#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<malloc.h>
#define MAX_PATH_SIZE 100
#define MAX_FILEDIR_NAME_SIZE 10
#define MAX_BLOCKS 20
#define BLOCK_SIZE 1024
typedef struct file_descriptor
{
int is_dir;
int fd_id;
int size;
char file_name[MAX_FILEDIR_NAME_SIZE];
char path[MAX_PATH_SIZE];
int blocks[MAX_BLOCKS];
}fd;
//N-ary tree node
typedef struct tree_node
{
fd *data;
int is_deleted;
struct tree_node *left_child;
struct tree_node *right_siblings;
struct tree_node *parent;
}t_node;
//binary search tree node
typedef struct bst_node
{
struct bst_node *parent;
struct bst_node *left;
struct bst_node *right;
char full_path[MAX_PATH_SIZE+MAX_FILEDIR_NAME_SIZE];
fd *file_desc;
}b_node;
b_node *bst_create()
{
b_node* root=NULL;
return root;
}
int bst_insert(b_node **bst_root_ptr,fd *data)
{
char fullpath[110];
strcpy(fullpath,data->path);
strcat(fullpath,"/");
strcat(fullpath,data->file_name);
if(*bst_root_ptr==NULL)
{
b_node* b1=(b_node*)malloc(sizeof(b_node));
b1->file_desc=data;
strcpy(b1->full_path,fullpath);
b1->left=NULL;
b1->right=NULL;
b1->parent=NULL;
*bst_root_ptr=b1;
return 0;
}
else
{
b_node* b1=(b_node*)malloc(sizeof(b_node));
b1->file_desc=data;
strcpy(b1->full_path,fullpath);
b1->left=NULL;
b1->right=NULL;
b1->parent=NULL;
b_node *curr,*par;
curr=*bst_root_ptr;
while(curr!=NULL)
{
par=curr;
if((strcmp(fullpath,curr->full_path))<0)
{
curr=curr->left;
}
else if((strcmp(fullpath,curr->full_path))>0)
{
curr=curr->right;
}
else if((strcmp(fullpath,curr->full_path))==0)
{
printf("file already present\n");
return 1;
}
}
if((strcmp(fullpath,par->full_path))<0)
{
par->left=b1;
b1->parent=par;
return 0;
}
else if((strcmp(fullpath,par->full_path))>0)
{
par->right=b1;
b1->parent=par;
return 0;
}
}
}
b_node *bst_search(b_node *bst_root,char *fullpath)
{
b_node* temp=bst_root;
if(bst_root==NULL)
{
return NULL;
}
else
{
while(temp!=NULL)
{
if((strcmp(temp->full_path,fullpath))==0)
{
return temp;
}
else if((strcmp(temp->full_path,fullpath))>0)
{
temp=temp->left;
}
else if((strcmp(temp->full_path,fullpath))<0)
{
temp=temp->right;
}
}
return NULL;
}
}
int bst_delete(b_node **bst_root_ptr,char *fullpath)
{
int isfound=0;
if(*bst_root_ptr==NULL)
{
printf("tree is empty\n");
return 1;
}
b_node* root=*bst_root_ptr;
b_node* location;
location=bst_search(root,fullpath);
if(location==NULL)
{
return 1;
}
//if location has no child
else if(location->left==NULL && location->right==NULL)
{
if(location->parent->left==location)
{
location->parent->left=NULL;
}
else if(location->parent->right==location)
{
location->parent->right=NULL;
}
return 0;
}
//if location has only right child
else if(location->left==NULL && location->right!=NULL)
{
if(location->parent->left==location)
{
location->parent->left=location->right;
}
else if(location->parent->right==location)
{
location->parent->right=location->right;
}
return 0;
}
//if location has only left child
else if(location->left!=NULL && location->right==NULL)
{
if(location->parent->left==location)
{
location->parent->left=location->left;
}
else if(location->parent->right==location)
{
location->parent->right=location->left;
}
return 0;
}
//if location has both child
else if(location->left!=NULL && location->right!=NULL)
{
b_node* parent,*x;
parent=location;
x=location;
while(x->left!=NULL)
{
parent=x;
x=x->left;
}
location->file_desc=x->file_desc;
strcpy(location->full_path,x->full_path);
x->parent->left=NULL;
return 0;
}
return 1;
}
int main()
{
b_node *root;
root=bst_create();
fd* dir1 = (fd*)malloc(sizeof(fd));
dir1->is_dir=1;
dir1->fd_id=1;
dir1->size=10;
strcpy(dir1->file_name,"BIN.txt");
strcpy(dir1->path,"/bin/");
dir1->blocks[0]=12;
fd* dir4 = (fd*)malloc(sizeof(fd));
dir4->is_dir=1;
dir4->fd_id=1;
dir4->size=10;
strcpy(dir4->file_name,"CIN.txt");
strcpy(dir4->path,"/bin/");
dir4->blocks[0]=12;
fd* dir5 = (fd*)malloc(sizeof(fd));
dir5->is_dir=1;
dir5->fd_id=1;
dir5->size=10;
strcpy(dir5->file_name,"YFS.txt");
strcpy(dir5->path,"/usr/");
dir5->blocks[0]=12;
int return_insert=bst_insert ( &root,dir1);
return_insert=bst_insert ( &root,dir4);
return_insert=bst_insert ( &root,dir5);
b_node *found =bst_search( root,"/usr/ADB.txt");
if(found != NULL)
printf("\nFilname :%s\n", found->full_path);
int return_delete=bst_delete(&root,"/usr/VFS.txt");
printf("\nReturn Value %d\n", return_delete);
}
BST.rar_binary search tree_tree
版权申诉
57 浏览量
2022-09-19
16:46:00
上传
评论
收藏 1KB RAR 举报

weixin_42653672
- 粉丝: 93
- 资源: 1万+
最新资源
- mybatis动态sql及其JAVA示例
- 微软常用运行库 游戏运行库 VC++各个版本
- 微信小程序开发教程.pptx
- MyBatis动态SQL是一种强大的特性,它允许我们在SQL语句中根据条件动态地添加或删除某些部分,从而实现更加灵活和高效的数据
- 锐捷网络认证中心网络管理.pdf
- MyBatis动态SQL是一种强大的特性,它允许我们在SQL语句中根据条件动态地添加或删除某些部分,从而实现更加灵活和高效的数据
- SD8233LF是一款用于单按键触摸及接近感应开关,其用途是替代传统的机械型开关芯片IC
- 基于YOLOv5的烟雾火焰检测算法研究
- 基于STM32的联合调试侦听设备解决方案原理图PCB源文件调试工具视频(大赛作品)
- MyBatis动态SQL是一种强大的特性,它允许我们在SQL语句中根据条件动态地添加或删除某些部分,从而实现更加灵活和高效的数据
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


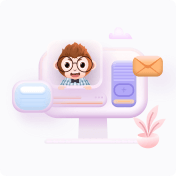