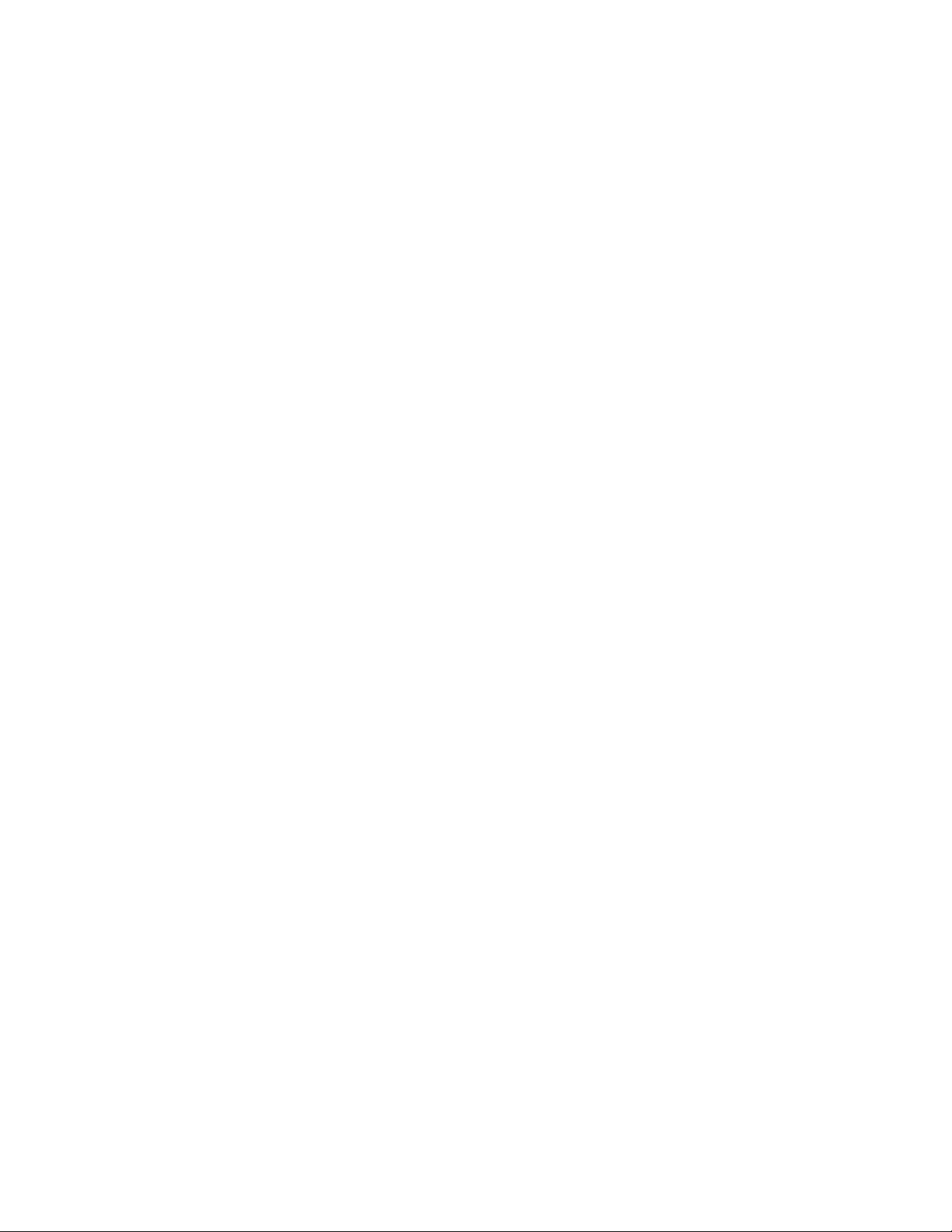
The shell file provides the public interface for the device objects by duplicating a function for each method
of the device object. The duplicated function call has the same parameter list as the method with the
addition of a device object array index parameter. In each case the function body becomes a de-reference of
the objects method. For example the interface shell would define a function as :
BOOL Function1(device_index, parameter1, parameter2)
for the object method:
BOOL device::Function1(parameter1, parameter2)
The function body in the interface shell would be :
{
device_array[device_index]->Function1(parameter1,parameter2)
}
In order to use this API, a calling application would first call StartPassServer, which starts the PASS 3200
server application if it is not already running and maps the shared memory mapped file into the calling
applications process space. The calling application would then create the device object for each SBS device
it wishes to access by calling the InitBoard function in the interface shell. The parameters of this call are
the physical index of the card (i.e. the order it appears in the registry) and the logical index. The logical
index is then used by the calling application for each subsequent call to indicate to which board the call is
directed. The indexes are unique for the particular instance of the DLL to allow individual calling
applications to access any subset of the existing boards.
In general, each of the interface shell functions will first check to see if device index is associated with a
device object. It then checks to see if the device is functioning. Finally the device function is called. If
either of these tests fail the shell function will return False. If the function is actually called, the shell
function will return the value returned by the device function.
Variables that need to be shared by all applications using a given board such as ownership information and
current state flags are kept in the memory-mapped file. A single server application handles a global timer
for performing data transfers that are on a set time schedule such as the 1553 count tables.
I. System Initialization Functions
1) USHORT StartPassServer(void)
On first call, starts server app and global timer, initializes memory mapped file used for global
memory, and retrieves current available board information from the registry and stores this
information in the memory mapped file. Returns the number of logical PASS boards available.
This call should be made before opening any devices with the InitBoard call.
2) BOOL ReturnDeviceIds( PULONG deviceIds)
Acts like StartPassServer to initialize server and memory mapped file. Returns in deviceIds a
linked list of device ids created by combining board type and setting information. These ids are
unique for each board. This function is used by DataXpress.
3) BOOL InitBoard(USHORT logical_index, USHORT physical_index)
Checks state of board, if the hardware is not initialized it performs the initialization including
performing power-up tests and loading firmware. Builds internal object for the board specified by
the physical index parameter. This object is placed in the DLL's object array at the index specified
by logical_index. This logical index is then used in all subsequent calls to access the board. If the
initialization is successful the function returns TRUE. If the initialization fails at any point, the
function returns FALSE.