《JDOM与XML解析:深度比较与应用》 XML(eXtensible Markup Language)是一种通用的数据交换格式,被广泛应用于网络数据交换、配置文件存储等领域。为了方便地解析和操作XML文档,Java社区开发了多种库,其中JDOM和DOM4J是两个常用的选择。本文将对JDOM和XML的解析进行详细介绍,并进行两者之间的比较。 **JDOM** JDOM(Java Document Object Model)是Java平台上的一个XML处理库,它提供了一种直接在Java对象层次上操作XML文档的方法。JDOM的主要优势在于其完全基于Java,因此在性能和效率上有较好的表现,同时提供了丰富的API,使得开发者能够便捷地创建、修改和读取XML文档。 1. **JDOM的结构** JDOM的结构基于XML文档的层次结构,包括Element(元素)、Attribute(属性)、Text(文本)、CDATASection(CDATA区域)等核心类。这些类对应XML文档中的各个部分,开发者可以通过它们构建和操作XML文档。 2. **JDOM解析XML** JDOM提供了SAXBuilder和DOMBuilder两种方式解析XML文档。SAXBuilder基于事件驱动的SAX解析器,适用于大文件,内存消耗较小;DOMBuilder则将整个XML文档加载到内存中,形成DOM树,适合小规模的XML文档。 3. **JDOM构建XML** 使用JDOM创建XML文档,可以通过Element的子类化来定义自定义的元素类型,然后通过添加子元素、属性和文本内容来构建XML结构。 **DOM4J** DOM4J是另一个流行的Java XML处理库,它不仅实现了DOM API,还提供了SAX和StAX的集成,以及XPath的支持。DOM4J的设计目标是提供一种灵活且易于使用的XML处理工具。 1. **DOM4J的特点** DOM4J以其灵活性和易用性著称,它提供了强大的文档操作功能,如元素的添加、删除和修改,以及XPath查询。此外,DOM4J还支持XML Schema和DTD。 2. **DOM4J解析XML** DOM4J支持SAX和DOM两种解析方式,同时也支持StAX,可以根据需求选择最合适的解析策略。 3. **DOM4J构建XML** DOM4J提供了类似JDOM的API,但更加简洁和直观,允许开发者通过Element、Attribute等类创建和操作XML文档。同时,DOM4J的XPath支持使得通过表达式查找和修改XML节点变得非常方便。 **JDOM与DOM4J的比较** 1. **性能** 在内存消耗方面,DOM4J由于提供了更多功能,可能会比JDOM消耗更多资源。而SAXBuilder在JDOM中通常用于处理大型XML文档,以减少内存占用。 2. **易用性** DOM4J通常被认为比JDOM更易于学习和使用,因为它的API设计更加直观,且提供了XPath支持。 3. **功能** DOM4J功能更全面,如XML Schema支持和XPath查询,而JDOM专注于基本的XML操作。 4. **社区支持** 两者都有活跃的社区,但DOM4J因为其广泛的应用,可能拥有更丰富的第三方插件和教程资源。 总结,JDOM和DOM4J都是强大的XML处理工具,各有优缺点。对于简单的需求,JDOM可能更为适用;而对于需要XPath查询或更复杂操作的情况,DOM4J可能是更好的选择。开发者应根据项目需求和团队熟悉程度来决定使用哪一个库。
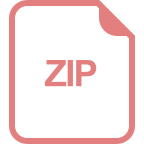
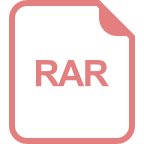
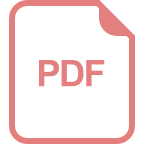
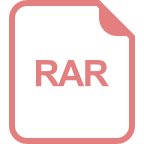
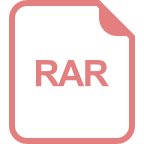
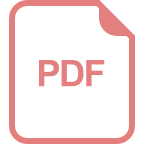
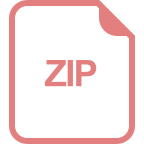
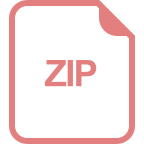
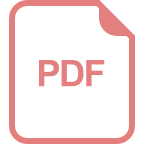
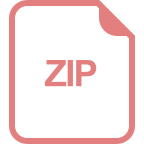





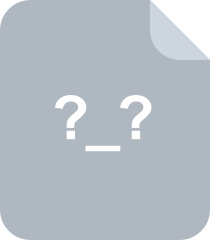
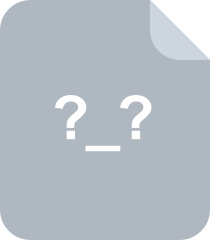

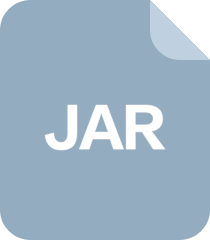
- 1
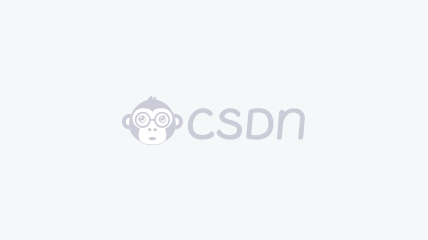

- 粉丝: 85
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

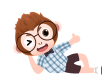
最新资源
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip
- (源码)基于计算机系统原理与Arduino技术的学习平台.zip
- (源码)基于SSM框架的大学消息通知系统服务端.zip
- (源码)基于Java Servlet的学生信息管理系统.zip
- (源码)基于Qt和AVR的FestosMechatronics系统终端.zip

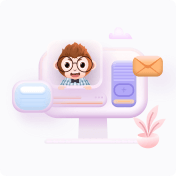
