jntbtysdestructor.zip_duke_其他
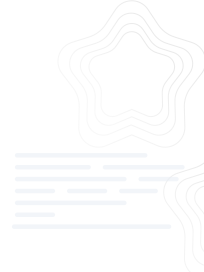

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
标题中的"jntbtysdestructor.zip_duke_其他"可能是一个被压缩的文件包,其中包含了由"duke"创建或与"duke"相关的某个项目或教程的源代码。"EM"在IT领域通常指的是 Expectation-Maximization(期望-最大化)算法,这是一种在概率模型中估计参数的常用方法,特别是当数据存在缺失或者是由多个未观察到的隐变量所驱动的时候。 描述中提到的"duke的tutorial on EM的matlab经典源码"表明这个压缩包可能是一个关于期望-最大化算法的MATLAB实现教程。MATLAB是一种强大的数值计算和数据分析环境,常用于科学计算、图像处理和机器学习等领域。这个教程可能包含了一系列的MATLAB脚本文件,帮助学习者理解和应用EM算法。 让我们逐一分析压缩包内的文件名,以提取更具体的知识点: 1. `em.m` - 这很可能是EM算法的核心实现代码。在MATLAB中,`.m`文件是函数或脚本文件,所以这个文件可能包含了完整的EM算法流程,包括初始化、期望步骤和最大化步骤。 2. `kmeans.m` - K-Means是另一种聚类算法,它在无监督学习中寻找数据的分组。在这个上下文中,它可能是作为EM算法的一个辅助工具,用于初始化聚类中心或者比较与EM聚类结果的差异。 3. `makeClusters.m` - 这个文件可能用于生成模拟数据集,以便在实际运行EM算法之前进行测试或演示。 4. `RUNME.m` - 这通常是主运行脚本,当你运行这个文件时,它会按照预设顺序执行其他所有相关脚本,展示整个流程。 5. `showClusters.m` - 这个函数可能用于可视化聚类结果,帮助用户直观地理解算法的输出。 6. `emi.m` - EMI可能代表"Expectation-Maximization Iteration",这是一个迭代过程,用以更新模型的参数直到收敛。 7. `zcheckSizes.m` - 这个函数可能是检查数据矩阵的大小,确保它们适合算法的要求。 8. `initClusters.m` - 另一个与聚类相关的函数,可能用于初始化EM算法中的聚类中心。 9. `gauss.m` - 在EM算法中,高斯分布常被用来建模隐变量的概率分布。这个文件可能是实现高斯分布函数的代码。 10. `rcolstd.m` - 这个函数名不常见,可能是一个内部使用的辅助函数,用于计算列的标准差,可能在标准化数据或调整变量尺度时使用。 通过这些文件,我们可以推测这个教程涵盖了从数据生成、预处理、模型训练到结果可视化的一系列步骤。学习者可以借此深入理解EM算法的工作原理,并通过实际操作来提升对算法的应用能力。MATLAB的脚本结构清晰,便于学习和调试,使得这个教程对初学者和有经验的开发者都有很高的价值。
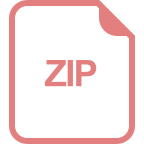
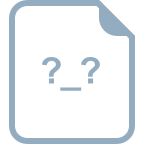
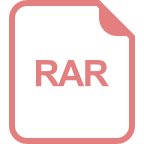
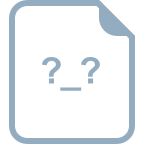
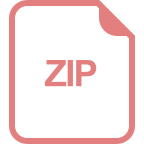
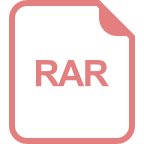
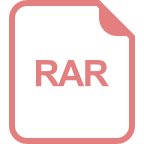
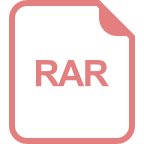
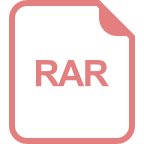
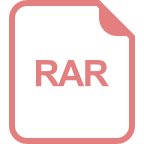
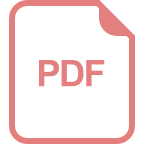
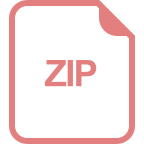
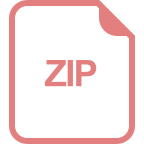
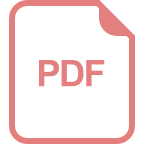

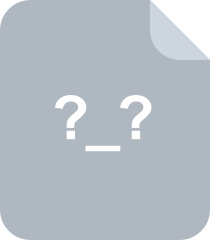
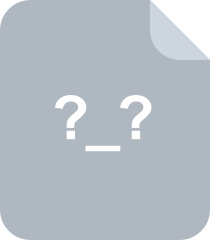
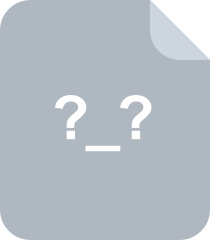
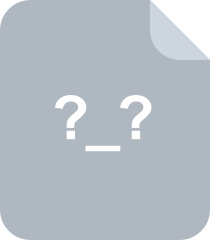
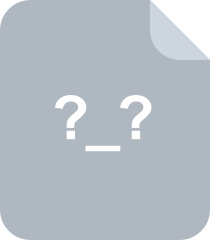
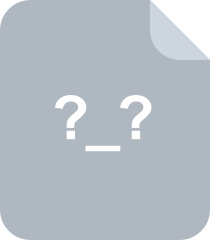
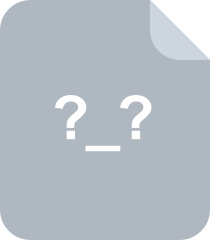
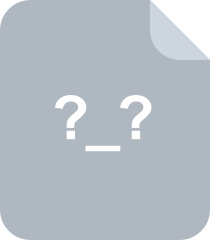
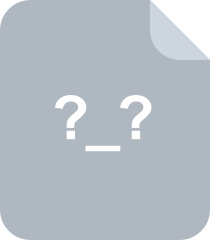
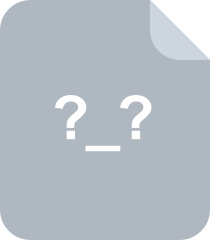
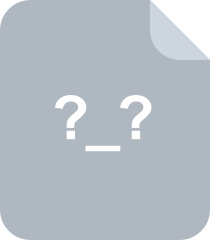
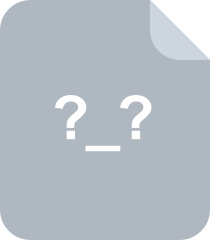
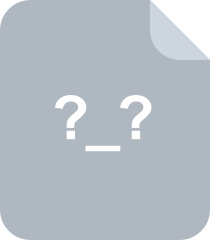
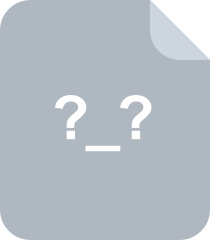
- 1
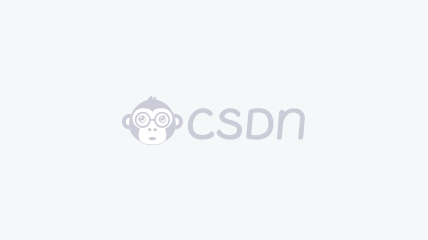
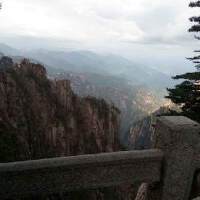
- 粉丝: 86
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

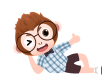
最新资源
- 软考 2024系统规划与管理师考试官方教材
- RabbitMQ练习(Hello World)抓包文件
- openstack 创建虚拟机的接口
- Java项目:台球室计费管理系统(java+SSM+JSP+HTML+JavaScript+mysql)
- Java项目:台球室计费管理系统(java+SSM+JSP+HTML+JavaScript+mysql)
- Java项目:台球室计费管理系统(java+SSM+JSP+HTML+JavaScript+mysql)
- 简单易用的stb-image图像库
- i2pinstall_0.9.18_windows (1).zip
- 9301ff42a3ad6b9f291c5a15f506b637.pdf
- 小米9高通9008免权限救砖刷机教程+工具

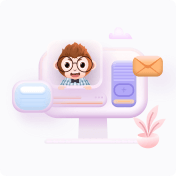
