/*
Copyright (c) 2012-2013, BogDan Vatra <bogdan@kde.org>
Contact: http://www.qt-project.org/legal
Commercial License Usage
Licensees holding valid commercial Qt licenses may use this file in
accordance with the commercial license agreement provided with the
Software or, alternatively, in accordance with the terms contained in
a written agreement between you and Digia. For licensing terms and
conditions see http://qt.digia.com/licensing. For further information
use the contact form at http://qt.digia.com/contact-us.
BSD License Usage
Alternatively, this file may be used under the BSD license as follows:
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions
are met:
1. Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package org.qtproject.qt5.android.bindings;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.io.InputStream;
import java.io.FileOutputStream;
import java.io.FileInputStream;
import java.io.DataOutputStream;
import java.io.DataInputStream;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Arrays;
import org.kde.necessitas.ministro.IMinistro;
import org.kde.necessitas.ministro.IMinistroCallback;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.content.ComponentName;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.ServiceConnection;
import android.content.pm.ActivityInfo;
import android.content.pm.PackageManager;
import android.content.pm.PackageManager.NameNotFoundException;
import android.content.pm.PackageInfo;
import android.content.res.Configuration;
import android.content.res.Resources.Theme;
import android.content.res.AssetManager;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.util.AttributeSet;
import android.util.Log;
import android.view.ContextMenu;
import android.view.ContextMenu.ContextMenuInfo;
import android.view.KeyEvent;
import android.view.Menu;
import android.view.MenuItem;
import android.view.MotionEvent;
import android.view.View;
import android.view.Window;
import android.view.WindowManager.LayoutParams;
import android.view.accessibility.AccessibilityEvent;
import dalvik.system.DexClassLoader;
public class QtActivity extends Activity
{
private final static int MINISTRO_INSTALL_REQUEST_CODE = 0xf3ee; // request code used to know when Ministro instalation is finished
private static final int MINISTRO_API_LEVEL = 4; // Ministro api level (check IMinistro.aidl file)
private static final int NECESSITAS_API_LEVEL = 2; // Necessitas api level used by platform plugin
private static final int QT_VERSION = 0x050100; // This app requires at least Qt version 5.1.0
private static final String ERROR_CODE_KEY = "error.code";
private static final String ERROR_MESSAGE_KEY = "error.message";
private static final String DEX_PATH_KEY = "dex.path";
private static final String LIB_PATH_KEY = "lib.path";
private static final String LOADER_CLASS_NAME_KEY = "loader.class.name";
private static final String NATIVE_LIBRARIES_KEY = "native.libraries";
private static final String ENVIRONMENT_VARIABLES_KEY = "environment.variables";
private static final String APPLICATION_PARAMETERS_KEY = "application.parameters";
private static final String BUNDLED_LIBRARIES_KEY = "bundled.libraries";
private static final String BUNDLED_IN_LIB_RESOURCE_ID_KEY = "android.app.bundled_in_lib_resource_id";
private static final String BUNDLED_IN_ASSETS_RESOURCE_ID_KEY = "android.app.bundled_in_assets_resource_id";
private static final String MAIN_LIBRARY_KEY = "main.library";
private static final String STATIC_INIT_CLASSES_KEY = "static.init.classes";
private static final String NECESSITAS_API_LEVEL_KEY = "necessitas.api.level";
/// Ministro server parameter keys
private static final String REQUIRED_MODULES_KEY = "required.modules";
private static final String APPLICATION_TITLE_KEY = "application.title";
private static final String MINIMUM_MINISTRO_API_KEY = "minimum.ministro.api";
private static final String MINIMUM_QT_VERSION_KEY = "minimum.qt.version";
private static final String SOURCES_KEY = "sources"; // needs MINISTRO_API_LEVEL >=3 !!!
// Use this key to specify any 3rd party sources urls
// Ministro will download these repositories into their
// own folders, check http://community.kde.org/Necessitas/Ministro
// for more details.
private static final String REPOSITORY_KEY = "repository"; // use this key to overwrite the default ministro repsitory
private static final String ANDROID_THEMES_KEY = "android.themes"; // themes that your application uses
public String APPLICATION_PARAMETERS = null; // use this variable to pass any parameters to your application,
// the parameters must not contain any white spaces
// and must be separated with "\t"
// e.g "-param1\t-param2=value2\t-param3\tvalue3"
public String ENVIRONMENT_VARIABLES = "QT_USE_ANDROID_NATIVE_STYLE=1\tQT_USE_ANDROID_NATIVE_DIALOGS=1\t";
// use this variable to add any environment variables to your application.
// the env vars must be separated with "\t"
// e.g. "ENV_VAR1=1\tENV_VAR2=2\t"
// Currently the following vars are used by the android plugin:
// * QT_USE_ANDROID_NATIVE_STYLE - 1 to use the android widget style if available.
// * QT_USE_ANDROID_NATIVE_DIALOGS -1 to use the android native dialogs.
public String[] QT_ANDROID_THEMES = null; // A list with all themes that your application want to use.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本产品是一款组态软件。通过本软件可以实现工业数据的采集与监控。在电力系统于电力系统、给水系统、石油、化工等领域的数据采集与监视控制以及过程控制等诸多领域,快速构建工业自动控制系统监控是一项重要需求。组态软件是自动控制系统监控层级的软件平台,使用灵活,部署方便,可以便捷地完成数据的采集与过程控制。基于此种需要,开发了本软件。(1)主要功能列表 1.图形绘制:绘制 点、线、圆、矩形、圆弧、扇形、弦、任意多边形等基本。 2.图形属性设置:图形的形状、颜色、位置的改变等。 3.编辑操作:剪切,复制,粘贴、删除,撤销  重做。 4.文件操作:打开、保存、关闭、另存等。 (2)产品特色 1.操作简单,易于掌握,上手即会。
资源详情
资源评论
资源推荐
收起资源包目录

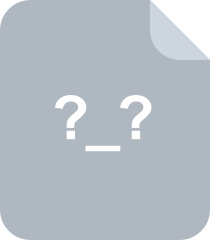
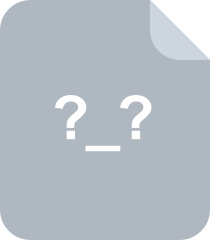
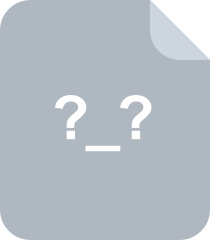
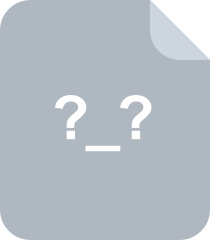
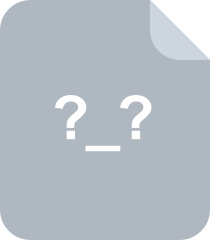
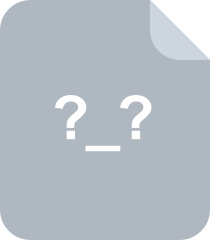
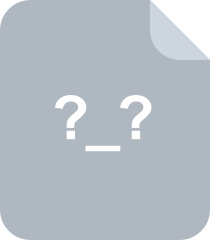
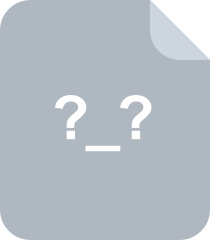
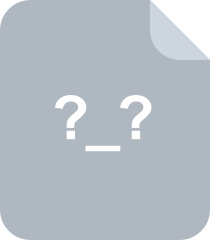
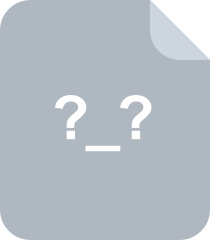
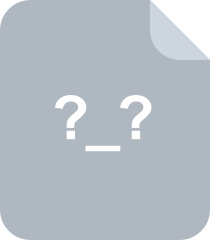
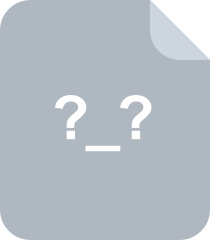
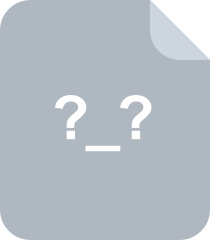
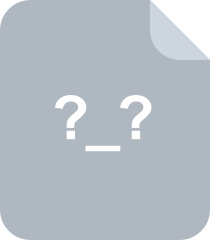
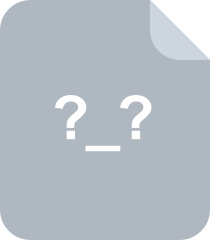
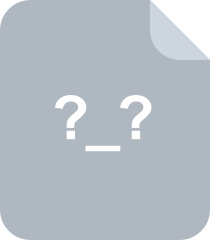
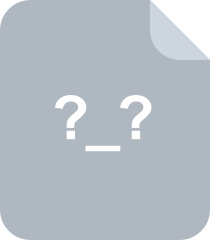
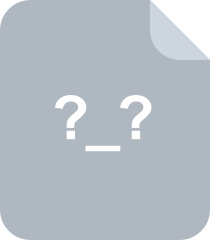
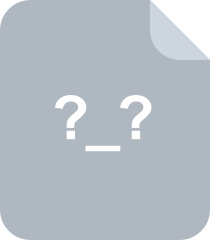
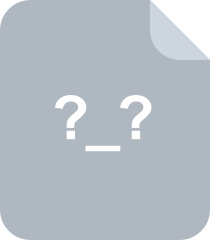
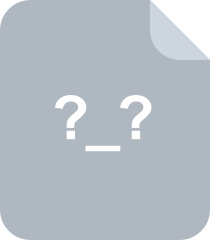
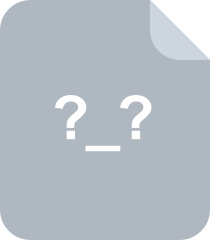
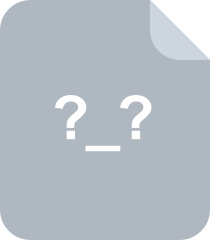
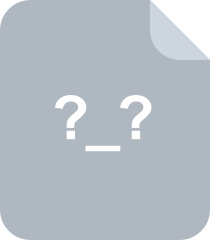
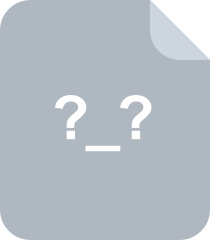
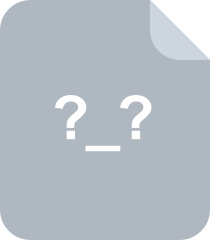
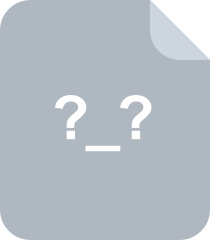
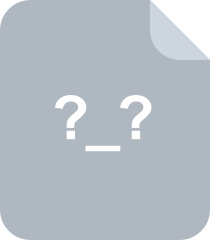
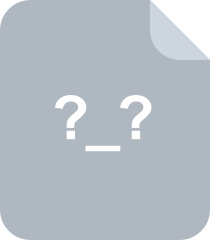
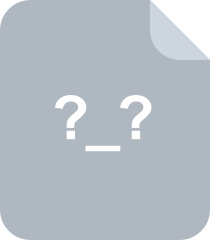
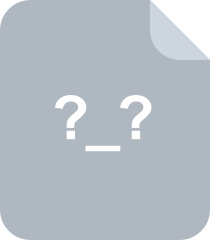
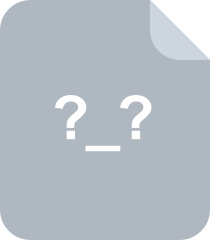
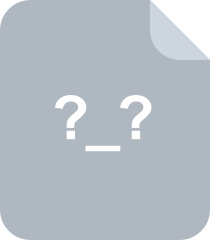
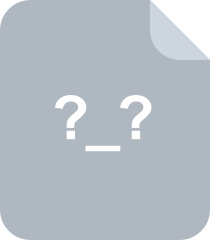
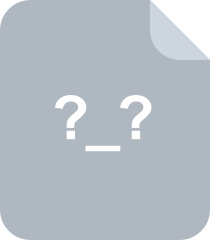
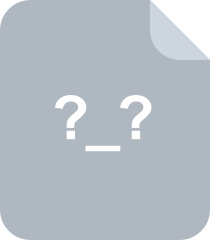
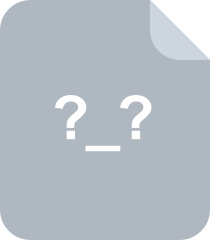
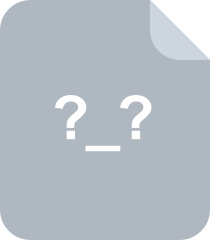
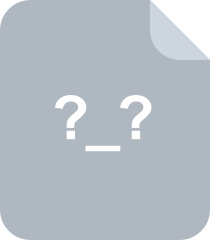
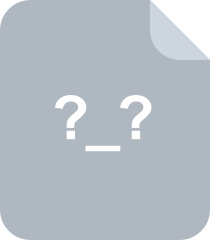
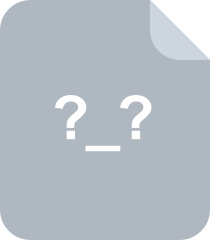
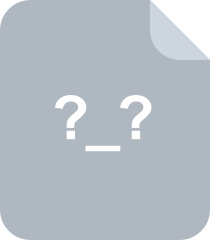
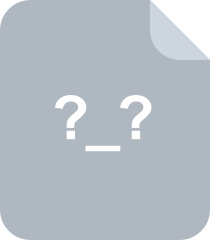
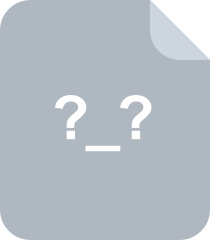
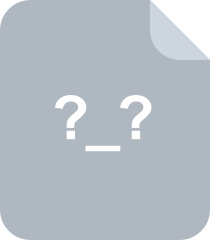
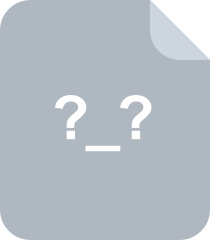
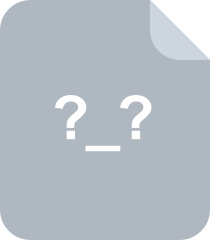
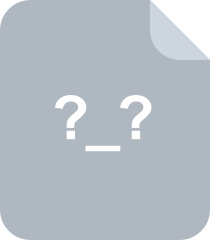
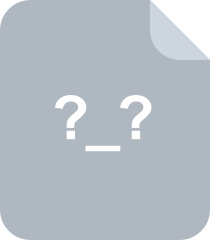
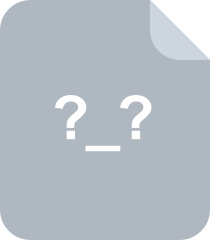
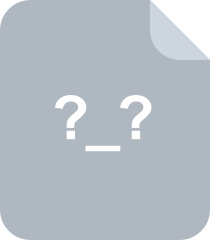
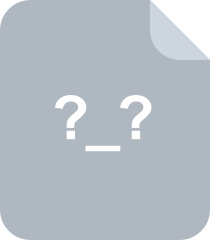
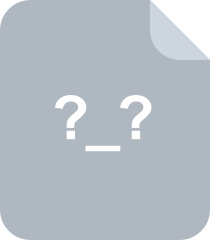
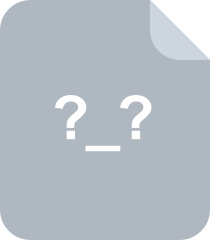
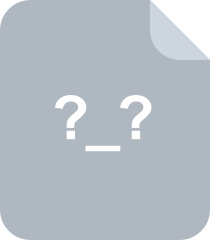
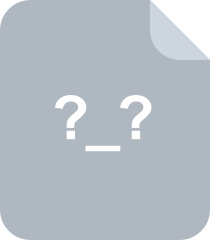
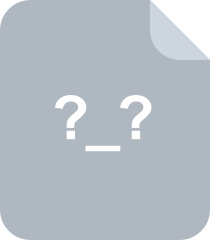
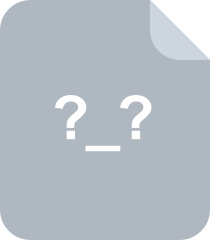
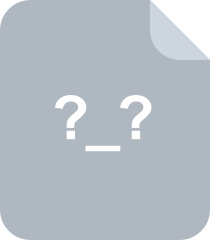
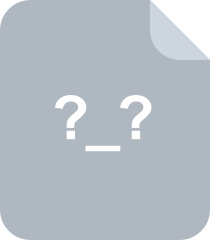
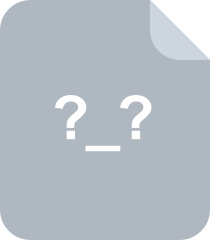
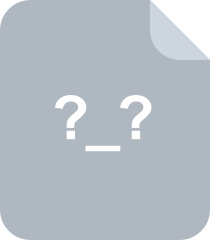
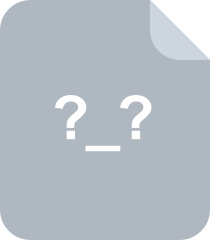
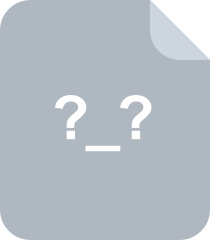
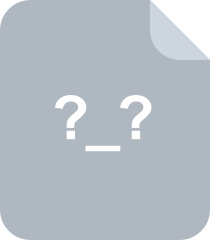
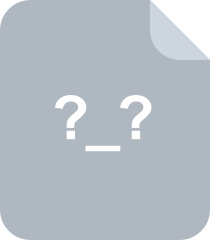
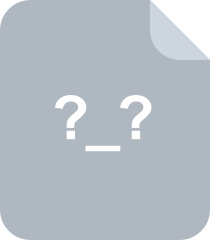
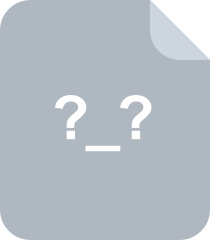
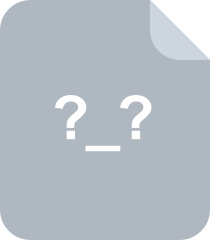
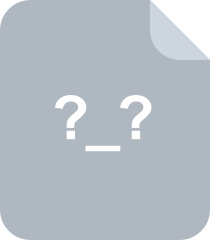
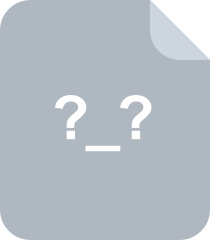
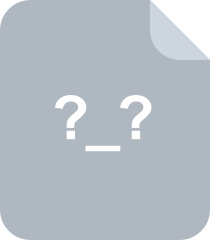
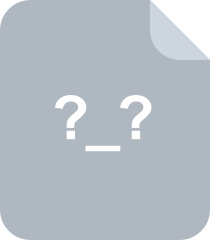
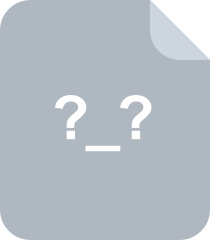
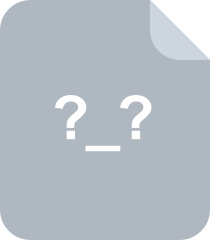
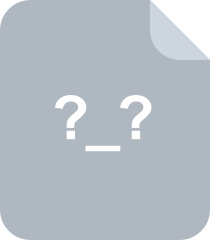
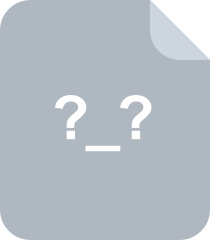
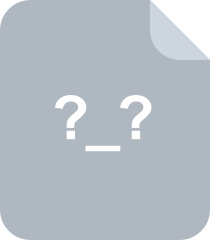
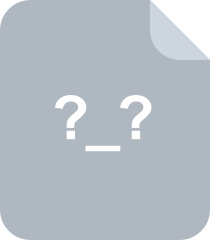
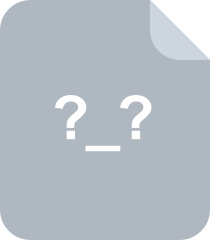
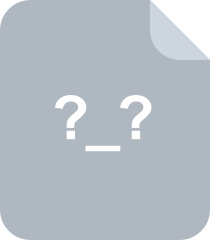
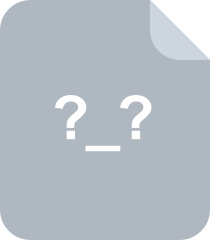
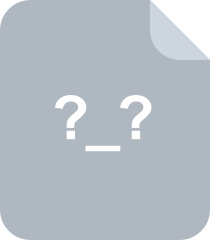
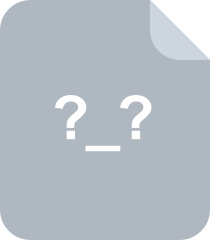
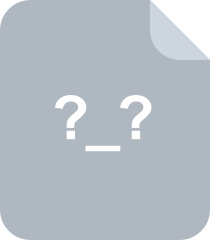
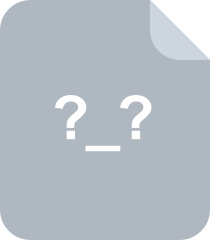
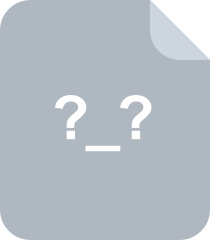
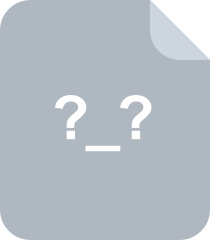
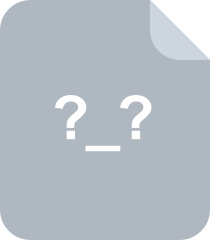
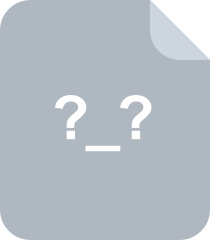
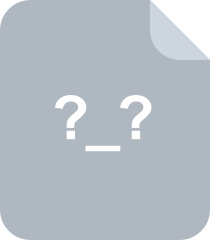
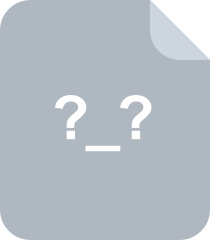
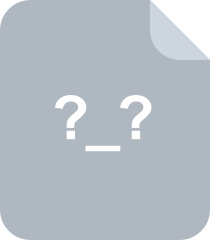
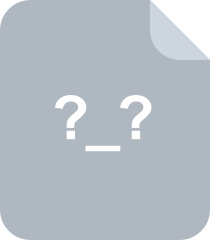
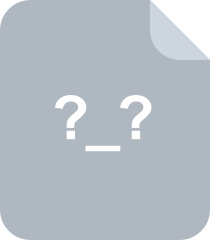
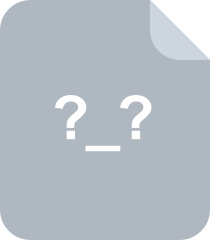
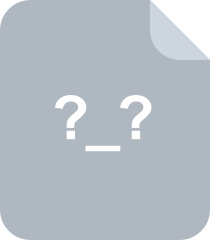
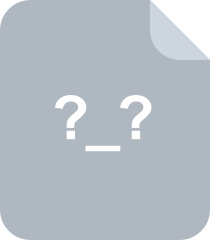
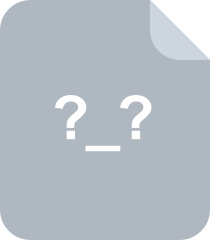
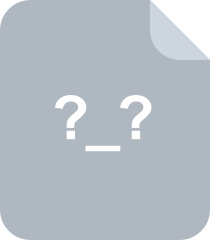
共 954 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
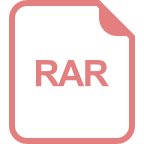
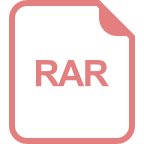
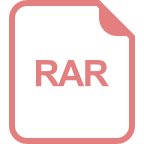
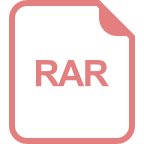
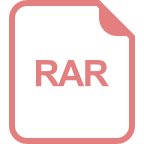
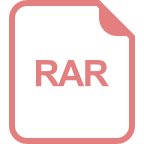
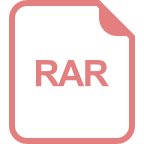
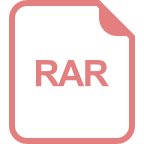
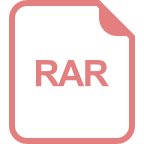
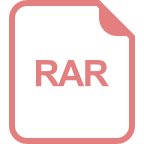
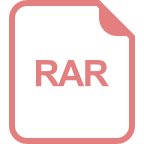
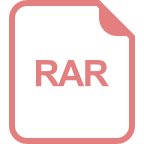
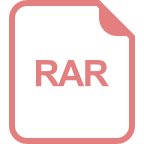
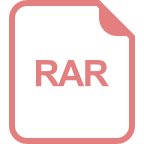
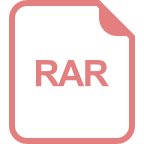
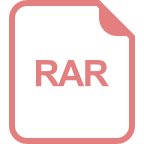
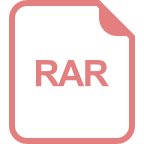
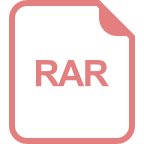
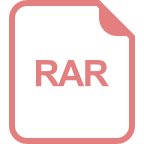
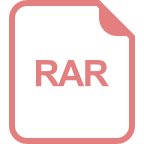
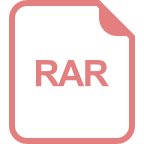
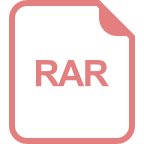
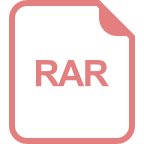
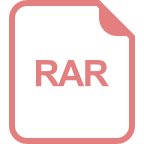
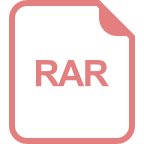
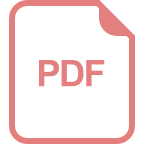
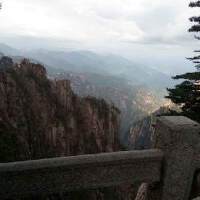
weixin_42651887
- 粉丝: 104
- 资源: 1万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

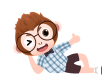
最新资源
- SIMULINK 基于反推控制速度控制器的永磁同步电机控制系统研究
- springboot037基于SpringBoot的墙绘产品展示交易平台的设计与实现.zip
- springboot239华府便利店信息管理系统_0303173844.zip
- springboot239华府便利店信息管理系统.zip
- springboot038基于SpringBoot的网上租赁系统设计与实现.zip
- 关于AUTOSAR组织结构的介绍ppt
- 三菱FX3U与台达变频器通讯 器件:三菱FX3U PLC+F X3U 485BD板,台达VFD变频器,昆仑通态触摸屏 功能:采用485方式,modbus RTU协议,对台达变频器频率设定,正反转,点动
- springboot040社区医院信息平台.zip
- springboot039基于Web足球青训俱乐部管理后台系统开发.zip
- springboot240基于Spring boot的名城小区物业管理系统.zip
- springboot041师生健康信息管理系统.zip
- springboot242基于SpringBoot的失物招领平台的设计与实现.zip
- 基于三菱PLC和组态王供暖控制系统热器控制 带解释的梯形图程序,接线图原理图图纸,io分配,组态画面
- springboot241基于SpringBoot+Vue的电商应用系统的设计与实现.zip
- C++、基于OpenCV和MFC框架的口罩缺陷检测.zip
- springboot042IT技术交流和分享平台的设计与实现.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


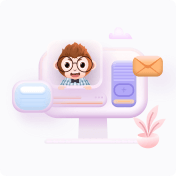
安全验证
文档复制为VIP权益,开通VIP直接复制

评论11