接复制
