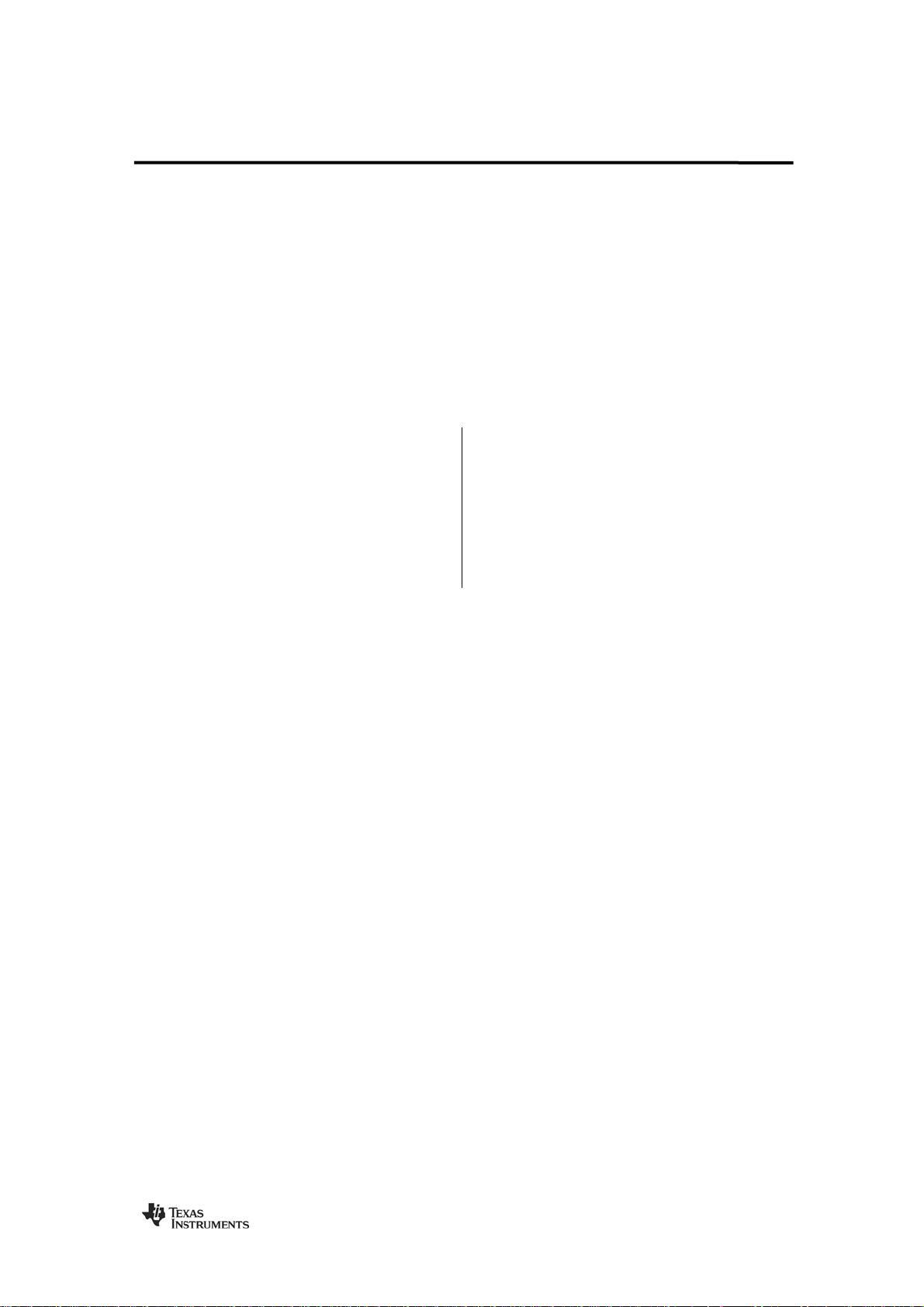
Design Note
DN112
SWRA222A Page 1 of 28
Using UART in CC111xFx, CC2431x, and CC251xFx
By Torgeir Sundet
Keywords
• UART
• CPU
• DMA
• Protocol
• Baud Rate
• Asynchronous
• CC1110Fx
• CC1111Fx
• CC2430Fx
• CC2431Fx
• CC2510Fx
• CC2511Fx
1 Introduction
This design note describes the key
elements and simple usage of the
CC111xFx/CC243x/CC251xFx USART
peripheral in UART mode. The main
objective is to explain the
CC111xFx/CC243x/CC251xFx software
required to operate the UART, with and
without DMA support.
The UART implements asynchronous
serial communication, and is typically used
as a diagnostic/command interface. In the
following sections an x represents USART
number 0 or 1 if nothing else is stated.
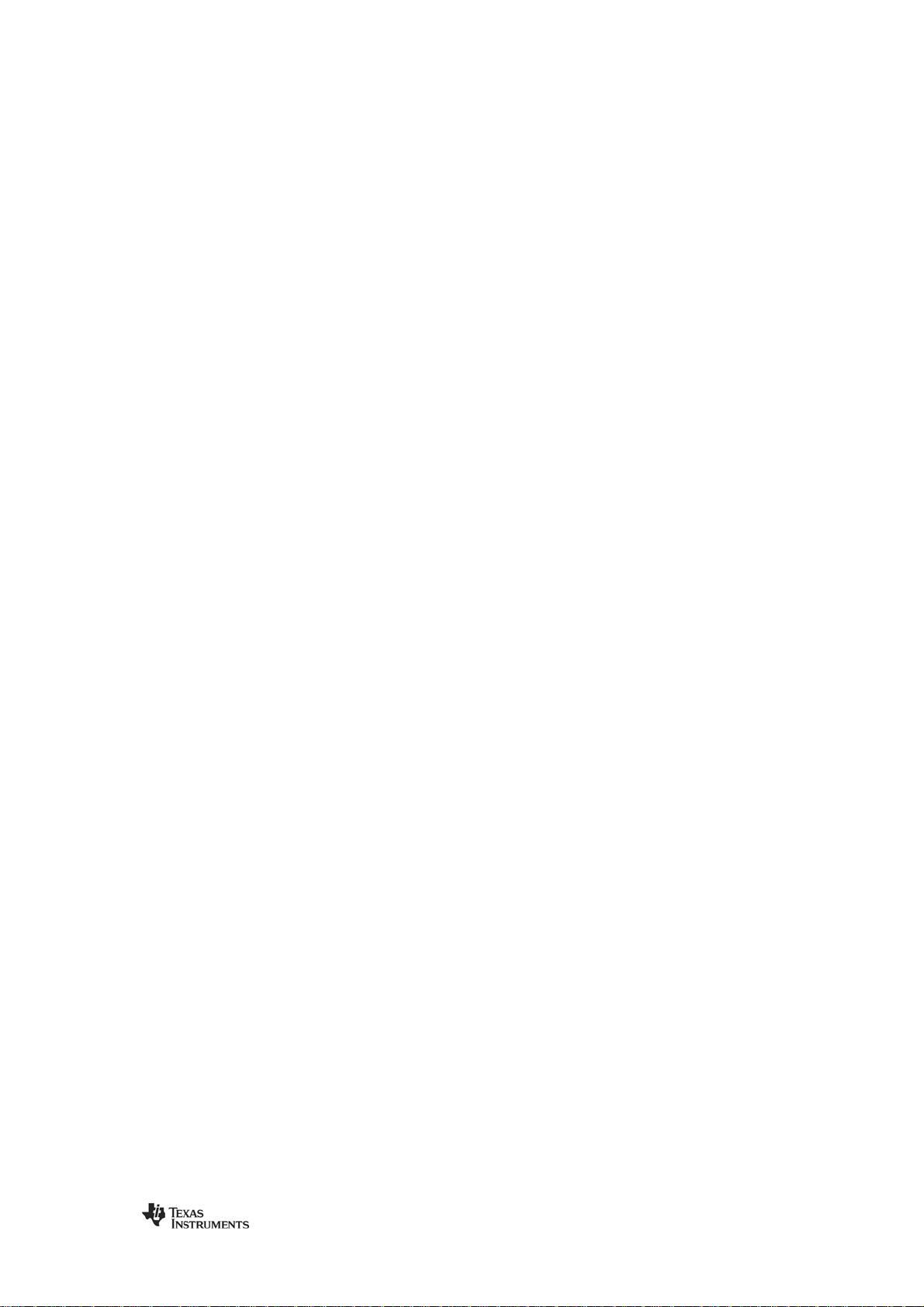
Design Note
DN112
SWRA222A Page 2 of 28
Table of Contents
KEYWORDS.............................................................................................................................. 1
1 INTRODUCTION............................................................................................................. 1
2 ABBREVIATIONS........................................................................................................... 2
3 SCOPE............................................................................................................................ 3
4 USING THE USART PERIPHERAL IN UART MODE ................................................... 3
4.1 MAPPING THE USART PERIPHERAL IN UART MODE ................................................... 4
4.1.1 Mapping the UART to the SoC I/O...................................................................................6
4.1.2 Interfacing the UART with the SmartRF
®
04EB................................................................8
4.1.3 Setting up the UART Protocol ..........................................................................................9
4.2 USING THE UART WITHOUT DMA SUPPORT.............................................................. 16
4.2.1 Processing UART Communication using UART Polling................................................16
4.2.2 Processing UART Communication using UART ISR......................................................18
4.3 USING THE UART WITH DMA SUPPORT.................................................................... 22
4.3.1 Allocating DMA Descriptor for UART RX/TX ...............................................................22
4.3.2 Processing UART Communication with DMA Support..................................................23
5 REFERENCES.............................................................................................................. 27
6 GENERAL INFORMATION .......................................................................................... 28
6.1 DOCUMENT HISTORY................................................................................................ 28
2 Abbreviations
EB Evaluation Board
CPU Central Processing Unit
CTS Clear To Send
DMA Direct Memory Access
DCE Data Circuit-terminating Equipment, according to [4].
DTE Data Terminal Equipment, according to [4].
GPIO General Purpose Input and Output
HS XOSC High Speed Xtal Oscillator, refer to relevant data sheets [1], [2], and
[3] for actual frequency.
HW Hard Ware
ISR Interrupt Service Routine
NA Not Applicable
NOP No Operation
SmartRF
®
Registered Trademark, used in this document to reference the Low
Power RF product line from Texas Instruments.
SoC System on Chip. A collective term used to refer to Texas
Instruments ICs with on-chip MCU and RF transceiver. Used in this
document to reference the CC1110, CC1111, CC2430, CC2431,
CC2510 and CC2511.
RF Radio Frequency.
RTS Ready To Send (implies Ready to Receive for DTE to DTE
communication)
RX Receive. Used in this document to reference UART/Radio receive.
RS232 Recommended Standard 232, a standard defining the format and
signal levels of a specific asynchronous serial interface.
TTL Transistor-transistor Logic
TX Transmit. Used in this document to reference UART/Radio transmit.
UART Universal Asynchronous serial Receiver and Transmitter
UART protocol Used in this document to represent the UART signalling format;
start/stop bit, data bit, parity.
USART Universal Synchronous/Asynchronous serial Receiver and
Transmitter.
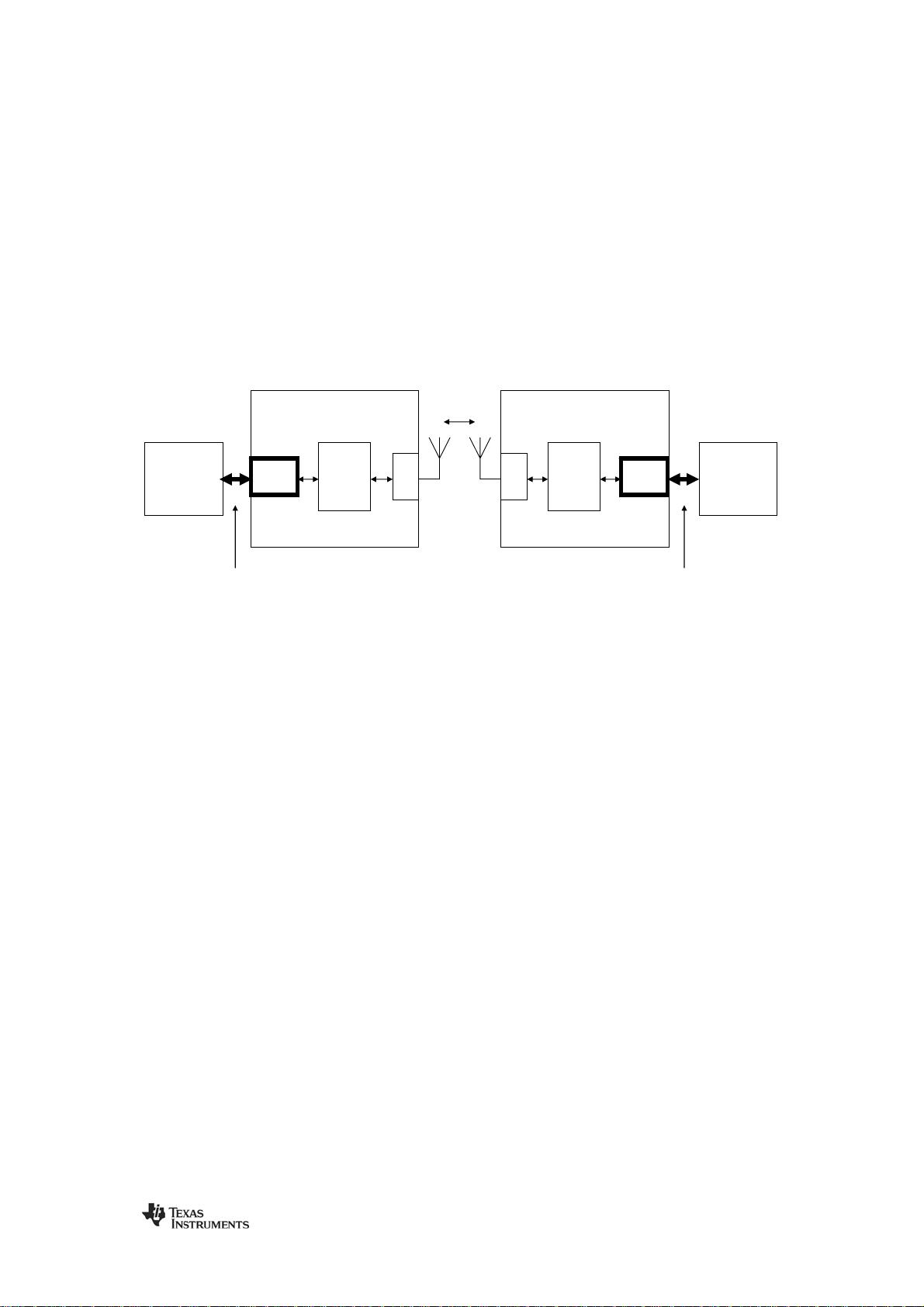
Design Note
DN112
SWRA222A Page 3 of 28
3 Scope
In UART mode the SoC USART peripheral can be used to interface any external device
(assuming TTL voltage level) which supports the UART protocol, meaning half/full-duplex
asynchronous serial transfer of 8 bit data. A common application would use the UART to
support a wireless modem, as shown in Figure 1. However, this design note focuses on the
fundamental UART receive/transmit operation, and how to use the different protocol settings,
including start/stop bit and parity.
Two main UART transmit/receive methods are described; using the UART with DMA support,
and using it without DMA support. Furthermore, when using the UART without DMA support,
two separate implementations are covered; UART transmit/receive by polling the UART
Interrupt Request Flags, and transmit/receive using UART ISR.
SoC
UART
CPU
DMA
RAM
UARTRF
UART Signals:
RX, TX, RTS, CTS
Hyperterminal
(e.g. PC)
SoC
CPU
DMA
RAM
RF
Hyperterminal
(e.g. PC)
Key UART Features:
• Designated SoC peripheral
• Wired Asynchronous Serial Bi-directional Communication
• Frame Recognition via Start/Stop bit
• Data Level Recognition via Over-sampling => No Clock
• Optional Data Flow Control - Hardware handshaking
• Optional Data Integrity Control – Parity Check
UART Signals:
RX, TX, RTS, CTS
Figure 1: Typical Application with UART Support
4 Using the USART Peripheral in UART Mode
The UART peripheral uses UxCSR.MODE to determine the desired USART operation mode
(UART or SPI) and the UxUCR/UxGCR registers to configure the UART format/signaling;
start/stop bit, data bit and parity. For data transfer on the UART interface the UxDBUF
registers are used. Internal data transfer, between the SoC memory and UART can be done
by the CPU or the DMA controller.
Using the CPU for internal data transfer prevents the CPU from performing other tasks
(except from ISRs) during memory transfer. Using the DMA controller allows the CPU to
continue with other tasks, while the DMA controller transfers data between the UART and
SoC memory. In a UART application it is typically desired that the DMA controller handles
block transfers, not just transfers of a single byte. This means that the DMA controller must
be configured to interface allocated RX/TX buffers and either download data (transmit) from
the allocated TX buffer to the UxDBUF, or upload data (receive) from the UxDBUF to the
allocated RX buffer. A DMA ISR can typically be implemented to automatically start a new
UART transmit/receive session upon completing each block transfer. This would be relevant
when the application needs efficient streaming of data between e.g. RF and UART.
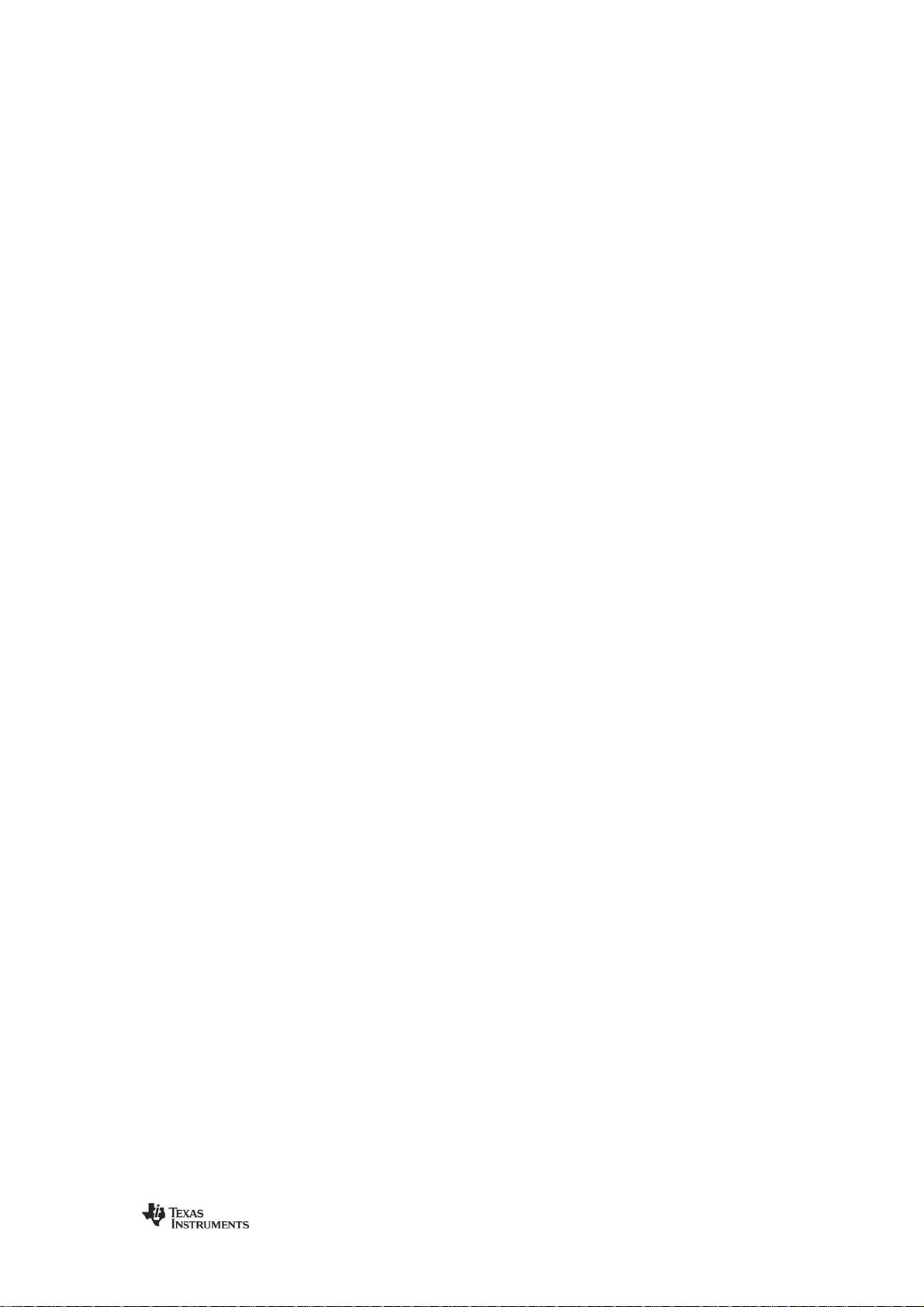
Design Note
DN112
SWRA222A Page 4 of 28
4.1 Mapping the USART Peripheral in UART Mode
In order for the UART to be mapped to the desired pins and generate the associated interrupt
requests and DMA triggers, the following register/descriptor fields must be set according to
the desired configuration/functionality (for cross referencing, please see Figure 2):
• PxSEL.SELPx_y (see Figure 2 for correlation of x and y)
Selects whether Port x Pin y shall be GPIO or mapped to the UART.
• IEN2.UTXxIE
UART TX CPU interrupt enable/disable.
• IEN0.URXxIE
UART RX CPU interrupt enable/disable.
• IEN0.EA
Global/master interrupt enable/disable.
• IEN1.DMAIE
DMA CPU interrupt enable/disable.
• DMA Descriptor.IRQMASK
DMA Transfer Complete interrupt enable/disable.
The corresponding UART / DMA interrupt flags are located in the register fields listed below.
If the corresponding interrupt enable flags have been set, then an interrupt request causes
the CPU to vector its code execution to the associated UART / DMA ISR (for cross
referencing, please see Figure 2):
• UxCSR.TX_BYTE and IRCON2.UTXxIF
Indicates that the UART has transmitted a byte, and that it is ready to transmit
another byte.
• UxCSR.RX_BYTE and TCON.URXxIF
Indicates that the UART has received a byte, and that it is ready to receive another
byte.
• IRCON.DMAIF and DMAIRQ.DMAIFn
Indicates that the DMA controller has reached its transfer count; that is; it has
transferred a defined range of data between the UART and SoC memory. As
generally noted above, if the corresponding DMA interrupt enable flags have been
set, the CPU would automatically vector its code execution to a DMA ISR, and thus
allow the CPU to prepare another block transfer between the UART and SoC
memory.
If supported by the DMA controller in UART TX then DMA trigger #15/17 will initiate a single
byte DMA transfer from the allocated UART TX source buffer to the UxDBUF register. For
UART RX DMA trigger #14/16 will initiate a single byte DMA transfer from the UxDBUF
register to the allocated UART RX destination buffer.
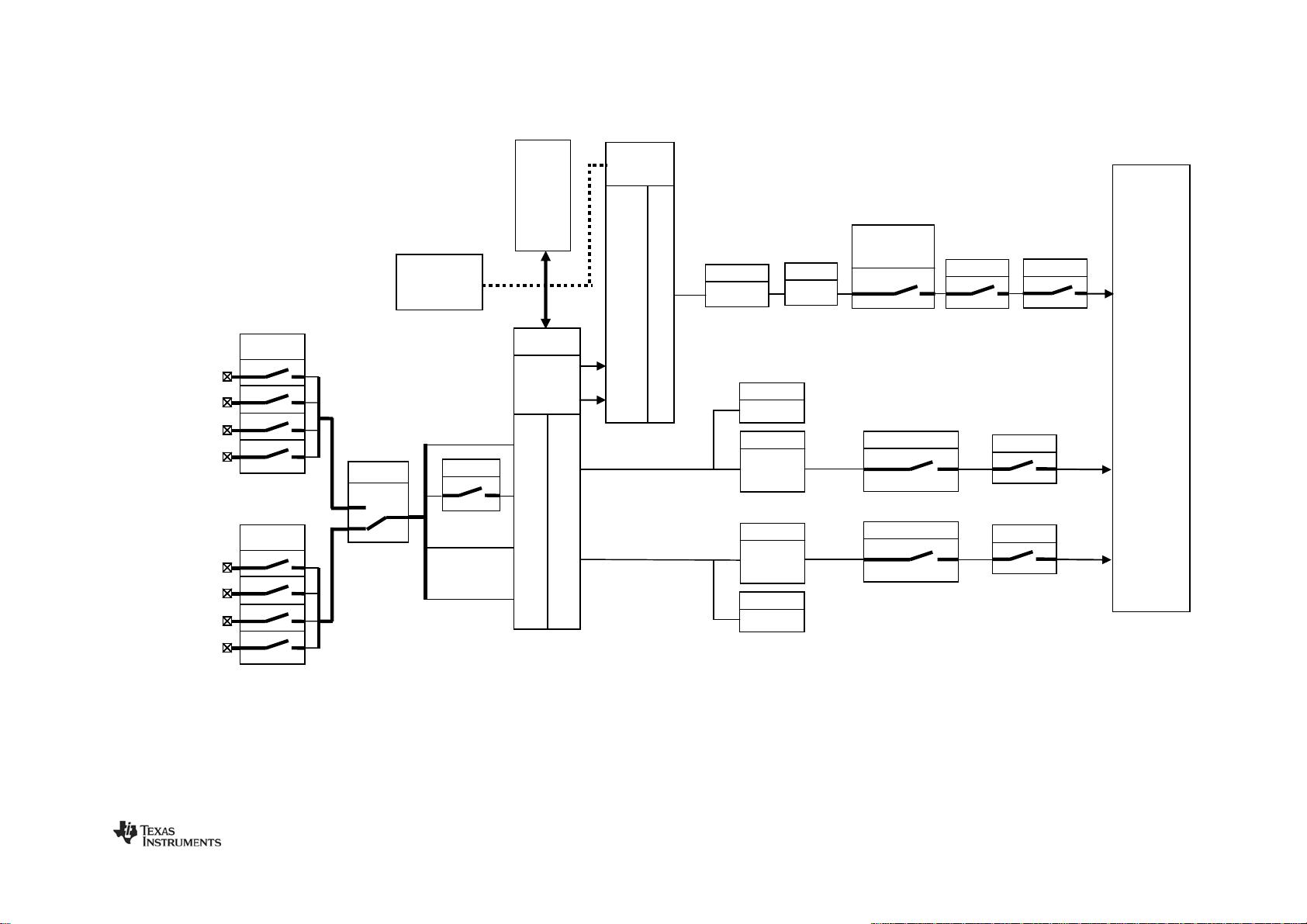
Design Note
DN112
SWRA222A Page 5 of 28
IEN0
P0SEL
SELP0 (a)
SELP0 (b)
P0.a (UARTx TX)
P0.b (UARTx RX)
USART
Peripheral
TX
RX
CT
RT
UTXx
x = 0 => V#7
x = 1 => V#14
IEN2
UTXxIE (y)
x = 0 => y = 2
x = 1 => y = 3
UART Interrupt Request Generation
IRCON2
UTXxIF (y)
x = 0 => y = 1
x = 1 => y = 2
EA (7)
PERCFG
1
CPU
Interrupt
Monitoring/
Processing
DMA
Controller
DMA Trigger
DMA Interrupt Request Generation
IEN0
DMA
(V#8)
IEN1
DMAIE (0)
DMA
Descriptor
IRQMASK
(Byte#7, Bit #3)
EA (7)
UARTx TX
x = 0 => #15
x = 1 => #17
UARTxRX
x = 0 => #14
x = 1 => #16
UxDBUF
IRCON
DMAIF (0)
DMAIRQ
DMAIFn (0-4)
SoC
Memory
CPU
RE (6)
UxCSR
UARTx Location Alternative 1:
x = 0 => a = 3, b = 2, c = 5, d = 4
x = 1 => a = 4, b = 5, c = 3, d = 2
SELP0 (c)
SELP0 (d)
P0.c (UARTx RT)
P0.d (UARTx CT)
IEN0
URXx
x = 0 => V#2
x = 1 => V#3
IEN0
URXxIE (y)
x = 0 => y = 2
x = 1 => y = 3
URXxIF (y)
x = 0 => y = 3
x = 1 => y = 7
EA (7)
UxCFG (x)
x = 0 or 1
0
P1SEL
SELP1 (a)
SELP1 (b)
P1.a (UARTx TX)
P1.b (UARTx RX)
SELP1 (c)
SELP1(d)
P1.c (UARTx RT)
P1.d (UARTx CT)
UARTx Location Alternative 2:
x = 0 => a = 5, b = 4, c = 3, d = 2
x = 1 => a = 6, b = 7, c = 5, d = 4
UxCSR
TX_BYTE (1)
TCON
UxCSR
RX_BYTE (2)
Figure 2: Mapping the USART Peripheral in UART Mode