// SnmpManagerDlg.cpp : implementation file
//
#include "stdafx.h"
#include "SnmpManager.h"
#include "SnmpManagerDlg.h"
#include "dlgset.h"
#include "dlgipin.h"
#include "dlgprint.h"
#ifdef _DEBUG
//CMemoryState oldMemState,newMemState,diffMemState;
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CAboutDlg dialog used for App About
class CAboutDlg : public CDialog
{
public:
CAboutDlg();
// Dialog Data
//{{AFX_DATA(CAboutDlg)
enum { IDD = IDD_ABOUTBOX };
//}}AFX_DATA
// ClassWizard generated virtual function overrides
//{{AFX_VIRTUAL(CAboutDlg)
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
//}}AFX_VIRTUAL
// Implementation
protected:
//{{AFX_MSG(CAboutDlg)
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD)
{
//{{AFX_DATA_INIT(CAboutDlg)
//}}AFX_DATA_INIT
}
void CAboutDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CAboutDlg)
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)
//{{AFX_MSG_MAP(CAboutDlg)
// No message handlers
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CSnmpManagerDlg dialog
CSnmpManagerDlg::CSnmpManagerDlg(CWnd* pParent /*=NULL*/)
: CDialog(CSnmpManagerDlg::IDD, pParent)
{
//{{AFX_DATA_INIT(CSnmpManagerDlg)
m_sAgent = _T("");
m_sIpin = _T("");
m_sIpout = _T("");
m_sName = _T("");
m_sDesr = _T("");
m_sSysOid = _T("");
m_sSysTime = _T("");
//}}AFX_DATA_INIT
// Note that LoadIcon does not require a subsequent DestroyIcon in Win32
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
void CSnmpManagerDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CSnmpManagerDlg)
DDX_Text(pDX, IDC_EDIT1, m_sAgent);
DDX_Text(pDX, IDC_IPIN, m_sIpin);
DDX_Text(pDX, IDC_IPOUT, m_sIpout);
DDX_Text(pDX, IDC_SYSNAME, m_sName);
DDX_Text(pDX, IDC_SYSDESR, m_sDesr);
DDX_Text(pDX, IDC_SYSOID, m_sSysOid);
DDX_Text(pDX, IDC_SYSUPTIME, m_sSysTime);
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CSnmpManagerDlg, CDialog)
//{{AFX_MSG_MAP(CSnmpManagerDlg)
ON_WM_SYSCOMMAND()
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
ON_BN_CLICKED(IDC_ADDAGENT, OnAddagent)
ON_LBN_SELCHANGE(IDC_LIST1, OnSelchangeList1)
ON_MESSAGE(wMsg,OnRecv)
ON_WM_TIMER()
ON_BN_CLICKED(IDC_END, OnEnd)
ON_BN_CLICKED(IDC_SET, OnSet)
ON_BN_CLICKED(IDC_GETNEXT, OnGetnext)
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CSnmpManagerDlg message handlers
BOOL CSnmpManagerDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Add "About..." menu item to system menu.
// IDM_ABOUTBOX must be in the system command range.
ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);
ASSERT(IDM_ABOUTBOX < 0xF000);
CMenu* pSysMenu = GetSystemMenu(FALSE);
if (pSysMenu != NULL)
{
CString strAboutMenu;
strAboutMenu.LoadString(IDS_ABOUTBOX);
if (!strAboutMenu.IsEmpty())
{
pSysMenu->AppendMenu(MF_SEPARATOR);
pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);
}
}
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
for(int i=0;i<=9;i++)
{
m_initOid[i]=new char[100];
}
m_initOid[1]="1.3.6.1.2.1.1.1.0";
m_initOid[2]="1.3.6.1.2.1.1.2.0";
m_initOid[3]="1.3.6.1.2.1.1.3.0";
m_initOid[4]="1.3.6.1.2.1.1.5.0";
m_initOid[5]="1.3.6.1.2.1.4.3.0";
m_initOid[6]="1.3.6.1.2.1.4.10.0";
CListBox* pList=(CListBox *)GetDlgItem(IDC_LIST1);
pList->AddString("127.0.0.1");
for(i=0;i<=9;i++ )
{
m_value[i]=new smiVALUE[100];
m_sOid[i]=new char[100];
str[i]=new char[100];
}
return TRUE; // return TRUE unless you set the focus to a control
}
void CSnmpManagerDlg::OnSysCommand(UINT nID, LPARAM lParam)
{
if ((nID & 0xFFF0) == IDM_ABOUTBOX)
{
CAboutDlg dlgAbout;
dlgAbout.DoModal();
}
else
{
CDialog::OnSysCommand(nID, lParam);
}
}
// If you add a minimize button to your dialog, you will need the code below
// to draw the icon. For MFC applications using the document/view model,
// this is automatically done for you by the framework.
void CSnmpManagerDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND, (WPARAM) dc.GetSafeHdc(), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
// The system calls this to obtain the cursor to display while the user drags
// the minimized window.
HCURSOR CSnmpManagerDlg::OnQueryDragIcon()
{
return (HCURSOR) m_hIcon;
}
void CSnmpManagerDlg::OnAddagent()
{
UpdateData(TRUE);
CListBox* pList=(CListBox *)GetDlgItem(IDC_LIST1);
pList->AddString(m_sAgent);
}
void CSnmpManagerDlg::OnSelchangeList1()
{
//KillTimer(1);
m_Agent=new char;
CListBox* pList=(CListBox *)GetDlgItem(IDC_LIST1);
pList->GetText(pList->GetCurSel(),m_Agent);
Start();
m_preIn=m_nIpin;
SetTimer(2,1000,NULL);
}
void CSnmpManagerDlg::Start()
{ //oldMemState.Checkpoint();
//oldMemState.DumpStatistics();
if(pSnmp.sessionID==FALSE)
{
pSnmp.CreateSession(CSnmpManagerDlg::m_hWnd,wMsg);
pSnmp.sessionID=TRUE;
}
//pSnmp.Register();
pSnmp.CreateVbl(m_initOid[1],NULL);
for(int i=2;i<=6;i++)
{
pSnmp.SetVbl(m_initOid[i]);
}
pSnmp.CreatePdu(SNMP_PDU_GET,NULL,NULL,NULL);
pSnmp.Send(m_Agent,"public");
/*#ifdef _DEBUG
newMemState.Checkpoint();
if(diffMemState.Difference(oldMemState,newMemState))
{TRACE("diffrence between fire and noe\n\n");
diffMemState.DumpStatistics();
}
TRACE("new:\n\n\n\n");
newMemState.DumpStatistics();
#endif*/
}
void CSnmpManagerDlg::OnRecv()
{
int nIpin;
CString strIp;
CString strTemp;
pSnmp.Receive(m_sOid,m_value);
for(int i=1;i<=pSnmp.nCount;i++)
{
switch(m_value[i]->syntax)
{
case SNMP_SYNTAX_INT:
//case SNMP_SYNTAX_INT32:
smiINT sNumber;
sNumber=m_value[i]->value.sNumber;
nIpin=sNumber;
wsprintf(str[i],"%d",sNumber);
break;
case SNMP_SYNTAX_UINT32:
case SNMP_SYNTAX_CNTR32:
case SNMP_SYNTAX_GAUGE32:
case SNMP_SYNTAX_TIMETICKS:
smiUINT32 uNumber;
uNumber=m_value[i]->value.uNumber;
nIpin=uNumber;
wsprintf(str[i],"%d",uNumber);
break;
case SNMP_SYNTAX_CNTR64:
smiCNTR64 hNumber;
hNumber=m_value[i]->value.hNumber;
break;
case SNMP_SYNTAX_OCTETS:
case SNMP_SYNTAX_OPAQUE:
case SNMP_SYNTAX_NSAPADDR:
str[i]=(char *)m_value[i]->value.string.ptr;
break;
case SNMP_SYNTAX_IPADDR:
strIp.Format("%d",*m_value[i]->value.string.ptr);
strIp+=".";
strTemp.Format("%d",*(m_value[i]->value.string.ptr+1));
strIp+=strTemp;
strIp+=".";
strTemp.Format("%d",*(m_value[i]->value.string.ptr+2));
strIp+=strTemp;
strIp+=".";
strTemp.Format("%d",*(m_value[i]->value.string.ptr+3));
strIp+=strTemp;
strcpy(str[i],strIp);
break;
case SNMP_SYNTAX_OID:
smiOID oid;
oid=m_value[i]->value.oid;
SnmpOidToStr(&oid,30,str[i]);
//SnmpFreeDescriptor (SNMP_SYNTAX_OID, (smiLPOPAQUE)&oid);
break;
case SNMP_SYNTAX_NULL:
case SNMP_SYNTAX_NOSUCHOBJECT:
case SNMP_SYNTAX_NOSUCHINSTANCE:
case SNMP_SYNTAX_ENDOFMIBVIEW:
smiBYTE empty;
empty=m_value[i]->value.empty;
str[i]="N

小波思基
- 粉丝: 86
- 资源: 1万+
最新资源
- 基于matlab的FFT分析和滤波程序,可对数据信号进行频谱分析,分析波形中所含谐波分量,并可以对特定频率波形进行提取 不需要通过示波器观察,直接导入数据即可,快捷便利 程序带有详细注释, 图a为
- 基于Springboot+Vue的精简博客系统的设计与实现-毕业源码案例设计(源码+论文).zip
- 基于Springboot+Vue交通管理在线服务系统的开发-毕业源码案例设计(95分以上).zip
- uDDS源程序publisher
- 机械手自动排列控制PLC与触摸屏程序设计
- 基于Springboot+Vue的客户关系管理系统(crm)的设计与实现-毕业源码案例设计(高分毕业设计).zip
- 基于Springboot+Vue的课程作业管理系统毕业源码案例设计(高分毕业设计).zip
- 基于Springboot+Vue的酒店客房管理系统-毕业源码案例设计(源码+数据库).zip
- (链家)上海市房屋租赁价格数据.zip
- ESP8266-调试.pdf
- 基于STM32设计的工地扬尘与噪音实时监测系统(网页).pdf
- 基于Springboot+Vue的库存管理系统-毕业源码案例设计(高分毕业设计).zip
- 基于Springboot+Vue的老年人体检管理系统-毕业源码案例设计(高分毕业设计).zip
- 基于Springboot+Vue的乐享田园系统-毕业源码案例设计(95分以上).zip
- 基于Springboot+Vue的流浪宠物管理系统的设计与实现-毕业源码案例设计(95分以上).zip
- 基于Springboot+Vue的论坛系统-毕业源码案例设计(高分项目).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


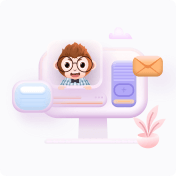
评论0