//----------------------------------------------------------------------------
// Description: This file contains functions that allow the MSP430 device to
// access the SPI interface of the CC1100/CC2500. There are multiple
// instances of each function; the one to be compiled is selected by the
// system variable TI_CC_RF_SER_INTF, defined in "TI_CC_hardware_board.h".
//
// MSP430/CC1100-2500 Interface Code Library v1.0
//
// K. Quiring
// Texas Instruments, Inc.
// July 2006
// IAR Embedded Workbench v3.41
//----------------------------------------------------------------------------
#include "include.h"
//----------------------------------------------------------------------------
// void TI_CC_SPISetup(void)
//
// DESCRIPTION:
// Configures the assigned interface to function as a SPI port and
// initializes it.
//----------------------------------------------------------------------------
// void TI_CC_SPIWriteReg(char addr, char value)
//
// DESCRIPTION:
// Writes "value" to a single configuration register at address "addr".
//----------------------------------------------------------------------------
// void TI_CC_SPIWriteBurstReg(char addr, char *buffer, char count)
//
// DESCRIPTION:
// Writes values to multiple configuration registers, the first register being
// at address "addr". First data byte is at "buffer", and both addr and
// buffer are incremented sequentially (within the CCxxxx and MSP430,
// respectively) until "count" writes have been performed.
//----------------------------------------------------------------------------
// char TI_CC_SPIReadReg(char addr)
//
// DESCRIPTION:
// Reads a single configuration register at address "addr" and returns the
// value read.
//----------------------------------------------------------------------------
// void TI_CC_SPIReadBurstReg(char addr, char *buffer, char count)
//
// DESCRIPTION:
// Reads multiple configuration registers, the first register being at address
// "addr". Values read are deposited sequentially starting at address
// "buffer", until "count" registers have been read.
//----------------------------------------------------------------------------
// char TI_CC_SPIReadStatus(char addr)
//
// DESCRIPTION:
// Special read function for reading status registers. Reads status register
// at register "addr" and returns the value read.
//----------------------------------------------------------------------------
// void TI_CC_SPIStrobe(char strobe)
//
// DESCRIPTION:
// Special write function for writing to command strobe registers. Writes
// to the strobe at address "addr".
//----------------------------------------------------------------------------
// Delay function. # of CPU cycles delayed is similar to "cycles". Specifically,
// it's ((cycles-15) % 6) + 15. Not exact, but gives a sense of the real-time
// delay. Also, if MCLK ~1MHz, "cycles" is similar to # of useconds delayed.
void TI_CC_Wait(unsigned int cycles)
{
while(cycles>15) // 15 cycles consumed by overhead
cycles = cycles - 6; // 6 cycles consumed each iteration
}
// SPI port functions
#if TI_CC_RF_SER_INTF == TI_CC_SER_INTF_USART0
void TI_CC_SPISetup(void)
{
TI_CC_CSn_PxOUT |= TI_CC_CSn_PIN; //#define TI_CC_CSn_PxOUT P5OUT
//#define TI_CC_CSn_PxDIR P5DIR
//#define TI_CC_CSn_PIN 0x01
TI_CC_CSn_PxDIR |= TI_CC_CSn_PIN; // /CS disable 用P5
ME1 |= USPIE0; // Enable USART0 SPI mode
UCTL0 |= CHAR + SYNC + MM; // 8-bit SPI Master **SWRST** 为什么后面不加上+SWRST
UTCTL0 |= CKPH + SSEL1 + SSEL0 + STC; // SMCLK, 3-pin mode
UBR00 = 0x02; // UCLK/2
UBR10 = 0x00; // 0
UMCTL0 = 0x00; // No modulation
TI_CC_SPI_USART0_PxSEL |= TI_CC_SPI_USART0_SIMO | TI_CC_SPI_USART0_SOMI | TI_CC_SPI_USART0_UCLK;
//#define TI_CC_SPI_USART0_PxSEL P3SEL
//#define TI_CC_SPI_USART0_SIMO 0x02
//#define TI_CC_SPI_USART0_SOMI 0x04
//#define TI_CC_SPI_USART0_UCLK 0x08
// SPI option select 用P3
TI_CC_SPI_USART0_PxDIR |= TI_CC_SPI_USART0_SIMO + TI_CC_SPI_USART0_UCLK;
// SPI TX out direction 设P3的输出
UCTL0 &= ~SWRST; // Initialize USART state machine
}
void TI_CC_SPIWriteReg(char addr, char value)
{
TI_CC_CSn_PxOUT &= ~TI_CC_CSn_PIN; // /CS enable用P5
while (TI_CC_SPI_USART0_PxIN&TI_CC_SPI_USART0_SOMI);// Wait for CCxxxx ready
//#define TI_CC_SPI_USART0_SOMI 0x04
//#define TI_CC_SPI_USART0_PxIN P3IN // chosen MSP430 device datasheet.
IFG1 &= ~URXIFG0; // Clear flag from first dummy byte
//#define IFG1_ (0x0002) // Interrupt Flag 1
//#define URXIFG0 (0x40)
U0TXBUF = addr; // Send address
while (!(IFG1&URXIFG0)); // Wait for TX to finish
IFG1 &= ~URXIFG0; // Clear flag from first dummy byte
U0TXBUF = value; // Send value
while (!(IFG1&URXIFG0)); // Wait for end of data TX
TI_CC_CSn_PxOUT |= TI_CC_CSn_PIN; // /CS disable
}
void TI_CC_SPIWriteBurstReg(char addr, char *buffer, char count)
{
char i;
TI_CC_CSn_PxOUT &= ~TI_CC_CSn_PIN; // /CS enable
while (TI_CC_SPI_USART0_PxIN&TI_CC_SPI_USART0_SOMI);// Wait for CCxxxx ready
U0TXBUF = addr | TI_CCxxx0_WRITE_BURST; // Send address TI_CCxxx0_WRITE_BURST=0x40就象协议规定的
//#define TI_CCxxx0_WRITE_BURST 0x40
while (!(IFG1&UTXIFG0)); // Wait for TX to finish
for (i = 0; i < count; i++)
{
U0TXBUF = buffer[i]; // Send data
while (!(IFG1&UTXIFG0)); // Wait for TX to finish
}
IFG1 &= ~URXIFG0;
while(!(IFG1&URXIFG0));
TI_CC_CSn_PxOUT |= TI_CC_CSn_PIN; // /CS disable
}
char TI_CC_SPIReadReg(char addr)
{
char x;
TI_CC_CSn_PxOUT &= ~TI_CC_CSn_PIN; // /CS enable
while (TI_CC_SPI_USART0_PxIN&TI_CC_SPI_USART0_SOMI);// Wait for CCxxxx ready
U0TXBUF = (addr | TI_CCxxx0_READ_SINGLE); // Send address
while (!(IFG1&URXIFG0)); // Wait for TX to finish
IFG1 &= ~URXIFG0; // Clear flag set during last write
U0TXBUF = 0; // Dummy write so we can read data
while (!(IFG1&URXIFG0)); // Wait for RX to finish
x = U0RXBUF; // Read data
TI_CC_CSn_PxOUT |= TI_CC_CSn_PIN; // /CS disable
return x;
}
void TI_CC_SPIReadBurstReg(char addr, char *buffer, char count)
{
unsigned int i;
TI_CC_CSn_PxOUT &= ~TI_CC_CSn_PIN; // /CS enable
while (TI_CC_SPI_USART0_PxIN&TI_CC_SPI_USART0_SOMI);// Wait for CCxxxx ready
IFG1 &= ~URXIFG0; // Clear flag
U0TXBUF = (addr | TI_CCxxx0_READ_BURST); // Send address TI_CCxxx0_READ_BURST=00x80
while (!(IFG1&UTXIFG0)); // Wait for TXBUF ready
U0TXBUF = 0; // Dummy write to read 1st data byte
// Addr byte is now being TX'ed, with dummy byte to follow immediately after
while (!(IFG1&URXIFG0)); // Wait for end of addr byte TX
IFG1 &= ~URXIFG0; // Clear flag
while (!(IFG1&URXIFG0)); // Wait for end of 1st data byte TX
// First data byte now in RXBUF
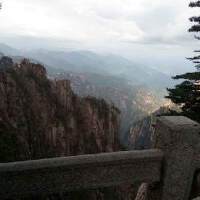
weixin_42651887
- 粉丝: 103
- 资源: 1万+
最新资源
- 阿尔法平台选择有批注(1-11).docx
- TA-Lib-0.4.28-cp311-cp311-win-amd64.whl
- 玄铁e907-r1s1用户手册-occ
- 阿尔法平台填空自测.pdf
- 匠芯创D13x芯片用户手册
- 阿尔法填空答案填空.pdf
- 匠芯创D13x硬件设计手册
- 阿尔法实验汇总.docx
- 匠芯创D13x数据手册
- 2024PPt资源02
- 手机拆螺丝机sw16可编辑全套技术资料100%好用.zip
- RISC-V 手册 中文版
- 四季除草机sw16可编辑全套技术资料100%好用.zip
- 水面垃圾自动收集装置sw18全套技术资料100%好用.zip
- 提砂机(砂水分离)sw18全套技术资料100%好用.zip
- 四柱油压机sw18可编辑全套技术资料100%好用.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


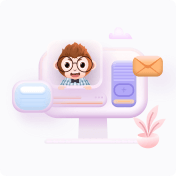