/*************************************************************
Function: phonebookeng.c
Description: sqlite3数据库操作接口函数集
Input:
Return:
Others:
*************************************************************/
#include <unistd.h>
#include <stdlib.h>
#include <stdio.h>
#include "phonebookeng.h"
#define CREATE_PHONEBOOK_DB "CREATE table phonebook(user_id integer primary key,contact_name text(64),mobile_phone text(32),email text(128),home_phone text(32),address text(128),company text(128));"
/*************************************************************
Function: open_phonebook_db
Description: 打开数据库文件,若不存在则创建
Input: 数据库文件名
Return: 数据库描述符
Others: 按CREATE_PHONEBOOK_DB方式创建数据库文件中的表
*************************************************************/
sqlite3 *open_phonebook_db(const char *db_file_path)
{
sqlite3 *db = NULL;
int rc = SQLITE_OK;
if(NULL == db_file_path)
{
return NULL;
}
if(access(db_file_path,F_OK) != 0){
rc = sqlite3_open(db_file_path,&db);
if(rc != SQLITE_OK){
perror("Error sqlite3_open");
exit(EXIT_FAILURE);
}
sqlite3_exec(db,CREATE_PHONEBOOK_DB,NULL,0,NULL);
}else{
rc = sqlite3_open(db_file_path,&db);
}
return db;
}
/*************************************************************
Function: add_contact
Description: 添加数据库表项
Input: 各个表项值
Return: 成功或各种错误如数据库错误 没成员名 没电话号码
Others:
*************************************************************/
int add_contact(sqlite3 *db, const char *contact_name,\
const char *mobile_phone, const char *home_phone,\
const char *email,const char *address,const char *company)
{
int ret = RESULT_OK;
char sql[1024] = {0};
if(NULL == db)
{
ret = RESULT_DB_ERROR;
return ret;
}
if(contact_name == NULL)
{
ret = RESULE_NO_CONTACT_NAME;
return ret;
}
if(mobile_phone == NULL && home_phone == NULL)
{
ret = RESULT_NO_PHONE_NUM;
return ret;
}
snprintf(sql,1024,"INSERT INTO phonebook VALUES(NULL,\'%s\',\'%s\',\'%s\',\'%s\',\'%s\',\'%s\');",contact_name,mobile_phone,home_phone,email,address,company);
printf("sql = %s-->\n",sql);
sqlite3_exec(db,sql,NULL,0,NULL);
return ret;
}
/*************************************************************
Function: query_all_contacts
Description: 显示所有数据库表项
Input: 数据库描述符
Return: 无
Others:
*************************************************************/
void query_all_contacts(sqlite3 *db)
{
int rc = SQLITE_OK;
char sql[1024] = {0};
char **azResult = NULL;
int n_row = 0;
int n_col = 0;
snprintf(sql,1024,"select * from phonebook;");
rc = sqlite3_get_table(db,sql,&azResult,&n_row,&n_col,NULL);
if(rc != SQLITE_OK){
printf("error on %s,%d----->\n",__FILE__,__LINE__);
}else{
int i = 0;
for(i=0; i < (n_row + 1) *n_col; ++i){
if(azResult[i] != NULL){
printf("azResult[%d]=%s\n",i,azResult[i]);
}
}
sqlite3_free_table(azResult);
}
}
/************************************************************
Function: get_contacts_List
Description: 从数据库中获得 contact_name mobile_phone 列表
Input: 数据库描述符 盛放获取结果的数组 结果的行列数
Return:
Others:
************************************************************/
void get_contacts_List(sqlite3 *db, char ***azResult, int *n_row, int *n_col)
{
int rc = SQLITE_OK;
char sql[1024] = {0};
snprintf(sql,1024,"select contact_name,mobile_phone from phonebook;");
rc = sqlite3_get_table(db,sql,azResult,n_row,n_col,NULL);
if(rc != SQLITE_OK)
{
printf("error on %s,%d----->\n",__FILE__,__LINE__);
}
}
/********************************************************
Function: get_contacts_by_name
Description: 从数据库中查找 指定contact_name 的表项
Input:
Return:
Others: 尚未实现模糊查询
********************************************************/
void get_contacts_by_name(sqlite3 *db, char ***azResult, int *n_row, int *n_col,char *name)
{
int rc = SQLITE_OK;
char sql[1024] = {0};
snprintf(sql,1024,"select contact_name,mobile_phone from phonebook where contact_name like:'%1%';",name);
// printf("error on %s,%d----->\n",__FILE__,__LINE__);
rc = sqlite3_get_table(db,sql,azResult,n_row,n_col,NULL);
if(rc != SQLITE_OK)
{
printf("error on %s,%d----->\n",__FILE__,__LINE__);
}
}
/*************************************************************
Function:
Description:
Input:
Return:
Others:
*************************************************************/
int edit_contact_name(sqlite3 *db,int contact_id,const char *name)
{
int rc = SQLITE_OK;
char sql[1024] = {0};
if(NULL == db){
rc = RESULT_DB_ERROR;
}
if(NULL == name){
rc = RESULT_DB_ERROR;
}
snprintf(sql,1024,"update phonebook set contact_name=\'%s\' where user_id = %d;",name,contact_id);
printf("sql = %s--->\n",sql);
rc = sqlite3_exec(db,sql,NULL,0,NULL);
return rc;
}
/*************************************************************
Function:
Description:
Input:
Return:
Others:
*************************************************************/
int delete_contact_by_id(sqlite3 *db, int contact_id)
{
int rc = SQLITE_OK;
char sql[1024] = {0};
snprintf(sql,1024,"delete from phonebook where user_id=%d;",contact_id);
rc =sqlite3_exec(db,sql,NULL,0,NULL);
return rc;
}
/*************************************************************
Function:
Description:
Input:
Return:
Others:
*************************************************************/
int close_phonebook_db(sqlite3 *db)
{
if(NULL == db)
{
return 0;
}
return sqlite3_close(db);
}
没有合适的资源?快使用搜索试试~ 我知道了~
pda.zip_maemo
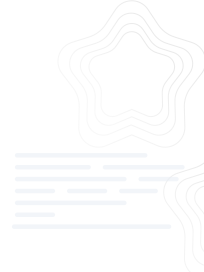
共34个文件
o:12个
cpp:11个
h:6个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 192 浏览量
2022-09-21
07:10:49
上传
评论
收藏 86KB ZIP 举报
温馨提示
MAEMO系统上QT4作为界面(GUI)开发工具,包括电话簿管理,通话记录管理等多个模块。使用目前嵌入式中流行的小巧但功能强大的数据库SQLITE3
资源推荐
资源详情
资源评论
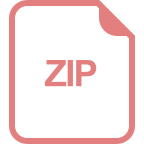
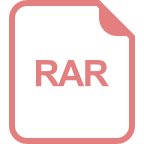
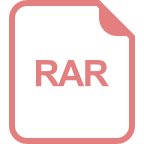
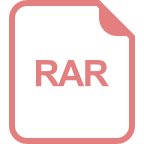
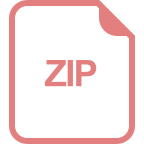
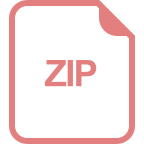
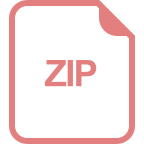
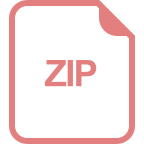
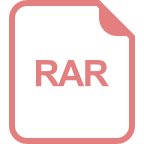
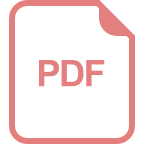
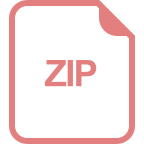
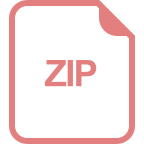
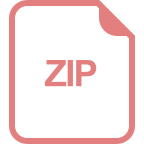
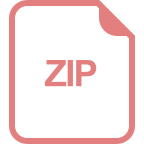
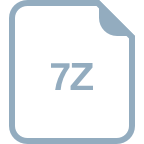
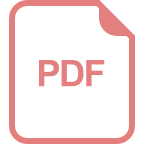
收起资源包目录


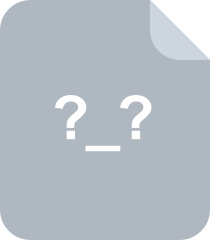
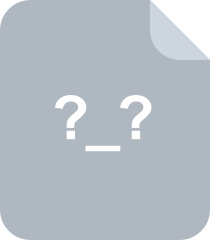
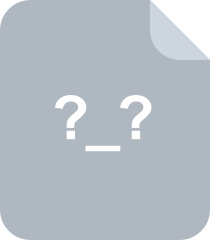
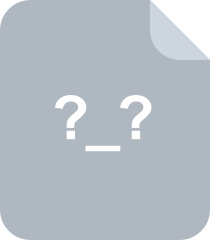
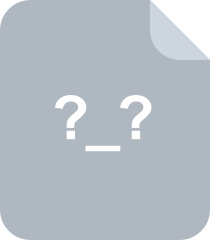
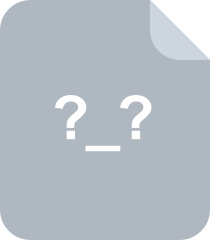
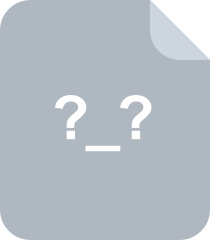
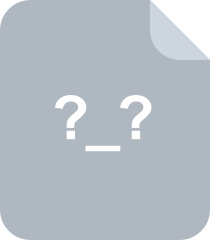
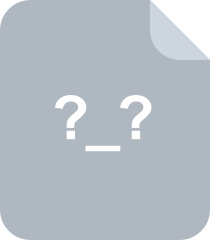

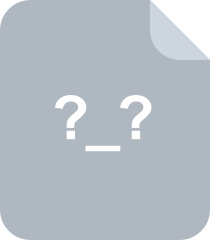
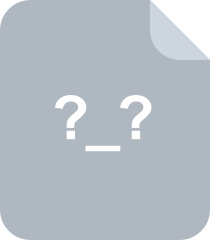
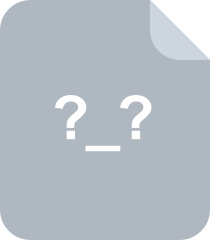
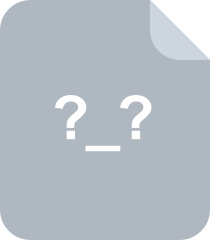


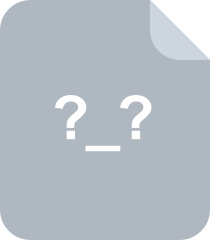
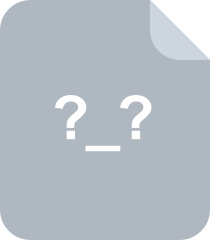
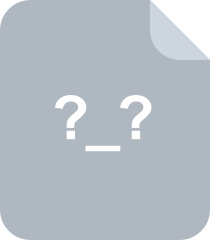
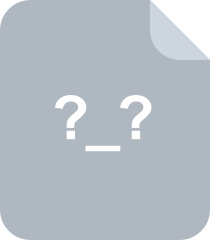
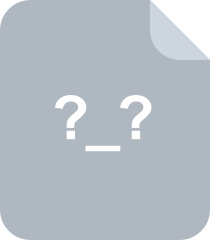
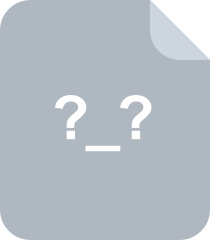

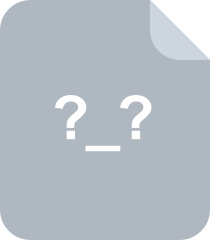
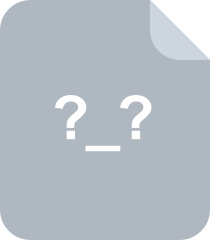
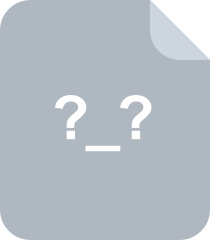
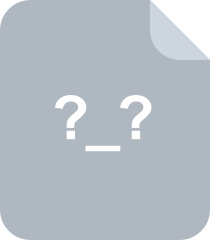
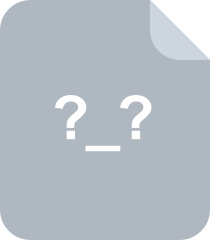
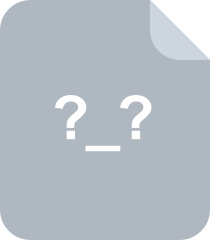
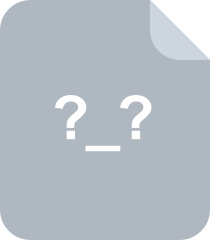
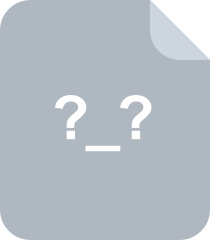
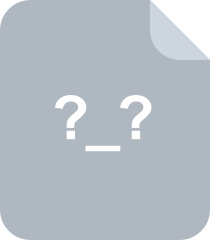
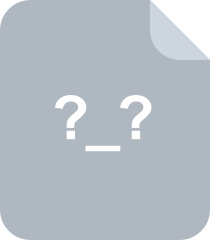
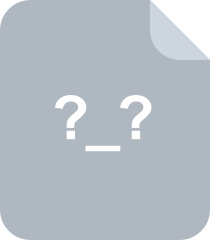
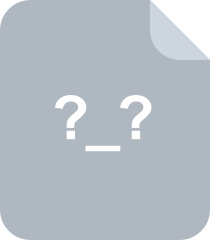
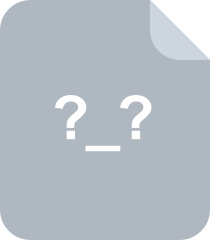
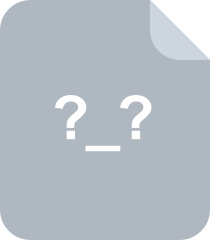
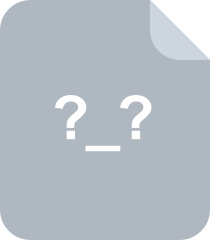
共 34 条
- 1
资源评论
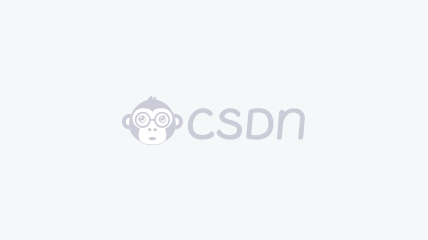
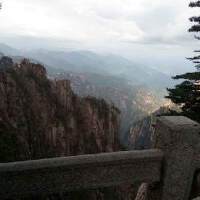
weixin_42651887
- 粉丝: 75
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

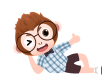
安全验证
文档复制为VIP权益,开通VIP直接复制
