//-----------------------------------------------------------------------------
// F04x_UARTs_STDIO_Polled_2UARTs.c
//-----------------------------------------------------------------------------
// Copyright 2005 Silicon Laboratories, Inc.
// http://www.silabs.com
//
// Program Description:
//
// This program demonstrates configuration of UART0 and UART1 and includes
// an example of how to overload the STDIO library functions to enable
// selection of either UART0 or UART1 in code, via the "Uart" global variable.
//
//
// How To Test:
//
// 1) Download code to a 'F04x device that is connected to a UART transceiver
// 2) Verify jumpers J6 and J9 are populated on the 'F04x TB.
// 3) Connect serial cable from the transceiver to a PC
// 4) On the PC, open HyperTerminal (or any other terminal program) and connect
// to the COM port at <BAUDRATE> and 8-N-1
// 5) HyperTerminal will print a message if everything is working properly
//
//
// FID: 04X000032
// Target: C8051F04x
// Tool chain: KEIL C51 7.50 / KEIL EVAL C51
// Command Line: None
//
// Release 1.0
// -Initial Revision (BW / TP)
// -07 DEC 2006
//
//-----------------------------------------------------------------------------
// Includes
//-----------------------------------------------------------------------------
#include <C8051F040.h> // SFR declarations
#include <stdio.h>
//-----------------------------------------------------------------------------
// 16-bit SFR Definitions for 'F04x
//-----------------------------------------------------------------------------
sfr16 RCAP2 = 0xCA; // Timer2 capture/reload
sfr16 TMR2 = 0xCC; // Timer2
//-----------------------------------------------------------------------------
// Global Constants
//-----------------------------------------------------------------------------
#define BAUDRATE 115200 // Baud rate of UART in bps
// SYSCLK = System clock frequency in Hz
#define SYSCLK 22118400L
//-----------------------------------------------------------------------------
// Function Prototypes
//-----------------------------------------------------------------------------
void OSCILLATOR_Init (void);
void PORT_Init_UART0 (void);
void PORT_Init_UART1 (void);
void UART0_Init (void);
void UART1_Init (void);
//-----------------------------------------------------------------------------
// Global Variables
//-----------------------------------------------------------------------------
unsigned char Uart; // Global variable -- when '0', UART0
// is used for stdio; when '1', UART1
// is used for stdio
//-----------------------------------------------------------------------------
// main() Routine
//-----------------------------------------------------------------------------
void main (void)
{
SFRPAGE = CONFIG_PAGE;
WDTCN = 0xDE; // Disable watchdog timer
WDTCN = 0xAD;
OSCILLATOR_Init (); // Initialize oscillator
PORT_Init_UART0 (); // Initialize crossbar and GPIO
UART0_Init (); // Initialize UART0
UART1_Init (); // Initialize UART1
while (1)
{
// print something to UART0
PORT_Init_UART0 (); // Configure Crossbar to pinout
// UART0 TX and RX to P0.0 and P0.1
Uart = 0;
printf ("UART 0\n\n");
// now print something to UART1
PORT_Init_UART1 (); // Configure Crossbar to pinout
// UART1 TX and RX to P0.0 and P0.1
Uart = 1;
printf ("UART 1\n\n");
}
}
//-----------------------------------------------------------------------------
// Initialization Subroutines
//-----------------------------------------------------------------------------
//-----------------------------------------------------------------------------
// OSCILLATOR_Init
//-----------------------------------------------------------------------------
//
// Return Value : None
// Parameters : None
//
// This function initializes the system clock to use an external 22.1184MHz
// crystal.
//
//-----------------------------------------------------------------------------
void OSCILLATOR_Init (void)
{
int i; // Software timer
char SFRPAGE_SAVE = SFRPAGE; // Save Current SFR page
SFRPAGE = CONFIG_PAGE; // Set SFR page
OSCICN = 0x80; // Set internal oscillator to run
// at its slowest frequency
CLKSEL = 0x00; // Select the internal osc. as
// the SYSCLK source
// Initialize external crystal oscillator to use 22.1184 MHz crystal
OSCXCN = 0x67; // Enable external crystal osc.
for (i=0; i < 256; i++); // Wait at least 1ms
while (!(OSCXCN & 0x80)); // Wait for crystal osc to settle
CLKSEL = 0x01; // Select external crystal as SYSCLK
// source
SFRPAGE = SFRPAGE_SAVE; // Restore SFRPAGE
}
//-----------------------------------------------------------------------------
// PORT_Init_UART0
//-----------------------------------------------------------------------------
//
// Return Value : None
// Parameters : None
//
// This function configures the crossbar and GPIO ports.
//
// Pinout:
//
// P0.0 digital push-pull UART TX
// P0.1 digital open-drain UART RX
// P1.6 digital push-pull LED
//-----------------------------------------------------------------------------
void PORT_Init_UART0 (void)
{
char SFRPAGE_SAVE = SFRPAGE; // Save Current SFR page
SFRPAGE = CONFIG_PAGE; // Set SFR page
XBR0 = 0x04; // Enable UART0
XBR1 = 0x00;
XBR2 = 0x40; // Enable crossbar and weak pull-up
P0MDOUT |= 0x01; // Set TX pin to push-pull
P1MDOUT |= 0x40; // Set P1.6(LED) to push-pull
SFRPAGE = SFRPAGE_SAVE; // Restore SFR page
}
//-----------------------------------------------------------------------------
// PORT_Init_UART1
//-----------------------------------------------------------------------------
//
// Return Value : None
// Parameters : None
//
// This function configures the crossbar and GPIO ports.
//
// Pinout:
//
// P0.0 digital push-pull UART TX
// P0.1 digital open-drain UART RX
// P1.6 digital push-pull LED
//-----------------------------------------------------------------------------
void PORT_Init_UART1 (void)
{
char SFRPAGE_SAVE = SFRPAGE; // Save Current SFR page
SFRPAGE = CONFIG_PAGE; // Set SFR page
XBR0 = 0x00;
XBR1 = 0x00;
XBR2 = 0x44; // Enable crossbar and weak pull-up
// Enable UART1
P0MDOUT |= 0x01; // Set TX pin to push-pull
P1MDOUT |= 0x40; // Set P1.6(LED) to push-pull
SFRPAGE = SFRPAGE_SAVE; // Restore SFR page
}
//-----------------------------------------------------------------------------
// UART0_Init Variable baud rate, Timer 2, 8-N-1
//-----------------------------------------------------------------------------
//
// Return Value : None
// Parameters : None
//
// Configure UART0 for operation at <BAUDRATE> 8-N-1 using Timer2 as
// baud rate source.
//
//-------------------------------------------
UART.rar_C8051F040_C8051F040 uart1_C8051f040 Uart0 _c8051f040 UA
版权申诉
36 浏览量
2022-09-14
20:49:12
上传
评论
收藏 8KB RAR 举报

weixin_42653672
- 粉丝: 93
- 资源: 1万+
最新资源
- Screenshot_2024-05-21-17-06-42-64_2332cb9b27b851b548ba47a91682926c.jpg
- 毕业设计参考 - 基于树莓派、OpenCV及Python的人脸识别
- node-v18.20.2-linux-arm64
- 222222222222
- 16张相机标定图片,可复现本文畸变矫正
- dbeaver-ce-23.3.1-x86_64-setup.zip
- 基于X86 AVX2指令的快速卷积实现
- VMware-ESXi-7.0U3n-21930508-depot.zip文件
- MySQL 在 Windows 系统下的安装教程.zip
- Access文件数据库访问的客户端
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


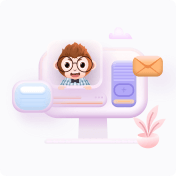