#include "regs.h"
#include "stdarg.h"
void uart_init(void)
{
GPACON &= ~0xff;
GPACON |= 0x22;
ULCON0 = 0x3;
UCON0 |= (1 << 2) | (1 << 0);
UFCON0 = 0;
UMCON0 = 0;
UBRDIV0 = 34;
UDIVSLOT0 = 0xdfdd;
}
void mputchar(char c)
{
while(!(UTRSTAT0&0x2)){
;
}
UTXH0 = c;
if(c == '\n'){
mputchar('\r');
}
}
int mgetchar(void)
{
int c;
while(!(UTRSTAT0&0x1)){
;
}
c = URXH0;
if(c == '\r'){
c = '\n';
}
mputchar(c);
return c;
}
void mputs(char *str)
{
while(*str){
mputchar(*str++);
}
}
char *mgets(char *dst)
{
char *tmp = dst;
while((*dst++ = mgetchar()) != '\n'){
continue;
}
*(--dst) = '\0';
return tmp;
}
int mprintf(const char *fmt, ...)
{
va_list ap;
va_start(ap, fmt);
while(*fmt != '\0'){
if(*fmt == '%'){
fmt++;
}else{
mputchar(*fmt);
}
fmt++;
}
return 0;
}
uart.zip_UART driver ARM_linux uart
版权申诉

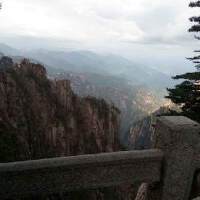
weixin_42651887
- 粉丝: 75
- 资源: 1万+