WebServer_Java.rar_java webserver_javawebserver开发
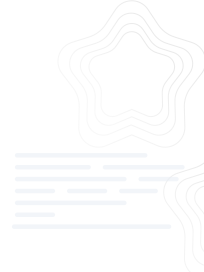

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
Java WebServer是一个基于Java语言实现的简易Web服务器,主要用于演示HTTP协议的基本工作原理以及服务器端的编程技术。这个项目可能适合初学者理解Web服务器的工作机制,或者作为学习网络编程和IO处理的一个实践案例。 在Java WebServer的实现中,主要涉及以下几个核心知识点: 1. **Java IO**:Java IO是实现Web服务器的基础,它负责读取静态资源文件(如HTML)并将其发送到客户端。服务器需要使用`FileInputStream`读取文件内容,然后通过`Socket`的`OutputStream`将数据流发送出去。同时,服务器还需要处理客户端的请求,这涉及到对输入流的读取和解析,可能使用到`BufferedReader`和`PrintWriter`等类。 2. **HTTP协议**:WebServer需要理解HTTP协议的基本概念,如请求方法(GET、POST等)、状态码(200、404等)、请求头和响应头等。开发者需要根据接收到的HTTP请求来构建合适的HTTP响应。 3. **多线程**:为了处理并发请求,Java WebServer通常会为每个连接创建一个新的线程。这是因为Java的Socket API是阻塞式的,当一个线程正在处理请求时,其他请求必须等待。因此,使用`Thread`或`ExecutorService`来管理线程池是非常重要的,以提高服务器的性能和资源利用率。 4. **URL映射与路由**:在实际的Web服务器中,URL与服务器上的资源进行映射是必要的。虽然这个简单的Java WebServer可能只处理根目录下的HTML文件,但在更复杂的服务器中,需要能够解析URL路径并将其映射到相应的处理逻辑或静态文件。 5. **错误处理**:对于无法找到的资源或服务器内部错误,WebServer需要返回适当的HTTP错误代码,例如404(Not Found)或500(Internal Server Error)。这可以通过自定义异常处理机制来实现。 6. **性能优化**:尽管这是一个简单的实现,但考虑到实际应用中的性能需求,可以考虑缓存静态资源、使用非阻塞IO(如NIO或Netty框架)、压缩输出内容等优化策略。 `www.pudn.com.txt`可能是服务器开发过程中的一个文档,记录了项目的相关信息或者是一些参考资料。而`WebServer`可能是服务器的源代码,包含了实现上述功能的类和方法。深入研究这个源代码,可以更好地理解上述知识点的实际应用,并且可以动手修改和扩展,提升自己的编程技能。 这个Java WebServer项目是一个很好的学习平台,可以帮助我们理解网络编程的核心概念,提升Java IO操作和多线程编程的能力,同时也让我们有机会实践HTTP协议的实现。
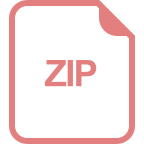
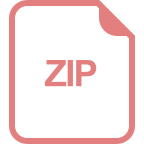
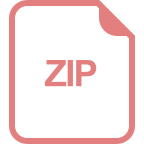
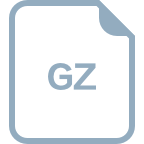
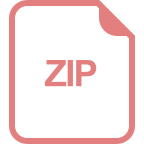
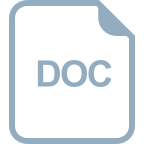
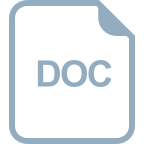
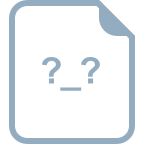
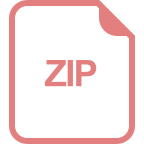
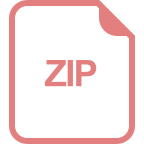
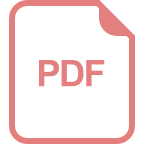
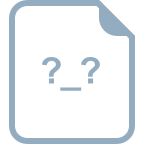
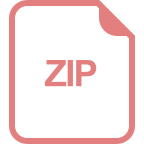

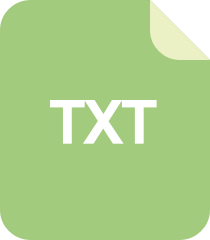


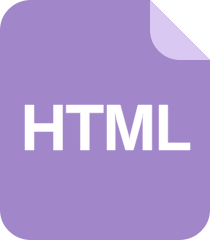

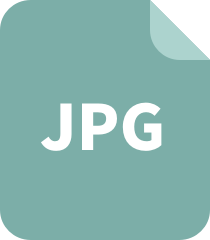
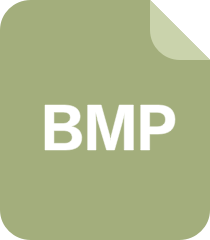
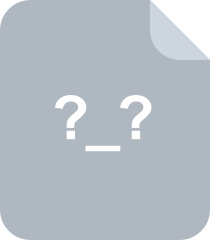
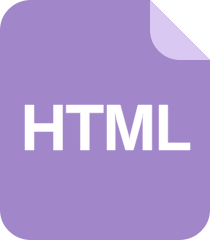
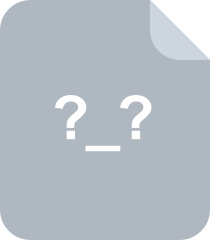
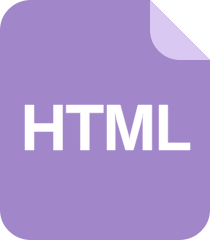
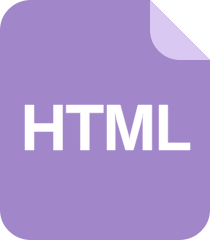

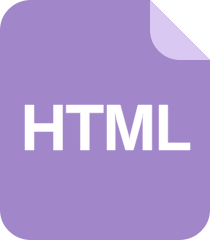

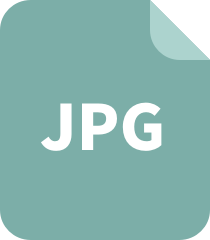
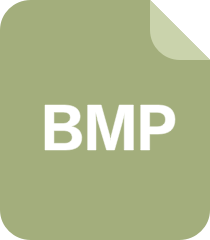
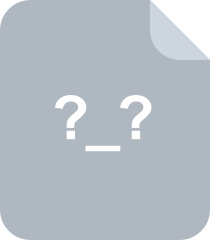
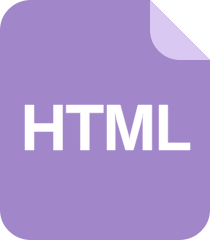
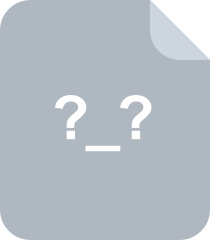
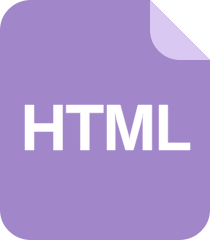
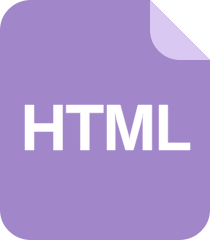
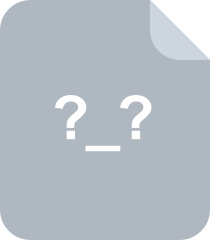
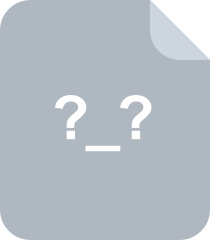
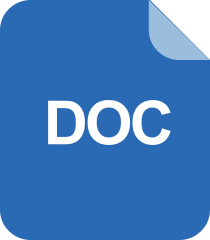
- 1
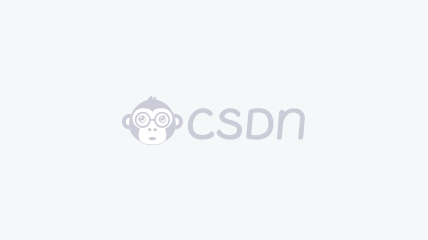

- 粉丝: 94
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

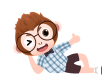
最新资源
- “高一”期中家长会教案课件模板.pptx
- “教育的智慧”读书分享会教案课件模板.pptx
- “相约七夕”节日介绍教案课件模板.pptx
- 2023-04-06-项目笔记 - 第三百五十八阶段 - 4.4.2.356全局变量的作用域-356 -2025.12.25
- 2023-4-8-笔记-第一阶段-第2节-分支循环语句- 4.goto语句 5.本章完 -2024.12.25
- 车辆机械设计基础_实验指导书.docx
- Origin教程008:热图所需练习数据
- Origin教程009所需练习数据
- PCle AI加速卡在医疗影像分析中的应用.docx
- PCle AI加速卡在智能制造中的应用.docx
- PCle AI加速卡在智能城市交通管理系统中的应用.docx
- PCle AI加速卡在金融交易系统中的应用.docx
- PCle AI加速卡在智能零售系统中的应用.docx
- PCle AI加速卡在自动驾驶系统中的应1.docx
- PCle AI加速卡在自动驾驶系统中的应用.docx
- PCle AI加速卡在智能推荐系统中的应用.docx

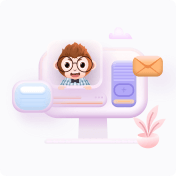
