#include<iostream>
using namespace std;
char Seats[101][100];
void B(int line,int start,int end);
void R(int line,int start,int end);
void F(int line,int start,int end);
int main()
{
char Operation;
int i,j,line,start,end;
for(i=0;i<101;i++)
for(j=0;j<100;j++)
Seats[i][j]='=';
while(cin>>Operation)
{
switch(Operation)
{
case 'B':
cin>>line>>start>>end;
B(line,start,end);
break;
case 'R':
cin>>line>>start>>end;
R(line,start,end);
break;
case 'F':
cin>>line>>start>>end;
F(line,start,end);
break;
case 'E':
return 0;
}
}
return 0;
}
void B(int line,int start,int end)
{
for(int i=start-1;i<end;i+=2)
Seats[line-1][i]='X';
}
void R(int line,int start,int end)
{
for(int i=start-1;i<end;i+=2)
Seats[line-1][i]='=';
}
void F(int line,int start,int end)
{
for(int i=start-1;i<end;i+=2)
cout<<Seats[line-1][i];
cout<<endl;
}
#include "stdafx.h"
//包含头文件以支持多线程
#include "windows.h"
#include "stdio.h"
//用于标志所有的子线程是否结束
//每次子线程结束后,此值便加1。
static long ThreadCompleted = 0;
//互斥量
HANDLE mutex;
//信号量,用于生产者通知消费者
HANDLE full;
//信号量,用于消费者通知生产者
HANDLE empty;
//信号量,当所有的子线程结束后,通知主线程,可以结束。
HANDLE evtTerminate;
//生产标志
#define p_item 1
//消费标志
#define c_item 0
//哨兵
#define END 3
//缓冲区最大长度
const int max_buf_size=4;
const int cur_size=3;
//缓冲区定义
int BUFFER[max_buf_size];
//放消息指针
int in=0;
//取消息指针
int out=0;
int front=0;
int tail=0;
int sleep_time=1000;
//线程函数的标准格式
unsigned long __stdcall p_Thread(void *theBuf);
unsigned long __stdcall c_Thread(void *theBuf);
//打印缓冲区内容
void PrintBuf(int buf[],int buf_size);
int main(int argc, char* argv[])
{
//初始化缓冲区
unsigned long TID1, TID2;
for(int i=0;i<cur_size;i++)
BUFFER[i]=0;
//互斥量和信号量的创建,函数用法可查看MSDN
mutex=CreateMutex(NULL,false,"mutex");
full=CreateSemaphore(NULL,0,1,"full");
empty=CreateSemaphore(NULL,max_buf_size,max_buf_size,"empty");
evtTerminate = CreateEvent(NULL, FALSE, FALSE, "Terminate");
//创建一个生产者线程和消费者线程。作为本程序的扩展,你可以增加线程的数目
CreateThread(NULL,0,p_Thread,BUFFER,NULL,&TID1);
CreateThread(NULL,0,c_Thread,BUFFER,NULL,&TID2);
//等待各子线程的结束
WaitForSingleObject(evtTerminate, INFINITE);
return 0;
}
//生产者线程函数
unsigned long __stdcall p_Thread(void *theBuf)
{
while(true)
{
Sleep(sleep_time);
//可以不要此函数,只是为了模拟缓冲区满的情况
if((front+1)%max_buf_size==tail)
{
printf("The buffer is full!And the product thread is blocked!\n");
sleep_time+=3000;
}
WaitForSingleObject(empty,INFINITE);//先申请信号量
WaitForSingleObject(mutex,INFINITE);//再申请互斥量
/*进入临界区*/
printf("I am producting,please waiting.....\n");
BUFFER[in]=p_item;
in=(in+1)%cur_size;
front=(front+1)%max_buf_size;
PrintBuf(BUFFER,cur_size);
printf("I am ready for exiting.\n");
//退出临界区
ReleaseMutex(mutex);
ReleaseSemaphore(full,1,0);
}
//线程结束后,将ThreadCompleted值加1
InterlockedIncrement(&ThreadCompleted);
if(ThreadCompleted ==2) SetEvent(evtTerminate);
return 0;
}
//消费者线程函数
unsigned long __stdcall c_Thread(void *theBuf)
{
while(true)
{
Sleep(3000);
if(front==tail)
{
printf("The buffer is empty!\n");
sleep_time-=3000;
}
//P操作
WaitForSingleObject(full,INFINITE);
WaitForSingleObject(mutex,INFINITE);
printf("A consumer has enter the critiction .\n");
BUFFER[out]=c_item;
out=(out+1)%cur_size;
tail=(tail+1)%max_buf_size;
PrintBuf(BUFFER,cur_size);
printf("the consumer has exit the critiction.\n");
//V操作
ReleaseMutex(mutex);
ReleaseSemaphore(empty,1,0);
}
//同生产者进程
InterlockedIncrement(&ThreadCompleted);
if(ThreadCompleted ==2) SetEvent(evtTerminate);
return 0;
}
//打印缓冲区
void PrintBuf(int buf[],int buf_size)
{
int i;
printf("The state of the buffer:\n");
for(i=0;i<buf_size;i++)
printf("%d,",buf[i]);
printf("\n");
Sleep(1000);
//此函数是为了线程相临两次访问临界区时有序的打印缓冲区内容,
//并不是必须的,你可以不要,但模拟的近似度就大打折扣了。
}

JonSco
- 粉丝: 94
- 资源: 1万+
最新资源
- 毕设和企业适用springboot企业资源规划类及在线学习平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及智慧安防系统源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及企业云管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及智能医疗监测系统源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及智能城市数据管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及智慧社区管理平台源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及数字营销平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车电商类及城市智能管理系统源码+论文+视频.zip
- 毕设和企业适用springboot汽车电商类及城市智能运营平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车电商类及广告效果评估平台源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及网络营销平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车电商类及跨境电商管理平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车电商类及教学资源共享平台源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及云端储物管理系统源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及在线教育管理系统源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及智能会议管理平台源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


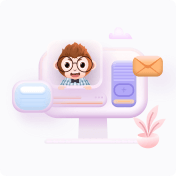