import java.awt.*;
import java.awt.event.*;
import java.sql.*;
import java.awt.List;
import javax.swing.*;
public class Tongxunlu extends WindowAdapter implements ActionListener,ItemListener{
JLabel name,sex,age,email,depart,tel,addr;
JFrame f;
JTextField tfname,tfemail,tftel,tfaddr;
Choice csex,cage,cdepart;
List ls1;
JButton b1,b2,b3,b4,b5;
Panel p1,p2,p3,p4,p5;
public void display(){
f=new JFrame("我的通讯录");
f.setSize(560,400);
f.setLocation(200,140);
f.setBackground(Color.lightGray);
f.setLayout(new GridLayout(2,1));
ls1 =new List();
ls1.addItemListener(this);
f.add(ls1);
p1=new Panel(new GridLayout(6,2));
name=new JLabel("姓名");
tfname=new JTextField("",20);
tftel = new JTextField("",20);
addr =new JLabel("住址");
tfaddr =new JTextField("");
sex = new JLabel("性别");
csex = new Choice();
csex.add("男");
csex.add("女");
age = new JLabel("年龄");
cage = new Choice();
for(int i=0;i<100;i++)
cage.addItem(String.valueOf(i));
email = new JLabel("电子邮件");
tfemail =new JTextField("",20);
p1.add(name);
p1.add(tfname);
p1.add(sex); p1.add(csex);
p1.add(age);
p1.add(cage);
p1.add(email);
p1.add(tfemail);
p2=new Panel(new GridLayout(6,2));
tel = new JLabel("电话");
depart = new JLabel("职业");
cdepart = new Choice();
cdepart.add("学生");
cdepart.add("农民");
cdepart.add("工人");
cdepart.add("老师");
cdepart.add("IT人员");
cdepart.add("其他");
p2.add(depart);
p2.add(cdepart);
p2.add(tel);
p2.add(tftel);p2.add(addr);
p2.add(tfaddr);
p3 = new Panel(new GridLayout(1,2));
p3.add(p1);
p3.add(p2);
b1 = new JButton("添加");
b2 = new JButton("删除");
b3 = new JButton("查询");
b4 = new JButton("清除");
b5 = new JButton("显示");
b1.addActionListener(this);
b2.addActionListener(this);
b3.addActionListener(this);
b4.addActionListener(this);
b5.addActionListener(this);
p4 = new Panel(new FlowLayout(FlowLayout.CENTER,20,5));
p1.add(b1);p1.add(b2);p1.add(b3);p1.add(b4);p4.add(b5);
p5 = new Panel(new BorderLayout());
p5.add(p3,BorderLayout.CENTER);
p5.add(p4,BorderLayout.SOUTH);
f.add(p5);
f.addWindowListener(this);
f.setVisible(true);
}
public void windowClosing(WindowEvent e){
System.exit(0);
}
public void actionPerformed(ActionEvent e){
String sql;
Connection conn;
Statement stmt;
ResultSet rs;
String str, strname;
if(e.getSource()==b1){
try{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
String url="jdbc:odbc:MyDatabase";
String user="sa";
String password="beibisiwofhsa";
conn= DriverManager.getConnection(url,user,password);
stmt=conn.createStatement();
strname=tfname.getText();
sql="select * from stu where name='"+strname+"'";
rs=stmt.executeQuery(sql);
if(!rs.next()){
sql="insert into stu(name,sex,age,email,depart,tel,addr)";
sql=sql+" values('"+strname+"','"+csex.getSelectedItem()+"','"+
cage.getSelectedItem()+"','"+tfemail.getText()+"','"+
cdepart.getSelectedItem()+"','"+tftel.getText()+"','"+tfaddr.getText()+"')";
stmt.execute(sql);
str = tfname.getText()+" ";
str = str + " "+csex.getSelectedItem()+" ";
str = str + " "+cage.getSelectedItem();
str = str+" "+tfemail.getText();
str = str + " "+cdepart.getSelectedItem();
str = str+" "+tftel.getText()+" "+tfaddr.getText();
ls1.add(str);
}
else
JOptionPane.showMessageDialog(f,"用户名已存在");
}
catch(Exception l){
l.printStackTrace();
}
}
if(e.getSource()==b2){
if(ls1.getSelectedIndex()!=0){
ls1.remove(ls1.getSelectedIndex());
}
try{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
String url="jdbc:odbc:MyDatabase";
String user="sa";
String password="beibisiwofhsa";
conn= DriverManager.getConnection(url,user,password);
stmt=conn.createStatement();
strname=tfname.getText();
sql="delete stu where name='"+strname+"'";
stmt.executeUpdate(sql);
}
catch(Exception l){
l.printStackTrace();
}
}
if(e.getSource()==b3){
try {
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
String url="jdbc:odbc:MyDatabase";
String user="sa";
String password="beibisiwofhsa";
conn= DriverManager.getConnection(url,user,password);
stmt=conn.createStatement();
rs=stmt.executeQuery(sql="select * from stu where name='"+tfname.getText()+"'");
while(rs.next()) {
str=rs.getString(1)+" "+rs.getString(2)+" "+rs.getString(3)+" "+rs.getString(4)+" "+rs.getString(5)+" "+
rs.getString(6)+" "+rs.getString(7)+" ";
ls1.add(str);
}
rs.close();
stmt.close();
conn.close();
}
catch(Exception a){
a.printStackTrace();
}
}
if(e.getSource()==b4){
ls1.removeAll();
tfname.setText("");
tftel.setText("");
tfaddr.setText("");
tfemail.setText("");
}
if(e.getSource()==b5){
try {
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
String url="jdbc:odbc:MyDatabase";
String user="sa";
String password="beibisiwofhsa";
conn= DriverManager.getConnection(url,user,password);
stmt=conn.createStatement();
sql="select * from stu";
rs=stmt.executeQuery(sql);
while(rs.next()) {
str=rs.getString(1)+" "+rs.getString(2)+" "+rs.getString(3)+" "+rs.getString(4)+" "+rs.getString(5)+" "+rs.getString(6)+" "+rs.getString(7)+" ";
ls1.add(str);
}
rs.close();
stmt.close();
conn.close();
}
catch(Exception a){
a.printStackTrace();
}
}
}
public void itemStateChanged(ItemEvent e){
System.out.println("12");
if(e.getSource()==ls1){
String strtemp = ls1.getItem(ls1.getSelectedIndex());
System.out.println(strtemp);
}
}
public static void main (String[] args){
(new Tongxunlu()).display();
}
}

JonSco
- 粉丝: 94
- 资源: 1万+
最新资源
- 基于springboot的G县乡村生活垃圾治理问题中运输地图的设计与实现源码(java毕业设计完整源码).zip
- 基于Vue框架的房东租客入住管理小程序设计源码
- 基于Java语言的FreeMarker模板引擎自动代码生成设计源码
- 基于Freemarker的MyBatis代码生成器设计源码
- 基于springboot的Java Move体育商城源码(java毕业设计完整源码).zip
- 基于springboot的Java Offer资讯交流Web系统源码(java毕业设计完整源码).zip
- 基于JavaScript的MediaWiki InPageEdit插件设计源码
- 基于springboot的Java“课件通”中小学教学课件共享平台源码(java毕业设计完整源码).zip
- 基于Vue3 + TypeScript的VTJ低代码开发工具设计源码
- 基于springboot的Java共享经济背景下校园闲置物品交易平台源码(java毕业设计完整源码).zip
- 基于Qt Creator与CMake的OpenCV C++示例设计源码
- 基于Vue+Webpack+Element+Axios+vueRouter的快餐店收银系统设计源码
- 基于Vue3和Tailwind的Ruoyi-APP动态菜单设计源码
- 基于Python、API和wxauto库的微信自动脚本发送趋势图设计源码
- 基于springboot的Java大学生心理咨询平台源码(java毕业设计完整源码).zip
- 基于Kotlin语言开发的企业进销存管理系统设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


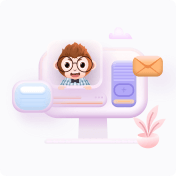
评论0