#include<stdio.h>
#include<stdlib.h>
typedef struct {
char * buf;
int head;
int tail;
int size;
} fifo_t;
//This initializes the FIFO structure with the given buffer and size
void fifo_init(fifo_t * f, char * buf, int size){
f->head = 0;
f->tail = 0;
f->size = size;
f->buf = buf;
}
//This reads nbytes bytes from the FIFO
//The number of bytes read is returned
int fifo_read(fifo_t * f, void * buf, int nbytes){
int i;
char * p;
p = buf;
for(i=0; i < nbytes; i++){
if( f->tail != f->head ){ //see if any data is available
*p++ = f->buf[f->tail]; //grab a byte from the buffer
f->tail++; //increment the tail
if( f->tail == f->size ){ //check for wrap-around
f->tail = 0;
}
} else {
return i; //number of bytes read
}
}
return nbytes;
}
//This writes up to nbytes bytes to the FIFO
//If the head runs in to the tail, not all bytes are written
//The number of bytes written is returned
int fifo_write(fifo_t * f, const void * buf, int nbytes){
int i;
const char * p;
p = buf;
for(i=0; i < nbytes; i++){
//first check to see if there is space in the buffer
if( (f->head + 1 == f->tail) || ( (f->head+1 == f->size) && (f->tail == 0) )){
return i; //no more room
} else {
f->buf[f->head] = *p++;
f->head++; //increment the head
if( (f->head == f->size)){ //check for wrap-around
f->head = 0;
}
}
}
return nbytes;
}
int main(){
fifo_t f;
char wr_buffer[10]={'a','n','v','s','d'};
char rd_buffer[10];
char * fifo_buffer;
int size, nb_wr, nb_rd, i;
printf("donner la taille du buffer :\n");
scanf("%d",&size);
fifo_buffer = malloc(size * sizeof(char));
fifo_init(&f, fifo_buffer, size);
nb_wr = fifo_write(&f, wr_buffer, 4);
nb_rd = fifo_read(&f, rd_buffer, 6);
printf("\n nombre de trames ecrites : \t %d \n",nb_wr);
for(i=0;i<nb_wr;i++){
printf("\t - trame %d : %c \n", i, f.buf[i]);
}
printf("\n nombre de trames lues : \t %d \n",nb_rd);
for(i=0;i<nb_rd;i++){
printf("\t - trame %d : %c \n", i, rd_buffer[i]);
}
}

小波思基
- 粉丝: 86
- 资源: 1万+
最新资源
- NSKeyValueObservationException如何解决.md
- 基于Java的环境保护与宣传网站论文.doc
- 前端开发中的JS快速排序算法原理及实现方法
- 常见排序算法概述及其性能比较
- 形状分类31-YOLO(v5至v11)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- 2018年最新 ECshop母婴用品商城新版系统(微商城+微分销+微信支付)
- BookShopTuto.zip
- 论文复现:结合 CNN 和 LSTM 的滚动轴承剩余使用寿命预测方法
- MySQL中的数据库管理语句-ALTER USER.pdf
- 冒泡排序算法解析及优化.md
- 2024年智算云市场发展与生态分析报告
- qwewq23132131231
- 《木兰诗》教学设计.docx
- 《台阶》教学设计.docx
- 《卖油翁》文言文教学方案.docx
- 《老王》教学设计方案.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


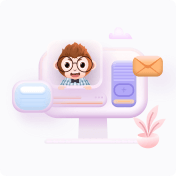