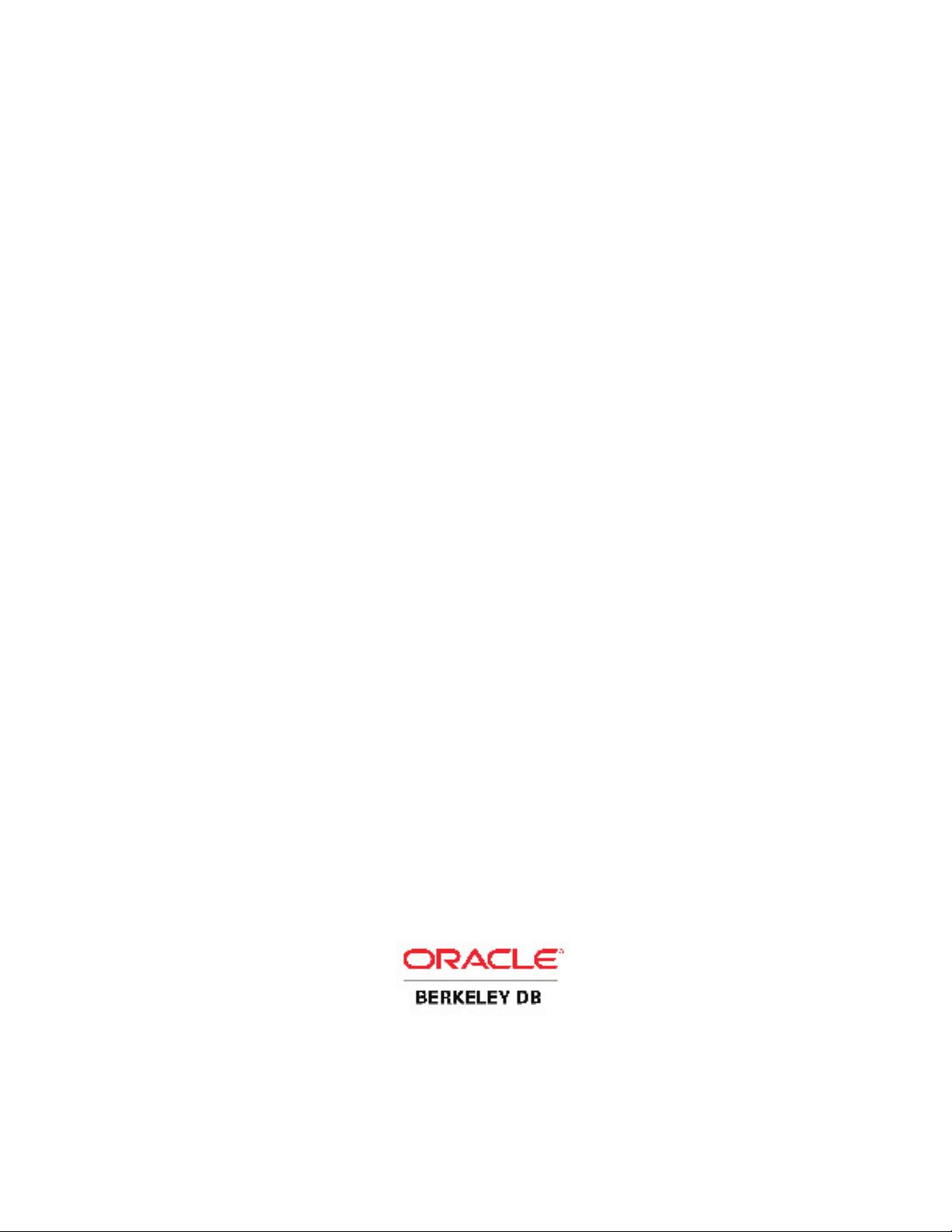
Oracle Berkeley DB
Getting Started with
Berkeley DB
for C++
Release 4.6
.
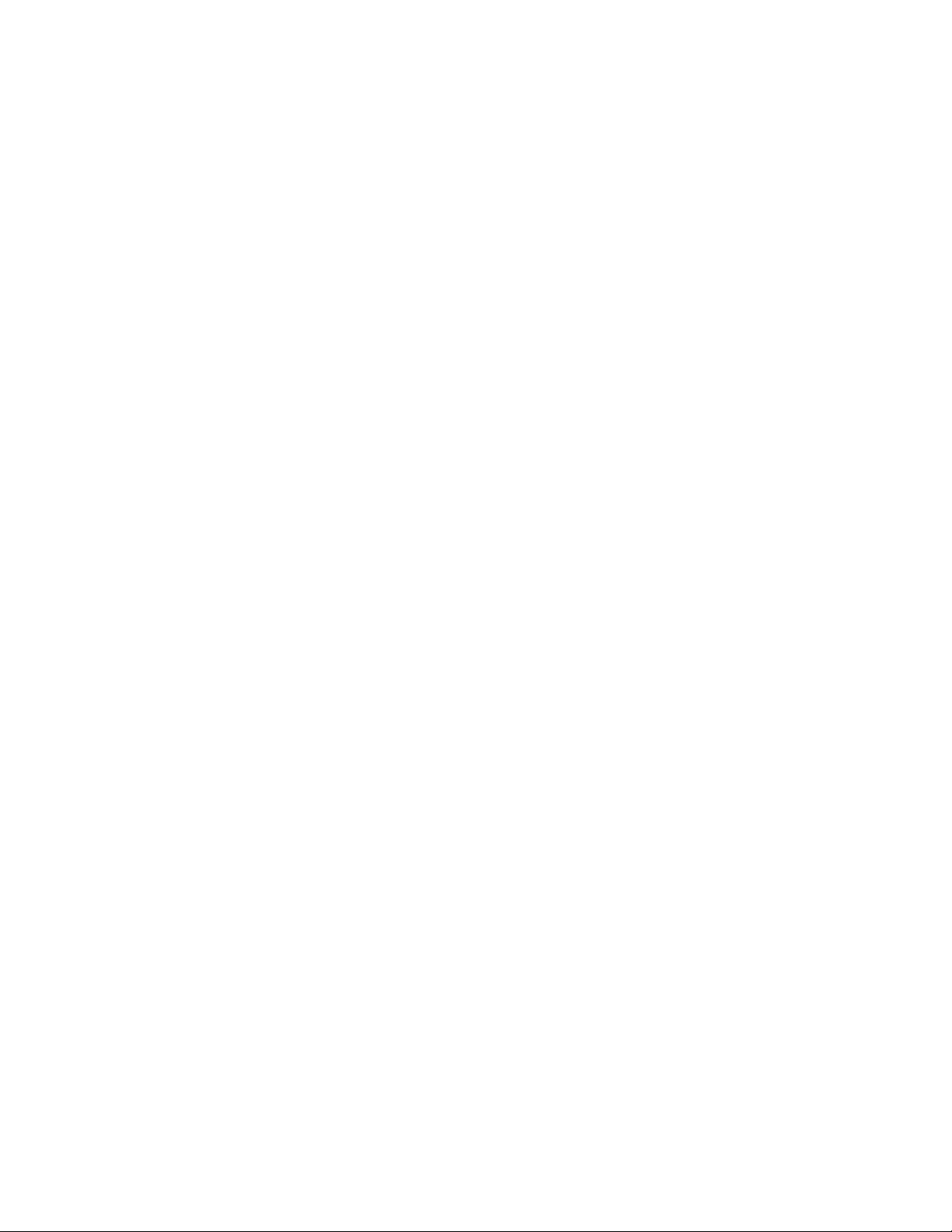
Legal Notice
This documentation is distributed under an open source license. You may review the terms of this license at:
http://www.oracle.com/technology/software/products/berkeley-db/htdocs/oslicense.html
Oracle, Berkeley DB, and Sleepycat are trademarks or registered trademarks of Oracle. All rights to these marks
are reserved. No third-party use is permitted without the express prior written consent of Oracle.
To obtain a copy of this document's original source code, please submit a request to the Oracle Technology
Network forum at: http://forums.oracle.com/forums/forum.jspa?forumID=271
Published 6/30/2007
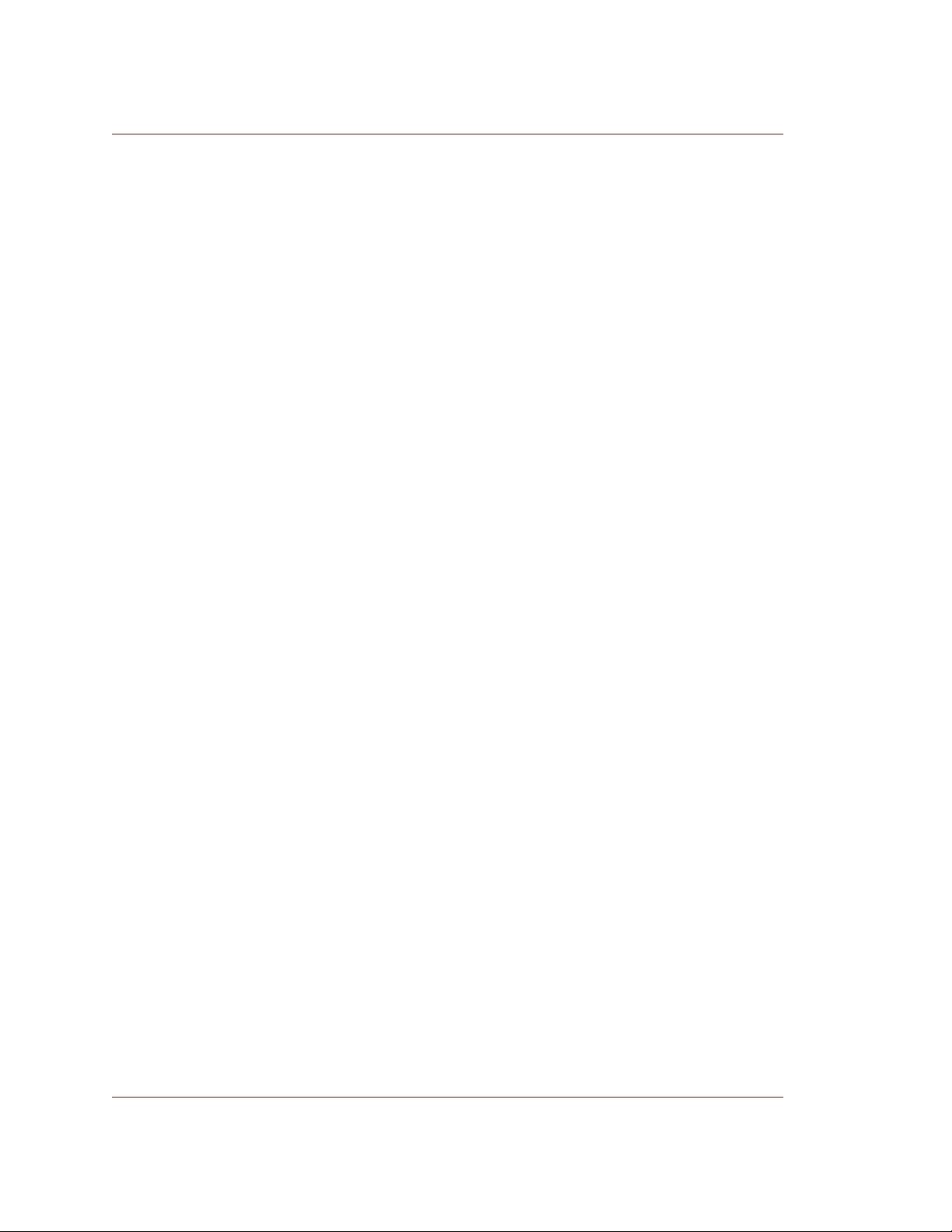
TableofContents
Preface .............................................................................................. iv
Conventions Used in this Book ............................................................. iv
For More Information .................................................................. v
1. Introduction to Berkeley DB .................................................................. 1
About This Manual ........................................................................... 2
Berkeley DB Concepts ....................................................................... 2
Access Methods ............................................................................... 4
Selecting Access Methods ............................................................ 4
Choosing between BTree and Hash ................................................. 5
Choosing between Queue and Recno ............................................... 5
Database Limits and Portability ............................................................ 6
Environments ................................................................................. 6
Exception Handling .......................................................................... 7
Error Returns .................................................................................. 8
Getting and Using DB ....................................................................... 8
2. Databases ......................................................................................... 9
Opening Databases ........................................................................... 9
Closing Databases ........................................................................... 10
Database Open Flags ....................................................................... 11
Administrative Methods .................................................................... 11
Error Reporting Functions ................................................................. 13
Managing Databases in Environments .................................................... 15
Database Example .......................................................................... 17
3. Database Records .............................................................................. 20
Using Database Records .................................................................... 20
Reading and Writing Database Records ................................................. 21
Writing Records to the Database .................................................. 21
Getting Records from the Database ............................................... 22
Deleting Records ..................................................................... 23
Data Persistence ..................................................................... 23
Database Usage Example .................................................................. 24
4. Using Cursors ................................................................................... 33
Opening and Closing Cursors .............................................................. 33
Getting Records Using the Cursor ........................................................ 34
Searching for Records ............................................................... 35
Working with Duplicate Records ................................................... 38
Putting Records Using Cursors ............................................................ 40
Deleting Records Using Cursors ........................................................... 42
Replacing Records Using Cursors ......................................................... 43
Cursor Example ............................................................................. 44
5. Secondary Databases .......................................................................... 49
Opening and Closing Secondary Databases ............................................. 50
Implementing Key Extractors ............................................................ 51
Working with Multiple Keys ......................................................... 52
Reading Secondary Databases ............................................................ 53
Deleting Secondary Database Records ................................................... 54
Page iiGetting Started with DB6/30/2007
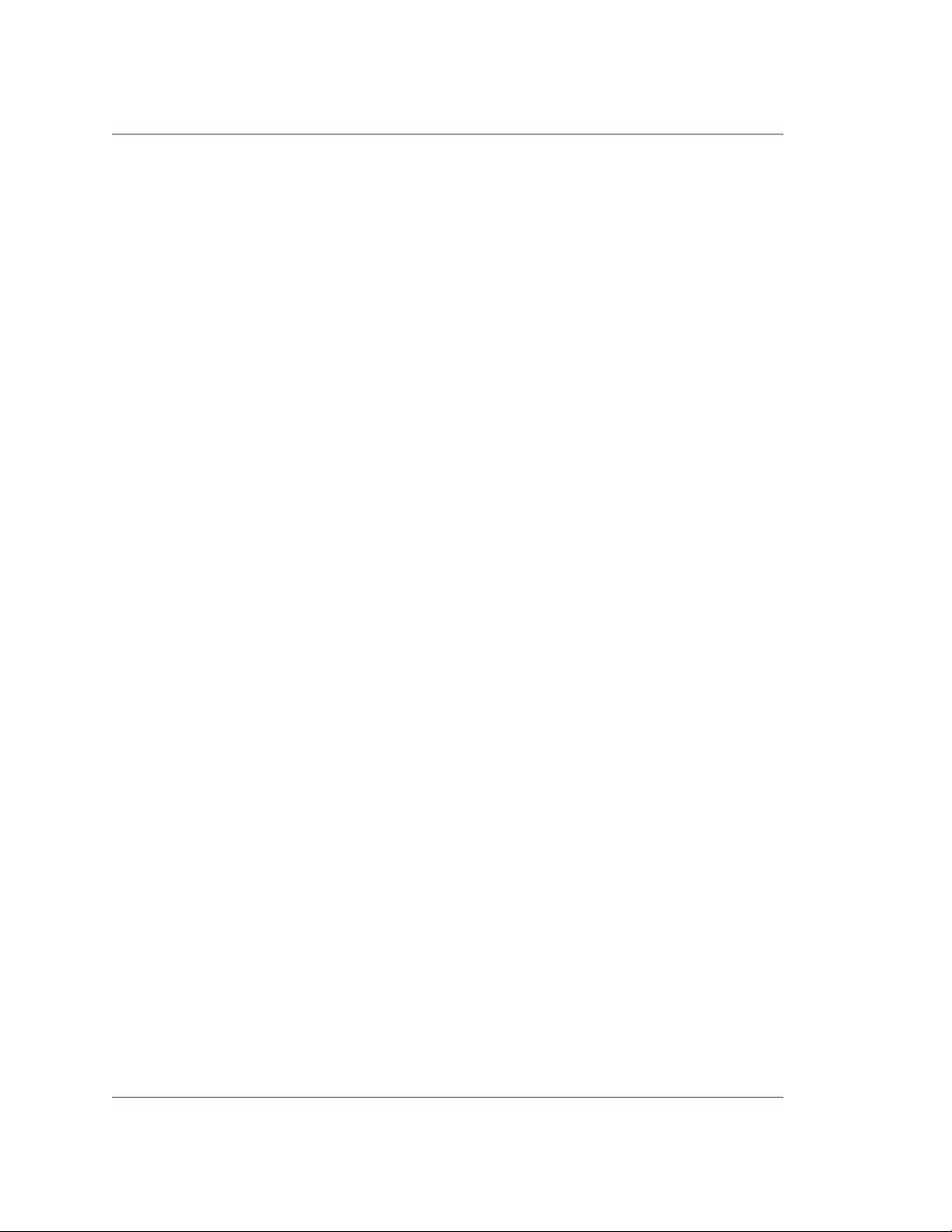
Using Cursors with Secondary Databases ............................................... 56
Database Joins .............................................................................. 57
Using Join Cursors .................................................................... 57
Secondary Database Example ............................................................. 59
Secondary Databases with example_database_load ............................ 60
Secondary Databases with example_database_read ............................ 64
6. Database Configuration ....................................................................... 68
Setting the Page Size ....................................................................... 68
Overflow Pages ....................................................................... 68
Locking ................................................................................. 69
IO Efficiency .......................................................................... 70
Page Sizing Advice ................................................................... 70
Selecting the Cache Size .................................................................. 71
BTree Configuration ........................................................................ 71
Allowing Duplicate Records ......................................................... 72
Sorted Duplicates .............................................................. 72
Unsorted Duplicates ........................................................... 72
Configuring a Database to Support Duplicates ............................ 73
Setting Comparison Functions ...................................................... 74
Creating Comparison Functions ............................................. 75
Page iiiGetting Started with DB6/30/2007
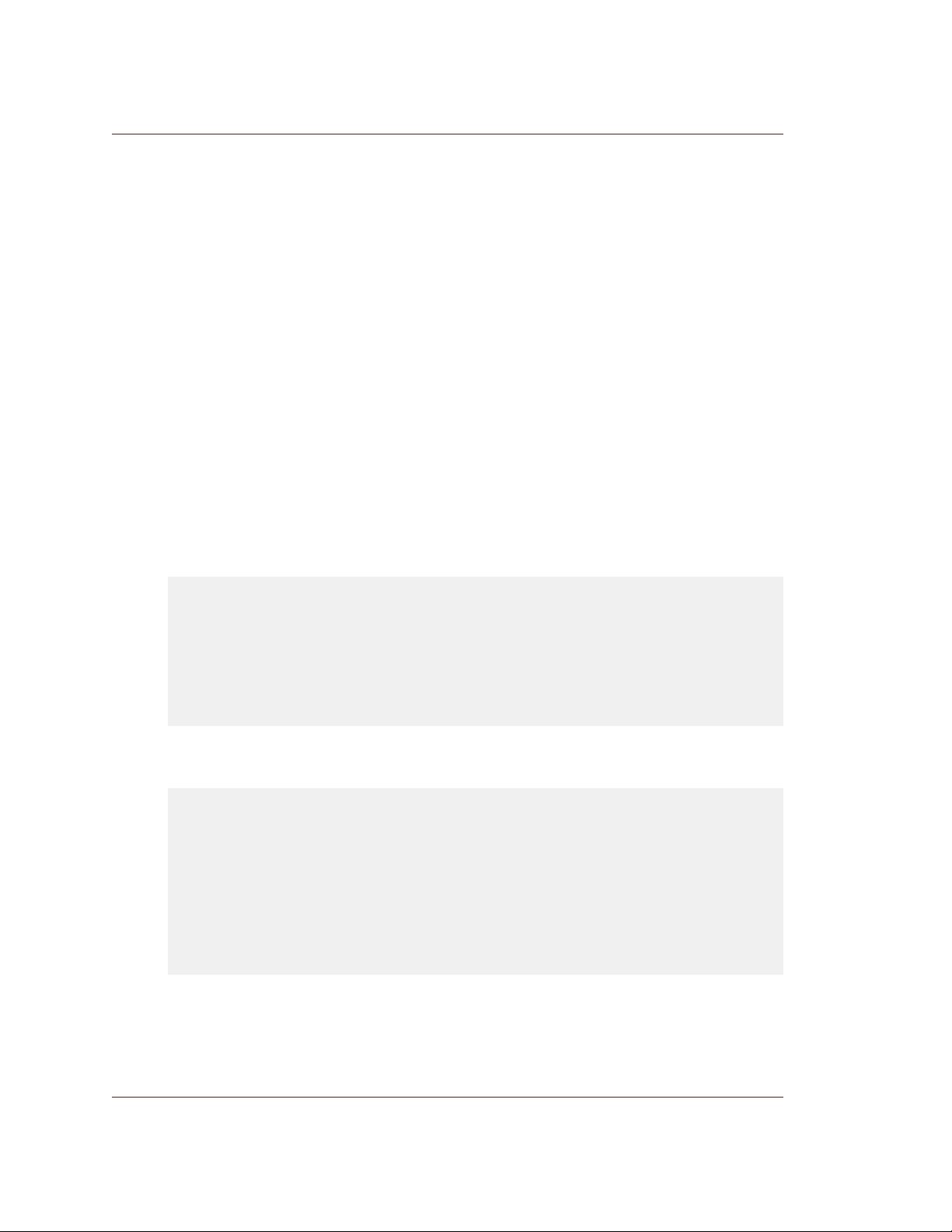
Preface
Welcome to Berkeley DB (DB). This document introduces DB, version 4.6. It is intended
to provide a rapid introduction to the DB API set and related concepts. The goal of this
document is to provide you with an efficient mechanism with which you can evaluate DB
against your project's technical requirements. As such, this document is intended for C++
developers and senior software architects who are looking for an in-process data
management solution. No prior experience with Berkeley DB is expected or required.
ConventionsUsedinthisBook
The following typographical conventions are used within in this manual:
Class names are represented in
monospaced font
, as are
method names
. For example:
"
Db::open()
is a
Db
class method."
Variable or non-literal text is presented in italics. For example: "Go to your DB_INSTALL
directory."
Program examples are displayed in a
monospaced font
on a shaded background. For
example:
typedef struct vendor {
char name[MAXFIELD]; // Vendor name
char street[MAXFIELD]; // Street name and number
char city[MAXFIELD]; // City
char state[3]; // Two-digit US state code
char zipcode[6]; // US zipcode
char phone_number[13]; // Vendor phone number
} VENDOR;
In some situations, programming examples are updated from one chapter to the next.
When this occurs, the new code is presented in
monospaced bold
font. For example:
typedef struct vendor {
char name[MAXFIELD]; // Vendor name
char street[MAXFIELD]; // Street name and number
char city[MAXFIELD]; // City
char state[3]; // Two-digit US state code
char zipcode[6]; // US zipcode
char phone_number[13]; // Vendor phone number
char sales_rep[MAXFIELD]; // Name of sales representative
char sales_rep_phone[MAXFIELD]; // Sales rep's phone number
} VENDOR;
Finally, notes of interest are represented using a note block such as this.
☞
Page ivGetting Started with DB6/30/2007