/*
* Misc utility routines for accessing chip-specific features
* of the SiliconBackplane-based Broadcom chips.
* Unless you and Broadcom execute a separate written software license
* agreement governing use of this software, this software is licensed to you
* under the terms of the GNU General Public License version 2 (the "GPL"),
* available at http://www.broadcom.com/licenses/GPLv2.php, with the
* following added to such license:
*
* As a special exception, the copyright holders of this software give you
* permission to link this software with independent modules, and to copy and
* distribute the resulting executable under terms of your choice, provided that
* you also meet, for each linked independent module, the terms and conditions of
* the license of that module. An independent module is a module which is not
* derived from this software. The special exception does not apply to any
* modifications of the software.
*
* Notwithstanding the above, under no circumstances may you combine this
* software in any way with any other Broadcom software provided under a license
* other than the GPL, without Broadcom's express prior written consent.
*
* $Id: siutils.c,v 1.662.4.4.4.16.4.28 2010/06/23 21:37:54 Exp $
*/
#include <typedefs.h>
#include <bcmdefs.h>
#include <osl.h>
#include <bcmutils.h>
#include <siutils.h>
#include <bcmdevs.h>
#include <hndsoc.h>
#include <sbchipc.h>
#include <pcicfg.h>
#include <sbpcmcia.h>
#include <sbsocram.h>
#include <bcmsdh.h>
#include <sdio.h>
#include <sbsdio.h>
#include <sbhnddma.h>
#include <sbsdpcmdev.h>
#include <bcmsdpcm.h>
#include <hndpmu.h>
#include "siutils_priv.h"
/* local prototypes */
static si_info_t *si_doattach(si_info_t *sii, uint devid, osl_t *osh, void *regs,
uint bustype, void *sdh, char **vars, uint *varsz);
static bool si_buscore_prep(si_info_t *sii, uint bustype, uint devid, void *sdh);
static bool si_buscore_setup(si_info_t *sii, chipcregs_t *cc, uint bustype, uint32 savewin,
uint *origidx, void *regs);
/* global variable to indicate reservation/release of gpio's */
static uint32 si_gpioreservation = 0;
static void *common_info_alloced = NULL;
/* global flag to prevent shared resources from being initialized multiple times in si_attach() */
/*
* Allocate a si handle.
* devid - pci device id (used to determine chip#)
* osh - opaque OS handle
* regs - virtual address of initial core registers
* bustype - pci/pcmcia/sb/sdio/etc
* vars - pointer to a pointer area for "environment" variables
* varsz - pointer to int to return the size of the vars
*/
si_t *
si_attach(uint devid, osl_t *osh, void *regs,
uint bustype, void *sdh, char **vars, uint *varsz)
{
si_info_t *sii;
/* alloc si_info_t */
if ((sii = MALLOC(osh, sizeof (si_info_t))) == NULL) {
SI_ERROR(("si_attach: malloc failed! malloced %d bytes\n", MALLOCED(osh)));
return (NULL);
}
if (si_doattach(sii, devid, osh, regs, bustype, sdh, vars, varsz) == NULL) {
if (NULL != sii->common_info)
MFREE(osh, sii->common_info, sizeof(si_common_info_t));
MFREE(osh, sii, sizeof(si_info_t));
return (NULL);
}
sii->vars = vars ? *vars : NULL;
sii->varsz = varsz ? *varsz : 0;
return (si_t *)sii;
}
/* global kernel resource */
static si_info_t ksii;
static uint32 wd_msticks; /* watchdog timer ticks normalized to ms */
/* generic kernel variant of si_attach() */
si_t *
si_kattach(osl_t *osh)
{
static bool ksii_attached = FALSE;
if (!ksii_attached) {
void *regs = REG_MAP(SI_ENUM_BASE, SI_CORE_SIZE);
if (si_doattach(&ksii, BCM4710_DEVICE_ID, osh, regs,
SI_BUS, NULL,
osh != SI_OSH ? &ksii.vars : NULL,
osh != SI_OSH ? &ksii.varsz : NULL) == NULL) {
if (NULL != ksii.common_info)
MFREE(osh, ksii.common_info, sizeof(si_common_info_t));
SI_ERROR(("si_kattach: si_doattach failed\n"));
REG_UNMAP(regs);
return NULL;
}
REG_UNMAP(regs);
/* save ticks normalized to ms for si_watchdog_ms() */
if (PMUCTL_ENAB(&ksii.pub)) {
/* based on 32KHz ILP clock */
wd_msticks = 32;
} else {
wd_msticks = ALP_CLOCK / 1000;
}
ksii_attached = TRUE;
SI_MSG(("si_kattach done. ccrev = %d, wd_msticks = %d\n",
ksii.pub.ccrev, wd_msticks));
}
return &ksii.pub;
}
static bool
si_buscore_prep(si_info_t *sii, uint bustype, uint devid, void *sdh)
{
/* need to set memseg flag for CF card first before any sb registers access */
if (BUSTYPE(bustype) == PCMCIA_BUS)
sii->memseg = TRUE;
if (BUSTYPE(bustype) == SDIO_BUS) {
int err;
uint8 clkset;
/* Try forcing SDIO core to do ALPAvail request only */
clkset = SBSDIO_FORCE_HW_CLKREQ_OFF | SBSDIO_ALP_AVAIL_REQ;
bcmsdh_cfg_write(sdh, SDIO_FUNC_1, SBSDIO_FUNC1_CHIPCLKCSR, clkset, &err);
if (!err) {
uint8 clkval;
/* If register supported, wait for ALPAvail and then force ALP */
clkval = bcmsdh_cfg_read(sdh, SDIO_FUNC_1, SBSDIO_FUNC1_CHIPCLKCSR, NULL);
if ((clkval & ~SBSDIO_AVBITS) == clkset) {
SPINWAIT(((clkval = bcmsdh_cfg_read(sdh, SDIO_FUNC_1,
SBSDIO_FUNC1_CHIPCLKCSR, NULL)), !SBSDIO_ALPAV(clkval)),
PMU_MAX_TRANSITION_DLY);
if (!SBSDIO_ALPAV(clkval)) {
SI_ERROR(("timeout on ALPAV wait, clkval 0x%02x\n",
clkval));
return FALSE;
}
clkset = SBSDIO_FORCE_HW_CLKREQ_OFF | SBSDIO_FORCE_ALP;
bcmsdh_cfg_write(sdh, SDIO_FUNC_1, SBSDIO_FUNC1_CHIPCLKCSR,
clkset, &err);
OSL_DELAY(65);
}
}
/* Also, disable the extra SDIO pull-ups */
bcmsdh_cfg_write(sdh, SDIO_FUNC_1, SBSDIO_FUNC1_SDIOPULLUP, 0, NULL);
}
return TRUE;
}
static bool
si_buscore_setup(si_info_t *sii, chipcregs_t *cc, uint bustype, uint32 savewin,
uint *origidx, void *regs)
{
bool pci, pcie;
uint i;
uint pciidx, pcieidx, pcirev, pcierev;
cc = si_setcoreidx(&sii->pub, SI_CC_IDX);
ASSERT((uintptr)cc);
/* get chipcommon rev */
sii->pub.ccrev = (int)si_corerev(&sii->pub);
/* get chipcommon chipstatus */
if (sii->pub.ccrev >= 11)
sii->pub.chipst = R_REG(sii->osh, &cc->chipstatus);
/* get chipcommon capabilites */
sii->pub.cccaps = R_REG(sii->osh, &cc->capabilities);
/* get pmu rev and caps */
if (sii->pub.cccaps & CC_CAP_PMU) {
sii->pub.pmucaps = R_REG(sii->osh, &cc->pmucapabilities);
sii->pub.pmurev = sii->pub.pmucaps & PCAP_REV_MASK;
}
SI_MSG(("Chipc: rev %d, caps 0x%x, chipst 0x%x pmurev %d, pmucaps 0x%x\n",
sii->pub.ccrev, sii->pub.cccaps, sii->pub.chipst, sii->pub.pmurev,
sii->pub.pmucaps));
/* figure out bus/orignal core idx */
sii->pub.buscoretype = NODEV_CORE_ID;
sii->pub.buscorerev = NOREV;
sii->pub.buscoreidx = BADIDX;
pci = pcie = FALSE;
pcirev = pcierev = NOREV;
pciidx = pcieidx = BADIDX;
for (i = 0; i < sii->numcores; i++) {
uint cid, crev;
si_setcoreidx(&sii->pub, i);
cid = si_coreid(&sii->pub);
crev = si_corerev(&sii->pub);
/* Display cores found */
SI_MSG(("CORE[%d]: id 0x%x rev %d base 0x%x regs 0x%p\n",
i, cid, crev, sii->common_info->coresba[i], sii->common_info->regs[i]));
if (BUSTYPE(bustype) == PCI_BUS) {
if (cid == PCI_CORE_ID) {
pciidx = i;
pcirev = crev;
pci = TRUE;
} else if (cid == PCIE_CORE_ID) {
pcieidx = i;
pcierev = crev;
pcie = TRUE;
}
} else if ((BUSTYPE(bustype) == PCMCIA_BUS) &&
(cid == PCMCIA_CORE_ID)) {
sii->pub.buscorerev = crev;
sii->pub.buscoretype = cid;
sii->pub.buscoreidx = i;
}
else if (((BUSTYPE(bustype) == SDIO_BUS) ||
(BUSTYPE(bustype) == SPI_BUS)) &&
((cid == PCMCIA_CORE_ID) ||
(cid == SDIOD_CORE_ID))) {
sii->pub.buscorerev = crev;
sii->pub.buscoretype = cid;
sii->pub.buscoreidx = i;
}
/* find the core idx before entering this func. */
if ((savewin && (savewin == sii->common_info->coresba[i])) ||
(regs == sii->common_info->regs[i]))
*origidx = i;
}
SI_MSG(("Buscore id/type/rev %d/0x%x/%d\n", sii->pub.buscoreidx, sii->pub.buscoret
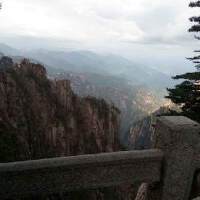
weixin_42651887
- 粉丝: 96
- 资源: 1万+
最新资源
- asp.net 原生js代码及HTML实现多文件分片上传功能(自定义上传文件大小、文件上传类型)
- whl@pip install pyaudio ERROR: Failed building wheel for pyaudio
- Constantsfd密钥和权限集合.kt
- 基于Java的财务报销管理系统后端开发源码
- 基于Python核心技术的cola项目设计源码介绍
- 基于Python及多语言集成的TSDT软件过程改进设计源码
- 基于Java语言的歌唱比赛评分系统设计源码
- 基于JavaEE技术的课程项目答辩源码设计——杨晔萌、李知林、岳圣杰、张俊范小组作品
- 基于Java原生安卓开发的蔚蓝档案娱乐应用设计源码
- 基于Java、Vue、JavaScript、CSS、HTML的毕设设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


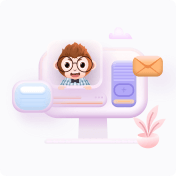